Ekos::Capture
#include <capture.h>
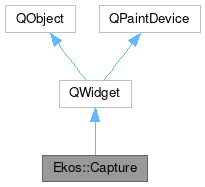
Signals | |
void | abortFocus (const QString &trainname) |
void | adaptiveFocus (const QString &trainname) |
void | captureAborted (double exposureSeconds) |
Q_SCRIPTABLE void | captureComplete (const QVariantMap &metadata, const QString &trainname) |
void | captureStarting (double exposureSeconds, const QString &filter) |
void | captureTarget (QString targetName) |
void | checkFocus (double, const QString &opticaltrain) |
void | dither () |
void | driverTimedout (const QString &deviceName) |
void | dslrInfoRequested (const QString &cameraName) |
void | filterManagerUpdated (ISD::FilterWheel *device) |
Q_SCRIPTABLE void | guideAfterMeridianFlip () |
Q_SCRIPTABLE void | meridianFlipStarted (const QString &trainname) |
void | newDownloadProgress (double, const QString &trainname) |
void | newExposureProgress (SequenceJob *job, const QString &trainname) |
void | newFilterStatus (FilterState state) |
void | newImage (SequenceJob *job, const QSharedPointer< FITSData > &data, const QString &trainname) |
void | newLocalPreview (const QString &preview) |
Q_SCRIPTABLE void | newLog (const QString &text) |
Q_SCRIPTABLE void | newStatus (CaptureState status, const QString &trainname, int cameraID) |
void | ready () |
void | resetFocusFrame (const QString &trainname) |
void | resetNonGuidedDither () |
void | resumeGuiding () |
void | runAutoFocus (AutofocusReason autofocusReason, const QString &reasonInfo, const QString &trainname) |
void | sequenceChanged (const QJsonArray &sequence) |
void | settingsUpdated (const QVariantMap &settings) |
void | suspendGuiding () |
void | trainChanged () |
Public Slots | |
Q_SCRIPTABLE Q_NOREPLY void | abort (QString train="") |
Q_SCRIPTABLE QString | getTargetName () |
Q_SCRIPTABLE Q_NOREPLY void | pause () |
Q_SCRIPTABLE Q_NOREPLY void | restartCamera (const QString &name) |
Q_SCRIPTABLE Q_NOREPLY void | setObserverName (const QString &value) |
Q_SCRIPTABLE Q_NOREPLY void | setTargetName (const QString &newTargetName) |
Q_SCRIPTABLE QString | start (QString train="") |
Q_SCRIPTABLE Q_NOREPLY void | stop (CaptureState targetState=CAPTURE_IDLE) |
Q_SCRIPTABLE Q_NOREPLY void | suspend () |
Q_SCRIPTABLE Q_NOREPLY void | toggleSequence () |
Q_SCRIPTABLE Q_NOREPLY void | toggleVideo (bool enabled) |
Public Member Functions | |
void | appendLogText (const QString &) |
Q_SCRIPTABLE QString | camera () |
QSharedPointer< Camera > & | camera (int i) |
const QList< QSharedPointer< Camera > > & | cameras () const |
void | checkCloseCameraTab (int tabIndex) |
Q_SCRIPTABLE Q_NOREPLY void | clearAutoFocusHFR () |
Q_SCRIPTABLE void | clearLog () |
Q_SCRIPTABLE Q_NOREPLY void | clearSequenceQueue () |
Q_SCRIPTABLE QString | filter () |
Q_SCRIPTABLE QString | filterWheel () |
int | findCamera (QString train, bool addIfNecessary) |
void | focusAdaptiveComplete (bool success, const QString &trainname) |
Q_SCRIPTABLE int | getActiveJobID () |
Q_SCRIPTABLE int | getActiveJobRemainingTime () |
Q_SCRIPTABLE int | getJobCount () |
Q_SCRIPTABLE double | getJobExposureDuration (int id) |
Q_SCRIPTABLE double | getJobExposureProgress (int id) |
Q_SCRIPTABLE QString | getJobFilterName (int id) |
Q_SCRIPTABLE CCDFrameType | getJobFrameType (int id) |
Q_SCRIPTABLE int | getJobImageCount (int id) |
Q_SCRIPTABLE int | getJobImageProgress (int id) |
Q_SCRIPTABLE QString | getJobPlaceholderFormat () |
Q_SCRIPTABLE QString | getJobPreviewFileName () |
Q_SCRIPTABLE QString | getJobState (int id) |
Q_SCRIPTABLE QString | getLogText () |
Q_SCRIPTABLE QString | getObserverName () |
Q_SCRIPTABLE int | getOverallRemainingTime () |
Q_SCRIPTABLE int | getPendingJobCount () |
Q_SCRIPTABLE double | getProgressPercentage () |
const QJsonArray & | getSequence () const |
Q_SCRIPTABLE QString | getSequenceQueueStatus () |
Q_SCRIPTABLE bool | hasCoolerControl () |
Q_SCRIPTABLE Q_NOREPLY void | ignoreSequenceHistory () |
void | inSequenceAFRequested (bool requested, const QString &trainname) |
Q_SCRIPTABLE bool | isActiveJobPreview () |
Q_SCRIPTABLE bool | loadSequenceQueue (const QString &fileURL, QString train="", bool isLead=true, QString targetName="") |
Q_SCRIPTABLE QStringList | logText () |
const QSharedPointer< Camera > | mainCamera () const |
Q_SCRIPTABLE QString | mainCameraDeviceName () |
QSharedPointer< CaptureModuleState > | moduleState () const |
QString | opticalTrain () const |
QSharedPointer< CameraProcess > | process () const |
void | registerNewModule (const QString &name) |
void | removeDevice (const QSharedPointer< ISD::GenericDevice > &device) |
Q_SCRIPTABLE bool | saveSequenceQueue (const QString &path) |
void | setAlignResults (double solverPA, double ra, double de, double pixscale) |
void | setAlignStatus (Ekos::AlignState newstate) |
Q_SCRIPTABLE Q_NOREPLY void | setCapturedFramesMap (const QString &signature, int count, QString train="") |
Q_SCRIPTABLE bool | setCoolerControl (bool enable) |
bool | setDome (ISD::Dome *device) |
Q_SCRIPTABLE bool | setFilter (const QString &filter) |
void | setFocusStatus (FocusState newstate, const QString &trainname) |
void | setFocusTemperatureDelta (double focusTemperatureDelta, double absTemperature, const QString &trainname) |
void | setGuideChip (ISD::CameraChip *guideChip) |
void | setGuideDeviation (double delta_ra, double delta_dec) |
void | setGuideStatus (GuideState newstate) |
void | setHFR (double newHFR, int position, bool inAutofocus, const QString &trainname) |
Q_SCRIPTABLE Q_NOREPLY void | setInSequenceFocus (bool enable, double HFR) |
Q_SCRIPTABLE Q_NOREPLY void | setMaximumGuidingDeviation (bool enable, double value) |
void | setMeridianFlipState (QSharedPointer< MeridianFlipState > newstate) |
void | setMountStatus (ISD::Mount::Status newState) |
void | setOpticalTrain (const QString &value) |
void | setupOptions () |
bool | setVideoLimits (uint16_t maxBufferSize, uint16_t maxPreviewFPS) |
Q_SCRIPTABLE CaptureState | status () |
void | updateCamera (int tabID, bool isValid) |
void | updateTargetDistance (double targetDiff) |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
virtual QSize | minimumSizeHint () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
virtual QSize | sizeHint () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
Public Attributes | |
OpsDslrSettings * | m_OpsDslrSettings { nullptr } |
OpsMiscSettings * | m_OpsMiscSettings { nullptr } |
![]() | |
DrawChildren | |
DrawWindowBackground | |
IgnoreMask | |
![]() | |
PdmDepth | |
PdmDevicePixelRatio | |
PdmDevicePixelRatioScaled | |
PdmDpiX | |
PdmDpiY | |
PdmHeight | |
PdmHeightMM | |
PdmNumColors | |
PdmPhysicalDpiX | |
PdmPhysicalDpiY | |
PdmWidth | |
PdmWidthMM | |
Additional Inherited Members | |
![]() | |
enum | RenderFlag |
typedef | RenderFlags |
![]() | |
typedef | QObjectList |
![]() | |
enum | PaintDeviceMetric |
![]() | |
QWidget * | createWindowContainer (QWindow *window, QWidget *parent, Qt::WindowFlags flags) |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
void | setTabOrder (std::initializer_list< QWidget * > widgets) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | changeEvent (QEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEnterEvent *event) |
virtual bool | event (QEvent *event) override |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | paintEvent (QPaintEvent *event) |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() |
Detailed Description
Captures single or sequence of images from a CCD.
The capture class support capturing single or multiple images from a CCD, it provides a powerful sequence queue with filter selection. Any sequence queue can be saved as Ekos Sequence Queue (.esq). All image capture operations are saved as Sequence Jobs that encapsulate all the different options in a capture process. The user may select in sequence autofocusing by setting limits for HFR, execution time or temperature delta. When the limit is exceeded, it automatically trigger autofocus operation. The capture process can also be linked with guide module. If guiding deviations exceed a certain threshold, the capture operation aborts until the guiding deviation resume to acceptable levels and the capture operation is resumed.
Controlling the capturing execution is a complex process, that is controlled by these classes:
- this class, that controll the UI and is the interface for all DBUS functions
- {
- See also
- CameraState} holds all state informations
- {
- See also
- CameraProcess} holds the business logic that controls the process For ore details about the capturing execution process, please visit {
- CameraProcess}.
- Version
- 1.4
Property Documentation
◆ camera
◆ coolerControl
◆ filter
◆ filterWheel
◆ logText
|
read |
◆ observerName
◆ opticalTrain
◆ status
|
read |
◆ targetName
Constructor & Destructor Documentation
◆ Capture()
Ekos::Capture::Capture | ( | ) |
Definition at line 52 of file capture.cpp.
Member Function Documentation
◆ appendLogText()
void Ekos::Capture::appendLogText | ( | const QString & | text | ) |
Definition at line 316 of file capture.cpp.
◆ camera()
QSharedPointer< Camera > & Ekos::Capture::camera | ( | int | i | ) |
Definition at line 437 of file capture.cpp.
◆ cameras()
|
inline |
◆ checkCloseCameraTab()
void Ekos::Capture::checkCloseCameraTab | ( | int | tabIndex | ) |
Definition at line 456 of file capture.cpp.
◆ clearLog()
void Ekos::Capture::clearLog | ( | ) |
Definition at line 326 of file capture.cpp.
◆ findCamera()
int Ekos::Capture::findCamera | ( | QString | train, |
bool | addIfNecessary ) |
find the camera using the given train
- Parameters
-
train optical train name addIfNecessary if true, add a new camera with the given train, if none uses this train
- Returns
- index in the lost of cameras (
- See also
- camera(int))
Definition at line 501 of file capture.cpp.
◆ focusAdaptiveComplete()
void Ekos::Capture::focusAdaptiveComplete | ( | bool | success, |
const QString & | trainname ) |
focusAdaptiveComplete Forward the new focus state to the capture module state machine
- Parameters
-
trainname name of the optical train to select the focuser
Definition at line 257 of file capture.cpp.
◆ getSequence()
|
inline |
◆ inSequenceAFRequested()
void Ekos::Capture::inSequenceAFRequested | ( | bool | requested, |
const QString & | trainname ) |
inSequenceAFRequested Focuser informs that the user wishes an AF run as soon as possible.
- Parameters
-
requested true iff AF is requested. trainname name of the optical train to select the focuser
Definition at line 606 of file capture.cpp.
◆ mainCamera()
const QSharedPointer< Camera > Ekos::Capture::mainCamera | ( | ) | const |
Definition at line 487 of file capture.cpp.
◆ moduleState()
|
inline |
◆ opticalTrain()
◆ process()
|
inline |
◆ registerNewModule()
void Ekos::Capture::registerNewModule | ( | const QString & | name | ) |
registerNewModule Register an Ekos module as it arrives via DBus and create the appropriate DBus interface to communicate with it.
- Parameters
-
name of module
Definition at line 213 of file capture.cpp.
◆ removeDevice()
void Ekos::Capture::removeDevice | ( | const QSharedPointer< ISD::GenericDevice > & | device | ) |
Generic method for removing any connected device.
Definition at line 585 of file capture.cpp.
◆ setAlignResults()
void Ekos::Capture::setAlignResults | ( | double | solverPA, |
double | ra, | ||
double | de, | ||
double | pixscale ) |
Definition at line 549 of file capture.cpp.
◆ setAlignStatus()
void Ekos::Capture::setAlignStatus | ( | Ekos::AlignState | newstate | ) |
Definition at line 372 of file capture.cpp.
◆ setDome()
bool Ekos::Capture::setDome | ( | ISD::Dome * | device | ) |
setDome Set dome device
- Parameters
-
device pointer to dome device
- Returns
- true if successfull, false otherewise.
Definition at line 208 of file capture.cpp.
◆ setFocusStatus()
void Ekos::Capture::setFocusStatus | ( | FocusState | newstate, |
const QString & | trainname ) |
setFocusStatus Forward the new focus state to the capture module state machine
- Parameters
-
trainname name of the optical train to select the focuser
Definition at line 249 of file capture.cpp.
◆ setFocusTemperatureDelta()
void Ekos::Capture::setFocusTemperatureDelta | ( | double | focusTemperatureDelta, |
double | absTemperature, | ||
const QString & | trainname ) |
setFocusTemperatureDelta update the focuser's temperature delta
- Parameters
-
trainname name of the optical train to select the focuser
Definition at line 332 of file capture.cpp.
◆ setGuideChip()
void Ekos::Capture::setGuideChip | ( | ISD::CameraChip * | guideChip | ) |
Definition at line 233 of file capture.cpp.
◆ setGuideDeviation()
void Ekos::Capture::setGuideDeviation | ( | double | delta_ra, |
double | delta_dec ) |
setGuideDeviation Set the guiding deviation as measured by the guiding module.
Abort capture if deviation exceeds user value. Resume capture if capture was aborted and guiding deviations are below user value.
- Parameters
-
delta_ra Deviation in RA in arcsecs from the selected guide star. delta_dec Deviation in DEC in arcsecs from the selected guide star.
Definition at line 341 of file capture.cpp.
◆ setGuideStatus()
void Ekos::Capture::setGuideStatus | ( | GuideState | newstate | ) |
Definition at line 378 of file capture.cpp.
◆ setHFR()
void Ekos::Capture::setHFR | ( | double | newHFR, |
int | position, | ||
bool | inAutofocus, | ||
const QString & | trainname ) |
setHFR Receive the measured HFR value of the latest frame
- Parameters
-
trainname name of the optical train to select the focuser
Definition at line 598 of file capture.cpp.
◆ setMeridianFlipState()
void Ekos::Capture::setMeridianFlipState | ( | QSharedPointer< MeridianFlipState > | newstate | ) |
Definition at line 558 of file capture.cpp.
◆ setMountStatus()
void Ekos::Capture::setMountStatus | ( | ISD::Mount::Status | newState | ) |
Definition at line 520 of file capture.cpp.
◆ setOpticalTrain()
|
inline |
◆ setVideoLimits()
bool Ekos::Capture::setVideoLimits | ( | uint16_t | maxBufferSize, |
uint16_t | maxPreviewFPS ) |
setVideoLimits sets the buffer size and max preview fps for live preview
- Parameters
-
maxBufferSize in bytes maxPreviewFPS number of frames per second
- Returns
- True if value is updated, false otherwise.
Definition at line 384 of file capture.cpp.
◆ updateCamera()
void Ekos::Capture::updateCamera | ( | int | tabID, |
bool | isValid ) |
Update the camera.
- Parameters
-
ID that holds the camera current camera is valid
Definition at line 183 of file capture.cpp.
◆ updateTargetDistance()
|
inline |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:47:17 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.