QCPPainter
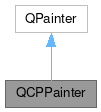
Public Types | |
enum | PainterMode { pmDefault = 0x00 , pmVectorized = 0x01 , pmNoCaching = 0x02 , pmNonCosmetic = 0x04 } |
typedef QFlags< PainterMode > | PainterModes |
![]() | |
enum | CompositionMode |
enum | PixmapFragmentHint |
enum | RenderHint |
Public Member Functions | |
QCPPainter () | |
QCPPainter (QPaintDevice *device) | |
bool | antialiasing () const |
bool | begin (QPaintDevice *device) |
void | drawLine (const QLineF &line) |
void | drawLine (const QPointF &p1, const QPointF &p2) |
void | makeNonCosmetic () |
PainterModes | modes () const |
void | restore () |
void | save () |
void | setAntialiasing (bool enabled) |
void | setMode (PainterMode mode, bool enabled=true) |
void | setModes (PainterModes modes) |
void | setPen (const QColor &color) |
void | setPen (const QPen &pen) |
void | setPen (Qt::PenStyle penStyle) |
![]() | |
QPainter (QPaintDevice *device) | |
const QBrush & | background () const const |
Qt::BGMode | backgroundMode () const const |
bool | begin (QPaintDevice *device) |
void | beginNativePainting () |
QRect | boundingRect (const QRect &rectangle, int flags, const QString &text) |
QRectF | boundingRect (const QRectF &rectangle, const QString &text, const QTextOption &option) |
QRectF | boundingRect (const QRectF &rectangle, int flags, const QString &text) |
QRect | boundingRect (int x, int y, int w, int h, int flags, const QString &text) |
const QBrush & | brush () const const |
QPoint | brushOrigin () const const |
QRectF | clipBoundingRect () const const |
QPainterPath | clipPath () const const |
QRegion | clipRegion () const const |
QTransform | combinedTransform () const const |
CompositionMode | compositionMode () const const |
QPaintDevice * | device () const const |
const QTransform & | deviceTransform () const const |
void | drawArc (const QRect &rectangle, int startAngle, int spanAngle) |
void | drawArc (const QRectF &rectangle, int startAngle, int spanAngle) |
void | drawArc (int x, int y, int width, int height, int startAngle, int spanAngle) |
void | drawChord (const QRect &rectangle, int startAngle, int spanAngle) |
void | drawChord (const QRectF &rectangle, int startAngle, int spanAngle) |
void | drawChord (int x, int y, int width, int height, int startAngle, int spanAngle) |
void | drawConvexPolygon (const QPoint *points, int pointCount) |
void | drawConvexPolygon (const QPointF *points, int pointCount) |
void | drawConvexPolygon (const QPolygon &polygon) |
void | drawConvexPolygon (const QPolygonF &polygon) |
void | drawEllipse (const QPoint ¢er, int rx, int ry) |
void | drawEllipse (const QPointF ¢er, qreal rx, qreal ry) |
void | drawEllipse (const QRect &rectangle) |
void | drawEllipse (const QRectF &rectangle) |
void | drawEllipse (int x, int y, int width, int height) |
void | drawGlyphRun (const QPointF &position, const QGlyphRun &glyphs) |
void | drawImage (const QPoint &point, const QImage &image) |
void | drawImage (const QPoint &point, const QImage &image, const QRect &source, Qt::ImageConversionFlags flags) |
void | drawImage (const QPointF &point, const QImage &image) |
void | drawImage (const QPointF &point, const QImage &image, const QRectF &source, Qt::ImageConversionFlags flags) |
void | drawImage (const QRect &rectangle, const QImage &image) |
void | drawImage (const QRect &target, const QImage &image, const QRect &source, Qt::ImageConversionFlags flags) |
void | drawImage (const QRectF &rectangle, const QImage &image) |
void | drawImage (const QRectF &target, const QImage &image, const QRectF &source, Qt::ImageConversionFlags flags) |
void | drawImage (int x, int y, const QImage &image, int sx, int sy, int sw, int sh, Qt::ImageConversionFlags flags) |
void | drawLine (const QLine &line) |
void | drawLine (const QLineF &line) |
void | drawLine (const QPoint &p1, const QPoint &p2) |
void | drawLine (const QPointF &p1, const QPointF &p2) |
void | drawLine (int x1, int y1, int x2, int y2) |
void | drawLines (const QLine *lines, int lineCount) |
void | drawLines (const QLineF *lines, int lineCount) |
void | drawLines (const QList< QLine > &lines) |
void | drawLines (const QList< QLineF > &lines) |
void | drawLines (const QList< QPoint > &pointPairs) |
void | drawLines (const QList< QPointF > &pointPairs) |
void | drawLines (const QPoint *pointPairs, int lineCount) |
void | drawLines (const QPointF *pointPairs, int lineCount) |
void | drawPath (const QPainterPath &path) |
void | drawPicture (const QPoint &point, const QPicture &picture) |
void | drawPicture (const QPointF &point, const QPicture &picture) |
void | drawPicture (int x, int y, const QPicture &picture) |
void | drawPie (const QRect &rectangle, int startAngle, int spanAngle) |
void | drawPie (const QRectF &rectangle, int startAngle, int spanAngle) |
void | drawPie (int x, int y, int width, int height, int startAngle, int spanAngle) |
void | drawPixmap (const QPoint &point, const QPixmap &pixmap) |
void | drawPixmap (const QPoint &point, const QPixmap &pixmap, const QRect &source) |
void | drawPixmap (const QPointF &point, const QPixmap &pixmap) |
void | drawPixmap (const QPointF &point, const QPixmap &pixmap, const QRectF &source) |
void | drawPixmap (const QRect &rectangle, const QPixmap &pixmap) |
void | drawPixmap (const QRect &target, const QPixmap &pixmap, const QRect &source) |
void | drawPixmap (const QRectF &target, const QPixmap &pixmap, const QRectF &source) |
void | drawPixmap (int x, int y, const QPixmap &pixmap) |
void | drawPixmap (int x, int y, const QPixmap &pixmap, int sx, int sy, int sw, int sh) |
void | drawPixmap (int x, int y, int w, int h, const QPixmap &pixmap, int sx, int sy, int sw, int sh) |
void | drawPixmap (int x, int y, int width, int height, const QPixmap &pixmap) |
void | drawPixmapFragments (const PixmapFragment *fragments, int fragmentCount, const QPixmap &pixmap, PixmapFragmentHints hints) |
void | drawPoint (const QPoint &position) |
void | drawPoint (const QPointF &position) |
void | drawPoint (int x, int y) |
void | drawPoints (const QPoint *points, int pointCount) |
void | drawPoints (const QPointF *points, int pointCount) |
void | drawPoints (const QPolygon &points) |
void | drawPoints (const QPolygonF &points) |
void | drawPolygon (const QPoint *points, int pointCount, Qt::FillRule fillRule) |
void | drawPolygon (const QPointF *points, int pointCount, Qt::FillRule fillRule) |
void | drawPolygon (const QPolygon &points, Qt::FillRule fillRule) |
void | drawPolygon (const QPolygonF &points, Qt::FillRule fillRule) |
void | drawPolyline (const QPoint *points, int pointCount) |
void | drawPolyline (const QPointF *points, int pointCount) |
void | drawPolyline (const QPolygon &points) |
void | drawPolyline (const QPolygonF &points) |
void | drawRect (const QRect &rectangle) |
void | drawRect (const QRectF &rectangle) |
void | drawRect (int x, int y, int width, int height) |
void | drawRects (const QList< QRect > &rectangles) |
void | drawRects (const QList< QRectF > &rectangles) |
void | drawRects (const QRect *rectangles, int rectCount) |
void | drawRects (const QRectF *rectangles, int rectCount) |
void | drawRoundedRect (const QRect &rect, qreal xRadius, qreal yRadius, Qt::SizeMode mode) |
void | drawRoundedRect (const QRectF &rect, qreal xRadius, qreal yRadius, Qt::SizeMode mode) |
void | drawRoundedRect (int x, int y, int w, int h, qreal xRadius, qreal yRadius, Qt::SizeMode mode) |
void | drawStaticText (const QPoint &topLeftPosition, const QStaticText &staticText) |
void | drawStaticText (const QPointF &topLeftPosition, const QStaticText &staticText) |
void | drawStaticText (int left, int top, const QStaticText &staticText) |
void | drawText (const QPoint &position, const QString &text) |
void | drawText (const QPointF &position, const QString &text) |
void | drawText (const QRect &rectangle, int flags, const QString &text, QRect *boundingRect) |
void | drawText (const QRectF &rectangle, const QString &text, const QTextOption &option) |
void | drawText (const QRectF &rectangle, int flags, const QString &text, QRectF *boundingRect) |
void | drawText (int x, int y, const QString &text) |
void | drawText (int x, int y, int width, int height, int flags, const QString &text, QRect *boundingRect) |
void | drawTiledPixmap (const QRect &rectangle, const QPixmap &pixmap, const QPoint &position) |
void | drawTiledPixmap (const QRectF &rectangle, const QPixmap &pixmap, const QPointF &position) |
void | drawTiledPixmap (int x, int y, int width, int height, const QPixmap &pixmap, int sx, int sy) |
bool | end () |
void | endNativePainting () |
void | eraseRect (const QRect &rectangle) |
void | eraseRect (const QRectF &rectangle) |
void | eraseRect (int x, int y, int width, int height) |
void | fillPath (const QPainterPath &path, const QBrush &brush) |
void | fillRect (const QRect &rectangle, const QBrush &brush) |
void | fillRect (const QRect &rectangle, const QColor &color) |
void | fillRect (const QRect &rectangle, QGradient::Preset preset) |
void | fillRect (const QRect &rectangle, Qt::BrushStyle style) |
void | fillRect (const QRect &rectangle, Qt::GlobalColor color) |
void | fillRect (const QRectF &rectangle, const QBrush &brush) |
void | fillRect (const QRectF &rectangle, const QColor &color) |
void | fillRect (const QRectF &rectangle, QGradient::Preset preset) |
void | fillRect (const QRectF &rectangle, Qt::BrushStyle style) |
void | fillRect (const QRectF &rectangle, Qt::GlobalColor color) |
void | fillRect (int x, int y, int width, int height, const QBrush &brush) |
void | fillRect (int x, int y, int width, int height, const QColor &color) |
void | fillRect (int x, int y, int width, int height, QGradient::Preset preset) |
void | fillRect (int x, int y, int width, int height, Qt::BrushStyle style) |
void | fillRect (int x, int y, int width, int height, Qt::GlobalColor color) |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
bool | hasClipping () const const |
bool | isActive () const const |
Qt::LayoutDirection | layoutDirection () const const |
qreal | opacity () const const |
QPaintEngine * | paintEngine () const const |
const QPen & | pen () const const |
RenderHints | renderHints () const const |
void | resetTransform () |
void | restore () |
void | rotate (qreal angle) |
void | save () |
void | scale (qreal sx, qreal sy) |
void | setBackground (const QBrush &brush) |
void | setBackgroundMode (Qt::BGMode mode) |
void | setBrush (const QBrush &brush) |
void | setBrush (Qt::BrushStyle style) |
void | setBrushOrigin (const QPoint &position) |
void | setBrushOrigin (const QPointF &position) |
void | setBrushOrigin (int x, int y) |
void | setClipPath (const QPainterPath &path, Qt::ClipOperation operation) |
void | setClipping (bool enable) |
void | setClipRect (const QRect &rectangle, Qt::ClipOperation operation) |
void | setClipRect (const QRectF &rectangle, Qt::ClipOperation operation) |
void | setClipRect (int x, int y, int width, int height, Qt::ClipOperation operation) |
void | setClipRegion (const QRegion ®ion, Qt::ClipOperation operation) |
void | setCompositionMode (CompositionMode mode) |
void | setFont (const QFont &font) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setOpacity (qreal opacity) |
void | setPen (const QColor &color) |
void | setPen (const QPen &pen) |
void | setPen (Qt::PenStyle style) |
void | setRenderHint (RenderHint hint, bool on) |
void | setRenderHints (RenderHints hints, bool on) |
void | setTransform (const QTransform &transform, bool combine) |
void | setViewport (const QRect &rectangle) |
void | setViewport (int x, int y, int width, int height) |
void | setViewTransformEnabled (bool enable) |
void | setWindow (const QRect &rectangle) |
void | setWindow (int x, int y, int width, int height) |
void | setWorldMatrixEnabled (bool enable) |
void | setWorldTransform (const QTransform &matrix, bool combine) |
void | shear (qreal sh, qreal sv) |
void | strokePath (const QPainterPath &path, const QPen &pen) |
bool | testRenderHint (RenderHint hint) const const |
const QTransform & | transform () const const |
void | translate (const QPoint &offset) |
void | translate (const QPointF &offset) |
void | translate (qreal dx, qreal dy) |
QRect | viewport () const const |
bool | viewTransformEnabled () const const |
QRect | window () const const |
bool | worldMatrixEnabled () const const |
const QTransform & | worldTransform () const const |
Protected Attributes | |
QStack< bool > | mAntialiasingStack |
bool | mIsAntialiasing |
PainterModes | mModes |
Detailed Description
QPainter subclass used internally.
This QPainter subclass is used to provide some extended functionality e.g. for tweaking position consistency between antialiased and non-antialiased painting. Further it provides workarounds for QPainter quirks.
- Warning
- This class intentionally hides non-virtual functions of QPainter, e.g. setPen, save and restore. So while it is possible to pass a QCPPainter instance to a function that expects a QPainter pointer, some of the workarounds and tweaks will be unavailable to the function (because it will call the base class implementations of the functions actually hidden by QCPPainter).
Definition at line 515 of file qcustomplot.h.
Member Typedef Documentation
◆ PainterModes
typedef QFlags< PainterMode > QCPPainter::PainterModes |
Definition at line 530 of file qcustomplot.h.
Member Enumeration Documentation
◆ PainterMode
Defines special modes the painter can operate in. They disable or enable certain subsets of features/fixes/workarounds, depending on whether they are wanted on the respective output device.
Definition at line 523 of file qcustomplot.h.
Constructor & Destructor Documentation
◆ QCPPainter() [1/2]
QCPPainter::QCPPainter | ( | ) |
Creates a new QCPPainter instance and sets default values
Definition at line 297 of file qcustomplot.cpp.
◆ QCPPainter() [2/2]
|
explicit |
Creates a new QCPPainter instance on the specified paint device and sets default values. Just like the analogous QPainter constructor, begins painting on device immediately.
Like begin, this method sets QPainter::NonCosmeticDefaultPen in Qt versions before Qt5.
Definition at line 311 of file qcustomplot.cpp.
Member Function Documentation
◆ antialiasing()
|
inline |
Definition at line 536 of file qcustomplot.h.
◆ begin()
bool QCPPainter::begin | ( | QPaintDevice * | device | ) |
Sets the QPainter::NonCosmeticDefaultPen in Qt versions before Qt5 after beginning painting on device. This is necessary to get cosmetic pen consistency across Qt versions, because since Qt5, all pens are non-cosmetic by default, and in Qt4 this render hint must be set to get that behaviour.
The Constructor QCPPainter(QPaintDevice *device) which directly starts painting also sets the render hint as appropriate.
- Note
- this function hides the non-virtual base class implementation.
Definition at line 421 of file qcustomplot.cpp.
◆ drawLine() [1/2]
void QCPPainter::drawLine | ( | const QLineF & | line | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Works around a Qt bug introduced with Qt 4.8 which makes drawing QLineF unpredictable when antialiasing is disabled. Thus when antialiasing is disabled, it rounds the line to integer coordinates and then passes it to the original drawLine.
- Note
- this function hides the non-virtual base class implementation.
Definition at line 371 of file qcustomplot.cpp.
◆ drawLine() [2/2]
Definition at line 550 of file qcustomplot.h.
◆ makeNonCosmetic()
void QCPPainter::makeNonCosmetic | ( | ) |
Changes the pen width to 1 if it currently is 0. This function is called in the setPen overrides when the pmNonCosmetic mode is set.
Definition at line 479 of file qcustomplot.cpp.
◆ modes()
|
inline |
Definition at line 537 of file qcustomplot.h.
◆ restore()
void QCPPainter::restore | ( | ) |
Restores the painter (see QPainter::restore). Since QCPPainter adds some new internal state to QPainter, the save/restore functions are reimplemented to also save/restore those members.
- Note
- this function hides the non-virtual base class implementation.
- See also
- save
Definition at line 466 of file qcustomplot.cpp.
◆ save()
void QCPPainter::save | ( | ) |
Saves the painter (see QPainter::save). Since QCPPainter adds some new internal state to QPainter, the save/restore functions are reimplemented to also save/restore those members.
- Note
- this function hides the non-virtual base class implementation.
- See also
- restore
Definition at line 452 of file qcustomplot.cpp.
◆ setAntialiasing()
void QCPPainter::setAntialiasing | ( | bool | enabled | ) |
Sets whether painting uses antialiasing or not. Use this method instead of using setRenderHint with QPainter::Antialiasing directly, as it allows QCPPainter to regain pixel exactness between antialiased and non-antialiased painting (Since Qt < 5.0 uses slightly different coordinate systems for AA/Non-AA painting).
Definition at line 385 of file qcustomplot.cpp.
◆ setMode()
void QCPPainter::setMode | ( | QCPPainter::PainterMode | mode, |
bool | enabled = true ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Sets the mode of the painter. This controls whether the painter shall adjust its fixes/workarounds optimized for certain output devices.
Definition at line 436 of file qcustomplot.cpp.
◆ setModes()
void QCPPainter::setModes | ( | QCPPainter::PainterModes | modes | ) |
Sets the mode of the painter. This controls whether the painter shall adjust its fixes/workarounds optimized for certain output devices.
Definition at line 405 of file qcustomplot.cpp.
◆ setPen() [1/3]
void QCPPainter::setPen | ( | const QColor & | color | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Sets the pen (by color) of the painter and applies certain fixes to it, depending on the mode of this QCPPainter.
- Note
- this function hides the non-virtual base class implementation.
Definition at line 342 of file qcustomplot.cpp.
◆ setPen() [2/3]
void QCPPainter::setPen | ( | const QPen & | pen | ) |
Sets the pen of the painter and applies certain fixes to it, depending on the mode of this QCPPainter.
- Note
- this function hides the non-virtual base class implementation.
Definition at line 328 of file qcustomplot.cpp.
◆ setPen() [3/3]
void QCPPainter::setPen | ( | Qt::PenStyle | penStyle | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Sets the pen (by style) of the painter and applies certain fixes to it, depending on the mode of this QCPPainter.
- Note
- this function hides the non-virtual base class implementation.
Definition at line 356 of file qcustomplot.cpp.
Member Data Documentation
◆ mAntialiasingStack
|
protected |
Definition at line 563 of file qcustomplot.h.
◆ mIsAntialiasing
|
protected |
Definition at line 560 of file qcustomplot.h.
◆ mModes
|
protected |
Definition at line 559 of file qcustomplot.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:01:38 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.