kviewshell
DjVuPalette Class Reference
#include <DjVuPalette.h>
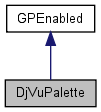
Public Member Functions | |
void | color_correct (double corr) |
int | color_to_index (const unsigned char *bgr) |
int | color_to_index (const GPixel &p) |
int | compute_palette (int maxcolors, int minboxsize=0) |
int | compute_palette_and_quantize (GPixmap &pm, int maxcolors, int minboxsize=0) |
int | compute_pixmap_palette (const GPixmap &pm, int ncolors, int minboxsize=0) |
void | decode (GP< ByteStream > bs) |
void | decode_rgb_entries (ByteStream &bs, const int palettesize) |
DjVuPalette (const DjVuPalette &ref) | |
void | encode (GP< ByteStream > bs) const |
void | encode_rgb_entries (ByteStream &bs) const |
void | get_color (int nth, GPixel &out) const |
void | histogram_add (const unsigned char *bgr, int weight) |
void | histogram_add (const GPixel &p, int weight) |
void | histogram_clear () |
void | histogram_norm_and_add (const int *bgr, int weight) |
void | index_to_color (int index, unsigned char *bgr) const |
void | index_to_color (int index, GPixel &p) const |
DjVuPalette & | operator= (const DjVuPalette &ref) |
void | quantize (GPixmap &pm) |
int | size () const |
~DjVuPalette () | |
Static Public Member Functions | |
static GP< DjVuPalette > | create (void) |
Public Attributes | |
GTArray< short > | colordata |
Protected Member Functions | |
DjVuPalette (void) |
Detailed Description
Definition at line 122 of file DjVuPalette.h.
Constructor & Destructor Documentation
DjVuPalette::DjVuPalette | ( | void | ) | [protected] |
Definition at line 109 of file DjVuPalette.cpp.
DjVuPalette::~DjVuPalette | ( | ) |
DjVuPalette::DjVuPalette | ( | const DjVuPalette & | ref | ) |
Definition at line 134 of file DjVuPalette.cpp.
Member Function Documentation
void DjVuPalette::color_correct | ( | double | corr | ) |
Applies a luminance gamma correction factor of corr# to the palette entries.
Values greater than #1.0# make the image brighter. Values smaller than #1.0# make the image darker. The documentation of program {ppmcoco} explains how to properly use this function.
Definition at line 439 of file DjVuPalette.cpp.
int DjVuPalette::color_to_index | ( | const unsigned char * | bgr | ) | [inline] |
Returns the best palette index for representing color bgr#.
Definition at line 286 of file DjVuPalette.h.
int DjVuPalette::color_to_index | ( | const GPixel & | p | ) | [inline] |
Returns the best palette index for representing color p#.
Definition at line 298 of file DjVuPalette.h.
int DjVuPalette::compute_palette | ( | int | maxcolors, | |
int | minboxsize = 0 | |||
) |
Computes an optimal palette for representing an image where colors appear according to the histogram.
Argument maxcolors# is the maximum number of colors allowed in the palette (up to 1024). Argument minboxsize# controls the minimal size of the color cube area affected to a color palette entry. Returns the index of the dominant color.
Definition at line 221 of file DjVuPalette.cpp.
int DjVuPalette::compute_palette_and_quantize | ( | GPixmap & | pm, | |
int | maxcolors, | |||
int | minboxsize = 0 | |||
) |
int DjVuPalette::compute_pixmap_palette | ( | const GPixmap & | pm, | |
int | ncolors, | |||
int | minboxsize = 0 | |||
) |
Computes the optimal palette for pixmap pm#.
This function builds the histogram for pixmap pm# and computes the optimal palette using {compute_palette}.
Definition at line 354 of file DjVuPalette.cpp.
static GP<DjVuPalette> DjVuPalette::create | ( | void | ) | [inline, static] |
void DjVuPalette::decode | ( | GP< ByteStream > | bs | ) |
Initializes the object by reading data from bytestream bs#.
This function reads a version byte, the palette size, the palette and the color index sequence from bytestream bs#. Note that the color histogram is never saved.
Definition at line 535 of file DjVuPalette.cpp.
void DjVuPalette::decode_rgb_entries | ( | ByteStream & | bs, | |
const int | palettesize | |||
) |
Reads palette colors.
This function initializes the palette colors by reading palettesize# RGB triples from bytestream bs#.
Definition at line 520 of file DjVuPalette.cpp.
void DjVuPalette::encode | ( | GP< ByteStream > | bs | ) | const |
Encodes the palette and the color index sequence.
This function encodes the a version byte, the palette size, the palette colors and the color index sequence into bytestream bs#. Note that the color histogram is never saved.
Definition at line 489 of file DjVuPalette.cpp.
void DjVuPalette::encode_rgb_entries | ( | ByteStream & | bs | ) | const |
Writes the palette colors.
This function writes each palette color as a RGB triple into bytestream bs#.
Definition at line 475 of file DjVuPalette.cpp.
void DjVuPalette::get_color | ( | int | nth, | |
GPixel & | out | |||
) | const [inline] |
Returns colors from the color index sequence.
Pixel out# is overwritten with the color corresponding to the nth# element of the color sequence {colordata}.
Definition at line 319 of file DjVuPalette.h.
void DjVuPalette::histogram_add | ( | const unsigned char * | bgr, | |
int | weight | |||
) | [inline] |
Adds the color specified by the triple bgr# to the histogram.
Argument weight# represent the number of pixels with this color.
Definition at line 247 of file DjVuPalette.h.
void DjVuPalette::histogram_add | ( | const GPixel & | p, | |
int | weight | |||
) | [inline] |
Adds the color specified by p# to the histogram.
Argument weight# represent the number of pixels with this color.
Definition at line 259 of file DjVuPalette.h.
void DjVuPalette::histogram_clear | ( | ) | [inline] |
void DjVuPalette::histogram_norm_and_add | ( | const int * | bgr, | |
int | weight | |||
) | [inline] |
Adds the color specified by the weighted triple bgr# to the histogram.
Argument weight# represent the number of pixels with this color. This function will compute the actual color by dividing the elements of the bgr# array by weight# and then use the unnormalized values to compute the average color per bucket. This is all a way to avoid excessive loss of accuracy.
Definition at line 265 of file DjVuPalette.h.
void DjVuPalette::index_to_color | ( | int | index, | |
unsigned char * | bgr | |||
) | const [inline] |
Overwrites rgb[0.
.3]# with the color located at position index# in the palette.
Definition at line 304 of file DjVuPalette.h.
void DjVuPalette::index_to_color | ( | int | index, | |
GPixel & | p | |||
) | const [inline] |
Overwrites p# with the color located at position index# in the palette.
Definition at line 313 of file DjVuPalette.h.
DjVuPalette & DjVuPalette::operator= | ( | const DjVuPalette & | ref | ) |
Definition at line 121 of file DjVuPalette.cpp.
void DjVuPalette::quantize | ( | GPixmap & | pm | ) |
Quantizes pixmap pm#.
All pixels are replaced by their closest approximation available in the palette.
Definition at line 420 of file DjVuPalette.cpp.
int DjVuPalette::size | ( | void | ) | const [inline] |
Member Data Documentation
GTArray<short> DjVuPalette::colordata |
Contains an optional sequence of color indices.
Function {encode} and {decode} also encode and decode this sequence when such a sequence is provided.
Definition at line 188 of file DjVuPalette.h.
The documentation for this class was generated from the following files: