KSDssDownloader
#include <ksdssdownloader.h>
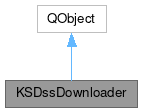
Signals | |
void | downloadCanceled () |
void | downloadComplete (bool success) |
Public Member Functions | |
KSDssDownloader (const SkyPoint *const p, const QString &destFileName, const std::function< void(bool)> &slotDownloadReady, QObject *parent=nullptr) | |
KSDssDownloader (QObject *parent=nullptr) | |
void | startSingleDownload (const QUrl srcUrl, const QString &destFileName, KSDssImage::Metadata &md) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static QString | getDSSURL (const dms &ra, const dms &dec, float width=0, float height=0, const QString &type_="gif", const QString &version_="all", struct KSDssImage::Metadata *md=nullptr) |
static QString | getDSSURL (const SkyPoint *const p, const QString &version="all", struct KSDssImage::Metadata *md=nullptr) |
static QString | getDSSURL (const SkyPoint *const p, float width, float height=0, const QString &version="all", struct KSDssImage::Metadata *md=nullptr) |
static bool | writeImageWithMetadata (const QString &srcFile, const QString &destFile, const KSDssImage::Metadata &md) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Helps download a DSS image.
- Note
- This object is designed to commit suicide (calls QObject::deleteLater() )! Never allocate this using anything but new – do not allocate it on the stack! This is ideal for its operation, as it deletes itself after downloading.
Definition at line 34 of file ksdssdownloader.h.
Constructor & Destructor Documentation
◆ KSDssDownloader() [1/2]
|
explicit |
Constructor.
Definition at line 17 of file ksdssdownloader.cpp.
◆ KSDssDownloader() [2/2]
KSDssDownloader::KSDssDownloader | ( | const SkyPoint *const | p, |
const QString & | destFileName, | ||
const std::function< void(bool)> & | slotDownloadReady, | ||
QObject * | parent = nullptr ) |
Constructor that initiates a "standard" DSS download job, calls the downloadReady slot, and finally self destructs.
- Note
- Very important that if you create with this constructor, the object will self-destruct. Avoid keeping pointers to it, or things may segfault!
Definition at line 29 of file ksdssdownloader.cpp.
Member Function Documentation
◆ getDSSURL() [1/3]
|
static |
Create a URL to obtain a DSS image for a given RA, Dec.
- Parameters
-
ra The J2000.0 Right Ascension of the point dec The J2000.0 Declination of the point width The width of the image in arcminutes height The height of the image in arcminutes type_ The image type, either gif or fits. version_ string describing which version to get md If a valid pointer is provided, fill with metadata
- Note
- This method resets height and width to fall within the range accepted by DSS
- Moved from namespace KSUtils (–asimha, Jan 5 2016)
- Valid versions are: dss1, poss2ukstu_red, poss2ukstu_ir, poss2ukstu_blue, poss1_blue, poss1_red, all, quickv, phase2_gsc2, phase2_gsc1. Of these, dss1 uses POSS1 Red in the north and POSS2/UKSTU Blue in the south. all uses the best of a combined list of all plates.
Definition at line 120 of file ksdssdownloader.cpp.
◆ getDSSURL() [2/3]
|
static |
High-level method to create a URL to obtain a DSS image for a given SkyPoint.
- Note
- If SkyPoint is a DeepSkyObject, this method automatically decides the image size required to fit the object.
- Moved from namespace KSUtils (–asimha, Jan 5 2016)
Definition at line 49 of file ksdssdownloader.cpp.
◆ getDSSURL() [3/3]
|
static |
High-level method to create a URL to obtain a DSS image for a given SkyPoint.
- Note
- This method includes an option to set the height, but uses default values for many parameters
Definition at line 99 of file ksdssdownloader.cpp.
◆ startSingleDownload()
void KSDssDownloader::startSingleDownload | ( | const QUrl | srcUrl, |
const QString & | destFileName, | ||
KSDssImage::Metadata & | md ) |
Stateful single-download of a supplied URL.
Use when the flexibility is required
- Note
- Does not self-delete this object. Construct with default constructor, and delete as usual.
- Parameters
-
srcUrl source DSS URL to download destFileName destination image file (will be of PNG format) md DSS image metadata to write into image file
- Note
- emits downloadComplete with success state when done
Definition at line 218 of file ksdssdownloader.cpp.
◆ writeImageWithMetadata()
|
static |
Write image metadata into file.
Definition at line 317 of file ksdssdownloader.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.