kmail
KMMessage Class Reference
This is a Mime Message. More...
#include <kmmessage.h>
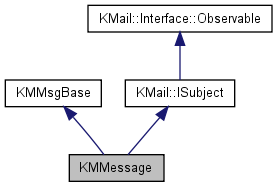
Public Types | |
enum | HeaderFieldType { Unstructured, Structured, Address } |
Public Member Functions | |
void | addBodyPart (const KMMessagePart *aPart) |
void | addDwBodyPart (DwBodyPart *aDwPart) |
void | applyIdentity (uint id) |
const DwMessage * | asDwMessage () |
const DwString & | asDwString () const |
QString | asPlainText (bool stripSignature, bool allowDecryption) const |
QString | asQuotedString (const QString &headerStr, const QString &indentStr, const QString &selection=QString::null, bool aStripSignature=true, bool allowDecryption=true) const |
QByteArray | asSendableString () const |
QCString | asString () const |
QString | bcc () const |
QCString | body () const |
QCString | bodyDecoded () const |
QByteArray | bodyDecodedBinary () const |
void | bodyPart (int aIdx, KMMessagePart *aPart) const |
QString | bodyToUnicode (const QTextCodec *codec=0) const |
QString | cc () const |
QString | ccStrip () const |
QCString | charset () const |
void | cleanupHeader () |
const QTextCodec * | codec () const |
int | contentTransferEncoding () const |
QCString | contentTransferEncodingStr () const |
KMMessage * | createDeliveryReceipt () const |
DwBodyPart * | createDWBodyPart (const KMMessagePart *aPart) |
KMMessage * | createForward (const QString &tmpl=QString::null) |
QCString | createForwardBody () |
KMMessage * | createMDN (KMime::MDN::ActionMode a, KMime::MDN::DispositionType d, bool allowGUI=false, QValueList< KMime::MDN::DispositionModifier > m=QValueList< KMime::MDN::DispositionModifier >()) |
KMMessage * | createRedirect (const QString &toStr) |
KMMessage * | createReply (KMail::ReplyStrategy replyStrategy=KMail::ReplySmart, QString selection=QString::null, bool noQuote=false, bool allowDecryption=true, bool selectionIsBody=false, const QString &tmpl=QString::null) |
int | cte () const |
QCString | cteStr () const |
time_t | date () const |
QString | dateIsoStr () const |
QCString | dateShortStr () const |
QString | dateStr () const |
void | del () |
void | deleteBodyParts () |
void | deleteWhenUnused () |
QString | drafts () const |
DwBodyPart * | dwBodyPart (int aIdx) const |
DwMediaType & | dwContentType () |
KMMsgEncryptionState | encryptionState () const |
KMime::Types::AddrSpecList | extractAddrSpecs (const QCString &headerNames) const |
QString | fcc () const |
QString | fileName () const |
DwBodyPart * | findDwBodyPart (DwBodyPart *part, const QString &partSpecifier) |
DwBodyPart * | findDwBodyPart (const QCString &type, const QCString &subtype) const |
DwBodyPart * | findDwBodyPart (int type, int subtype) const |
off_t | folderOffset () const |
QString | formatString (const QString &) const |
QString | from () const |
void | fromByteArray (const QByteArray &ba, bool setStatus=false) |
void | fromDwString (const DwString &str, bool setStatus=false) |
void | fromString (const QCString &str, bool setStatus=false) |
QString | fromStrip () const |
int | getCursorPos () |
DwBodyPart * | getFirstDwBodyPart () const |
void | getLink (int n, ulong *retMsgSerNum, KMMsgStatus *retStatus) const |
QCString | getRefStr () const |
DwMessage * | getTopLevelPart () const |
bool | hasUnencryptedMsg () const |
KMime::Types::AddressList | headerAddrField (const QCString &name) const |
QCString | headerAsSendableString () const |
QString | headerAsString () const |
QString | headerField (const QCString &name) const |
QStringList | headerFields (const QCString &name) const |
DwHeaders & | headers () const |
QCString | id () const |
uint | identityUoid () const |
void | initFromMessage (const KMMessage *msg, bool idHeaders=true) |
void | initHeader (uint identity=0) |
void | initStrippedSubjectMD5 () |
bool | isBeingParsed () const |
bool | isComplete () const |
bool | isMessage () const |
bool | isUrgent () const |
KMMessage (const KMMessage &other) | |
KMMessage (KMMsgInfo &msgInfo) | |
KMMessage (DwMessage *) | |
KMMessage (KMFolder *parent=0) | |
DwBodyPart * | lastUpdatedPart () |
void | link (const KMMessage *aMsg, KMMsgStatus aStatus) |
QCString | mboxMessageSeparator () |
KMMsgMDNSentState | mdnSentState () const |
QString | msgId () const |
QString | msgIdMD5 () const |
KMMsgInfo * | msgInfo () |
size_t | msgLength () const |
size_t | msgSize () const |
size_t | msgSizeServer () const |
int | numBodyParts () const |
void | parseTextStringFromDwPart (partNode *root, QCString &parsedString, const QTextCodec *&codec, bool &isHTML) const |
int | partNumber (DwBodyPart *aDwBodyPart) const |
QCString | rawHeaderField (const QCString &name) const |
QValueList< QCString > | rawHeaderFields (const QCString &field) const |
bool | readyToShow () const |
QString | references () const |
void | removeHeaderField (const QCString &name) |
void | removeHeaderFields (const QCString &name) |
void | removePrivateHeaderFields () |
QString | replaceHeadersInString (const QString &s) const |
QString | replyTo () const |
QString | replyToAuxIdMD5 () const |
QString | replyToId () const |
QString | replyToIdMD5 () const |
void | sanitizeHeaders (const QStringList &whiteList=QStringList()) |
QString | sender () const |
void | setAutomaticFields (bool isMultipart=false) |
void | setBcc (const QString &aStr) |
void | setBody (const char *aStr) |
void | setBody (const DwString &aStr) |
void | setBody (const QCString &aStr) |
void | setBodyAndGuessCte (const QCString &aBuf, QValueList< int > &allowedCte, bool allow8Bit=false, bool willBeSigned=false) |
void | setBodyAndGuessCte (const QByteArray &aBuf, QValueList< int > &allowedCte, bool allow8Bit=false, bool willBeSigned=false) |
void | setBodyEncoded (const QCString &aStr) |
void | setBodyEncodedBinary (const QByteArray &aStr) |
void | setBodyFromUnicode (const QString &str) |
void | setCc (const QString &aStr) |
void | setCharset (const QCString &aStr) |
void | setComplete (bool v) |
void | setContentTransferEncoding (int aCte) |
void | setContentTransferEncodingStr (const QCString &aStr) |
void | setContentTypeParam (const QCString &attr, const QCString &val) |
void | setCte (int aCte) |
void | setCteStr (const QCString &aStr) |
void | setCursorPos (int pos) |
void | setDate (time_t aUnixTime) |
void | setDate (const QCString &str) |
void | setDateToday () |
void | setDecodeHTML (bool aDecodeHTML) |
void | setDrafts (const QString &aStr) |
void | setEncryptionState (const KMMsgEncryptionState, int idx=-1) |
void | setFcc (const QString &aStr) |
void | setFileName (const QString &file) |
void | setFolderOffset (off_t offs) |
void | setFrom (const QString &aStr) |
void | setHeaderField (const QCString &name, const QString &value, HeaderFieldType type=Unstructured, bool prepend=false) |
void | setIsBeingParsed (bool t) |
void | setMDNSentState (KMMsgMDNSentState status, int idx=-1) |
void | setMsgId (const QString &aStr) |
void | setMsgInfo (KMMsgInfo *msgInfo) |
void | setMsgLength (size_t sz) |
void | setMsgSerNum (unsigned long newMsgSerNum=0) |
void | setMsgSize (size_t sz) |
void | setMsgSizeServer (size_t sz) |
void | setMultiPartBody (const QCString &aStr) |
void | setNeedsAssembly () |
void | setOverrideCodec (const QTextCodec *codec) |
void | setReadyToShow (bool v) |
void | setReferences (const QCString &aStr) |
void | setReplyTo (KMMessage *) |
void | setReplyTo (const QString &aStr) |
void | setReplyToId (const QString &aStr) |
void | setSignatureState (const KMMsgSignatureState, int idx=-1) |
void | setStatus (const char *s1, const char *s2=0) |
void | setStatus (const KMMsgStatus status, int idx=-1) |
void | setStatusFields () |
void | setSubject (const QString &aStr) |
void | setSubtype (int aSubtype) |
void | setSubtypeStr (const QCString &aStr) |
void | setTemplates (const QString &aStr) |
void | setTo (const QString &aStr) |
void | setTransferInProgress (bool value, bool force=false) |
void | setType (int aType) |
void | setTypeStr (const QCString &aStr) |
void | setUID (ulong uid) |
void | setUnencryptedMsg (KMMessage *unencrypted) |
void | setXMark (const QString &aStr) |
KMMsgSignatureState | signatureState () const |
KMMsgStatus | status () const |
QString | strippedSubjectMD5 () const |
QString | subject () const |
bool | subjectIsPrefixed () const |
QString | subjectMD5 () const |
int | subtype () const |
QCString | subtypeStr () const |
KMMessage * | takeUnencryptedMsg () |
QString | templates () const |
QString | to () const |
const KMMsgBase & | toMsgBase () const |
KMMsgBase & | toMsgBase () |
QString | toStrip () const |
void | touch () |
bool | transferInProgress () const |
int | type () const |
QCString | typeStr () const |
ulong | UID () const |
void | undel () |
KMMessage * | unencryptedMsg () const |
void | updateAttachmentState (DwBodyPart *part=0) |
void | updateBodyPart (const QString partSpecifier, const QByteArray &data) |
QString | who () const |
QString | xmark () const |
virtual | ~KMMessage () |
Static Public Member Functions | |
static bool | addressIsInAddressList (const QString &address, const QStringList &addresses) |
static void | bodyPart (DwBodyPart *aDwBodyPart, KMMessagePart *aPart, bool withBody=true) |
static QString | decodeMailtoUrl (const QString &url) |
static QCString | defaultCharset () |
static QValueList< int > | determineAllowedCtes (const KMime::CharFreq &cf, bool allow8Bit, bool willBeSigned) |
static QString | emailAddrAsAnchor (const QString &emailAddr, bool stripped=true, const QString &cssStyle=QString::null, bool link=true) |
static QString | encodeMailtoUrl (const QString &str) |
static QString | expandAliases (const QString &recipients) |
static QString | generateMessageId (const QString &addr) |
static QString | guessEmailAddressFromLoginName (const QString &userName) |
static QCString | html2source (const QCString &src) |
static KPIM::EmailParseResult | isValidEmailAddressList (const QString &aStr, QString &brokenAddress) |
static const QStringList & | preferredCharsets () |
static QString | quoteHtmlChars (const QString &str, bool removeLineBreaks=false) |
static void | readConfig () |
static void | setDwMediaTypeParam (DwMediaType &mType, const QCString &attr, const QCString &val) |
static QString | smartQuote (const QString &msg, int maxLineLength) |
static KMime::Types::AddressList | splitAddrField (const QCString &str) |
static QStringList | stripAddressFromAddressList (const QString &address, const QStringList &addresses) |
static QString | stripEmailAddr (const QString &emailAddr) |
static QCString | stripEmailAddr (const QCString &emailAddr) |
static QStringList | stripMyAddressesFromAddressList (const QStringList &list) |
Detailed Description
This is a Mime Message.
Definition at line 67 of file kmmessage.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
KMMessage::KMMessage | ( | KMFolder * | parent = 0 |
) |
KMMessage::KMMessage | ( | DwMessage * | aMsg | ) |
Constructor from a DwMessage.
KMMessage takes possession of the DwMessage, so don't dare to delete it.
Definition at line 91 of file kmmessage.cpp.
KMMessage::KMMessage | ( | KMMsgInfo & | msgInfo | ) |
Copy constructor.
Does *not* automatically load the message.
Definition at line 107 of file kmmessage.cpp.
KMMessage::KMMessage | ( | const KMMessage & | other | ) |
KMMessage::~KMMessage | ( | ) | [virtual] |
Member Function Documentation
void KMMessage::addBodyPart | ( | const KMMessagePart * | aPart | ) |
void KMMessage::addDwBodyPart | ( | DwBodyPart * | aDwPart | ) |
bool KMMessage::addressIsInAddressList | ( | const QString & | address, | |
const QStringList & | addresses | |||
) | [static] |
Returns true if the given address is contained in the given address list.
Definition at line 3849 of file kmmessage.cpp.
void KMMessage::applyIdentity | ( | uint | id | ) |
Set the from, to, cc, bcc, encrytion etc headers as specified in the given identity.
Definition at line 1643 of file kmmessage.cpp.
const DwMessage * KMMessage::asDwMessage | ( | ) |
Definition at line 306 of file kmmessage.cpp.
const DwString & KMMessage::asDwString | ( | ) | const |
Return the entire message contents in the DwString.
This function is *fast* even for large message since it does *not* involve a string copy.
Definition at line 295 of file kmmessage.cpp.
QString KMMessage::asPlainText | ( | bool | stripSignature, | |
bool | allowDecryption | |||
) | const |
Return the textual content of the message as plain text, converting HTML to plain text if necessary.
Definition at line 746 of file kmmessage.cpp.
QString KMMessage::asQuotedString | ( | const QString & | headerStr, | |
const QString & | indentStr, | |||
const QString & | selection = QString::null , |
|||
bool | aStripSignature = true , |
|||
bool | allowDecryption = true | |||
) | const |
Returns message body with quoting header and indented by the given indentation string.
This is suitable for including the message in another message of for replies, forwards. The header string is a template where the following fields are replaced with the corresponding values:
D: date of this message S: subject of this message F: sender (from) of this message %%: a single percent signNo attachments are handled if includeAttach is false. The signature is stripped if aStripSignature is true and smart quoting is turned on. Signed or encrypted texts get converted to plain text when allowDecryption is true.
Definition at line 823 of file kmmessage.cpp.
QByteArray KMMessage::asSendableString | ( | ) | const |
Return the message contents with the headers that should not be sent stripped off.
Definition at line 322 of file kmmessage.cpp.
QCString KMMessage::asString | ( | ) | const |
Return the entire message contents as a string.
This function is slow for large message since it involves a string copy. If you need the string representation only for a short time (i.e. without the chance of calling any function in the underlying mimelib, then you should use the asDwString function.
- See also:
- asDwString
Definition at line 317 of file kmmessage.cpp.
QString KMMessage::bcc | ( | ) | const |
QCString KMMessage::body | ( | ) | const |
QCString KMMessage::bodyDecoded | ( | ) | const |
Returns a decoded version of the body from the current content transfer encoding.
The first method returns a null terminated string, the second method is meant for binary data, not null is appended
Definition at line 2569 of file kmmessage.cpp.
QByteArray KMMessage::bodyDecodedBinary | ( | ) | const |
Definition at line 2543 of file kmmessage.cpp.
void KMMessage::bodyPart | ( | int | aIdx, | |
KMMessagePart * | aPart | |||
) | const |
Get the body part at position in aIdx.
Indexing starts at 0. If there is no body part at that index, aPart will have its attributes set to empty values.
Definition at line 3119 of file kmmessage.cpp.
void KMMessage::bodyPart | ( | DwBodyPart * | aDwBodyPart, | |
KMMessagePart * | aPart, | |||
bool | withBody = true | |||
) | [static] |
Fill the KMMessagePart structure for a given DwBodyPart.
If withBody is false the body of the KMMessagePart will be left empty and only the headers of the part will be filled in
Definition at line 3068 of file kmmessage.cpp.
QString KMMessage::bodyToUnicode | ( | const QTextCodec * | codec = 0 |
) | const |
QString KMMessage::cc | ( | ) | const |
QString KMMessage::ccStrip | ( | ) | const |
Definition at line 1942 of file kmmessage.cpp.
QCString KMMessage::charset | ( | ) | const |
void KMMessage::cleanupHeader | ( | ) |
Removes empty fields from the header, e.g.
an empty Cc: or Bcc: field.
Definition at line 1735 of file kmmessage.cpp.
const QTextCodec * KMMessage::codec | ( | ) | const |
int KMMessage::contentTransferEncoding | ( | ) | const |
Definition at line 2490 of file kmmessage.cpp.
QCString KMMessage::contentTransferEncodingStr | ( | ) | const |
Get or set the 'Content-Transfer-Encoding' header field The member functions that involve enumerated types (ints) will work only for well-known encodings.
Definition at line 2480 of file kmmessage.cpp.
KMMessage * KMMessage::createDeliveryReceipt | ( | ) | const |
Create a new message that is a delivery receipt of this message, filling required header fileds with the proper values.
The returned message is not stored in any folder.
Definition at line 1616 of file kmmessage.cpp.
DwBodyPart * KMMessage::createDWBodyPart | ( | const KMMessagePart * | aPart | ) |
Compose a DwBodyPart (needed for adding a part to the message).
Definition at line 3140 of file kmmessage.cpp.
Create a new message that is a forward of this message, filling all required header fields with the proper values.
The returned message is not stored in any folder. Marks this message as forwarded.
Definition at line 1211 of file kmmessage.cpp.
QCString KMMessage::createForwardBody | ( | ) |
KMMessage* KMMessage::createMDN | ( | KMime::MDN::ActionMode | a, | |
KMime::MDN::DispositionType | d, | |||
bool | allowGUI = false , |
|||
QValueList< KMime::MDN::DispositionModifier > | m = QValueList< KMime::MDN::DispositionModifier >() | |||
) |
Create a new message that is a MDN for this message, filling all required fields with proper values.
The returned message is not stored in any folder.
- Parameters:
-
a Use AutomaticAction for filtering and ManualAction for user-induced events. d See docs for KMime::MDN::DispositionType m See docs for KMime::MDN::DispositionModifier allowGUI Set to true if this method is allowed to ask the user questions
- Returns:
- The notification message or 0, if none should be sent.
Create a new message that is a redirect to this message, filling all required header fields with the proper values.
The returned message is not stored in any folder. Marks this message as replied. Redirects differ from forwards so they are forwarded to some other user, mail is not changed and the reply-to field is set to the email address of the original sender
Definition at line 1113 of file kmmessage.cpp.
KMMessage * KMMessage::createReply | ( | KMail::ReplyStrategy | replyStrategy = KMail::ReplySmart , |
|
QString | selection = QString::null , |
|||
bool | noQuote = false , |
|||
bool | allowDecryption = true , |
|||
bool | selectionIsBody = false , |
|||
const QString & | tmpl = QString::null | |||
) |
Create a new message that is a reply to this message, filling all required header fields with the proper values.
The returned message is not stored in any folder. Marks this message as replied.
Definition at line 851 of file kmmessage.cpp.
int KMMessage::cte | ( | ) | const [inline] |
Definition at line 517 of file kmmessage.h.
QCString KMMessage::cteStr | ( | ) | const [inline] |
Cte is short for ContentTransferEncoding.
These functions are an alternative to the ones with longer names.
Definition at line 516 of file kmmessage.h.
time_t KMMessage::date | ( | void | ) | const [virtual] |
QString KMMessage::dateIsoStr | ( | ) | const |
Definition at line 1814 of file kmmessage.cpp.
QCString KMMessage::dateShortStr | ( | ) | const |
Returns the message date in asctime format or an empty string if the message lacks a Date header.
Definition at line 1796 of file kmmessage.cpp.
QString KMMessage::dateStr | ( | void | ) | const [virtual] |
Get or set the 'Date' header field.
Reimplemented from KMMsgBase.
Definition at line 1778 of file kmmessage.cpp.
QCString KMMessage::defaultCharset | ( | ) | [static] |
void KMMessage::del | ( | ) | [inline] |
void KMMessage::deleteBodyParts | ( | ) |
void KMMessage::deleteWhenUnused | ( | ) |
static QValueList<int> KMMessage::determineAllowedCtes | ( | const KMime::CharFreq & | cf, | |
bool | allow8Bit, | |||
bool | willBeSigned | |||
) | [static] |
Returns a list of content-transfer-encodings that can be used with the given result of the character frequency analysis of a message or message part under the given restrictions.
QString KMMessage::drafts | ( | ) | const [inline] |
DwBodyPart * KMMessage::dwBodyPart | ( | int | aIdx | ) | const |
Get the DwBodyPart at position in aIdx.
Indexing starts at 0. If there is no body part at that index, return value will be zero.
Definition at line 2859 of file kmmessage.cpp.
DwMediaType & KMMessage::dwContentType | ( | ) |
Return reference to Content-Type header for direct manipulation.
Definition at line 392 of file kmmessage.cpp.
QString KMMessage::emailAddrAsAnchor | ( | const QString & | emailAddr, | |
bool | stripped = true , |
|||
const QString & | cssStyle = QString::null , |
|||
bool | link = true | |||
) | [static] |
Converts the email address(es) to (a) nice HTML mailto: anchor(s).
If stripped is TRUE then the visible part of the anchor contains only the name part and not the given emailAddr.
Definition at line 3768 of file kmmessage.cpp.
KMMsgEncryptionState KMMessage::encryptionState | ( | ) | const [inline, virtual] |
Expands aliases (distribution lists and nick names) and appends a domain part to all email addresses which are missing the domain part.
Definition at line 3865 of file kmmessage.cpp.
AddrSpecList KMMessage::extractAddrSpecs | ( | const QCString & | headerNames | ) | const |
Definition at line 2236 of file kmmessage.cpp.
QString KMMessage::fcc | ( | ) | const |
QString KMMessage::fileName | ( | void | ) | const [inline, virtual] |
DwBodyPart * KMMessage::findDwBodyPart | ( | DwBodyPart * | part, | |
const QString & | partSpecifier | |||
) |
Return the first DwBodyPart matching a given partSpecifier or zero, if no found.
Definition at line 4140 of file kmmessage.cpp.
Return the first DwBodyPart matching a given Content-Type or zero, if no found.
Definition at line 2948 of file kmmessage.cpp.
DwBodyPart * KMMessage::findDwBodyPart | ( | int | type, | |
int | subtype | |||
) | const |
Return the first DwBodyPart matching a given Content-Type or zero, if no found.
Definition at line 2899 of file kmmessage.cpp.
off_t KMMessage::folderOffset | ( | void | ) | const [inline, virtual] |
QString KMMessage::from | ( | ) | const |
void KMMessage::fromByteArray | ( | const QByteArray & | ba, | |
bool | setStatus = false | |||
) |
Definition at line 397 of file kmmessage.cpp.
void KMMessage::fromDwString | ( | const DwString & | str, | |
bool | setStatus = false | |||
) |
void KMMessage::fromString | ( | const QCString & | str, | |
bool | setStatus = false | |||
) |
Definition at line 401 of file kmmessage.cpp.
QString KMMessage::fromStrip | ( | void | ) | const [virtual] |
Generates the Message-Id.
It uses either the Message-Id suffix defined by the user or the given email address as suffix. The address must be given as addr-spec as defined in RFC 2822.
Definition at line 3292 of file kmmessage.cpp.
int KMMessage::getCursorPos | ( | ) | [inline] |
DwBodyPart * KMMessage::getFirstDwBodyPart | ( | ) | const |
Get the 1st DwBodyPart.
If there is no body part, return value will be zero.
Definition at line 2812 of file kmmessage.cpp.
void KMMessage::getLink | ( | int | n, | |
ulong * | retMsgSerNum, | |||
KMMsgStatus * | retStatus | |||
) | const |
Returns the information for the Nth link into retMsg
and retStatus
.
Definition at line 4118 of file kmmessage.cpp.
QCString KMMessage::getRefStr | ( | ) | const |
Creates reference string for reply to messages.
reference = original first reference + original last reference + original msg-id
Definition at line 1085 of file kmmessage.cpp.
DwMessage* KMMessage::getTopLevelPart | ( | ) | const [inline] |
Definition at line 605 of file kmmessage.h.
Uses the hostname as domain part and tries to determine the real name from the entries in the password file.
Definition at line 3915 of file kmmessage.cpp.
bool KMMessage::hasUnencryptedMsg | ( | ) | const [inline] |
Returns TRUE if the message contains an unencrypted copy of itself.
Definition at line 134 of file kmmessage.h.
AddressList KMMessage::headerAddrField | ( | const QCString & | name | ) | const |
Returns header address list as string list.
Valid for the following fields: To, Bcc, Cc, ReplyTo, ResentBcc, ResentCc, ResentReplyTo, ResentTo
Definition at line 2232 of file kmmessage.cpp.
QCString KMMessage::headerAsSendableString | ( | ) | const |
Return the message header with the headers that should not be sent stripped off.
Definition at line 330 of file kmmessage.cpp.
QString KMMessage::headerAsString | ( | ) | const |
Returns the value of a header field with the given name.
If multiple header fields with the given name might exist then you should use headerFields() instead.
Definition at line 2270 of file kmmessage.cpp.
QStringList KMMessage::headerFields | ( | const QCString & | name | ) | const |
Returns a list of the values of all header fields with the given name.
Definition at line 2283 of file kmmessage.cpp.
DwHeaders & KMMessage::headers | ( | ) | const |
get the DwHeaders (make sure to call setNeedsAssembly() function after directly modyfying internal data like the headers)
Definition at line 2517 of file kmmessage.cpp.
Convert '<' into "<" resp.
'>' into ">" in order to prevent their interpretation by KHTML. Does *not* use the Qt replace function but runs a very fast C code the same way as lf2crlf() does.
Definition at line 3317 of file kmmessage.cpp.
QCString KMMessage::id | ( | ) | const |
uint KMMessage::identityUoid | ( | ) | const |
- Returns:
- the UOID of the identity for this message. Searches the "x-kmail-identity" header and if that fails, searches with KPIM::IdentityManager::identityForAddress() and if that fails queries the KMMsgBase::parent() folder for a default.
Definition at line 1708 of file kmmessage.cpp.
void KMMessage::initFromMessage | ( | const KMMessage * | msg, | |
bool | idHeaders = true | |||
) |
Initialize headers fields according to the identity and the transport header of the given original message.
Definition at line 1723 of file kmmessage.cpp.
void KMMessage::initHeader | ( | uint | identity = 0 |
) |
Initialize header fields.
Should be called on new messages if they are not set manually. E.g. before composing. Calling of setAutomaticFields(), see below, is still required.
Definition at line 1696 of file kmmessage.cpp.
void KMMessage::initStrippedSubjectMD5 | ( | ) | [inline, virtual] |
bool KMMessage::isBeingParsed | ( | ) | const [inline] |
Definition at line 876 of file kmmessage.h.
bool KMMessage::isComplete | ( | ) | const [inline] |
Return true if the complete message is available without referring to the backing store.
Definition at line 816 of file kmmessage.h.
bool KMMessage::isMessage | ( | void | ) | const [virtual] |
Returns TRUE if object is a real message (not KMMsgInfo or KMMsgBase).
Reimplemented from KMMsgBase.
Definition at line 233 of file kmmessage.cpp.
bool KMMessage::isUrgent | ( | ) | const |
- Returns:
- whether the priority: or x-priority headers indicate that this message should be considered urgent
Definition at line 262 of file kmmessage.cpp.
KPIM::EmailParseResult KMMessage::isValidEmailAddressList | ( | const QString & | aStr, | |
QString & | brokenAddress | |||
) | [static] |
Validate a list of email addresses, and also allow aliases and distribution lists to be expanded before validation.
- Returns:
- Enum to describe the error.
brokenAddress the address that was faulty. FIXME: this should be in libemailfucntions but that requires moving expandAliases and all that it brings
Definition at line 276 of file kmmessage.cpp.
DwBodyPart* KMMessage::lastUpdatedPart | ( | ) | [inline] |
void KMMessage::link | ( | const KMMessage * | aMsg, | |
KMMsgStatus | aStatus | |||
) |
Links this message to aMsg
, setting link type to aStatus
.
Definition at line 4092 of file kmmessage.cpp.
QCString KMMessage::mboxMessageSeparator | ( | ) |
Returns an mbox message separator line for this message, i.e.
a string of the form "From local@domain.invalid Sat Jun 12 14:00:00 2004\n".
Definition at line 4354 of file kmmessage.cpp.
KMMsgMDNSentState KMMessage::mdnSentState | ( | ) | const [inline, virtual] |
QString KMMessage::msgId | ( | ) | const |
QString KMMessage::msgIdMD5 | ( | void | ) | const [virtual] |
KMMsgInfo* KMMessage::msgInfo | ( | ) | [inline] |
Get the KMMsgInfo object that was set with setMsgInfo().
Definition at line 869 of file kmmessage.h.
size_t KMMessage::msgLength | ( | ) | const [inline] |
Unlike the above function this works also, if the message is not in a folder.
Definition at line 766 of file kmmessage.h.
size_t KMMessage::msgSize | ( | void | ) | const [inline, virtual] |
Get/set size of message in the folder including the whole header in bytes.
Can be 0, if the message is not in a folder. The setting of mMsgSize = mMsgLength = sz is needed for popFilter
Implements KMMsgBase.
Definition at line 761 of file kmmessage.h.
size_t KMMessage::msgSizeServer | ( | void | ) | const [virtual] |
int KMMessage::numBodyParts | ( | ) | const |
Number of body parts the message has.
This is one for plain messages without any attachment.
Definition at line 2770 of file kmmessage.cpp.
void KMMessage::parseTextStringFromDwPart | ( | partNode * | root, | |
QCString & | parsedString, | |||
const QTextCodec *& | codec, | |||
bool & | isHTML | |||
) | const |
Returns a decoded body part string to be further processed by function asQuotedString().
THIS FUNCTION WILL BE REPLACED ONCE KMime IS FULLY INTEGRATED (khz, June 05 2002)
Definition at line 715 of file kmmessage.cpp.
int KMMessage::partNumber | ( | DwBodyPart * | aDwBodyPart | ) | const |
Get the number of the given DwBodyPart.
If no body part is given, return value will be -1.
Definition at line 2819 of file kmmessage.cpp.
const QStringList & KMMessage::preferredCharsets | ( | ) | [static] |
Quotes the following characters which have a special meaning in HTML: '<' '>' '&' '"'. Additionally '\n' is converted to "<br />" if removeLineBreaks
is false.
If removeLineBreaks
is true, then '\n' is removed. Last but not least '\r' is removed.
Definition at line 3732 of file kmmessage.cpp.
Returns the raw value of a header field with the given name.
If multiple header fields with the given name might exist then you should use rawHeaderFields() instead.
Definition at line 2245 of file kmmessage.cpp.
QValueList< QCString > KMMessage::rawHeaderFields | ( | const QCString & | field | ) | const |
Returns a list of the raw values of all header fields with the given name.
Definition at line 2256 of file kmmessage.cpp.
void KMMessage::readConfig | ( | void | ) | [static] |
Reads config settings from group "KMMessage" and sets all internal variables (e.g.
indent-prefix, etc.)
Reimplemented from KMMsgBase.
Definition at line 3945 of file kmmessage.cpp.
bool KMMessage::readyToShow | ( | ) | const [inline] |
QString KMMessage::references | ( | ) | const |
void KMMessage::removeHeaderField | ( | const QCString & | name | ) |
void KMMessage::removeHeaderFields | ( | const QCString & | name | ) |
void KMMessage::removePrivateHeaderFields | ( | ) |
Remove all private header fields: *Status: and X-KMail-*.
Definition at line 338 of file kmmessage.cpp.
Replaces every occurrence of "${foo}" in s
with headerField("foo").
Definition at line 1591 of file kmmessage.cpp.
QString KMMessage::replyTo | ( | ) | const |
QString KMMessage::replyToAuxIdMD5 | ( | ) | const [virtual] |
Get the second to last id from the References header field.
If outgoing messages are not kept in the same folder as incoming ones, this will be a good place to thread the message beneath. bob <- second to last reference points to this |_kmailuser <- not in our folder, but Outbox |_bob <- In-Reply-To points to our mail above
Thread like this: bob |_bob
using replyToAuxIdMD5
Implements KMMsgBase.
Definition at line 2127 of file kmmessage.cpp.
QString KMMessage::replyToId | ( | ) | const |
QString KMMessage::replyToIdMD5 | ( | void | ) | const [virtual] |
void KMMessage::sanitizeHeaders | ( | const QStringList & | whiteList = QStringList() |
) |
Remove all headers but the content description ones, and those in the white list.
Definition at line 1192 of file kmmessage.cpp.
QString KMMessage::sender | ( | ) | const |
- Returns:
- The addr-spec of either the Sender: (if one is given) or the first addr-spec in From:
Definition at line 2020 of file kmmessage.cpp.
void KMMessage::setAutomaticFields | ( | bool | isMultipart = false |
) |
Set fields that are either automatically set (Message-id) or that do not change from one message to another (MIME-Version).
Call this method before sending *after* all changes to the message are done because this method does things different if there are attachments / multiple body parts.
Definition at line 1758 of file kmmessage.cpp.
void KMMessage::setBcc | ( | const QString & | aStr | ) |
Definition at line 1956 of file kmmessage.cpp.
void KMMessage::setBody | ( | const char * | aStr | ) |
Definition at line 2749 of file kmmessage.cpp.
void KMMessage::setBody | ( | const DwString & | aStr | ) |
Definition at line 2744 of file kmmessage.cpp.
void KMMessage::setBody | ( | const QCString & | aStr | ) |
void KMMessage::setBodyAndGuessCte | ( | const QCString & | aBuf, | |
QValueList< int > & | allowedCte, | |||
bool | allow8Bit = false , |
|||
bool | willBeSigned = false | |||
) |
Definition at line 2669 of file kmmessage.cpp.
void KMMessage::setBodyAndGuessCte | ( | const QByteArray & | aBuf, | |
QValueList< int > & | allowedCte, | |||
bool | allow8Bit = false , |
|||
bool | willBeSigned = false | |||
) |
Sets body, encoded in the best fitting content-transfer-encoding, which is determined by character frequency count.
- Parameters:
-
aBuf input buffer allowedCte return: list of allowed cte's allow8Bit whether "8bit" is allowed as cte. willBeSigned whether "7bit"/"8bit" is allowed as cte according to RFC 3156
Definition at line 2646 of file kmmessage.cpp.
void KMMessage::setBodyEncoded | ( | const QCString & | aStr | ) |
Set the message body, encoding it according to the current content transfer encoding.
The first method for null terminated strings, the second for binary data
Definition at line 2692 of file kmmessage.cpp.
void KMMessage::setBodyEncodedBinary | ( | const QByteArray & | aStr | ) |
Definition at line 2715 of file kmmessage.cpp.
void KMMessage::setBodyFromUnicode | ( | const QString & | str | ) |
Sets this body part's content to str
.
str
is subject to automatic charset and CTE detection.
Definition at line 4315 of file kmmessage.cpp.
void KMMessage::setCc | ( | const QString & | aStr | ) |
Definition at line 1935 of file kmmessage.cpp.
void KMMessage::setCharset | ( | const QCString & | aStr | ) |
void KMMessage::setComplete | ( | bool | v | ) | [inline] |
void KMMessage::setContentTransferEncoding | ( | int | aCte | ) |
Definition at line 2509 of file kmmessage.cpp.
void KMMessage::setContentTransferEncodingStr | ( | const QCString & | aStr | ) |
Definition at line 2500 of file kmmessage.cpp.
void KMMessage::setCte | ( | int | aCte | ) | [inline] |
Definition at line 519 of file kmmessage.h.
void KMMessage::setCteStr | ( | const QCString & | aStr | ) | [inline] |
Definition at line 518 of file kmmessage.h.
void KMMessage::setCursorPos | ( | int | pos | ) | [inline] |
void KMMessage::setDate | ( | time_t | aUnixTime | ) | [virtual] |
void KMMessage::setDate | ( | const QCString & | aStrDate | ) | [virtual] |
void KMMessage::setDateToday | ( | ) |
void KMMessage::setDecodeHTML | ( | bool | aDecodeHTML | ) | [inline] |
void KMMessage::setDrafts | ( | const QString & | aStr | ) |
Definition at line 1975 of file kmmessage.cpp.
void KMMessage::setEncryptionState | ( | const KMMsgEncryptionState | s, | |
int | idx = -1 | |||
) | [virtual] |
Set encryption status of the message.
Reimplemented from KMMsgBase.
Definition at line 4063 of file kmmessage.cpp.
void KMMessage::setFcc | ( | const QString & | aStr | ) |
Definition at line 1969 of file kmmessage.cpp.
void KMMessage::setFileName | ( | const QString & | file | ) | [inline, virtual] |
void KMMessage::setFolderOffset | ( | off_t | offs | ) | [inline, virtual] |
void KMMessage::setFrom | ( | const QString & | aStr | ) |
Definition at line 2003 of file kmmessage.cpp.
void KMMessage::setHeaderField | ( | const QCString & | name, | |
const QString & | value, | |||
HeaderFieldType | type = Unstructured , |
|||
bool | prepend = false | |||
) |
Set the header field with the given name to the given value.
If prepend is set to true, the header is inserted at the beginning and does not overwrite an existing header field with the same name.
Definition at line 2320 of file kmmessage.cpp.
void KMMessage::setIsBeingParsed | ( | bool | t | ) | [inline] |
Definition at line 877 of file kmmessage.h.
void KMMessage::setMDNSentState | ( | KMMsgMDNSentState | status, | |
int | idx = -1 | |||
) | [virtual] |
Set "MDN sent" status of the message.
Reimplemented from KMMsgBase.
Definition at line 4081 of file kmmessage.cpp.
void KMMessage::setMsgId | ( | const QString & | aStr | ) |
Definition at line 2186 of file kmmessage.cpp.
void KMMessage::setMsgInfo | ( | KMMsgInfo * | msgInfo | ) | [inline] |
void KMMessage::setMsgLength | ( | size_t | sz | ) | [inline] |
Definition at line 768 of file kmmessage.h.
void KMMessage::setMsgSerNum | ( | unsigned long | newMsgSerNum = 0 |
) |
Sets the message serial number.
If defaulted to zero, the serial number will be assigned using the dictionary. Note that unless it is explicitely set the serial number will remain 0 as long as the mail is not in a folder.
Definition at line 226 of file kmmessage.cpp.
void KMMessage::setMsgSize | ( | size_t | sz | ) | [inline, virtual] |
void KMMessage::setMsgSizeServer | ( | size_t | sz | ) | [virtual] |
void KMMessage::setMultiPartBody | ( | const QCString & | aStr | ) |
Hack to enable structured body parts to be set as flat text.
..
Definition at line 2755 of file kmmessage.cpp.
void KMMessage::setNeedsAssembly | ( | ) |
tell the message that internal data were changed (must be called after directly modifying message structures e.g.
when like changing header information by accessing the header via headers() function)
Definition at line 2524 of file kmmessage.cpp.
void KMMessage::setOverrideCodec | ( | const QTextCodec * | codec | ) | [inline] |
Set the charset the user selected for the message to display.
Definition at line 735 of file kmmessage.h.
void KMMessage::setReadyToShow | ( | bool | v | ) | [inline] |
void KMMessage::setReferences | ( | const QCString & | aStr | ) |
Definition at line 202 of file kmmessage.cpp.
void KMMessage::setReplyTo | ( | KMMessage * | aMsg | ) |
Definition at line 1914 of file kmmessage.cpp.
void KMMessage::setReplyTo | ( | const QString & | aStr | ) |
Definition at line 1907 of file kmmessage.cpp.
void KMMessage::setReplyToId | ( | const QString & | aStr | ) |
Definition at line 2155 of file kmmessage.cpp.
void KMMessage::setSignatureState | ( | const | KMMsgSignatureState, | |
int | idx = -1 | |||
) | [virtual] |
Set signature status of the message.
Reimplemented from KMMsgBase.
Definition at line 4072 of file kmmessage.cpp.
void KMMessage::setStatus | ( | const char * | s1, | |
const char * | s2 = 0 | |||
) | [inline, virtual] |
void KMMessage::setStatus | ( | const KMMsgStatus | status, | |
int | idx = -1 | |||
) | [virtual] |
Set status and mark dirty.
Reimplemented from KMMsgBase.
Definition at line 4056 of file kmmessage.cpp.
void KMMessage::setStatusFields | ( | ) |
Set "Status" and "X-Status" fields of the message from the internal message status.
Definition at line 354 of file kmmessage.cpp.
void KMMessage::setSubject | ( | const QString & | ) | [virtual] |
Set subject/from/date and xmark.
Implements KMMsgBase.
Definition at line 2037 of file kmmessage.cpp.
void KMMessage::setSubtype | ( | int | aSubtype | ) |
Definition at line 2436 of file kmmessage.cpp.
void KMMessage::setSubtypeStr | ( | const QCString & | aStr | ) |
Definition at line 2427 of file kmmessage.cpp.
void KMMessage::setTemplates | ( | const QString & | aStr | ) |
Definition at line 1981 of file kmmessage.cpp.
void KMMessage::setTo | ( | const QString & | aStr | ) |
Definition at line 1888 of file kmmessage.cpp.
void KMMessage::setTransferInProgress | ( | bool | value, | |
bool | force = false | |||
) |
Set that the message shall not be deleted because it is still required.
Definition at line 246 of file kmmessage.cpp.
void KMMessage::setType | ( | int | aType | ) |
Definition at line 2399 of file kmmessage.cpp.
void KMMessage::setTypeStr | ( | const QCString & | aStr | ) |
Definition at line 2390 of file kmmessage.cpp.
void KMMessage::setUID | ( | ulong | uid | ) | [virtual] |
void KMMessage::setUnencryptedMsg | ( | KMMessage * | unencrypted | ) |
Specifies an unencrypted copy of this message to be stored in a separate member variable to allow saving messages in unencrypted form that were sent in encrypted form.
NOTE: Ownership of unencrypted
transfers to this KMMessage, and it will be deleted in the d'tor.
Definition at line 268 of file kmmessage.cpp.
void KMMessage::setXMark | ( | const QString & | aStr | ) | [virtual] |
KMMsgSignatureState KMMessage::signatureState | ( | ) | const [inline, virtual] |
Given argument msg add quoting characters and relayout for max width maxLength.
- Parameters:
-
msg the string which it to be quoted maxLineLength reformat text to be this amount of columns at maximum, adding linefeeds at word boundaries to make it fit.
Definition at line 647 of file kmmessage.cpp.
AddressList KMMessage::splitAddrField | ( | const QCString & | str | ) | [static] |
Splits the given address list into separate addresses.
Definition at line 2219 of file kmmessage.cpp.
KMMsgStatus KMMessage::status | ( | void | ) | const [inline, virtual] |
QStringList KMMessage::stripAddressFromAddressList | ( | const QString & | address, | |
const QStringList & | addresses | |||
) | [static] |
Strips an address from an address list.
This is for example used when replying to all.
Definition at line 3806 of file kmmessage.cpp.
Does the same as the above function.
Shouldn't be used.
Definition at line 3570 of file kmmessage.cpp.
This function generates a displayable string from a list of email addresses.
Input : mailbox-list Output: comma separated list of display name resp. comment resp. address
Definition at line 3411 of file kmmessage.cpp.
QStringList KMMessage::stripMyAddressesFromAddressList | ( | const QStringList & | list | ) | [static] |
Strips all the user's addresses from an address list.
This is used when replying.
Definition at line 3828 of file kmmessage.cpp.
QString KMMessage::strippedSubjectMD5 | ( | ) | const [virtual] |
Get a hash of the subject with all prefixes such as Re: removed.
Used for threading.
Implements KMMsgBase.
Definition at line 2140 of file kmmessage.cpp.
QString KMMessage::subject | ( | void | ) | const [virtual] |
Get or set the 'Subject' header field.
Implements KMMsgBase.
Definition at line 2030 of file kmmessage.cpp.
bool KMMessage::subjectIsPrefixed | ( | ) | const [virtual] |
Is the subject prefixed by Re: or similar?
Implements KMMsgBase.
Definition at line 2150 of file kmmessage.cpp.
QString KMMessage::subjectMD5 | ( | ) | const |
int KMMessage::subtype | ( | ) | const |
Definition at line 2418 of file kmmessage.cpp.
QCString KMMessage::subtypeStr | ( | ) | const |
KMMessage* KMMessage::takeUnencryptedMsg | ( | ) | [inline] |
Returns an unencrypted copy of this message or 0 if none exists.
- Note:
- This function removes the internal unencrypted message pointer from the message: the process calling takeUnencryptedMsg() must delete the returned pointer when no longer needed.
Definition at line 144 of file kmmessage.h.
QString KMMessage::templates | ( | ) | const [inline] |
QString KMMessage::to | ( | ) | const |
const KMMsgBase& KMMessage::toMsgBase | ( | ) | const [inline] |
Definition at line 115 of file kmmessage.h.
KMMsgBase& KMMessage::toMsgBase | ( | ) | [inline] |
QString KMMessage::toStrip | ( | void | ) | const [virtual] |
void KMMessage::touch | ( | ) | [inline] |
bool KMMessage::transferInProgress | ( | ) | const |
int KMMessage::type | ( | void | ) | const |
Definition at line 2381 of file kmmessage.cpp.
QCString KMMessage::typeStr | ( | ) | const |
Get or set the 'Content-Type' header field The member functions that involve enumerated types (ints) will work only for well-known types or subtypes.
Definition at line 2372 of file kmmessage.cpp.
ulong KMMessage::UID | ( | void | ) | const [virtual] |
void KMMessage::undel | ( | ) | [inline] |
KMMessage* KMMessage::unencryptedMsg | ( | ) | const [inline] |
Returns an unencrypted copy of this message or 0 if none exists.
Definition at line 137 of file kmmessage.h.
void KMMessage::updateAttachmentState | ( | DwBodyPart * | part = 0 |
) |
Definition at line 4251 of file kmmessage.cpp.
void KMMessage::updateBodyPart | ( | const QString | partSpecifier, | |
const QByteArray & | data | |||
) |
QString KMMessage::who | ( | ) | const |
Get or set the 'Who' header field.
The actual field that is returned depends on the contents of the owning folders whoField(). Usually this is 'From', but it can also contain 'To'.
Definition at line 1987 of file kmmessage.cpp.
QString KMMessage::xmark | ( | void | ) | const [virtual] |
Get or set the 'X-Mark' header field.
Implements KMMsgBase.
Definition at line 2045 of file kmmessage.cpp.
The documentation for this class was generated from the following files: