kmail
KMFolder Class Reference
Mail folder. More...
#include <kmfolder.h>
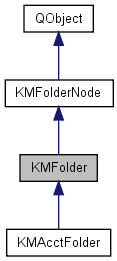
Public Types | |
enum | CompactOptions { CompactLater, CompactNow, CompactSilentlyNow } |
enum | ExpireAction { ExpireDelete, ExpireMove } |
Public Slots | |
void | reallyAddCopyOfMsg (KMMessage *aMsg) |
void | reallyAddMsg (KMMessage *aMsg) |
int | updateIndex () |
Signals | |
void | changed () |
void | cleared () |
void | closed () |
void | expunged (KMFolder *) |
void | folderSizeChanged (KMFolder *) |
void | iconsChanged () |
void | msgAdded (KMFolder *, Q_UINT32 sernum) |
void | msgAdded (int idx) |
void | msgChanged (KMFolder *, Q_UINT32 sernum, int delta) |
void | msgHeaderChanged (KMFolder *, int) |
void | msgRemoved (KMFolder *) |
void | msgRemoved (int idx, QString msgIdMD5) |
void | msgRemoved (KMFolder *, Q_UINT32 sernum) |
void | nameChanged () |
void | numUnreadMsgsChanged (KMFolder *) |
void | removed (KMFolder *, bool) |
void | shortcutChanged (KMFolder *) |
void | statusMsg (const QString &) |
void | viewConfigChanged () |
Public Member Functions | |
AccountList * | acctList () |
int | addMsg (QPtrList< KMMessage > &, QValueList< int > &index_return) |
int | addMsg (KMMessage *msg, int *index_return=0) |
int | addMsgKeepUID (KMMessage *msg, int *index_return=0) |
int | canAccess () |
KMFolderDir * | child () const |
void | close (const char *owner, bool force=false) |
void | compact (CompactOptions options) |
void | correctUnreadMsgsCount () |
int | count (bool cache=false) const |
int | countUnread () |
int | countUnreadRecursive () |
KMFolderDir * | createChildFolder () |
FolderJob * | createJob (QPtrList< KMMessage > &msgList, const QString &sets, FolderJob::JobType jt=FolderJob::tGetMessage, KMFolder *folder=0) const |
FolderJob * | createJob (KMMessage *msg, FolderJob::JobType jt=FolderJob::tGetMessage, KMFolder *folder=0, QString partSpecifier=QString::null, const AttachmentStrategy *as=0) const |
void | daysToExpire (int &unreadDays, int &readDays) |
bool | dirty () const |
void | emitMsgAddedSignals (int idx) |
ExpireAction | expireAction () const |
void | expireOldMessages (bool immediate) |
QString | expireToFolderId () const |
int | expunge () |
int | expungeOldMsg (int days) |
QString | fileName () const |
int | find (const KMMessage *msg) const |
int | find (const KMMsgBase *msg) const |
KMFolderType | folderType () const |
DwString | getDwString (int idx) |
KMMessage * | getMsg (int idx) |
KMMsgBase * | getMsgBase (int idx) |
const KMMsgBase * | getMsgBase (int idx) const |
int | getReadExpireAge () const |
ExpireUnits | getReadExpireUnits () const |
int | getUnreadExpireAge () const |
ExpireUnits | getUnreadExpireUnits () const |
bool | hasAccounts () const |
uint | identity () const |
QString | idString () const |
void | ignoreJobsForMessage (KMMessage *) |
bool | ignoreNewMail () const |
QString | indexLocation () const |
bool | isAutoExpire () const |
bool | isDrafts () |
bool | isMailingListEnabled () const |
bool | isMainInbox () |
bool | isMessage (int idx) |
bool | isMoveable () const |
bool | isOpened () const |
bool | isOutbox () |
bool | isReadOnly () const |
bool | isSent () |
bool | isSystemFolder () const |
bool | isTemplates () |
bool | isTrash () |
KMFolder (KMFolderDir *parent, const QString &name, KMFolderType aFolderType, bool withIndex=true, bool exportedSernums=true) | |
virtual QString | label () const |
QString | location () const |
MailingList | mailingList () const |
QString | mailingListPostAddress () const |
void | markNewAsUnread () |
void | markUnreadAsRead () |
bool | moveInProgress () const |
int | moveMsg (QPtrList< KMMessage >, int *index_return=0) |
int | moveMsg (KMMessage *msg, int *index_return=0) |
void | msgStatusChanged (const KMMsgStatus oldStatus, const KMMsgStatus newStatus, int idx) |
bool | needsCompacting () const |
bool | noChildren () const |
bool | noContent () const |
QString | normalIconPath () const |
int | open (const char *owner) |
KMMsgBase * | operator[] (int idx) |
const KMMsgBase * | operator[] (int idx) const |
virtual QString | prettyURL () const |
bool | putRepliesInSameFolder () const |
void | quiet (bool beQuiet) |
void | readConfig (KConfig *config) |
void | remove () |
void | removeJobs () |
void | removeMsg (QPtrList< KMMessage > msgList, bool imapQuiet=false) |
void | removeMsg (int i, bool imapQuiet=false) |
int | rename (const QString &newName, KMFolderDir *aParent=0) |
void | setAcctList (AccountList *list) |
void | setAutoExpire (bool enabled) |
void | setChild (KMFolderDir *aChild) |
void | setDirty (bool f) |
void | setExpireAction (ExpireAction a) |
void | setExpireToFolderId (const QString &id) |
void | setIconPaths (const QString &normalPath, const QString &unreadPath) |
void | setIdentity (uint identity) |
void | setIgnoreNewMail (bool b) |
void | setLabel (const QString &l) |
void | setMailingList (const MailingList &mlist) |
void | setMailingListEnabled (bool enabled) |
void | setMoveInProgress (bool b) |
void | setNeedsCompacting (bool f) |
void | setNoChildren (bool aNoChildren) |
void | setNoContent (bool aNoContent) |
void | setPutRepliesInSameFolder (bool b) |
void | setReadExpireAge (int age) |
void | setReadExpireUnits (ExpireUnits units) |
void | setShortcut (const KShortcut &) |
void | setStatus (QValueList< int > &ids, KMMsgStatus status, bool toggle=false) |
void | setStatus (int idx, KMMsgStatus status, bool toggle=false) |
void | setSystemFolder (bool itIs) |
void | setSystemLabel (const QString &l) |
void | setUnreadExpireAge (int age) |
void | setUnreadExpireUnits (ExpireUnits units) |
void | setUseCustomIcons (bool useCustomIcons) |
void | setUserWhoField (const QString &whoField, bool writeConfig=true) |
void | setWhoField (const QString &aWhoField) |
const KShortcut & | shortcut () const |
const FolderStorage * | storage () const |
FolderStorage * | storage () |
QString | subdirLocation () const |
void | sync () |
virtual QString | systemLabel () const |
void | take (QPtrList< KMMessage > msgList) |
KMMessage * | take (int idx) |
KMFolder * | trashFolder () const |
KMMsgInfo * | unGetMsg (int idx) |
QString | unreadIconPath () const |
bool | useCustomIcons () const |
QString | userWhoField (void) |
QString | whoField () const |
void | writeConfig (KConfig *config) const |
int | writeIndex (bool createEmptyIndex=false) |
~KMFolder () |
Detailed Description
Mail folder.(description will be here).
Accounts
The accounts (of KMail) that are fed into the folder are represented as the children of the folder. They are only stored here during runtime to have a reference for which accounts point to a specific folder.Definition at line 68 of file kmfolder.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
KMFolder::KMFolder | ( | KMFolderDir * | parent, | |
const QString & | name, | |||
KMFolderType | aFolderType, | |||
bool | withIndex = true , |
|||
bool | exportedSernums = true | |||
) |
Constructs a new Folder object.
- Parameters:
-
parent The directory in the folder storage hierarchy under which the folder's storage will be found or created. name If name of the folder. In case there is no parent directory, because the folder is free-standing (/var/spool/mail/foo), this is used for the full path to the folder's storage location. aFolderType The type of folder to create. withIndex Wether this folder has an index. No-index folders are those used by KMail internally, the Outbox, and those of local spool accounts, for example. exportedSernums whether this folder exports its serial numbers to the global MsgDict for lookup.
- Returns:
- A new folder instance.
Definition at line 48 of file kmfolder.cpp.
KMFolder::~KMFolder | ( | ) |
Definition at line 149 of file kmfolder.cpp.
Member Function Documentation
AccountList* KMFolder::acctList | ( | ) | [inline] |
Definition at line 124 of file kmfolder.h.
int KMFolder::addMsg | ( | QPtrList< KMMessage > & | list, | |
QValueList< int > & | index_return | |||
) |
Adds the given messages to the folder.
Behaviour is identical to addMsg(msg)
Definition at line 396 of file kmfolder.cpp.
int KMFolder::addMsg | ( | KMMessage * | msg, | |
int * | index_return = 0 | |||
) |
Add the given message to the folder.
Usually the message is added at the end of the folder. Returns zero on success and an errno error code on failure. The index of the new message is stored in index_return if given. Please note that the message is added as is to the folder and the folder takes ownership of the message (deleting it in the destructor).
Definition at line 386 of file kmfolder.cpp.
int KMFolder::addMsgKeepUID | ( | KMMessage * | msg, | |
int * | index_return = 0 | |||
) |
(Note(bo): This needs to be fixed better at a later point.
) This is overridden by dIMAP because addMsg strips the X-UID header from the mail.
Definition at line 391 of file kmfolder.cpp.
int KMFolder::canAccess | ( | ) |
Check folder for permissions Returns zero if readable and writable.
Definition at line 480 of file kmfolder.cpp.
void KMFolder::changed | ( | ) | [signal] |
Emitted when the status, name, or associated accounts of this folder changed.
KMFolderDir* KMFolder::child | ( | ) | const [inline] |
Returns the folder directory associated with this node or 0 if no such directory exists.
Definition at line 156 of file kmfolder.h.
void KMFolder::cleared | ( | ) | [signal] |
Emitted when the contents of a folder have been cleared (new search in a search folder, for example).
void KMFolder::close | ( | const char * | owner, | |
bool | force = false | |||
) |
Close folder.
If force is true the files are closed even if others still use it (e.g. other mail reader windows).
Definition at line 485 of file kmfolder.cpp.
void KMFolder::closed | ( | ) | [signal] |
Emitted when the folder is closed for real - ticket holders should discard any messages.
void KMFolder::compact | ( | CompactOptions | options | ) |
Compact this folder.
Options: CompactLater: schedule it as a background task CompactNow: do it now, and inform the user of the result (manual compaction) CompactSilentlyNow: do it now, and keep silent about it (e.g. for outbox)
Definition at line 792 of file kmfolder.cpp.
void KMFolder::correctUnreadMsgsCount | ( | ) |
A cludge to help make sure the count of unread messges is kept in sync.
Definition at line 681 of file kmfolder.cpp.
int KMFolder::count | ( | bool | cache = false |
) | const |
int KMFolder::countUnread | ( | ) |
int KMFolder::countUnreadRecursive | ( | ) |
Number of new or unread messages in this folder and all folders contained by this folder.
Definition at line 451 of file kmfolder.cpp.
KMFolderDir * KMFolder::createChildFolder | ( | ) |
Create a child folder directory and associates it with this folder.
Definition at line 260 of file kmfolder.cpp.
FolderJob * KMFolder::createJob | ( | QPtrList< KMMessage > & | msgList, | |
const QString & | sets, | |||
FolderJob::JobType | jt = FolderJob::tGetMessage , |
|||
KMFolder * | folder = 0 | |||
) | const |
Definition at line 349 of file kmfolder.cpp.
FolderJob * KMFolder::createJob | ( | KMMessage * | msg, | |
FolderJob::JobType | jt = FolderJob::tGetMessage , |
|||
KMFolder * | folder = 0 , |
|||
QString | partSpecifier = QString::null , |
|||
const AttachmentStrategy * | as = 0 | |||
) | const |
These methods create respective FolderJob (You should derive FolderJob for each derived KMFolder).
Definition at line 342 of file kmfolder.cpp.
void KMFolder::daysToExpire | ( | int & | unreadDays, | |
int & | readDays | |||
) |
Definition at line 777 of file kmfolder.cpp.
bool KMFolder::dirty | ( | ) | const |
Returns true if the table of contents is dirty.
This happens when a message is deleted from the folder. The toc will then be re-created when the folder is closed.
Definition at line 532 of file kmfolder.cpp.
void KMFolder::emitMsgAddedSignals | ( | int | idx | ) |
Called by derived classes implementation of addMsg.
Emits msgAdded signals
Definition at line 401 of file kmfolder.cpp.
ExpireAction KMFolder::expireAction | ( | ) | const [inline] |
void KMFolder::expireOldMessages | ( | bool | immediate | ) |
Expire old messages in this folder.
If immediate is true, do it immediately; otherwise schedule it for later
Definition at line 782 of file kmfolder.cpp.
QString KMFolder::expireToFolderId | ( | ) | const [inline] |
If expiry should move to folder, return the ID of that folder.
Definition at line 471 of file kmfolder.h.
int KMFolder::expunge | ( | ) |
Delete entire folder.
Forces a close *but* opens the folder again afterwards. Returns errno(3) error code or zero on success. see KMFolder::expungeContents
Definition at line 522 of file kmfolder.cpp.
void KMFolder::expunged | ( | KMFolder * | ) | [signal] |
Emitted after an expunge.
If not quiet, changed() will be emmitted first.
int KMFolder::expungeOldMsg | ( | int | days | ) |
Delete messages in the folder that are older than days.
Return the number of deleted messages.
Definition at line 416 of file kmfolder.cpp.
QString KMFolder::fileName | ( | ) | const |
Returns the filename of the folder (reimplemented in KMFolderImap).
Definition at line 234 of file kmfolder.cpp.
int KMFolder::find | ( | const KMMessage * | msg | ) | const |
Definition at line 436 of file kmfolder.cpp.
int KMFolder::find | ( | const KMMsgBase * | msg | ) | const |
Returns the index of the given message or -1 if not found.
Definition at line 431 of file kmfolder.cpp.
void KMFolder::folderSizeChanged | ( | KMFolder * | ) | [signal] |
Emitted when the folder's size changes.
KMFolderType KMFolder::folderType | ( | ) | const |
DwString KMFolder::getDwString | ( | int | idx | ) |
KMMessage * KMFolder::getMsg | ( | int | idx | ) |
KMMsgBase * KMFolder::getMsgBase | ( | int | idx | ) |
Definition at line 361 of file kmfolder.cpp.
const KMMsgBase * KMFolder::getMsgBase | ( | int | idx | ) | const |
Provides access to the basic message fields that are also stored in the index.
Whenever you only need subject, from, date, status you should use this method instead of getMsg() because getMsg() will load the message if necessary and this method does not.
Definition at line 356 of file kmfolder.cpp.
int KMFolder::getReadExpireAge | ( | ) | const [inline] |
Get the age at which read messages are expired.
Units are determined by getReadExpireUnits().
Definition at line 447 of file kmfolder.h.
ExpireUnits KMFolder::getReadExpireUnits | ( | ) | const [inline] |
Units getReadExpireAge() is returned in.
1 = days, 2 = weeks, 3 = months.
Definition at line 459 of file kmfolder.h.
int KMFolder::getUnreadExpireAge | ( | ) | const [inline] |
Get the age at which unread messages are expired.
Units are determined by getUnreadExpireUnits().
Definition at line 441 of file kmfolder.h.
ExpireUnits KMFolder::getUnreadExpireUnits | ( | ) | const [inline] |
Units getUnreadExpireAge() is returned in.
1 = days, 2 = weeks, 3 = months.
Definition at line 453 of file kmfolder.h.
bool KMFolder::hasAccounts | ( | ) | const [inline] |
Returns TRUE if accounts are associated with this folder.
Definition at line 127 of file kmfolder.h.
void KMFolder::iconsChanged | ( | ) | [signal] |
Emitted when the icon paths are set.
uint KMFolder::identity | ( | ) | const |
Definition at line 619 of file kmfolder.cpp.
QString KMFolder::idString | ( | ) | const |
Returns a string that can be used to identify this folder.
Definition at line 686 of file kmfolder.cpp.
void KMFolder::ignoreJobsForMessage | ( | KMMessage * | m | ) |
Removes and deletes all jobs associated with the particular message.
Definition at line 337 of file kmfolder.cpp.
bool KMFolder::ignoreNewMail | ( | ) | const [inline] |
Returns true if the user doesn't want to get notified about new mail in this folder.
Definition at line 518 of file kmfolder.h.
QString KMFolder::indexLocation | ( | ) | const |
bool KMFolder::isAutoExpire | ( | ) | const [inline] |
bool KMFolder::isDrafts | ( | ) | [inline] |
Returns true if this folder is the drafts box of the local account, or is configured to be the drafts box of any of the users identities.
Definition at line 114 of file kmfolder.h.
bool KMFolder::isMailingListEnabled | ( | ) | const [inline] |
Definition at line 377 of file kmfolder.h.
bool KMFolder::isMainInbox | ( | ) | [inline] |
Returns true if this folder is the inbox on the local disk.
Definition at line 95 of file kmfolder.h.
bool KMFolder::isMessage | ( | int | idx | ) |
bool KMFolder::isMoveable | ( | ) | const |
bool KMFolder::isOpened | ( | ) | const |
bool KMFolder::isOutbox | ( | ) | [inline] |
Returns true only if this is the outbox for outgoing mail.
Definition at line 99 of file kmfolder.h.
bool KMFolder::isReadOnly | ( | ) | const |
bool KMFolder::isSent | ( | ) | [inline] |
Returns true if this folder is the sent-mail box of the local account, or is configured to be the sent mail box of any of the users identities.
Definition at line 104 of file kmfolder.h.
bool KMFolder::isSystemFolder | ( | ) | const [inline] |
Returns true if the folder is a kmail system folder.
These are the folders 'inbox', 'outbox', 'sent', 'trash', 'drafts', 'templates'. The name of these folders is nationalized in the folder display and they cannot have accounts associated. Deletion is also forbidden. Etc.
Definition at line 361 of file kmfolder.h.
bool KMFolder::isTemplates | ( | ) | [inline] |
Returns true if this folder is the templates folder of the local account, or is configured to be the templates folder of any of the users identities.
Definition at line 119 of file kmfolder.h.
bool KMFolder::isTrash | ( | ) | [inline] |
Returns true if this folder is configured as a trash folder, locally or for one of the accounts.
Definition at line 109 of file kmfolder.h.
QString KMFolder::label | ( | void | ) | const [virtual] |
Returns the label of the folder for visualization.
Reimplemented from KMFolderNode.
Definition at line 562 of file kmfolder.cpp.
QString KMFolder::location | ( | void | ) | const |
MailingList KMFolder::mailingList | ( | ) | const [inline] |
Definition at line 380 of file kmfolder.h.
QString KMFolder::mailingListPostAddress | ( | ) | const |
Definition at line 586 of file kmfolder.cpp.
void KMFolder::markNewAsUnread | ( | ) |
void KMFolder::markUnreadAsRead | ( | ) |
bool KMFolder::moveInProgress | ( | ) | const [inline] |
Returns true if there is currently a move or copy operation going on with this folder as target.
Definition at line 530 of file kmfolder.h.
Definition at line 426 of file kmfolder.cpp.
int KMFolder::moveMsg | ( | KMMessage * | msg, | |
int * | index_return = 0 | |||
) |
Detaches the given message from it's current folder and adds it to this folder.
Returns zero on success and an errno error code on failure. The index of the new message is stored in index_return if given.
Definition at line 421 of file kmfolder.cpp.
void KMFolder::msgAdded | ( | KMFolder * | , | |
Q_UINT32 | sernum | |||
) | [signal] |
void KMFolder::msgAdded | ( | int | idx | ) | [signal] |
Emitted when a message is added from the folder.
void KMFolder::msgChanged | ( | KMFolder * | , | |
Q_UINT32 | sernum, | |||
int | delta | |||
) | [signal] |
Emitted, when the status of a message is changed.
void KMFolder::msgHeaderChanged | ( | KMFolder * | , | |
int | ||||
) | [signal] |
Emitted when a field of the header of a specific message changed.
void KMFolder::msgRemoved | ( | KMFolder * | ) | [signal] |
void KMFolder::msgRemoved | ( | int | idx, | |
QString | msgIdMD5 | |||
) | [signal] |
Emitted after a message is removed from the folder.
void KMFolder::msgRemoved | ( | KMFolder * | , | |
Q_UINT32 | sernum | |||
) | [signal] |
Emitted before a message is removed from the folder.
void KMFolder::msgStatusChanged | ( | const KMMsgStatus | oldStatus, | |
const KMMsgStatus | newStatus, | |||
int | idx | |||
) |
Called by KMMsgBase::setStatus when status of a message has changed required to keep the number unread messages variable current.
Definition at line 469 of file kmfolder.cpp.
void KMFolder::nameChanged | ( | ) | [signal] |
Emitted when the name of the folder changes.
bool KMFolder::needsCompacting | ( | ) | const |
bool KMFolder::noChildren | ( | ) | const |
bool KMFolder::noContent | ( | ) | const |
Returns, if the folder can't contain mails, but only subfolder.
Definition at line 297 of file kmfolder.cpp.
QString KMFolder::normalIconPath | ( | ) | const [inline] |
Definition at line 493 of file kmfolder.h.
void KMFolder::numUnreadMsgsChanged | ( | KMFolder * | ) | [signal] |
Emitted when number of unread messages has changed.
int KMFolder::open | ( | const char * | owner | ) |
Open folder for access.
Does nothing if the folder is already opened. To reopen a folder call close() first. Returns zero on success and an error code equal to the c-library fopen call otherwise (errno).
Definition at line 475 of file kmfolder.cpp.
KMMsgBase * KMFolder::operator[] | ( | int | idx | ) |
const KMMsgBase * KMFolder::operator[] | ( | int | idx | ) | const |
QString KMFolder::prettyURL | ( | ) | const [virtual] |
URL of the node for visualization purposes.
Implements KMFolderNode.
Definition at line 574 of file kmfolder.cpp.
bool KMFolder::putRepliesInSameFolder | ( | ) | const [inline] |
Returns true if the replies to mails from this folder should be put in the same folder.
Definition at line 511 of file kmfolder.h.
void KMFolder::quiet | ( | bool | beQuiet | ) |
If set to quiet the folder will not emit msgAdded(idx) signal.
This is necessary because adding the messages to the listview one by one as they come in ( as happens on msgAdded(idx) ) is very slow for large ( >10000 ) folders. For pop, where whole bodies are downloaded this is not an issue, but for imap, where we only download headers it becomes a bottleneck. We therefore set the folder quiet() and rebuild the listview completely once the complete folder has been checked.
Definition at line 552 of file kmfolder.cpp.
void KMFolder::readConfig | ( | KConfig * | config | ) |
This is used by the storage to read the folder specific configuration.
Definition at line 157 of file kmfolder.cpp.
void KMFolder::reallyAddCopyOfMsg | ( | KMMessage * | aMsg | ) | [slot] |
Add a copy of the message to the folder after it has been retrieved from an IMAP server.
Definition at line 847 of file kmfolder.cpp.
void KMFolder::reallyAddMsg | ( | KMMessage * | aMsg | ) | [slot] |
Add the message to the folder after it has been retrieved from an IMAP server.
Definition at line 842 of file kmfolder.cpp.
void KMFolder::remove | ( | ) |
Removes the folder physically from disk and empties the contents of the folder in memory.
Note that the folder is closed during this process, whether there are others using it or not. see KMFolder::removeContents
Definition at line 511 of file kmfolder.cpp.
void KMFolder::removed | ( | KMFolder * | , | |
bool | ||||
) | [signal] |
Emitted when a folder was removed.
void KMFolder::removeJobs | ( | ) |
Definition at line 832 of file kmfolder.cpp.
Definition at line 411 of file kmfolder.cpp.
void KMFolder::removeMsg | ( | int | i, | |
bool | imapQuiet = false | |||
) |
Remove (first occurrence of) given message from the folder.
Definition at line 406 of file kmfolder.cpp.
int KMFolder::rename | ( | const QString & | newName, | |
KMFolderDir * | aParent = 0 | |||
) |
Physically rename the folder.
Returns zero on success and an errno on failure.
Definition at line 527 of file kmfolder.cpp.
void KMFolder::setAcctList | ( | AccountList * | list | ) | [inline] |
Definition at line 123 of file kmfolder.h.
void KMFolder::setAutoExpire | ( | bool | enabled | ) |
Set whether this folder automatically expires messages.
Definition at line 706 of file kmfolder.cpp.
void KMFolder::setChild | ( | KMFolderDir * | aChild | ) |
void KMFolder::setDirty | ( | bool | f | ) |
void KMFolder::setExpireAction | ( | ExpireAction | a | ) |
Definition at line 745 of file kmfolder.cpp.
void KMFolder::setExpireToFolderId | ( | const QString & | id | ) |
Definition at line 753 of file kmfolder.cpp.
Definition at line 823 of file kmfolder.cpp.
void KMFolder::setIdentity | ( | uint | identity | ) |
Definition at line 613 of file kmfolder.cpp.
void KMFolder::setIgnoreNewMail | ( | bool | b | ) | [inline] |
Definition at line 519 of file kmfolder.h.
void KMFolder::setLabel | ( | const QString & | l | ) | [inline] |
Definition at line 366 of file kmfolder.h.
void KMFolder::setMailingList | ( | const MailingList & | mlist | ) |
Definition at line 607 of file kmfolder.cpp.
void KMFolder::setMailingListEnabled | ( | bool | enabled | ) |
Returns true if this folder is associated with a mailing-list.
Definition at line 601 of file kmfolder.cpp.
void KMFolder::setMoveInProgress | ( | bool | b | ) | [inline] |
void KMFolder::setNeedsCompacting | ( | bool | f | ) |
Definition at line 547 of file kmfolder.cpp.
void KMFolder::setNoChildren | ( | bool | aNoChildren | ) |
void KMFolder::setNoContent | ( | bool | aNoContent | ) |
void KMFolder::setPutRepliesInSameFolder | ( | bool | b | ) | [inline] |
Definition at line 512 of file kmfolder.h.
void KMFolder::setReadExpireAge | ( | int | age | ) |
Set the maximum age for read messages in this folder.
Age should not be negative. Units are set using setReadExpireUnits().
Definition at line 729 of file kmfolder.cpp.
void KMFolder::setReadExpireUnits | ( | ExpireUnits | units | ) |
Set units to use for expiry of read messages.
Values are 1 = days, 2 = weeks, 3 = months.
Definition at line 737 of file kmfolder.cpp.
void KMFolder::setShortcut | ( | const KShortcut & | sc | ) |
Definition at line 852 of file kmfolder.cpp.
void KMFolder::setStatus | ( | QValueList< int > & | ids, | |
KMMsgStatus | status, | |||
bool | toggle = false | |||
) |
Set the status of the message(s) in the QValueList ids
to status
.
Definition at line 817 of file kmfolder.cpp.
void KMFolder::setStatus | ( | int | idx, | |
KMMsgStatus | status, | |||
bool | toggle = false | |||
) |
void KMFolder::setSystemFolder | ( | bool | itIs | ) | [inline] |
Definition at line 362 of file kmfolder.h.
void KMFolder::setSystemLabel | ( | const QString & | l | ) | [inline] |
Definition at line 370 of file kmfolder.h.
void KMFolder::setUnreadExpireAge | ( | int | age | ) |
Set the maximum age for unread messages in this folder.
Age should not be negative. Units are set using setUnreadExpireUnits().
Definition at line 714 of file kmfolder.cpp.
void KMFolder::setUnreadExpireUnits | ( | ExpireUnits | units | ) |
Set units to use for expiry of unread messages.
Values are 1 = days, 2 = weeks, 3 = months.
Definition at line 722 of file kmfolder.cpp.
void KMFolder::setUseCustomIcons | ( | bool | useCustomIcons | ) | [inline] |
Definition at line 492 of file kmfolder.h.
void KMFolder::setUserWhoField | ( | const QString & | whoField, | |
bool | writeConfig = true | |||
) |
Definition at line 638 of file kmfolder.cpp.
void KMFolder::setWhoField | ( | const QString & | aWhoField | ) |
Definition at line 629 of file kmfolder.cpp.
const KShortcut& KMFolder::shortcut | ( | ) | const [inline] |
Definition at line 521 of file kmfolder.h.
void KMFolder::shortcutChanged | ( | KMFolder * | ) | [signal] |
Emitted when the shortcut associated with this folder changes.
void KMFolder::statusMsg | ( | const QString & | ) | [signal] |
Emmited to display a message somewhere in a status line.
const FolderStorage* KMFolder::storage | ( | ) | const [inline] |
FolderStorage* KMFolder::storage | ( | ) | [inline] |
Definition at line 135 of file kmfolder.h.
QString KMFolder::subdirLocation | ( | ) | const |
void KMFolder::sync | ( | ) |
virtual QString KMFolder::systemLabel | ( | ) | const [inline, virtual] |
Definition at line 381 of file kmfolder.cpp.
KMMessage * KMFolder::take | ( | int | idx | ) |
Detach message from this folder.
Usable to call addMsg() afterwards. Loads the message if it is not loaded up to now.
Definition at line 376 of file kmfolder.cpp.
KMFolder * KMFolder::trashFolder | ( | ) | const |
If this folder has a special trash folder set, return it.
Otherwise return 0.
Definition at line 802 of file kmfolder.cpp.
KMMsgInfo * KMFolder::unGetMsg | ( | int | idx | ) |
QString KMFolder::unreadIconPath | ( | ) | const [inline] |
Definition at line 494 of file kmfolder.h.
int KMFolder::updateIndex | ( | ) | [slot] |
Incrementally update the index if possible else call writeIndex.
Definition at line 837 of file kmfolder.cpp.
bool KMFolder::useCustomIcons | ( | ) | const [inline] |
QString KMFolder::userWhoField | ( | void | ) | [inline] |
Get / set the user-settings for the WhoField (From/To/Empty).
Definition at line 392 of file kmfolder.h.
void KMFolder::viewConfigChanged | ( | ) | [signal] |
Emitted when the variables for the config of the view have changed.
QString KMFolder::whoField | ( | ) | const [inline] |
Get / set the name of the field that is used for the Sender/Receiver column in the headers (From/To).
Definition at line 388 of file kmfolder.h.
void KMFolder::writeConfig | ( | KConfig * | config | ) | const |
This is used by the storage to save the folder specific configuration.
Definition at line 196 of file kmfolder.cpp.
int KMFolder::writeIndex | ( | bool | createEmptyIndex = false |
) |
Write index to index-file.
Returns 0 on success and errno error on failure.
Definition at line 807 of file kmfolder.cpp.
The documentation for this class was generated from the following files: