ctk3Slider
#include <ctk3slider.h>
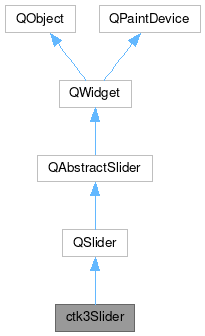
Public Types | |
typedef QSlider | Superclass |
![]() | |
enum | TickPosition |
![]() | |
enum | SliderAction |
enum | SliderChange |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
Signals | |
void | maximumPositionChanged (int max) |
void | maximumValueChanged (int max) |
void | midPositionChanged (int max) |
void | midValueChanged (int mid) |
void | minimumPositionChanged (int min) |
void | minimumValueChanged (int min) |
void | positionsChanged (int min, int mid, int max) |
void | released (int min, int mid, int max) |
void | valuesChanged (int min, int mid, int max) |
Public Slots | |
void | setMaximumValue (int max) |
void | setMidValue (int mid) |
void | setMinimumValue (int min) |
void | setValues (int min, int mid, int max) |
Public Member Functions | |
ctk3Slider (Qt::Orientation o, QWidget *par=0) | |
ctk3Slider (QWidget *par=0) | |
QString | handleToolTip () const |
bool | isMaximumSliderDown () const |
bool | isMidSliderDown () const |
bool | isMinimumSliderDown () const |
int | maximumPosition () const |
int | maximumValue () const |
int | midPosition () const |
int | midValue () const |
int | minimumPosition () const |
int | minimumValue () const |
void | setHandleToolTip (const QString &toolTip) |
void | setMaximumPosition (int max) |
void | setMidPosition (int mid) |
void | setMinimumPosition (int min) |
void | setPositions (int min, int mid, int max) |
![]() | |
QSlider (Qt::Orientation orientation, QWidget *parent) | |
QSlider (QWidget *parent) | |
virtual QSize | minimumSizeHint () const const override |
void | setTickInterval (int ti) |
void | setTickPosition (TickPosition position) |
virtual QSize | sizeHint () const const override |
int | tickInterval () const const |
TickPosition | tickPosition () const const |
![]() | |
QAbstractSlider (QWidget *parent) | |
void | actionTriggered (int action) |
bool | hasTracking () const const |
bool | invertedAppearance () const const |
bool | invertedControls () const const |
bool | isSliderDown () const const |
int | maximum () const const |
int | minimum () const const |
Qt::Orientation | orientation () const const |
int | pageStep () const const |
void | rangeChanged (int min, int max) |
void | setInvertedAppearance (bool) |
void | setInvertedControls (bool) |
void | setMaximum (int) |
void | setMinimum (int) |
void | setOrientation (Qt::Orientation) |
void | setPageStep (int) |
void | setRange (int min, int max) |
void | setSingleStep (int) |
void | setSliderDown (bool) |
void | setSliderPosition (int) |
void | setTracking (bool enable) |
void | setValue (int) |
int | singleStep () const const |
void | sliderMoved (int value) |
int | sliderPosition () const const |
void | sliderPressed () |
void | sliderReleased () |
void | triggerAction (SliderAction action) |
int | value () const const |
void | valueChanged (int value) |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
Protected Slots | |
void | onRangeChanged (int minimum, int maximum) |
Protected Member Functions | |
ctk3Slider (ctk3SliderPrivate *impl, Qt::Orientation o, QWidget *par=0) | |
ctk3Slider (ctk3SliderPrivate *impl, QWidget *par=0) | |
virtual bool | event (QEvent *event) override |
virtual void | initMaximumSliderStyleOption (QStyleOptionSlider *option) const |
virtual void | initMidSliderStyleOption (QStyleOptionSlider *option) const |
virtual void | initMinimumSliderStyleOption (QStyleOptionSlider *option) const |
virtual void | mouseMoveEvent (QMouseEvent *ev) override |
virtual void | mousePressEvent (QMouseEvent *ev) override |
virtual void | mouseReleaseEvent (QMouseEvent *ev) override |
virtual void | paintEvent (QPaintEvent *ev) override |
![]() | |
virtual void | initStyleOption (QStyleOptionSlider *option) const const |
![]() | |
virtual void | changeEvent (QEvent *ev) override |
virtual void | keyPressEvent (QKeyEvent *ev) override |
SliderAction | repeatAction () const const |
void | setRepeatAction (SliderAction action, int thresholdTime, int repeatTime) |
virtual void | sliderChange (SliderChange change) |
virtual void | timerEvent (QTimerEvent *e) override |
virtual void | wheelEvent (QWheelEvent *e) override |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEnterEvent *event) |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
Protected Attributes | |
QScopedPointer< ctk3SliderPrivate > | d_ptr |
Additional Inherited Members | |
![]() | |
QWidget * | createWindowContainer (QWindow *window, QWidget *parent, Qt::WindowFlags flags) |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
void | setTabOrder (std::initializer_list< QWidget * > widgets) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
NoTicks | |
TicksAbove | |
TicksBelow | |
TicksBothSides | |
TicksLeft | |
TicksRight | |
![]() | |
SliderMove | |
SliderNoAction | |
SliderOrientationChange | |
SliderPageStepAdd | |
SliderPageStepSub | |
SliderRangeChange | |
SliderSingleStepAdd | |
SliderSingleStepSub | |
SliderStepsChange | |
SliderToMaximum | |
SliderToMinimum | |
SliderValueChange | |
![]() | |
DrawChildren | |
DrawWindowBackground | |
IgnoreMask | |
typedef | RenderFlags |
![]() | |
typedef | QObjectList |
![]() | |
PdmDepth | |
PdmDevicePixelRatio | |
PdmDevicePixelRatioScaled | |
PdmDpiX | |
PdmDpiY | |
PdmHeight | |
PdmHeightMM | |
PdmNumColors | |
PdmPhysicalDpiX | |
PdmPhysicalDpiY | |
PdmWidth | |
PdmWidthMM | |
Detailed Description
A ctk3Slider is a slider that lets you input 2 values instead of one (see QSlider).
These values are typically a lower and upper bound. Values are comprised between the range of the slider. See setRange(), minimum() and maximum(). The upper bound can't be smaller than the lower bound and vice-versa. When setting new values (setMinimumValue(), setMaximumValue() or setValues()), make sure they lie between the range (minimum(), maximum()) of the slider, they would be forced otherwised. If it is not the behavior you desire, you can set the range first (setRange(), setMinimum(), setMaximum())
- See also
- ctkDoubleRangeSlider, ctkDoubleSlider, ctkRangeWidget
Definition at line 30 of file ctk3slider.h.
Member Typedef Documentation
◆ Superclass
typedef QSlider ctk3Slider::Superclass |
Definition at line 43 of file ctk3slider.h.
Property Documentation
◆ handleToolTip
|
readwrite |
Definition at line 39 of file ctk3slider.h.
◆ maximumPosition
|
readwrite |
Definition at line 37 of file ctk3slider.h.
◆ maximumValue
|
readwrite |
Definition at line 34 of file ctk3slider.h.
◆ midPosition
|
readwrite |
Definition at line 38 of file ctk3slider.h.
◆ midValue
|
readwrite |
Definition at line 35 of file ctk3slider.h.
◆ minimumPosition
|
readwrite |
Definition at line 36 of file ctk3slider.h.
◆ minimumValue
|
readwrite |
Definition at line 33 of file ctk3slider.h.
Constructor & Destructor Documentation
◆ ctk3Slider() [1/4]
|
explicit |
Constructor, builds a ctk3Slider that ranges from 0 to 100 and has a lower and upper values of 0 and 100 respectively, other properties are set the QSlider default properties.
Definition at line 337 of file ctk3slider.cpp.
◆ ctk3Slider() [2/4]
|
explicit |
Definition at line 328 of file ctk3slider.cpp.
◆ ~ctk3Slider()
|
virtual |
Definition at line 366 of file ctk3slider.cpp.
◆ ctk3Slider() [3/4]
|
protected |
Definition at line 356 of file ctk3slider.cpp.
◆ ctk3Slider() [4/4]
|
protected |
Definition at line 347 of file ctk3slider.cpp.
Member Function Documentation
◆ event()
|
overrideprotectedvirtual |
Reimplemented from QSlider.
Definition at line 973 of file ctk3slider.cpp.
◆ handleToolTip()
QString ctk3Slider::handleToolTip | ( | ) | const |
Controls the text to display for the handle tooltip.
It is in addition to the widget tooltip. "%1" is replaced by the current value of the slider. Empty string (by default) means no tooltip.
Definition at line 959 of file ctk3slider.cpp.
◆ initMaximumSliderStyleOption()
|
protectedvirtual |
Definition at line 948 of file ctk3slider.cpp.
◆ initMidSliderStyleOption()
|
protectedvirtual |
Definition at line 953 of file ctk3slider.cpp.
◆ initMinimumSliderStyleOption()
|
protectedvirtual |
Definition at line 942 of file ctk3slider.cpp.
◆ isMaximumSliderDown()
bool ctk3Slider::isMaximumSliderDown | ( | ) | const |
Returns true if the maximum value handle is down, false if it is up.
- See also
- isMinimumSliderDown()
Definition at line 929 of file ctk3slider.cpp.
◆ isMidSliderDown()
bool ctk3Slider::isMidSliderDown | ( | ) | const |
Returns true if the mid value handle is down, false if it is up.
- See also
- isMinimumSliderDown()
Definition at line 935 of file ctk3slider.cpp.
◆ isMinimumSliderDown()
bool ctk3Slider::isMinimumSliderDown | ( | ) | const |
Returns true if the minimum value handle is down, false if it is up.
- See also
- isMaximumSliderDown()
Definition at line 922 of file ctk3slider.cpp.
◆ maximumPosition()
int ctk3Slider::maximumPosition | ( | ) | const |
This property holds the current slider maximum position.
If tracking is enabled (the default), this is identical to maximumValue.
Definition at line 549 of file ctk3slider.cpp.
◆ maximumPositionChanged
|
signal |
This signal is emitted when sliderDown is true and the slider moves.
This usually happens when the user is dragging the maximum slider. The value is the new slider maximum position. This signal is emitted even when tracking is turned off.
◆ maximumValue()
int ctk3Slider::maximumValue | ( | ) | const |
This property holds the slider's current maximum value.
The slider forces the maximum value to be within the legal range: The slider silently forces maximumValue to be within the legal range: Changing the maximumValue also changes the maximumPosition.
Definition at line 387 of file ctk3slider.cpp.
◆ maximumValueChanged
|
signal |
This signal is emitted when the slider maximum value has changed, with the new slider value as argument.
◆ midPosition()
int ctk3Slider::midPosition | ( | ) | const |
This property holds the current slider mid position.
If tracking is enabled (the default), this is identical to midValue.
Definition at line 555 of file ctk3slider.cpp.
◆ midPositionChanged
|
signal |
This signal is emitted when sliderDown is true and the slider moves.
This usually happens when the user is dragging the mid slider. The value is the new slider mid position. This signal is emitted even when tracking is turned off.
◆ midValue()
int ctk3Slider::midValue | ( | ) | const |
This property holds the slider's current mid value.
The slider forces the mid value to be within the legal range: The slider silently forces midValue to be within the legal range: Changing the midValue also changes the midPosition.
Definition at line 403 of file ctk3slider.cpp.
◆ midValueChanged
|
signal |
This signal is emitted when the slider mid value has changed, with the new slider value as argument.
◆ minimumPosition()
int ctk3Slider::minimumPosition | ( | ) | const |
This property holds the current slider minimum position.
If tracking is enabled (the default), this is identical to minimumValue.
Definition at line 542 of file ctk3slider.cpp.
◆ minimumPositionChanged
|
signal |
This signal is emitted when sliderDown is true and the slider moves.
This usually happens when the user is dragging the minimum slider. The value is the new slider minimum position. This signal is emitted even when tracking is turned off.
◆ minimumValue()
int ctk3Slider::minimumValue | ( | ) | const |
This property holds the slider's current minimum value.
The slider silently forces minimumValue to be within the legal range: minimum() <= minimumValue() <= maximumValue() <= maximum(). Changing the minimumValue also changes the minimumPosition.
Definition at line 371 of file ctk3slider.cpp.
◆ minimumValueChanged
|
signal |
This signal is emitted when the slider minimum value has changed, with the new slider value as argument.
◆ mouseMoveEvent()
|
overrideprotectedvirtual |
Reimplemented from QSlider.
Definition at line 840 of file ctk3slider.cpp.
◆ mousePressEvent()
|
overrideprotectedvirtual |
Reimplemented from QSlider.
Definition at line 763 of file ctk3slider.cpp.
◆ mouseReleaseEvent()
|
overrideprotectedvirtual |
Reimplemented from QSlider.
Definition at line 909 of file ctk3slider.cpp.
◆ onRangeChanged
|
protectedslot |
Definition at line 644 of file ctk3slider.cpp.
◆ paintEvent()
|
overrideprotectedvirtual |
Reimplemented from QSlider.
Definition at line 654 of file ctk3slider.cpp.
◆ positionsChanged
|
signal |
Utility signal that is fired when minimum or maximum positions have changed.
◆ setHandleToolTip()
void ctk3Slider::setHandleToolTip | ( | const QString & | toolTip | ) |
Definition at line 966 of file ctk3slider.cpp.
◆ setMaximumPosition()
void ctk3Slider::setMaximumPosition | ( | int | max | ) |
Definition at line 571 of file ctk3slider.cpp.
◆ setMaximumValue
|
slot |
This property holds the slider's current maximum value.
The slider silently forces max to be within the legal range: minimum() <= minimumValue() <= mid <= max <= maximum(). Note: Changing the maximumValue also changes the maximumPosition.
- See also
- stMinimumValue, setValues, setMinimum, setMaximum, setRange
Definition at line 394 of file ctk3slider.cpp.
◆ setMidPosition()
void ctk3Slider::setMidPosition | ( | int | mid | ) |
Definition at line 579 of file ctk3slider.cpp.
◆ setMidValue
|
slot |
This property holds the slider's current mid value.
The slider silently forces max to be within the legal range: minimum() <= minimumValue() <= mid <= max <= maximum(). Note: Changing the midValue also changes the midPosition.
- See also
- setMidValue, setValues, setMinimum, setMaximum, setRange
Definition at line 410 of file ctk3slider.cpp.
◆ setMinimumPosition()
void ctk3Slider::setMinimumPosition | ( | int | min | ) |
Definition at line 562 of file ctk3slider.cpp.
◆ setMinimumValue
|
slot |
This property holds the slider's current minimum value.
The slider silently forces min to be within the legal range: minimum() <= min <= mid <= maximumValue() <= maximum(). Note: Changing the minimumValue also changes the minimumPosition.
- See also
- stMaximumValue, setValues, setMinimum, setMaximum, setRange
Definition at line 378 of file ctk3slider.cpp.
◆ setPositions()
void ctk3Slider::setPositions | ( | int | min, |
int | mid, | ||
int | max ) |
Utility function that set the minimum, mid and maximum position at once.
Definition at line 589 of file ctk3slider.cpp.
◆ setValues
|
slot |
Utility function that set the minimum value and maximum value at once.
The slider silently forces min and max to be within the legal range: minimum() <= min <= max <= maximum(). Note: Changing the minimumValue and maximumValue also changes the minimumPosition and maximumPosition.
- See also
- setMinimumValue, setMaximumValue, setMinimum, setMaximum, setRange
Definition at line 470 of file ctk3slider.cpp.
◆ valuesChanged
|
signal |
Utility signal that is fired when minimum, mid or maximum values have changed.
Member Data Documentation
◆ d_ptr
|
protected |
Definition at line 217 of file ctk3slider.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.