HoughLine
#include <houghline.h>
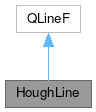
Public Types | |
enum | IntersectResult { PARALLEL , COINCIDENT , NOT_INTERESECTING , INTERESECTING } |
![]() | |
enum | IntersectionType |
Public Member Functions | |
HoughLine (double theta, double r, int width, int height, int score) | |
bool | DistancePointLine (const QPointF &point, QPointF &intersection, double &distance) |
double | getR () const |
int | getScore () const |
double | getTheta () const |
IntersectResult | Intersect (const HoughLine &other_line, QPointF &intersection) |
void | Offset (const int offsetX, const int offsetY) |
bool | operator< (const HoughLine &other) const |
HoughLine & | operator= (const HoughLine &other) |
void | printHoughLine () |
QPointF | RotatePoint (int x1, double r, double theta, int width, int height) |
void | setTheta (const double theta) |
![]() | |
QLineF (const QLine &line) | |
QLineF (const QPointF &p1, const QPointF &p2) | |
QLineF (qreal x1, qreal y1, qreal x2, qreal y2) | |
qreal | angle () const const |
qreal | angleTo (const QLineF &line) const const |
QPointF | center () const const |
qreal | dx () const const |
qreal | dy () const const |
IntersectionType | intersects (const QLineF &line, QPointF *intersectionPoint) const const |
bool | isNull () const const |
qreal | length () const const |
QLineF | normalVector () const const |
bool | operator!= (const QLineF &line) const const |
QDataStream & | operator<< (QDataStream &stream, const QLineF &line) |
bool | operator== (const QLineF &line) const const |
QDataStream & | operator>> (QDataStream &stream, QLineF &line) |
QPointF | p1 () const const |
QPointF | p2 () const const |
QPointF | pointAt (qreal t) const const |
void | setAngle (qreal angle) |
void | setLength (qreal length) |
void | setLine (qreal x1, qreal y1, qreal x2, qreal y2) |
void | setP1 (const QPointF &p1) |
void | setP2 (const QPointF &p2) |
void | setPoints (const QPointF &p1, const QPointF &p2) |
QLine | toLine () const const |
void | translate (const QPointF &offset) |
void | translate (qreal dx, qreal dy) |
QLineF | translated (const QPointF &offset) const const |
QLineF | translated (qreal dx, qreal dy) const const |
QLineF | unitVector () const const |
qreal | x1 () const const |
qreal | x2 () const const |
qreal | y1 () const const |
qreal | y2 () const const |
Static Public Member Functions | |
static bool | compareByScore (const HoughLine *line1, const HoughLine *line2) |
static bool | compareByTheta (const HoughLine *line1, const HoughLine *line2) |
static void | getSortedTopThreeLines (QVector< HoughLine * > &houghLines, QVector< HoughLine * > &top3Lines) |
![]() | |
QLineF | fromPolar (qreal length, qreal angle) |
Additional Inherited Members | |
![]() | |
BoundedIntersection | |
typedef | IntersectType |
NoIntersection | |
UnboundedIntersection | |
Detailed Description
Line representation for HoughTransform Based on the java implementation found on http://vase.essex.ac.uk/software/HoughTransform.
- Version
- 1.0
Definition at line 22 of file houghline.h.
Member Enumeration Documentation
◆ IntersectResult
enum HoughLine::IntersectResult |
Definition at line 25 of file houghline.h.
Constructor & Destructor Documentation
◆ HoughLine()
HoughLine::HoughLine | ( | double | theta, |
double | r, | ||
int | width, | ||
int | height, | ||
int | score ) |
Initialises the hough line.
Definition at line 16 of file houghline.cpp.
Member Function Documentation
◆ compareByScore()
Definition at line 72 of file houghline.cpp.
◆ compareByTheta()
Definition at line 77 of file houghline.cpp.
◆ DistancePointLine()
bool HoughLine::DistancePointLine | ( | const QPointF & | point, |
QPointF & | intersection, | ||
double & | distance ) |
Definition at line 134 of file houghline.cpp.
◆ getR()
double HoughLine::getR | ( | ) | const |
Definition at line 57 of file houghline.cpp.
◆ getScore()
int HoughLine::getScore | ( | ) | const |
Definition at line 52 of file houghline.cpp.
◆ getSortedTopThreeLines()
|
static |
Definition at line 160 of file houghline.cpp.
◆ getTheta()
double HoughLine::getTheta | ( | ) | const |
Definition at line 62 of file houghline.cpp.
◆ Intersect()
HoughLine::IntersectResult HoughLine::Intersect | ( | const HoughLine & | other_line, |
QPointF & | intersection ) |
Sources for intersection and distance calculations came from http://paulbourke.net/geometry/pointlineplane/ Also check https://doc.qt.io/archives/qt-4.8/qlinef.html for more line methods.
Definition at line 94 of file houghline.cpp.
◆ Offset()
void HoughLine::Offset | ( | const int | offsetX, |
const int | offsetY ) |
Definition at line 154 of file houghline.cpp.
◆ operator<()
|
inline |
Definition at line 52 of file houghline.h.
◆ operator=()
Definition at line 42 of file houghline.h.
◆ printHoughLine()
void HoughLine::printHoughLine | ( | ) |
Definition at line 82 of file houghline.cpp.
◆ RotatePoint()
QPointF HoughLine::RotatePoint | ( | int | x1, |
double | r, | ||
double | theta, | ||
int | width, | ||
int | height ) |
Definition at line 27 of file houghline.cpp.
◆ setTheta()
void HoughLine::setTheta | ( | const double | theta | ) |
Definition at line 67 of file houghline.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.