kio
KIO::SlaveInterface Class Reference
There are two classes that specifies the protocol between application (KIO::Job) and kioslave. More...
#include <slaveinterface.h>
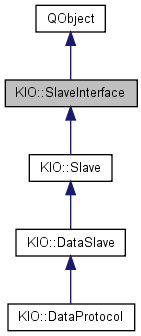
Signals | |
void | authorizationKey (const QCString &, const QCString &, bool) |
void | canResume (KIO::filesize_t) |
void | connected () |
void | connectFinished () |
void | data (const QByteArray &) |
void | dataReq () |
void | delAuthorization (const QCString &grpkey) |
void | error (int, const QString &) |
void | errorPage () |
void | finished () |
void | infoMessage (const QString &) |
void | listEntries (const KIO::UDSEntryList &) |
void | metaData (const KIO::MetaData &) |
void | mimeType (const QString &) |
void | needProgressId () |
void | needSubURLData () |
void | processedSize (KIO::filesize_t) |
void | redirection (const KURL &) |
void | slaveStatus (pid_t, const QCString &, const QString &, bool) |
void | speed (unsigned long) |
void | statEntry (const KIO::UDSEntry &) |
void | totalSize (KIO::filesize_t) |
void | warning (const QString &) |
Public Member Functions | |
Connection * | connection () const |
KIO::filesize_t | offset () const |
int | progressId () const |
void | sendResumeAnswer (bool resume) |
void | setConnection (Connection *connection) |
void | setOffset (KIO::filesize_t offset) |
void | setProgressId (int id) |
SlaveInterface (Connection *connection) | |
virtual | ~SlaveInterface () |
Protected Slots | |
void | calcSpeed () |
Protected Member Functions | |
virtual bool | dispatch (int _cmd, const QByteArray &data) |
virtual bool | dispatch () |
void | dropNetwork (const QString &, const QString &) |
void | messageBox (int type, const QString &text, const QString &caption, const QString &buttonYes, const QString &buttonNo, const QString &dontAskAgainName) |
void | messageBox (int type, const QString &text, const QString &caption, const QString &buttonYes, const QString &buttonNo) |
void | openPassDlg (const QString &prompt, const QString &user, bool readOnly) KDE_DEPRECATED |
void | openPassDlg (const QString &prompt, const QString &user, const QString &caption, const QString &comment, const QString &label, bool readOnly) KDE_DEPRECATED |
void | openPassDlg (KIO::AuthInfo &info) |
void | requestNetwork (const QString &, const QString &) |
virtual void | virtual_hook (int id, void *data) |
Static Protected Member Functions | |
static void | sigpipe_handler (int) |
Protected Attributes | |
Connection * | m_pConnection |
Detailed Description
There are two classes that specifies the protocol between application (KIO::Job) and kioslave.SlaveInterface is the class to use on the application end, SlaveBase is the one to use on the slave end.
A call to foo() results in a call to slotFoo() on the other end.
Definition at line 92 of file slaveinterface.h.
Constructor & Destructor Documentation
SlaveInterface::SlaveInterface | ( | Connection * | connection | ) |
Definition at line 132 of file slaveinterface.cpp.
SlaveInterface::~SlaveInterface | ( | ) | [virtual] |
Definition at line 141 of file slaveinterface.cpp.
Member Function Documentation
- Deprecated:
- . Obsolete as of 3.1. Replaced by kpassword, a kded module.
void SlaveInterface::calcSpeed | ( | ) | [protected, slot] |
Definition at line 176 of file slaveinterface.cpp.
void KIO::SlaveInterface::canResume | ( | KIO::filesize_t | ) | [signal] |
void KIO::SlaveInterface::connected | ( | ) | [signal] |
void KIO::SlaveInterface::connectFinished | ( | ) | [signal] |
Connection* KIO::SlaveInterface::connection | ( | ) | const [inline] |
Definition at line 101 of file slaveinterface.h.
void KIO::SlaveInterface::data | ( | const QByteArray & | ) | [signal] |
void KIO::SlaveInterface::dataReq | ( | ) | [signal] |
void KIO::SlaveInterface::delAuthorization | ( | const QCString & | grpkey | ) | [signal] |
- Deprecated:
- . Obsolete as of 3.1. Replaced by kpassword, a kded module.
bool SlaveInterface::dispatch | ( | int | _cmd, | |
const QByteArray & | data | |||
) | [protected, virtual] |
Definition at line 221 of file slaveinterface.cpp.
bool SlaveInterface::dispatch | ( | ) | [protected, virtual] |
Definition at line 163 of file slaveinterface.cpp.
Definition at line 435 of file slaveinterface.cpp.
void KIO::SlaveInterface::error | ( | int | , | |
const QString & | ||||
) | [signal] |
void KIO::SlaveInterface::errorPage | ( | ) | [signal] |
void KIO::SlaveInterface::finished | ( | ) | [signal] |
void KIO::SlaveInterface::infoMessage | ( | const QString & | ) | [signal] |
void KIO::SlaveInterface::listEntries | ( | const KIO::UDSEntryList & | ) | [signal] |
void SlaveInterface::messageBox | ( | int | type, | |
const QString & | text, | |||
const QString & | caption, | |||
const QString & | buttonYes, | |||
const QString & | buttonNo | |||
) | [protected] |
Definition at line 492 of file slaveinterface.cpp.
void KIO::SlaveInterface::metaData | ( | const KIO::MetaData & | ) | [signal] |
void KIO::SlaveInterface::mimeType | ( | const QString & | ) | [signal] |
void KIO::SlaveInterface::needProgressId | ( | ) | [signal] |
void KIO::SlaveInterface::needSubURLData | ( | ) | [signal] |
KIO::filesize_t SlaveInterface::offset | ( | ) | const |
Definition at line 424 of file slaveinterface.cpp.
void SlaveInterface::openPassDlg | ( | const QString & | prompt, | |
const QString & | user, | |||
bool | readOnly | |||
) | [protected] |
- Deprecated:
- . Use openPassDlg( AuthInfo& ) instead.
Definition at line 446 of file slaveinterface.cpp.
void SlaveInterface::openPassDlg | ( | const QString & | prompt, | |
const QString & | user, | |||
const QString & | caption, | |||
const QString & | comment, | |||
const QString & | label, | |||
bool | readOnly | |||
) | [protected] |
- Deprecated:
- . Use openPassDlg( AuthInfo& ) instead.
Definition at line 455 of file slaveinterface.cpp.
void SlaveInterface::openPassDlg | ( | KIO::AuthInfo & | info | ) | [protected] |
Prompt the user for authrization info (login & password).
Use this function to request authorization info from the the end user. For example to open an empty password dialog using default values:
KIO::AuthInfo authInfo; bool result = openPassDlg( authInfo ); if ( result ) { printf( "Username: %s", result.username.latin1() ); printf( "Username: %s", result.username.latin1() ); }
You can also pre-set some values like the username before hand if it is known as well as the comment and caption to be displayed:
authInfo.comment= "Enter username and password to access acmeone"; authInfo.caption= "Acme Password Dialog"; authInfo.username= "Wily E. kaiody"; bool result = openPassDlg( authInfo ); if ( result ) { printf( "Username: %s", result.username.latin1() ); printf( "Username: %s", result.username.latin1() ); }
NOTE: A call to this function can also fail and result in a return value of false
, if the UIServer could not be started for whatever reason.
- Parameters:
-
info See AuthInfo.
- Returns:
- true if user clicks on "OK", false otherwsie.
Definition at line 469 of file slaveinterface.cpp.
void KIO::SlaveInterface::processedSize | ( | KIO::filesize_t | ) | [signal] |
int KIO::SlaveInterface::progressId | ( | ) | const [inline] |
Definition at line 104 of file slaveinterface.h.
void KIO::SlaveInterface::redirection | ( | const KURL & | ) | [signal] |
Definition at line 426 of file slaveinterface.cpp.
void SlaveInterface::sendResumeAnswer | ( | bool | resume | ) |
Send our answer to the MSG_RESUME (canResume) request (to tell the "put" job whether to resume or not).
Definition at line 440 of file slaveinterface.cpp.
void KIO::SlaveInterface::setConnection | ( | Connection * | connection | ) | [inline] |
Definition at line 100 of file slaveinterface.h.
void SlaveInterface::setOffset | ( | KIO::filesize_t | offset | ) |
Definition at line 419 of file slaveinterface.cpp.
void KIO::SlaveInterface::setProgressId | ( | int | id | ) | [inline] |
Definition at line 103 of file slaveinterface.h.
void SlaveInterface::sigpipe_handler | ( | int | ) | [static, protected] |
void KIO::SlaveInterface::slaveStatus | ( | pid_t | , | |
const QCString & | , | |||
const QString & | , | |||
bool | ||||
) | [signal] |
void KIO::SlaveInterface::speed | ( | unsigned | long | ) | [signal] |
void KIO::SlaveInterface::statEntry | ( | const KIO::UDSEntry & | ) | [signal] |
void KIO::SlaveInterface::totalSize | ( | KIO::filesize_t | ) | [signal] |
void SlaveInterface::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
void KIO::SlaveInterface::warning | ( | const QString & | ) | [signal] |
Member Data Documentation
Connection* KIO::SlaveInterface::m_pConnection [protected] |
Definition at line 241 of file slaveinterface.h.
The documentation for this class was generated from the following files: