kviewshell
GIFFManager Class Reference
Intuitive interface to IFF files. More...
#include <GIFFManager.h>
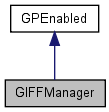
Public Member Functions | |
void | add_chunk (GUTF8String name, const TArray< char > &data) |
void | add_chunk (GUTF8String parent_name, const GP< GIFFChunk > &chunk, int pos=-1) |
void | del_chunk (void) |
void | del_chunk (GUTF8String name) |
GP< GIFFChunk > | get_chunk (GUTF8String name, int *position=0) |
int | get_chunks_number (void) |
int | get_chunks_number (const GUTF8String &name) |
void | load_chunk (IFFByteStream &istr, GP< GIFFChunk > chunk) |
void | load_file (const TArray< char > &data) |
void | load_file (GP< ByteStream > str) |
void | save_file (TArray< char > &data) |
void | save_file (GP< ByteStream > str) |
virtual | ~GIFFManager (void) |
Static Public Member Functions | |
static GP< GIFFManager > | create (const GUTF8String &name) |
static GP< GIFFManager > | create (void) |
GIFFManager.h | |
Files #"GIFFManager.h"# and #"GIFFManager.cpp"# define more convenient interface to IFF files. You may want to use the { GIFFManager} class instead of coping with { IFFByteStream} especially when you have to insert or move chunks, which is a kind of tricky with sequential access provided by { IFFByteStream}. You will mostly deal with { GIFFManager} class, but sometimes you may want to use { GIFFChunk}s as well thus bypassing { GIFFManager}'s interface and working with the chunks hierarchy yourself. Interface to IFF files.
| |
GIFFManager (void) | |
void | init (const GUTF8String &name) |
void | init (void) |
void | set_name (const GUTF8String &name) |
Detailed Description
Intuitive interface to IFF files.It's too terrible to keep reading/writing IFF files chunk after chunk using { IFFByteStream}s. This class allows you to operate with chunks as with structures or arrays without even caring about the byte streams.
Some of the examples are below: {verbatim} GP<GIFFChunk> chunk; chunk=manager1.get_chunk("BG44[2]"); manager2.add_chunk(".FORM:DJVU.BG44[-1]", chunk); {verbatim}
{ Chunk name} {itemize} Every chunk name may contain optional prefix FORM:#, LIST:#, PROP:# or CAT:#. If the prefix is omitted and the chunk happens to contain other chunks, FORM:# will be assumed. Every chunk name may be {short} or {complete}. {short} chunk names may not contain dots as they're a subchunks names with respect to a given chunk. {complete} chunk names may contain dots. But there may be or may not be the {leading dot} in the name. If the {leading dot} is present, then the name is assumed to contain the name of the top-level chunk as well. Otherwise it's treated {with respect} to the top-level chunk. You may want to use the leading dot only when you add a chunk to an empty document, since a command like manager.addChunk(".FORM:DJVU.BG44", chunk)# will create the top level chunk of the requested type (FORM:DJVU#) and will add chunk BG44# to it {automatically}. You may use {brackets} in the name to specify the chunk's position. The meaning of the number inside the brackets depends on the function you call. In most of the cases this is the number of the chunk with the given name in the parent chunk. But sometimes (as in addChunk(name, buffer, length)#) the brackets at the end of the name# actually specify the {position} of the chunk in the parent. For example, to insert INCL# chunk into DJVU# form at position #1# (make it the second) you may want to use manager.addChunk(".DJVU.INCL[1]", data, size)#. At the same time, to get 2-nd chunk with name BG44# from form DJVU# you should do smth like chunk=manager.getChunk("BG44[1]")#. Note, that here the manager will search for chunk BG44# in form DJVU# and will take the second {found} one. {itemize}
Definition at line 248 of file GIFFManager.h.
Constructor & Destructor Documentation
GIFFManager::GIFFManager | ( | void | ) | [inline, protected] |
Definition at line 373 of file GIFFManager.h.
GIFFManager::~GIFFManager | ( | void | ) | [virtual] |
Member Function Documentation
void GIFFManager::add_chunk | ( | GUTF8String | name, | |
const TArray< char > & | data | |||
) |
If {name}={name1}.
{name2} where {name2} doesn't contain dots, then addChunk()# will create plain chunk with name {name2} with data {buffer} of size {length} and will add it to chunk {name1} in the same way as addChunk(name, chunk, pos)# function would do it. The pos# in this case is either #-1# (append) or is extracted from between brackets if the {name} ends with them.
{ Examples:} {verbatim} ;; To insert INCL chunk at position 2 (make it 3rd) m.addChunk("INCL[2]", data, length); ;; To append chunk BG44 to 2nd DjVu file inside DjVm archive: m.addChunk(".DJVM.DJVU[1].BG44", data, length); {verbatim}
Definition at line 414 of file GIFFManager.cpp.
void GIFFManager::add_chunk | ( | GUTF8String | parent_name, | |
const GP< GIFFChunk > & | chunk, | |||
int | pos = -1 | |||
) |
Adds the chunk {chunk} to chunk with name {parent_name} at position {pos}.
{parent_name} may contain dots, brackets and colons. All missing chunks in the chain will be created.
{ Examples:} {verbatim} ;; To set the top-level chunk to 'ch' m.addChunk(".", ch); ;; To add 'ch' to the top-level chunk "DJVU" creating it if necessary m.addChunk(".DJVU", ch); ;; Same as above regardless of top-level chunk name m.addChunk("", ch); ;; To add 'ch' to 2nd FORM DJVU in top-level form DJVM m.addChunk(".FORM:DJVM.FORM:DJVU[1]", ch); ;; Same thing regardless of the top-level chunk name m.addChunk("FORM:DJVU[1]", ch); {verbatim}
Definition at line 328 of file GIFFManager.cpp.
GP< GIFFManager > GIFFManager::create | ( | const GUTF8String & | name | ) | [static] |
Creates the { GIFFManager} and assigns name {name} to the top-level chunk.
you may use chunk type names (before colon) to set the top-level chunk type, or omit it to work with FORM#
Definition at line 91 of file GIFFManager.cpp.
GP< GIFFManager > GIFFManager::create | ( | void | ) | [static] |
void GIFFManager::del_chunk | ( | void | ) |
Definition at line 453 of file GIFFManager.cpp.
void GIFFManager::del_chunk | ( | GUTF8String | name | ) |
Will remove chunk with name {name}.
You may use dots, colons and brackets to specify the chunk uniquely.
{ Examples:} {verbatim} ;; To remove 2nd DjVu document from DjVm archive use m.delChunk(".DJVM.DJVU[1]"); ;; Same thing without top-level chunk name specification m.delChunk("DJVU[1]"); ;; Same thing for the first DJVU chunk m.delChunk("DJVU"); {verbatim}
Definition at line 462 of file GIFFManager.cpp.
GP< GIFFChunk > GIFFManager::get_chunk | ( | GUTF8String | name, | |
int * | position = 0 | |||
) |
Returns the chunk with name {name}.
The {name} may contain dots colons and slashes. If {position} is not zero, #*position# will be assigned the position of the found chunk in the parent chunk.
{ Examples:} {verbatim} ;; To get the directory chunk of DjVm document m.getChunk(".DJVM.DIR0"); ;; To get chunk corresponding to 2nd DJVU form m.getChunk(".DJVU[1]"); {verbatim}
Definition at line 512 of file GIFFManager.cpp.
int GIFFManager::get_chunks_number | ( | void | ) |
Definition at line 556 of file GIFFManager.cpp.
int GIFFManager::get_chunks_number | ( | const GUTF8String & | name | ) |
Will return the number of chunks with given name.
The {name} may not end with brackets, but may contain them inside. It may also contain dots and colons. If {name} is ZERO, the total number of chunks will be returned.
{ Examples:} {verbatim} ;; To get the number of DJVU forms inside DjVm document m.getChunksNumber(".DJVM.DJVU"); ;; Same thing without top-level chunk name specification m.getChunksNumber("DJVU"); {verbatim}
Definition at line 564 of file GIFFManager.cpp.
void GIFFManager::init | ( | const GUTF8String & | name | ) | [inline, protected] |
Definition at line 382 of file GIFFManager.h.
void GIFFManager::init | ( | void | ) | [inline, protected] |
Definition at line 376 of file GIFFManager.h.
void GIFFManager::load_chunk | ( | IFFByteStream & | istr, | |
GP< GIFFChunk > | chunk | |||
) |
Loads the composite {chunk}'s contents from stream {istr}.
Definition at line 589 of file GIFFManager.cpp.
void GIFFManager::load_file | ( | const TArray< char > & | data | ) |
void GIFFManager::load_file | ( | GP< ByteStream > | str | ) |
void GIFFManager::save_file | ( | TArray< char > & | data | ) |
void GIFFManager::save_file | ( | GP< ByteStream > | str | ) |
void GIFFManager::set_name | ( | const GUTF8String & | name | ) | [inline] |
The documentation for this class was generated from the following files: