TelescopeLite
#include <inditelescopelite.h>
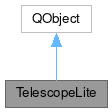
Public Types | |
enum | TelescopeMotionCommand { MOTION_START , MOTION_STOP } |
enum | TelescopeMotionNS { MOTION_NORTH , MOTION_SOUTH } |
enum | TelescopeMotionWE { MOTION_WEST , MOTION_EAST } |
Properties | |
QString | deviceName |
bool | slewDecreasable |
bool | slewIncreasable |
QString | slewRateLabel |
![]() | |
objectName | |
Signals | |
void | deviceNameChanged (QString) |
void | newTelescopeLiteCreated (TelescopeLite *) |
void | slewDecreasableChanged (bool) |
void | slewIncreasableChanged (bool) |
void | slewRateLabelChanged (QString) |
void | slewRateUpdate (int index, int count) |
Public Slots | |
Q_INVOKABLE bool | abort () |
bool | park () |
bool | setSlewRate (int index) |
bool | unPark () |
void | updateSlewRate (const QString &deviceName, const QString &propName) |
Public Member Functions | |
TelescopeLite (INDI::BaseDevice *device) | |
bool | canGuide () |
bool | canPark () |
bool | canSync () |
Q_INVOKABLE bool | decreaseSlewRate () |
INDI::BaseDevice * | getDevice () |
QString | getDeviceName () |
bool | getEqCoords (double *ra, double *dec) |
QString | getSlewRateLabel () |
Q_INVOKABLE QStringList | getSlewRateLabels () |
Q_INVOKABLE bool | increaseSlewRate () |
Q_INVOKABLE bool | isConnected () |
bool | isInMotion () |
bool | isParked () |
bool | isSlewDecreasable () |
bool | isSlewIncreasable () |
bool | isSlewing () |
Q_INVOKABLE bool | moveNS (TelescopeMotionNS dir, TelescopeMotionCommand cmd) |
Q_INVOKABLE bool | moveWE (TelescopeMotionWE dir, TelescopeMotionCommand cmd) |
void | processNumber (INumberVectorProperty *nvp) |
void | processSwitch (ISwitchVectorProperty *svp) |
void | processText (ITextVectorProperty *tvp) |
void | registerProperty (INDI::Property prop) |
void | setAltLimits (double minAltitude, double maxAltitude) |
void | setDeviceName (const QString &deviceName) |
void | setSlewDecreasable (bool slewDecreasable) |
void | setSlewIncreasable (bool slewIncreasable) |
void | setSlewRateLabel (const QString &slewRateLabel) |
bool | slew (double ra, double dec) |
Q_INVOKABLE bool | slew (SkyPoint *ScopeTarget) |
Q_INVOKABLE bool | slew (SkyPointLite *ScopeTarget) |
bool | sync (double ra, double dec) |
Q_INVOKABLE bool | sync (SkyPoint *ScopeTarget) |
Q_INVOKABLE bool | sync (SkyPointLite *ScopeTarget) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
bool | sendCoords (SkyPoint *ScopeTarget) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
device handle controlling telescope.
It can slew and sync to a specific sky point and supports all standard properties with INDI telescope device.
Definition at line 26 of file inditelescopelite.h.
Member Enumeration Documentation
◆ TelescopeMotionCommand
enum TelescopeLite::TelescopeMotionCommand |
Definition at line 50 of file inditelescopelite.h.
◆ TelescopeMotionNS
enum TelescopeLite::TelescopeMotionNS |
Definition at line 40 of file inditelescopelite.h.
◆ TelescopeMotionWE
enum TelescopeLite::TelescopeMotionWE |
Definition at line 45 of file inditelescopelite.h.
Property Documentation
◆ deviceName
|
readwrite |
Definition at line 33 of file inditelescopelite.h.
◆ slewDecreasable
|
readwrite |
Definition at line 30 of file inditelescopelite.h.
◆ slewIncreasable
|
readwrite |
Definition at line 31 of file inditelescopelite.h.
◆ slewRateLabel
|
readwrite |
Definition at line 32 of file inditelescopelite.h.
Constructor & Destructor Documentation
◆ TelescopeLite() [1/2]
|
explicit |
Definition at line 17 of file inditelescopelite.cpp.
◆ TelescopeLite() [2/2]
|
inline |
Definition at line 37 of file inditelescopelite.h.
◆ ~TelescopeLite()
TelescopeLite::~TelescopeLite | ( | ) |
Definition at line 51 of file inditelescopelite.cpp.
Member Function Documentation
◆ abort
|
slot |
Definition at line 446 of file inditelescopelite.cpp.
◆ canGuide()
bool TelescopeLite::canGuide | ( | ) |
Definition at line 199 of file inditelescopelite.cpp.
◆ canPark()
bool TelescopeLite::canPark | ( | ) |
Definition at line 219 of file inditelescopelite.cpp.
◆ canSync()
bool TelescopeLite::canSync | ( | ) |
Definition at line 207 of file inditelescopelite.cpp.
◆ decreaseSlewRate()
bool TelescopeLite::decreaseSlewRate | ( | ) |
Definition at line 653 of file inditelescopelite.cpp.
◆ getDevice()
|
inline |
Definition at line 65 of file inditelescopelite.h.
◆ getDeviceName()
|
inline |
Definition at line 67 of file inditelescopelite.h.
◆ getEqCoords()
bool TelescopeLite::getEqCoords | ( | double * | ra, |
double * | dec ) |
Definition at line 511 of file inditelescopelite.cpp.
◆ getSlewRateLabel()
|
inline |
Definition at line 73 of file inditelescopelite.h.
◆ getSlewRateLabels()
|
inline |
Definition at line 103 of file inditelescopelite.h.
◆ increaseSlewRate()
bool TelescopeLite::increaseSlewRate | ( | ) |
Definition at line 658 of file inditelescopelite.cpp.
◆ isConnected()
|
inline |
Definition at line 102 of file inditelescopelite.h.
◆ isInMotion()
bool TelescopeLite::isInMotion | ( | ) |
Definition at line 240 of file inditelescopelite.cpp.
◆ isParked()
bool TelescopeLite::isParked | ( | ) |
Definition at line 669 of file inditelescopelite.cpp.
◆ isSlewDecreasable()
|
inline |
Definition at line 70 of file inditelescopelite.h.
◆ isSlewIncreasable()
|
inline |
Definition at line 71 of file inditelescopelite.h.
◆ isSlewing()
bool TelescopeLite::isSlewing | ( | ) |
Definition at line 231 of file inditelescopelite.cpp.
◆ moveNS()
bool TelescopeLite::moveNS | ( | TelescopeMotionNS | dir, |
TelescopeMotionCommand | cmd ) |
Definition at line 532 of file inditelescopelite.cpp.
◆ moveWE()
bool TelescopeLite::moveWE | ( | TelescopeMotionWE | dir, |
TelescopeMotionCommand | cmd ) |
Definition at line 575 of file inditelescopelite.cpp.
◆ park
|
slot |
Definition at line 467 of file inditelescopelite.cpp.
◆ processNumber()
void TelescopeLite::processNumber | ( | INumberVectorProperty * | nvp | ) |
Definition at line 119 of file inditelescopelite.cpp.
◆ processSwitch()
void TelescopeLite::processSwitch | ( | ISwitchVectorProperty * | svp | ) |
Definition at line 181 of file inditelescopelite.cpp.
◆ registerProperty()
void TelescopeLite::registerProperty | ( | INDI::Property | prop | ) |
Definition at line 91 of file inditelescopelite.cpp.
◆ sendCoords()
|
protected |
Definition at line 256 of file inditelescopelite.cpp.
◆ setAltLimits()
void TelescopeLite::setAltLimits | ( | double | minAltitude, |
double | maxAltitude ) |
Definition at line 663 of file inditelescopelite.cpp.
◆ setDeviceName()
void TelescopeLite::setDeviceName | ( | const QString & | deviceName | ) |
Definition at line 82 of file inditelescopelite.cpp.
◆ setSlewDecreasable()
void TelescopeLite::setSlewDecreasable | ( | bool | slewDecreasable | ) |
Definition at line 55 of file inditelescopelite.cpp.
◆ setSlewIncreasable()
void TelescopeLite::setSlewIncreasable | ( | bool | slewIncreasable | ) |
Definition at line 64 of file inditelescopelite.cpp.
◆ setSlewRate
|
slot |
Definition at line 618 of file inditelescopelite.cpp.
◆ setSlewRateLabel()
void TelescopeLite::setSlewRateLabel | ( | const QString & | slewRateLabel | ) |
Definition at line 73 of file inditelescopelite.cpp.
◆ slew() [1/3]
bool TelescopeLite::slew | ( | double | ra, |
double | dec ) |
Definition at line 373 of file inditelescopelite.cpp.
◆ slew() [2/3]
bool TelescopeLite::slew | ( | SkyPoint * | ScopeTarget | ) |
Definition at line 383 of file inditelescopelite.cpp.
◆ slew() [3/3]
|
inline |
Definition at line 84 of file inditelescopelite.h.
◆ sync() [1/3]
bool TelescopeLite::sync | ( | double | ra, |
double | dec ) |
Definition at line 411 of file inditelescopelite.cpp.
◆ sync() [2/3]
bool TelescopeLite::sync | ( | SkyPoint * | ScopeTarget | ) |
Definition at line 421 of file inditelescopelite.cpp.
◆ sync() [3/3]
|
inline |
Definition at line 87 of file inditelescopelite.h.
◆ unPark
|
slot |
Definition at line 489 of file inditelescopelite.cpp.
◆ updateSlewRate
Definition at line 27 of file inditelescopelite.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:59:54 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.