kio
Observer Class Reference
Observer for KIO::Job progress information. More...
#include <observer.h>
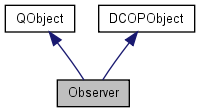
Public Slots | |
void | slotCanResume (KIO::Job *, KIO::filesize_t offset) |
void | slotCopying (KIO::Job *, const KURL &from, const KURL &to) |
void | slotCreatingDir (KIO::Job *, const KURL &dir) |
void | slotDeleting (KIO::Job *, const KURL &url) |
void | slotInfoMessage (KIO::Job *, const QString &msg) |
void | slotMoving (KIO::Job *, const KURL &from, const KURL &to) |
void | slotPercent (KIO::Job *, unsigned long percent) |
void | slotProcessedDirs (KIO::Job *, unsigned long dirs) |
void | slotProcessedFiles (KIO::Job *, unsigned long files) |
void | slotProcessedSize (KIO::Job *, KIO::filesize_t size) |
void | slotSpeed (KIO::Job *, unsigned long speed) |
void | slotTotalDirs (KIO::Job *, unsigned long dirs) |
void | slotTotalFiles (KIO::Job *, unsigned long files) |
void | slotTotalSize (KIO::Job *, KIO::filesize_t size) |
void | slotTransferring (KIO::Job *, const KURL &url) |
Public Member Functions | |
void | jobFinished (int progressId) |
void | mounting (KIO::Job *, const QString &dev, const QString &point) |
int | newJob (KIO::Job *job, bool showProgress) |
KIO::RenameDlg_Result | open_RenameDlg (KIO::Job *job, const QString &caption, const QString &src, const QString &dest, KIO::RenameDlg_Mode mode, QString &newDest, KIO::filesize_t sizeSrc=(KIO::filesize_t)-1, KIO::filesize_t sizeDest=(KIO::filesize_t)-1, time_t ctimeSrc=(time_t)-1, time_t ctimeDest=(time_t)-1, time_t mtimeSrc=(time_t)-1, time_t mtimeDest=(time_t)-1) |
KIO::SkipDlg_Result | open_SkipDlg (KIO::Job *job, bool multi, const QString &error_text) |
bool | openPassDlg (KIO::AuthInfo &info) |
bool | openPassDlg (const QString &prompt, QString &user, QString &pass, bool readOnly) |
void | stating (KIO::Job *, const KURL &url) |
void | unmounting (KIO::Job *, const QString &point) |
Static Public Member Functions | |
static int | messageBox (int progressId, int type, const QString &text, const QString &caption, const QString &buttonYes, const QString &buttonNo, const QString &dontAskAgainName) |
static int | messageBox (int progressId, int type, const QString &text, const QString &caption, const QString &buttonYes, const QString &buttonNo) |
static Observer * | self () |
Protected Member Functions | |
Observer () | |
virtual void | virtual_hook (int id, void *data) |
~Observer () | |
Protected Attributes | |
QIntDict< KIO::Job > | m_dctJobs |
UIServer_stub * | m_uiserver |
Static Protected Attributes | |
static Observer * | s_pObserver = 0L |
Detailed Description
Observer for KIO::Job progress information.This class, of which there is always only one instance, "observes" what jobs do and forwards this information to the progress-info server.
It is a DCOP object so that the UI server can call the kill method when the user presses Cancel.
Usually jobs are automatically registered by the KIO::Scheduler, so you do not have to care about that.
Observer for KIO::Job progress information
Definition at line 55 of file observer.h.
Constructor & Destructor Documentation
Observer::Observer | ( | ) | [protected] |
Definition at line 52 of file observer.cpp.
Observer::~Observer | ( | ) | [inline, protected] |
Definition at line 173 of file observer.h.
Member Function Documentation
void Observer::jobFinished | ( | int | progressId | ) |
Called by the job destructor, to tell the UI Server that the job ended.
- Parameters:
-
progressId the progress ID of the job, as returned by newJob()
Definition at line 93 of file observer.cpp.
void Observer::killJob | ( | int | progressId | ) |
Called by the UI Server (using DCOP) if the user presses cancel.
- Parameters:
-
progressId the progress ID of the job, as returned by newJob()
Definition at line 99 of file observer.cpp.
int Observer::messageBox | ( | int | progressId, | |
int | type, | |||
const QString & | text, | |||
const QString & | caption, | |||
const QString & | buttonYes, | |||
const QString & | buttonNo, | |||
const QString & | dontAskAgainName | |||
) | [static] |
Popup a message box.
See KIO::SlaveBase. This doesn't use DCOP anymore, it shows the dialog in the application's process. Otherwise, other apps would block when trying to communicate with UIServer.
- Parameters:
-
progressId the progress ID of the job, as returned by newJob() type the type of the message box text the text to show caption the window caption buttonYes the text of the "Yes" button buttonNo the text of the "No button dontAskAgainName A checkbox is added with which further confirmation can be turned off. The string is used to lookup and store the setting in kioslaverc.
- Since:
- 3.3
Definition at line 261 of file observer.cpp.
int Observer::messageBox | ( | int | progressId, | |
int | type, | |||
const QString & | text, | |||
const QString & | caption, | |||
const QString & | buttonYes, | |||
const QString & | buttonNo | |||
) | [static] |
Popup a message box.
See KIO::SlaveBase. This doesn't use DCOP anymore, it shows the dialog in the application's process. Otherwise, other apps would block when trying to communicate with UIServer.
- Parameters:
-
progressId the progress ID of the job, as returned by newJob() type the type of the message box text the text to show caption the window caption buttonYes the text of the "Yes" button buttonNo the text of the "No button
Definition at line 254 of file observer.cpp.
MetaData Observer::metadata | ( | int | progressId | ) |
Called by the UI Server (using DCOP) to get all the metadata of the job.
- Parameters:
-
progressId the progress IDof the job, as returned by newJob()
Definition at line 110 of file observer.cpp.
Definition at line 211 of file observer.cpp.
int Observer::newJob | ( | KIO::Job * | job, | |
bool | showProgress | |||
) |
Called by the job constructor, to signal its presence to the UI Server.
- Parameters:
-
job the new job showProgress true to show progress, false otherwise
- Returns:
- the progress ID assigned by the UI Server to the Job.
Definition at line 81 of file observer.cpp.
RenameDlg_Result Observer::open_RenameDlg | ( | KIO::Job * | job, | |
const QString & | caption, | |||
const QString & | src, | |||
const QString & | dest, | |||
KIO::RenameDlg_Mode | mode, | |||
QString & | newDest, | |||
KIO::filesize_t | sizeSrc = (KIO::filesize_t) -1 , |
|||
KIO::filesize_t | sizeDest = (KIO::filesize_t) -1 , |
|||
time_t | ctimeSrc = (time_t) -1 , |
|||
time_t | ctimeDest = (time_t) -1 , |
|||
time_t | mtimeSrc = (time_t) -1 , |
|||
time_t | mtimeDest = (time_t) -1 | |||
) |
SkipDlg_Result Observer::open_SkipDlg | ( | KIO::Job * | job, | |
bool | multi, | |||
const QString & | error_text | |||
) |
bool Observer::openPassDlg | ( | KIO::AuthInfo & | info | ) |
Opens a password dialog.
- Parameters:
-
info the authentication information
- Returns:
- true if successful ("ok" clicked), false otherwise
Definition at line 238 of file observer.cpp.
static Observer* Observer::self | ( | ) | [inline, static] |
Returns the unique observer object.
- Returns:
- the observer object
Definition at line 66 of file observer.h.
void Observer::slotCanResume | ( | KIO::Job * | job, | |
KIO::filesize_t | offset | |||
) | [slot] |
Definition at line 200 of file observer.cpp.
void Observer::slotCopying | ( | KIO::Job * | job, | |
const KURL & | from, | |||
const KURL & | to | |||
) | [slot] |
Definition at line 170 of file observer.cpp.
void Observer::slotCreatingDir | ( | KIO::Job * | job, | |
const KURL & | dir | |||
) | [slot] |
Definition at line 194 of file observer.cpp.
void Observer::slotDeleting | ( | KIO::Job * | job, | |
const KURL & | url | |||
) | [slot] |
Definition at line 182 of file observer.cpp.
Definition at line 165 of file observer.cpp.
void Observer::slotMoving | ( | KIO::Job * | job, | |
const KURL & | from, | |||
const KURL & | to | |||
) | [slot] |
Definition at line 176 of file observer.cpp.
void Observer::slotPercent | ( | KIO::Job * | job, | |
unsigned long | percent | |||
) | [slot] |
Definition at line 159 of file observer.cpp.
void Observer::slotProcessedDirs | ( | KIO::Job * | job, | |
unsigned long | dirs | |||
) | [slot] |
Definition at line 147 of file observer.cpp.
void Observer::slotProcessedFiles | ( | KIO::Job * | job, | |
unsigned long | files | |||
) | [slot] |
Definition at line 141 of file observer.cpp.
void Observer::slotProcessedSize | ( | KIO::Job * | job, | |
KIO::filesize_t | size | |||
) | [slot] |
Definition at line 135 of file observer.cpp.
void Observer::slotSpeed | ( | KIO::Job * | job, | |
unsigned long | speed | |||
) | [slot] |
Definition at line 153 of file observer.cpp.
void Observer::slotTotalDirs | ( | KIO::Job * | job, | |
unsigned long | dirs | |||
) | [slot] |
Definition at line 129 of file observer.cpp.
void Observer::slotTotalFiles | ( | KIO::Job * | job, | |
unsigned long | files | |||
) | [slot] |
Definition at line 123 of file observer.cpp.
void Observer::slotTotalSize | ( | KIO::Job * | job, | |
KIO::filesize_t | size | |||
) | [slot] |
Definition at line 117 of file observer.cpp.
void Observer::slotTransferring | ( | KIO::Job * | job, | |
const KURL & | url | |||
) | [slot] |
void Observer::stating | ( | KIO::Job * | job, | |
const KURL & | url | |||
) |
Definition at line 206 of file observer.cpp.
Definition at line 216 of file observer.cpp.
void Observer::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 414 of file observer.cpp.
Member Data Documentation
QIntDict< KIO::Job > Observer::m_dctJobs [protected] |
Definition at line 177 of file observer.h.
UIServer_stub* Observer::m_uiserver [protected] |
Definition at line 175 of file observer.h.
Observer * Observer::s_pObserver = 0L [static, protected] |
Definition at line 171 of file observer.h.
The documentation for this class was generated from the following files: