kmail
KMSearchRule Class Reference
Incoming mail is sent through the list of mail filter rules before it is placed in the associated mail folder (usually "inbox"). More...
#include <kmsearchpattern.h>
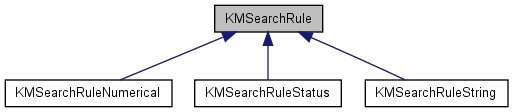
Public Types | |
enum | Function { FuncNone = -1, FuncContains = 0, FuncContainsNot, FuncEquals, FuncNotEqual, FuncRegExp, FuncNotRegExp, FuncIsGreater, FuncIsLessOrEqual, FuncIsLess, FuncIsGreaterOrEqual, FuncIsInAddressbook, FuncIsNotInAddressbook, FuncIsInCategory, FuncIsNotInCategory, FuncHasAttachment, FuncHasNoAttachment } |
Public Member Functions | |
const QString | asString () const |
QString | contents () const |
QCString | field () const |
Function | function () const |
virtual bool | isEmpty () const =0 |
KMSearchRule (const KMSearchRule &other) | |
KMSearchRule (const QCString &field=0, Function=FuncContains, const QString &contents=QString::null) | |
virtual bool | matches (const DwString &str, KMMessage &msg, const DwBoyerMoore *headerField=0, int headerLen=-1) const |
virtual bool | matches (const KMMessage *msg) const =0 |
const KMSearchRule & | operator= (const KMSearchRule &other) |
virtual bool | requiresBody () const |
void | setContents (const QString &aContents) |
void | setField (const QCString &field) |
void | setFunction (Function aFunction) |
void | writeConfig (KConfig *config, int aIdx) const |
virtual | ~KMSearchRule () |
Static Public Member Functions | |
static KMSearchRule * | createInstance (const KMSearchRule &other) |
static KMSearchRule * | createInstance (const QCString &field, const char *function, const QString &contents) |
static KMSearchRule * | createInstance (const QCString &field=0, Function function=FuncContains, const QString &contents=QString::null) |
static KMSearchRule * | createInstanceFromConfig (const KConfig *config, int aIdx) |
Detailed Description
Incoming mail is sent through the list of mail filter rules before it is placed in the associated mail folder (usually "inbox").This class represents one mail filter rule. It is also used to represent a search rule as used by the search dialog and folders.
This class represents one search pattern rule.
Definition at line 31 of file kmsearchpattern.h.
Member Enumeration Documentation
Operators for comparison of field and contents.
If you change the order or contents of the enum: do not forget to change funcConfigNames[], sFilterFuncList and matches() in KMSearchRule, too. Also, it is assumed that these functions come in pairs of logical opposites (ie. "=" <-> "!=", ">" <-> "<=", etc.).
- Enumerator:
Definition at line 41 of file kmsearchpattern.h.
Constructor & Destructor Documentation
KMSearchRule::KMSearchRule | ( | const QCString & | field = 0 , |
|
Function | func = FuncContains , |
|||
const QString & | contents = QString::null | |||
) |
Definition at line 73 of file kmsearchpattern.cpp.
KMSearchRule::KMSearchRule | ( | const KMSearchRule & | other | ) |
Definition at line 80 of file kmsearchpattern.cpp.
virtual KMSearchRule::~KMSearchRule | ( | ) | [inline, virtual] |
Definition at line 75 of file kmsearchpattern.h.
Member Function Documentation
const QString KMSearchRule::asString | ( | ) | const |
QString KMSearchRule::contents | ( | ) | const [inline] |
Return the value.
This can be either a substring to search for in or a regexp pattern to match against the header.
Definition at line 134 of file kmsearchpattern.h.
KMSearchRule * KMSearchRule::createInstance | ( | const KMSearchRule & | other | ) | [static] |
Definition at line 120 of file kmsearchpattern.cpp.
KMSearchRule * KMSearchRule::createInstance | ( | const QCString & | field, | |
const char * | function, | |||
const QString & | contents | |||
) | [static] |
Definition at line 113 of file kmsearchpattern.cpp.
KMSearchRule * KMSearchRule::createInstance | ( | const QCString & | field = 0 , |
|
Function | function = FuncContains , |
|||
const QString & | contents = QString::null | |||
) | [static] |
Create a search rule of a certain type by instantiating the appro- priate subclass depending on the field
.
Definition at line 98 of file kmsearchpattern.cpp.
KMSearchRule * KMSearchRule::createInstanceFromConfig | ( | const KConfig * | config, | |
int | aIdx | |||
) | [static] |
Initialize the object from a given config file.
The group must be preset. aIdx
is an identifier that is used to distinguish rules within a single config group. This function does no validation of the data obtained from the config file. You should call isEmpty yourself if you need valid rules.
Definition at line 125 of file kmsearchpattern.cpp.
QCString KMSearchRule::field | ( | ) | const [inline] |
Return message header field name (without the trailing ':').
There are also six pseudo-headers:
- <message>: Try to match against the whole message.
- <body>: Try to match against the body of the message.
- <any header>: Try to match against any header field.
- <recipients>: Try to match against both To: and Cc: header fields.
- <size>: Try to match against size of message (numerical).
- <age in days>: Try to match against age of message (numerical).
- <status>: Try to match against status of message (status).
Definition at line 126 of file kmsearchpattern.h.
Function KMSearchRule::function | ( | ) | const [inline] |
Return filter function.
This can be any of the operators defined in Function.
Definition at line 111 of file kmsearchpattern.h.
virtual bool KMSearchRule::isEmpty | ( | ) | const [pure virtual] |
Determine whether the rule is worth considering.
It isn't if either the field is not set or the contents is empty. KFilter should make sure that it's rule list contains only non-empty rules, as matches doesn't check this.
Implemented in KMSearchRuleString, KMSearchRuleNumerical, and KMSearchRuleStatus.
bool KMSearchRule::matches | ( | const DwString & | str, | |
KMMessage & | msg, | |||
const DwBoyerMoore * | headerField = 0 , |
|||
int | headerLen = -1 | |||
) | const [virtual] |
Optimized version tries to match the rule against the given.
- See also:
- DwString.
- Returns:
- TRUE if the rule matched, FALSE otherwise.
Reimplemented in KMSearchRuleString, and KMSearchRuleStatus.
Definition at line 172 of file kmsearchpattern.cpp.
virtual bool KMSearchRule::matches | ( | const KMMessage * | msg | ) | const [pure virtual] |
Tries to match the rule against the given KMMessage.
- Returns:
- TRUE if the rule matched, FALSE otherwise. Must be implemented by subclasses.
Implemented in KMSearchRuleString, KMSearchRuleNumerical, and KMSearchRuleStatus.
const KMSearchRule & KMSearchRule::operator= | ( | const KMSearchRule & | other | ) |
Definition at line 87 of file kmsearchpattern.cpp.
virtual bool KMSearchRule::requiresBody | ( | ) | const [inline, virtual] |
Returns true if the rule depends on a complete message, otherwise returns false.
Reimplemented in KMSearchRuleString.
Definition at line 99 of file kmsearchpattern.h.
void KMSearchRule::setContents | ( | const QString & | aContents | ) | [inline] |
void KMSearchRule::setField | ( | const QCString & | field | ) | [inline] |
Set message header field name (make sure there's no trailing colon ':').
Definition at line 130 of file kmsearchpattern.h.
void KMSearchRule::setFunction | ( | Function | aFunction | ) | [inline] |
void KMSearchRule::writeConfig | ( | KConfig * | config, | |
int | aIdx | |||
) | const |
Save the object into a given config file.
The group must be preset. aIdx
is an identifier that is used to distinguish rules within a single config group. This function will happily write itself even when it's not valid, assuming higher layers to Do The Right Thing(TM).
Definition at line 161 of file kmsearchpattern.cpp.
The documentation for this class was generated from the following files: