ISD::Camera
#include <indicamera.h>
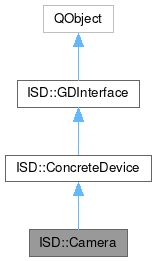
Public Types | |
enum | BlobType { BLOB_IMAGE , BLOB_FITS , BLOB_XISF , BLOB_RAW , BLOB_OTHER } |
enum | ErrorType { ERROR_CAPTURE , ERROR_SAVE , ERROR_LOAD , ERROR_VIEWER } |
enum | TelescopeType { TELESCOPE_PRIMARY , TELESCOPE_GUIDE , TELESCOPE_UNKNOWN } |
enum | UploadMode { UPLOAD_CLIENT , UPLOAD_REMOTE , UPLOAD_BOTH } |
Properties | |
bool | StreamingEnabled |
![]() | |
bool | connected |
QString | name |
![]() | |
objectName | |
Signals | |
void | closeVideoWindow () |
void | coolerToggled (bool enabled) |
void | error (ErrorType type) |
void | newBLOBManager (INDI::Property prop) |
void | newExposureValue (ISD::CameraChip *chip, double value, IPState state) |
void | newFPS (double instantFPS, double averageFPS) |
void | newGuideStarData (ISD::CameraChip *chip, double dx, double dy, double fit) |
void | newImage (const QSharedPointer< FITSData > &data, const QString &extension="") |
void | newRemoteFile (QString) |
void | newTemperatureValue (double value) |
void | newVideoFrame (const QSharedPointer< QImage > &frame) |
void | newView (const QSharedPointer< FITSView > &view) |
void | showVideoFrame (INDI::Property prop, int width, int height) |
void | updateVideoWindow (int width, int height, bool streamEnabled) |
void | videoRecordToggled (bool enabled) |
void | videoStreamToggled (bool enabled) |
![]() | |
void | Connected () |
void | Disconnected () |
void | propertyDefined (INDI::Property prop) |
void | propertyDeleted (INDI::Property prop) |
void | propertyUpdated (INDI::Property prop) |
void | ready () |
Public Slots | |
void | setBLOBManager (const char *device, INDI::Property prop) |
void | StreamWindowHidden () |
Public Member Functions | |
Camera (ISD::GenericDevice *parent) | |
bool | canCool () |
bool | configureRapidGuide (CameraChip *targetChip, bool autoLoop, bool sendImage=false, bool showMarker=false) |
QString | getCaptureFormat () const |
const QStringList & | getCaptureFormats () const |
CameraChip * | getChip (CameraChip::ChipType cType) |
const QString & | getEncodingFormat () const |
const QStringList & | getEncodingFormats () const |
const QMap< QString, double > & | getExposurePresets () const |
const QPair< double, double > | getExposurePresetsMinMax () const |
bool | getGain (double *value) |
bool | getGainMinMaxStep (double *min, double *max, double *step) |
IPerm | getGainPermission () const |
bool | getOffset (double *value) |
bool | getOffsetMinMaxStep (double *min, double *max, double *step) |
IPerm | getOffsetPermission () const |
bool | getSERNameDirectory (QString &filename, QString &directory) |
const QString & | getStreamEncoding () const |
const QStringList & | getStreamEncodings () const |
bool | getStreamExposure (double *duration) |
const QString & | getStreamRecording () const |
TelescopeType | getTelescopeType () |
bool | getTemperature (double *value) |
bool | getTemperatureRegulation (double &ramp, double &threshold) |
UploadMode | getUploadMode () |
const QStringList & | getVideoFormats () const |
bool | hasCooler () |
bool | hasCoolerControl () |
bool | hasGain () |
bool | hasGuideHead () |
bool | hasOffset () |
bool | hasVideoStream () |
bool | isBLOBEnabled () |
bool | isCoolerOn () |
bool | isFastExposureEnabled () const |
bool | isStreamingEnabled () |
bool | processBLOB (INDI::Property prop) override |
void | processNumber (INDI::Property prop) override |
void | processSwitch (INDI::Property prop) override |
void | processText (INDI::Property prop) override |
void | registerProperty (INDI::Property prop) override |
void | removeProperty (INDI::Property prop) override |
bool | resetStreamingFrame () |
bool | saveCurrentImage (QString &filename) |
bool | setBLOBEnabled (bool enable, const QString &prop=QString()) |
bool | setCaptureFormat (const QString &format) |
bool | setCoolerControl (bool enable) |
bool | setEncodingFormat (const QString &value) |
bool | setFastCount (uint32_t count) |
bool | setFastExposureEnabled (bool enable) |
bool | setFITSHeaders (const QList< FITSData::Record > &values) |
bool | setGain (double value) |
void | setNextSequenceID (int count) |
bool | setOffset (double value) |
bool | setRapidGuide (CameraChip *targetChip, bool enable) |
bool | setScopeInfo (double focalLength, double aperture) |
void | setSeqPrefix (const QString &preFix) |
bool | setSERNameDirectory (const QString &filename, const QString &directory) |
bool | setStreamEncoding (const QString &value) |
bool | setStreamExposure (double duration) |
bool | setStreamingFrame (int x, int y, int w, int h) |
bool | setStreamLimits (uint16_t maxBufferSize, uint16_t maxPreviewFPS) |
bool | setStreamRecording (const QString &value) |
bool | setTelescopeType (TelescopeType type) |
bool | setTemperature (double value) |
bool | setTemperatureRegulation (double ramp, double threshold) |
bool | setUploadMode (UploadMode mode) |
bool | setVideoStreamEnabled (bool enable) |
bool | startDurationRecording (double duration) |
bool | startFramesRecording (uint32_t frames) |
bool | startRecording () |
bool | stopRecording () |
void | updateUploadSettings (const QString &uploadDirectory, const QString &uploadFile) |
![]() | |
ConcreteDevice (GenericDevice *parent) | |
void | Connect () |
void | Disconnect () |
GenericDevice * | genericDevice () const |
INDI::PropertyView< IBLOB > * | getBLOB (const QString &name) const |
const QString & | getDeviceName () const |
const QSharedPointer< DriverInfo > & | getDriverInfo () const |
uint32_t | getDriverInterface () |
const QString & | getDUBSObjectPath () const |
INDI::PropertyView< ILight > * | getLight (const QString &name) const |
QString | getMessage (int id) const |
bool | getMinMaxStep (const QString &propName, const QString &elementName, double *min, double *max, double *step) const |
INDI::PropertyView< INumber > * | getNumber (const QString &name) const |
IPerm | getPermission (const QString &propName) const |
Properties | getProperties () const |
INDI::Property | getProperty (const QString &name) const |
IPState | getState (const QString &propName) const |
INDI::PropertyView< ISwitch > * | getSwitch (const QString &name) const |
INDI::PropertyView< IText > * | getText (const QString &name) const |
bool | isConnected () const |
bool | isReady () const |
virtual void | processLight (INDI::Property) override |
virtual void | processMessage (int) override |
void | processProperties () |
void | registeProperties () |
void | sendNewProperty (INDI::Property prop) |
bool | setConfig (INDIConfig tConfig) |
virtual void | updateProperty (INDI::Property prop) override |
![]() | |
GDInterface (QObject *parent) | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Public Attributes | |
enum ISD::Camera::BlobType | BType |
![]() | |
typedef | QObjectList |
Protected Slots | |
void | setWSBLOB (const QByteArray &message, const QString &extension) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
static uint8_t | getID () |
![]() | |
QString | m_DBusObjectPath |
QString | m_Name |
GenericDevice * | m_Parent |
QScopedPointer< QTimer > | m_ReadyTimer |
![]() | |
static uint8_t | m_ID = 1 |
Detailed Description
Camera class controls an INDI Camera device.
It can be used to issue and abort capture commands, receive and process BLOBs, and return information on the capabilities of the camera.
Definition at line 44 of file indicamera.h.
Member Enumeration Documentation
◆ BlobType
enum ISD::Camera::BlobType |
Definition at line 54 of file indicamera.h.
◆ ErrorType
Enumerator | |
---|---|
ERROR_SAVE | INDI Camera error. |
ERROR_LOAD | Saving to disk error. |
ERROR_VIEWER | Loading image buffer error. Loading in FITS Viewer Error |
Definition at line 63 of file indicamera.h.
◆ TelescopeType
enum ISD::Camera::TelescopeType |
Definition at line 62 of file indicamera.h.
◆ UploadMode
enum ISD::Camera::UploadMode |
Definition at line 53 of file indicamera.h.
Property Documentation
◆ StreamingEnabled
bool ISD::Camera::StreamingEnabled |
Definition at line 47 of file indicamera.h.
Constructor & Destructor Documentation
◆ Camera()
|
explicit |
Definition at line 43 of file indicamera.cpp.
◆ ~Camera()
|
overridevirtual |
Definition at line 54 of file indicamera.cpp.
Member Function Documentation
◆ canCool()
|
inline |
Definition at line 84 of file indicamera.h.
◆ configureRapidGuide()
bool ISD::Camera::configureRapidGuide | ( | CameraChip * | targetChip, |
bool | autoLoop, | ||
bool | sendImage = false, | ||
bool | showMarker = false ) |
Definition at line 834 of file indicamera.cpp.
◆ getCaptureFormat()
QString ISD::Camera::getCaptureFormat | ( | ) | const |
Definition at line 1628 of file indicamera.cpp.
◆ getCaptureFormats()
|
inline |
Definition at line 183 of file indicamera.h.
◆ getChip()
CameraChip * ISD::Camera::getChip | ( | CameraChip::ChipType | cType | ) |
Definition at line 789 of file indicamera.cpp.
◆ getEncodingFormat()
|
inline |
Definition at line 154 of file indicamera.h.
◆ getEncodingFormats()
|
inline |
Definition at line 159 of file indicamera.h.
◆ getExposurePresets()
Definition at line 230 of file indicamera.h.
◆ getExposurePresetsMinMax()
|
inline |
Definition at line 234 of file indicamera.h.
◆ getGain()
bool ISD::Camera::getGain | ( | double * | value | ) |
Definition at line 1415 of file indicamera.cpp.
◆ getGainMinMaxStep()
bool ISD::Camera::getGainMinMaxStep | ( | double * | min, |
double * | max, | ||
double * | step ) |
Definition at line 1425 of file indicamera.cpp.
◆ getGainPermission()
|
inline |
Definition at line 124 of file indicamera.h.
◆ getOffset()
bool ISD::Camera::getOffset | ( | double * | value | ) |
Definition at line 1447 of file indicamera.cpp.
◆ getOffsetMinMaxStep()
bool ISD::Camera::getOffsetMinMaxStep | ( | double * | min, |
double * | max, | ||
double * | step ) |
Definition at line 1457 of file indicamera.cpp.
◆ getOffsetPermission()
|
inline |
Definition at line 137 of file indicamera.h.
◆ getSERNameDirectory()
Definition at line 1251 of file indicamera.cpp.
◆ getStreamEncoding()
|
inline |
Definition at line 163 of file indicamera.h.
◆ getStreamEncodings()
|
inline |
Definition at line 168 of file indicamera.h.
◆ getStreamExposure()
bool ISD::Camera::getStreamExposure | ( | double * | duration | ) |
Definition at line 1539 of file indicamera.cpp.
◆ getStreamRecording()
|
inline |
Definition at line 172 of file indicamera.h.
◆ getTelescopeType()
|
inline |
Definition at line 212 of file indicamera.h.
◆ getTemperature()
bool ISD::Camera::getTemperature | ( | double * | value | ) |
Definition at line 972 of file indicamera.cpp.
◆ getTemperatureRegulation()
bool ISD::Camera::getTemperatureRegulation | ( | double & | ramp, |
double & | threshold ) |
Definition at line 1561 of file indicamera.cpp.
◆ getUploadMode()
Camera::UploadMode ISD::Camera::getUploadMode | ( | ) |
Definition at line 894 of file indicamera.cpp.
◆ getVideoFormats()
|
inline |
Definition at line 177 of file indicamera.h.
◆ hasCooler()
bool ISD::Camera::hasCooler | ( | ) |
Definition at line 756 of file indicamera.cpp.
◆ hasCoolerControl()
bool ISD::Camera::hasCoolerControl | ( | ) |
Definition at line 761 of file indicamera.cpp.
◆ hasGain()
|
inline |
Definition at line 119 of file indicamera.h.
◆ hasGuideHead()
bool ISD::Camera::hasGuideHead | ( | ) |
Definition at line 751 of file indicamera.cpp.
◆ hasOffset()
|
inline |
Definition at line 132 of file indicamera.h.
◆ hasVideoStream()
|
inline |
Definition at line 93 of file indicamera.h.
◆ isBLOBEnabled()
bool ISD::Camera::isBLOBEnabled | ( | ) |
Definition at line 1469 of file indicamera.cpp.
◆ isCoolerOn()
bool ISD::Camera::isCoolerOn | ( | ) |
Definition at line 1551 of file indicamera.cpp.
◆ isFastExposureEnabled()
|
inline |
Definition at line 224 of file indicamera.h.
◆ isStreamingEnabled()
bool ISD::Camera::isStreamingEnabled | ( | ) |
Definition at line 1225 of file indicamera.cpp.
◆ processBLOB()
|
overridevirtual |
Reimplemented from ISD::ConcreteDevice.
Definition at line 621 of file indicamera.cpp.
◆ processNumber()
|
overridevirtual |
Reimplemented from ISD::ConcreteDevice.
Definition at line 304 of file indicamera.cpp.
◆ processSwitch()
|
overridevirtual |
Reimplemented from ISD::ConcreteDevice.
Definition at line 381 of file indicamera.cpp.
◆ processText()
|
overridevirtual |
Reimplemented from ISD::ConcreteDevice.
Definition at line 502 of file indicamera.cpp.
◆ registerProperty()
|
overridevirtual |
Reimplemented from ISD::ConcreteDevice.
Definition at line 73 of file indicamera.cpp.
◆ removeProperty()
|
overridevirtual |
Reimplemented from ISD::ConcreteDevice.
Definition at line 296 of file indicamera.cpp.
◆ resetStreamingFrame()
bool ISD::Camera::resetStreamingFrame | ( | ) |
Definition at line 1135 of file indicamera.cpp.
◆ saveCurrentImage()
bool ISD::Camera::saveCurrentImage | ( | QString & | filename | ) |
saveCurrentImage save the image that is currently in the image data buffer
- Returns
- true if saving succeeded
Definition at line 593 of file indicamera.cpp.
◆ setBLOBEnabled()
Definition at line 1474 of file indicamera.cpp.
◆ setBLOBManager
|
slot |
Definition at line 64 of file indicamera.cpp.
◆ setCaptureFormat()
bool ISD::Camera::setCaptureFormat | ( | const QString & | format | ) |
Definition at line 1498 of file indicamera.cpp.
◆ setCoolerControl()
bool ISD::Camera::setCoolerControl | ( | bool | enable | ) |
Definition at line 766 of file indicamera.cpp.
◆ setEncodingFormat()
bool ISD::Camera::setEncodingFormat | ( | const QString & | value | ) |
Definition at line 1006 of file indicamera.cpp.
◆ setFastCount()
bool ISD::Camera::setFastCount | ( | uint32_t | count | ) |
Definition at line 1511 of file indicamera.cpp.
◆ setFastExposureEnabled()
bool ISD::Camera::setFastExposureEnabled | ( | bool | enable | ) |
Definition at line 1481 of file indicamera.cpp.
◆ setFITSHeaders()
bool ISD::Camera::setFITSHeaders | ( | const QList< FITSData::Record > & | values | ) |
Definition at line 1385 of file indicamera.cpp.
◆ setGain()
bool ISD::Camera::setGain | ( | double | value | ) |
Definition at line 1405 of file indicamera.cpp.
◆ setNextSequenceID()
|
inline |
Definition at line 113 of file indicamera.h.
◆ setOffset()
bool ISD::Camera::setOffset | ( | double | value | ) |
Definition at line 1437 of file indicamera.cpp.
◆ setRapidGuide()
bool ISD::Camera::setRapidGuide | ( | CameraChip * | targetChip, |
bool | enable ) |
Definition at line 803 of file indicamera.cpp.
◆ setScopeInfo()
bool ISD::Camera::setScopeInfo | ( | double | focalLength, |
double | aperture ) |
Definition at line 1584 of file indicamera.cpp.
◆ setSeqPrefix()
|
inline |
Definition at line 109 of file indicamera.h.
◆ setSERNameDirectory()
Definition at line 1230 of file indicamera.cpp.
◆ setStreamEncoding()
bool ISD::Camera::setStreamEncoding | ( | const QString & | value | ) |
Definition at line 1031 of file indicamera.cpp.
◆ setStreamExposure()
bool ISD::Camera::setStreamExposure | ( | double | duration | ) |
Definition at line 1525 of file indicamera.cpp.
◆ setStreamingFrame()
bool ISD::Camera::setStreamingFrame | ( | int | x, |
int | y, | ||
int | w, | ||
int | h ) |
Definition at line 1192 of file indicamera.cpp.
◆ setStreamLimits()
bool ISD::Camera::setStreamLimits | ( | uint16_t | maxBufferSize, |
uint16_t | maxPreviewFPS ) |
Definition at line 1167 of file indicamera.cpp.
◆ setStreamRecording()
bool ISD::Camera::setStreamRecording | ( | const QString & | value | ) |
Definition at line 1056 of file indicamera.cpp.
◆ setTelescopeType()
bool ISD::Camera::setTelescopeType | ( | TelescopeType | type | ) |
Definition at line 1081 of file indicamera.cpp.
◆ setTemperature()
bool ISD::Camera::setTemperature | ( | double | value | ) |
Definition at line 987 of file indicamera.cpp.
◆ setTemperatureRegulation()
bool ISD::Camera::setTemperatureRegulation | ( | double | ramp, |
double | threshold ) |
Definition at line 1572 of file indicamera.cpp.
◆ setUploadMode()
bool ISD::Camera::setUploadMode | ( | UploadMode | mode | ) |
Definition at line 925 of file indicamera.cpp.
◆ setVideoStreamEnabled()
bool ISD::Camera::setVideoStreamEnabled | ( | bool | enable | ) |
Definition at line 1111 of file indicamera.cpp.
◆ setWSBLOB
|
protectedslot |
Definition at line 513 of file indicamera.cpp.
◆ startDurationRecording()
bool ISD::Camera::startDurationRecording | ( | double | duration | ) |
Definition at line 1293 of file indicamera.cpp.
◆ startFramesRecording()
bool ISD::Camera::startFramesRecording | ( | uint32_t | frames | ) |
Definition at line 1329 of file indicamera.cpp.
◆ startRecording()
bool ISD::Camera::startRecording | ( | ) |
Definition at line 1270 of file indicamera.cpp.
◆ stopRecording()
bool ISD::Camera::stopRecording | ( | ) |
Definition at line 1361 of file indicamera.cpp.
◆ StreamWindowHidden
|
slot |
Definition at line 714 of file indicamera.cpp.
◆ updateUploadSettings()
void ISD::Camera::updateUploadSettings | ( | const QString & | uploadDirectory, |
const QString & | uploadFile ) |
Definition at line 869 of file indicamera.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:57:27 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.