kio
KURLRequester Class Reference
This class is a widget showing a lineedit and a button, which invokes a filedialog. More...
#include <kurlrequester.h>
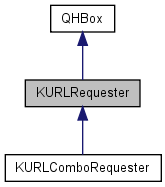
Public Slots | |
void | clear () |
virtual void | setCaption (const QString &caption) |
void | setKURL (const KURL &url) |
void | setURL (const QString &url) |
Signals | |
void | openFileDialog (KURLRequester *) |
void | returnPressed (const QString &) |
void | returnPressed () |
void | textChanged (const QString &) |
void | urlSelected (const QString &) |
Public Member Functions | |
KPushButton * | button () const |
KComboBox * | comboBox () const |
KURLCompletion * | completionObject () const |
KEditListBox::CustomEditor | customEditor () |
virtual KFileDialog * | fileDialog () const |
QString | filter () const |
KURLRequester (QWidget *editWidget, QWidget *parent, const char *name=0) | |
KURLRequester (const QString &url, QWidget *parent=0, const char *name=0) | |
KURLRequester (QWidget *parent=0, const char *name=0) | |
KLineEdit * | lineEdit () const |
uint | mode () const |
void | setFilter (const QString &filter) |
void | setMode (uint m) |
void | setShowLocalProtocol (bool b) |
bool | showLocalProtocol () const |
QString | url () const |
~KURLRequester () | |
Protected Slots | |
void | slotOpenDialog () |
Protected Member Functions | |
bool | eventFilter (QObject *obj, QEvent *ev) |
void | init () |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
KURLCompletion * | myCompletion |
Properties | |
QString | filter |
uint | mode |
bool | showLocalProtocol |
QString | url |
Detailed Description
This class is a widget showing a lineedit and a button, which invokes a filedialog.File name completion is available in the lineedit.
The defaults for the filedialog are to ask for one existing local file, i.e. KFileDialog::setMode( KFile::File | KFile::ExistingOnly | KFile::LocalOnly ) The default filter is "*", i.e. show all files, and the start directory is the current working directory, or the last directory where a file has been selected.
You can change this behavior by using setMode() or setFilter().
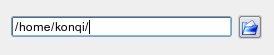
KDE URL Requester
Definition at line 56 of file kurlrequester.h.
Constructor & Destructor Documentation
KURLRequester::KURLRequester | ( | QWidget * | parent = 0 , |
|
const char * | name = 0 | |||
) |
Constructs a KURLRequester widget with the initial URL url
.
// TODO KDE4: Use KURL instead
Definition at line 182 of file kurlrequester.cpp.
Special constructor, which creates a KURLRequester widget with a custom edit-widget.
The edit-widget can be either a KComboBox or a KLineEdit (or inherited thereof). Note: for geometry management reasons, the edit-widget is reparented to have the KURLRequester as parent.
Definition at line 159 of file kurlrequester.cpp.
KURLRequester::~KURLRequester | ( | ) |
Member Function Documentation
KPushButton * KURLRequester::button | ( | ) | const |
- Returns:
- a pointer to the pushbutton. It is provided so that you can specify an own pixmap or a text, if you really need to.
Definition at line 398 of file kurlrequester.cpp.
void KURLRequester::clear | ( | ) | [slot] |
KComboBox * KURLRequester::comboBox | ( | ) | const |
- Returns:
- a pointer to the combobox, in case you have set one using the special constructor. Returns 0L otherwise.
Definition at line 374 of file kurlrequester.cpp.
KURLCompletion* KURLRequester::completionObject | ( | ) | const [inline] |
- Returns:
- the KURLCompletion object used in the lineedit/combobox.
Definition at line 175 of file kurlrequester.h.
KEditListBox::CustomEditor KURLRequester::customEditor | ( | ) |
- Returns:
- an object, suitable for use with KEditListBox. It allows you to put this KURLRequester into a KEditListBox. Basically, do it like this:
KURLRequester *req = new KURLRequester( someWidget ); [...] KEditListBox *editListBox = new KEditListBox( i18n("Some Title"), req->customEditor(), someWidget );
- Since:
- 3.1
Definition at line 403 of file kurlrequester.cpp.
Definition at line 387 of file kurlrequester.cpp.
KFileDialog * KURLRequester::fileDialog | ( | ) | const [virtual] |
- Returns:
- a pointer to the filedialog You can use this to customize the dialog, e.g. to specify a filter. Never returns 0L.
Definition at line 340 of file kurlrequester.cpp.
QString KURLRequester::filter | ( | ) | const |
Returns the current filter for the file dialog.
- See also:
- KFileDialog::filter()
- Since:
- 3.3
void KURLRequester::init | ( | ) | [protected] |
Definition at line 200 of file kurlrequester.cpp.
KLineEdit * KURLRequester::lineEdit | ( | ) | const |
- Returns:
- a pointer to the lineedit, either the default one, or the special one, if you used the special constructor.
Definition at line 369 of file kurlrequester.cpp.
uint KURLRequester::mode | ( | ) | const |
void KURLRequester::openFileDialog | ( | KURLRequester * | ) | [signal] |
Emitted before the filedialog is going to open.
Connect to this signal to "configure" the filedialog, e.g. set the filefilter, the mode, a preview-widget, etc. It's usually not necessary to set a URL for the filedialog, as it will get set properly from the editfield contents.
If you use multiple KURLRequesters, you can connect all of them to the same slot and use the given KURLRequester pointer to know which one is going to open.
void KURLRequester::returnPressed | ( | const QString & | ) | [signal] |
Emitted when return or enter was pressed in the lineedit.
The parameter contains the contents of the lineedit.
void KURLRequester::returnPressed | ( | ) | [signal] |
Emitted when return or enter was pressed in the lineedit.
void KURLRequester::setCaption | ( | const QString & | caption | ) | [virtual, slot] |
void KURLRequester::setFilter | ( | const QString & | filter | ) |
Sets the filter for the file dialog.
- See also:
- KFileDialog::setFilter()
Definition at line 327 of file kurlrequester.cpp.
void KURLRequester::setKURL | ( | const KURL & | url | ) | [slot] |
Sets the url in the lineedit to url
.
- Since:
- 3.4 // TODO KDE4: rename to setURL
Definition at line 253 of file kurlrequester.cpp.
void KURLRequester::setMode | ( | uint | m | ) |
Sets the mode of the file dialog.
Note: you can only select one file with the filedialog, so KFile::Files doesn't make much sense.
- See also:
- KFileDialog::setMode()
Definition at line 311 of file kurlrequester.cpp.
void KURLRequester::setShowLocalProtocol | ( | bool | b | ) |
Enables/disables showing file:/ in the lineedit, when a local file has been selected in the filedialog or was set via setURL().
Default is false, not showing file:/
- See also:
- showLocalProtocol
Definition at line 355 of file kurlrequester.cpp.
void KURLRequester::setURL | ( | const QString & | url | ) | [slot] |
Sets the url in the lineedit to url
.
Depending on the state of showLocalProtocol(), file:/ on local files will be shown or not.
- Since:
- 3.1 // TODO KDE4: Use KURL instead
Definition at line 235 of file kurlrequester.cpp.
bool KURLRequester::showLocalProtocol | ( | ) | const [inline] |
- Returns:
- whether local files will be prefixed with file:/ in the lineedit
- See also:
- setShowLocalProtocol
Definition at line 138 of file kurlrequester.h.
void KURLRequester::slotOpenDialog | ( | ) | [protected, slot] |
Called when the button is pressed to open the filedialog.
Also called when KStdAccel::Open (default is Ctrl-O) is pressed.
Definition at line 273 of file kurlrequester.cpp.
void KURLRequester::textChanged | ( | const QString & | ) | [signal] |
Emitted when the text in the lineedit changes.
The parameter contains the contents of the lineedit.
- Since:
- 3.1
QString KURLRequester::url | ( | ) | const |
- Returns:
- the current url in the lineedit. May be malformed, if the user entered something weird. ~user or environment variables are substituted for local files. // TODO KDE4: Use KURL so that the result is properly defined
void KURLRequester::urlSelected | ( | const QString & | ) | [signal] |
Emitted when the user changed the URL via the file dialog.
The parameter contains the contents of the lineedit. // TODO KDE4: Use KURL instead
void KURLRequester::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 421 of file kurlrequester.cpp.
Member Data Documentation
KURLCompletion* KURLRequester::myCompletion [protected] |
Definition at line 260 of file kurlrequester.h.
Property Documentation
QString KURLRequester::filter [read, write] |
Definition at line 61 of file kurlrequester.h.
unsigned int KURLRequester::mode [read, write] |
Definition at line 62 of file kurlrequester.h.
bool KURLRequester::showLocalProtocol [read, write] |
Definition at line 60 of file kurlrequester.h.
QString KURLRequester::url [read, write] |
Definition at line 59 of file kurlrequester.h.
The documentation for this class was generated from the following files: