kio
KIO::ProgressBase Class Reference
This class does all initialization stuff for progress, like connecting signals to slots. More...
#include <progressbase.h>
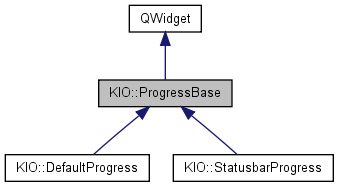
Public Slots | |
virtual void | slotCanResume (KIO::Job *job, KIO::filesize_t from) |
virtual void | slotClean () |
virtual void | slotCopying (KIO::Job *job, const KURL &src, const KURL &dest) |
virtual void | slotCreatingDir (KIO::Job *job, const KURL &dir) |
virtual void | slotDeleting (KIO::Job *job, const KURL &url) |
virtual void | slotMoving (KIO::Job *job, const KURL &src, const KURL &dest) |
virtual void | slotPercent (KIO::Job *job, unsigned long percent) |
virtual void | slotProcessedDirs (KIO::Job *job, unsigned long dirs) |
virtual void | slotProcessedFiles (KIO::Job *job, unsigned long files) |
virtual void | slotProcessedSize (KIO::Job *job, KIO::filesize_t bytes) |
virtual void | slotSpeed (KIO::Job *job, unsigned long speed) |
void | slotStop () |
virtual void | slotTotalDirs (KIO::Job *job, unsigned long dirs) |
virtual void | slotTotalFiles (KIO::Job *job, unsigned long files) |
virtual void | slotTotalSize (KIO::Job *job, KIO::filesize_t size) |
Signals | |
void | stopped () |
Public Member Functions | |
void | finished () |
bool | onlyClean () const |
ProgressBase (QWidget *parent) | |
void | setJob (KIO::DeleteJob *job) |
void | setJob (KIO::CopyJob *job) |
void | setJob (KIO::Job *job) |
void | setOnlyClean (bool onlyClean) |
void | setStopOnClose (bool stopOnClose) |
bool | stopOnClose () const |
~ProgressBase () | |
Protected Slots | |
void | slotFinished (KIO::Job *) |
Protected Member Functions | |
virtual void | closeEvent (QCloseEvent *) |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
KIO::Job * | m_pJob |
Detailed Description
This class does all initialization stuff for progress, like connecting signals to slots.All slots are implemented as pure virtual methods.
All custom IO progress dialog should inherit this class. Add your GUI code to the constructor and implemement those virtual methods which you need in order to display progress.
E.g. StatusbarProgress only implements slotTotalSize(), slotPercent() and slotSpeed().
Custom progress dialog will be used like this :
// create job CopyJob* job = KIO::copy(...); // create a dialog MyCustomProgress *customProgress; customProgress = new MyCustomProgress(); // connect progress with job customProgress->setJob( job ); ...
There is a special method setStopOnClose() that controls the behavior of the dialog. Base class for IO progress dialogs.
Definition at line 70 of file progressbase.h.
Constructor & Destructor Documentation
KIO::ProgressBase::ProgressBase | ( | QWidget * | parent | ) |
Creates a new progress dialog.
- Parameters:
-
parent the parent of this dialog window, or 0
Definition at line 24 of file progressbase.cpp.
KIO::ProgressBase::~ProgressBase | ( | ) | [inline] |
Definition at line 81 of file progressbase.h.
Member Function Documentation
void KIO::ProgressBase::closeEvent | ( | QCloseEvent * | ) | [protected, virtual] |
void KIO::ProgressBase::finished | ( | ) |
bool KIO::ProgressBase::onlyClean | ( | ) | const [inline] |
Checks whether the dialog should be deleted or cleaned.
- Returns:
- true if the dialog only calls slotClean, false if it will be deleted
- See also:
- setOnlyClean()
Definition at line 123 of file progressbase.h.
void KIO::ProgressBase::setJob | ( | KIO::DeleteJob * | job | ) |
Assign a KIO::Job to this progress dialog.
- Parameters:
-
job the job to assign
Definition at line 94 of file progressbase.cpp.
void KIO::ProgressBase::setJob | ( | KIO::CopyJob * | job | ) |
Assign a KIO::Job to this progress dialog.
- Parameters:
-
job the job to assign
Definition at line 54 of file progressbase.cpp.
void KIO::ProgressBase::setJob | ( | KIO::Job * | job | ) |
Assign a KIO::Job to this progress dialog.
- Parameters:
-
job the job to assign
Reimplemented in KIO::StatusbarProgress.
Definition at line 37 of file progressbase.cpp.
void KIO::ProgressBase::setOnlyClean | ( | bool | onlyClean | ) | [inline] |
This controls whether the dialog should be deleted or only cleaned when the KIO::Job is finished (or canceled).
If your dialog is an embedded widget and not a separate window, you should setOnlyClean(true) in the constructor of your custom dialog.
- Parameters:
-
onlyClean If true the dialog will only call method slotClean. If false the dialog will be deleted.
- See also:
- onlyClean()
Definition at line 115 of file progressbase.h.
void KIO::ProgressBase::setStopOnClose | ( | bool | stopOnClose | ) | [inline] |
Definition at line 100 of file progressbase.h.
virtual void KIO::ProgressBase::slotCanResume | ( | KIO::Job * | job, | |
KIO::filesize_t | from | |||
) | [inline, virtual, slot] |
Called when the job is resuming.
- Parameters:
-
job the KIO::Job from the position to resume from in bytes
Reimplemented in KIO::DefaultProgress.
Definition at line 240 of file progressbase.h.
void KIO::ProgressBase::slotClean | ( | ) | [virtual, slot] |
This method is called when the widget should be cleaned (after job is finished).
redefine this for custom behavior.
Reimplemented in KIO::DefaultProgress, and KIO::StatusbarProgress.
Definition at line 170 of file progressbase.cpp.
virtual void KIO::ProgressBase::slotCopying | ( | KIO::Job * | job, | |
const KURL & | src, | |||
const KURL & | dest | |||
) | [inline, virtual, slot] |
Called when the job is copying.
- Parameters:
-
job the KIO::Job src the source of the operation dest the destination of the operation
Reimplemented in KIO::DefaultProgress.
Definition at line 210 of file progressbase.h.
virtual void KIO::ProgressBase::slotCreatingDir | ( | KIO::Job * | job, | |
const KURL & | dir | |||
) | [inline, virtual, slot] |
Called when the job is creating a directory.
- Parameters:
-
job the KIO::Job dir the URL of the directory to create
Reimplemented in KIO::DefaultProgress.
Definition at line 232 of file progressbase.h.
virtual void KIO::ProgressBase::slotDeleting | ( | KIO::Job * | job, | |
const KURL & | url | |||
) | [inline, virtual, slot] |
Called when the job is deleting.
- Parameters:
-
job the KIO::Job url the URL to delete
Reimplemented in KIO::DefaultProgress.
Definition at line 225 of file progressbase.h.
void KIO::ProgressBase::slotFinished | ( | KIO::Job * | ) | [protected, slot] |
Definition at line 153 of file progressbase.cpp.
virtual void KIO::ProgressBase::slotMoving | ( | KIO::Job * | job, | |
const KURL & | src, | |||
const KURL & | dest | |||
) | [inline, virtual, slot] |
Called when the job is moving.
- Parameters:
-
job the KIO::Job src the source of the operation dest the destination of the operation
Reimplemented in KIO::DefaultProgress.
Definition at line 218 of file progressbase.h.
virtual void KIO::ProgressBase::slotPercent | ( | KIO::Job * | job, | |
unsigned long | percent | |||
) | [inline, virtual, slot] |
Called to set the percentage.
- Parameters:
-
job the KIO::Job percent the percentage
Reimplemented in KIO::DefaultProgress, and KIO::StatusbarProgress.
Definition at line 201 of file progressbase.h.
virtual void KIO::ProgressBase::slotProcessedDirs | ( | KIO::Job * | job, | |
unsigned long | dirs | |||
) | [inline, virtual, slot] |
Called to set the number of processed directories.
- Parameters:
-
job the KIO::Job dirs the number of directories
Reimplemented in KIO::DefaultProgress.
Definition at line 185 of file progressbase.h.
virtual void KIO::ProgressBase::slotProcessedFiles | ( | KIO::Job * | job, | |
unsigned long | files | |||
) | [inline, virtual, slot] |
Called to set the number of processed files.
- Parameters:
-
job the KIO::Job files the number of files
Reimplemented in KIO::DefaultProgress.
Definition at line 178 of file progressbase.h.
virtual void KIO::ProgressBase::slotProcessedSize | ( | KIO::Job * | job, | |
KIO::filesize_t | bytes | |||
) | [inline, virtual, slot] |
Called to set the processed size.
- Parameters:
-
job the KIO::Job bytes the processed size in bytes
Reimplemented in KIO::DefaultProgress.
Definition at line 171 of file progressbase.h.
virtual void KIO::ProgressBase::slotSpeed | ( | KIO::Job * | job, | |
unsigned long | speed | |||
) | [inline, virtual, slot] |
Called to set the speed.
- Parameters:
-
job the KIO::Job speed the speed in bytes/second
Reimplemented in KIO::DefaultProgress, and KIO::StatusbarProgress.
Definition at line 193 of file progressbase.h.
void KIO::ProgressBase::slotStop | ( | ) | [slot] |
This method should be called for correct cancellation of IO operation Connect this to the progress widgets buttons etc.
Definition at line 158 of file progressbase.cpp.
virtual void KIO::ProgressBase::slotTotalDirs | ( | KIO::Job * | job, | |
unsigned long | dirs | |||
) | [inline, virtual, slot] |
Called to set the total number of directories.
- Parameters:
-
job the KIO::Job dirs the number of directories
Reimplemented in KIO::DefaultProgress.
Definition at line 163 of file progressbase.h.
virtual void KIO::ProgressBase::slotTotalFiles | ( | KIO::Job * | job, | |
unsigned long | files | |||
) | [inline, virtual, slot] |
Called to set the total number of files.
- Parameters:
-
job the KIO::Job files the number of files
Reimplemented in KIO::DefaultProgress.
Definition at line 156 of file progressbase.h.
virtual void KIO::ProgressBase::slotTotalSize | ( | KIO::Job * | job, | |
KIO::filesize_t | size | |||
) | [inline, virtual, slot] |
Called to set the total size.
- Parameters:
-
job the KIO::Job size the total size in bytes
Reimplemented in KIO::DefaultProgress, and KIO::StatusbarProgress.
Definition at line 149 of file progressbase.h.
bool KIO::ProgressBase::stopOnClose | ( | ) | const [inline] |
Definition at line 101 of file progressbase.h.
void KIO::ProgressBase::stopped | ( | ) | [signal] |
Called when the operation stopped.
void KIO::ProgressBase::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Reimplemented in KIO::DefaultProgress, and KIO::StatusbarProgress.
Definition at line 174 of file progressbase.cpp.
Member Data Documentation
KIO::Job* KIO::ProgressBase::m_pJob [protected] |
Definition at line 256 of file progressbase.h.
The documentation for this class was generated from the following files: