kmail
KMFolderImap Class Reference
#include <kmfolderimap.h>
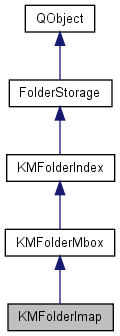
Public Types | |
enum | imapState { imapNoInformation = 0, imapListingInProgress = 1, imapDownloadInProgress = 2, imapFinished = 3 } |
Public Slots | |
virtual int | addMsg (QPtrList< KMMessage > &, QValueList< int > &index_return) |
virtual int | addMsg (KMMessage *msg, int *index_return=0) |
virtual void | addMsgQuiet (QPtrList< KMMessage >) |
virtual void | addMsgQuiet (KMMessage *) |
void | copyMsg (QPtrList< KMMessage > &msgList) |
static void | flagsToStatus (KMMsgBase *msg, int flags, bool newMsg=TRUE, int supportedFalgs=31) |
static void | seenFlagToStatus (KMMsgBase *msg, int flags, bool newMsg=true) |
void | slotCheckNamespace (const QStringList &, const QStringList &, const QStringList &, const QStringList &, const ImapAccountBase::jobData &) |
void | slotCopyMsgResult (KMail::FolderJob *job) |
void | slotListResult (const QStringList &, const QStringList &, const QStringList &, const QStringList &, const ImapAccountBase::jobData &) |
void | slotSearchDone (Q_UINT32 serNum, const KMSearchPattern *pattern, bool matches) |
void | slotSearchDone (QValueList< Q_UINT32 > serNums, const KMSearchPattern *pattern, bool complete) |
void | slotSimpleData (KIO::Job *job, const QByteArray &data) |
virtual void | take (QPtrList< KMMessage >) |
virtual KMMessage * | take (int idx) |
Signals | |
void | deleted (KMFolderImap *) |
void | directoryListingFinished (KMFolderImap *) |
void | folderComplete (KMFolderImap *folder, bool success) |
void | folderCreationResult (const QString &name, bool success) |
Public Member Functions | |
KMAcctImap * | account () const |
bool | autoExpunge () |
virtual int | compact (bool) |
virtual int | create () |
void | createFolder (const QString &name, const QString &imapPath=QString::null, bool askUser=true) |
void | deleteMessage (const QPtrList< KMMessage > &msgList) |
void | deleteMessage (KMMessage *msg) |
void | expungeFolder (KMFolderImap *aFolder, bool quiet) |
virtual QString | fileName () const |
virtual KMFolderType | folderType () const |
void | getAndCheckFolder (bool force=FALSE) |
virtual imapState | getContentState () |
void | getFolder (bool force=FALSE) |
void | getMessage (KMFolder *folder, KMMessage *msg) |
virtual KMMessage * | getMsg (int idx) |
virtual imapState | getSubfolderState () |
void | getUids (const QPtrList< KMMessage > &msgList, QValueList< ulong > &uids) |
void | getUids (QValueList< int > &ids, QValueList< ulong > &uids) |
virtual void | ignoreJobsForMessage (KMMessage *) |
QString | imapPath () const |
bool | includeInMailCheck () |
void | initializeFrom (KMFolderImap *parent, QString path, QString mimeType) |
virtual bool | isAutoExpire () const |
virtual bool | isMoveable () const |
bool | isReadOnly () const |
bool | isSelected () |
KMFolderImap (KMFolder *folder, const char *name=0) | |
ulong | lastUid () |
virtual bool | listDirectory () |
int | permanentFlags () const |
bool | processNewMail (bool interactive) |
virtual void | readConfig () |
virtual void | reallyDoClose (const char *owner) |
virtual void | remove () |
virtual void | removeMsg (const QPtrList< KMMessage > &msgList, bool quiet=FALSE) |
virtual void | removeMsg (int i, bool quiet=FALSE) |
virtual int | rename (const QString &newName, KMFolderDir *aParent=0) |
void | saveMsgMetaData (KMMessage *msg, ulong uid=0) |
virtual void | search (const KMSearchPattern *, Q_UINT32 serNum) |
virtual void | search (const KMSearchPattern *) |
void | sendFolderComplete (bool success) |
const ulong | serNumForUID (ulong uid) |
void | setAccount (KMAcctImap *acct) |
void | setAlreadyRemoved (bool removed) |
void | setCheckingValidity (bool val) |
virtual void | setContentState (imapState state) |
void | setImapPath (const QString &path) |
void | setIncludeInMailCheck (bool check) |
void | setSelected (bool selected) |
virtual void | setStatus (QValueList< int > &_ids, KMMsgStatus status, bool toggle) |
virtual void | setStatus (int idx, KMMsgStatus status, bool toggle) |
virtual void | setSubfolderState (imapState state) |
void | setUidValidity (const QString &validity) |
void | setUserRights (unsigned int userRights) |
KMFolder * | trashFolder () const |
QString | uidValidity () |
unsigned int | userRights () const |
virtual void | writeConfig () |
virtual | ~KMFolderImap () |
Static Public Member Functions | |
static QString | cacheLocation () |
static QString | decodeFileName (const QString &) |
static QString | encodeFileName (const QString &) |
static QStringList | makeSets (const QStringList &, bool sort=true) |
static QStringList | makeSets (QValueList< ulong > &, bool sort=true) |
static QPtrList< KMMessage > | splitMessageList (const QString &set, QPtrList< KMMessage > &msgList) |
static QValueList< ulong > | splitSets (const QString) |
static QString | statusToFlags (KMMsgStatus status, int supportedFalgs) |
static QTextCodec * | utf7Codec () |
Protected Slots | |
void | checkValidity () |
void | reallyGetFolder (const QString &startUid=QString::null) |
void | slotCheckValidityResult (KIO::Job *job) |
void | slotCompleteMailCheckProgress () |
void | slotCreateFolderResult (KIO::Job *job) |
void | slotCreatePendingFolders (int errorCode, const QString &errorMsg) |
void | slotGetLastMessagesResult (KIO::Job *job) |
void | slotGetMessagesData (KIO::Job *job, const QByteArray &data) |
void | slotGetMessagesResult (KIO::Job *job) |
void | slotListFolderEntries (KIO::Job *job, const KIO::UDSEntryList &uds) |
void | slotListFolderResult (KIO::Job *job) |
void | slotListNamespaces () |
void | slotProcessNewMail (int errorCode, const QString &errorMsg) |
void | slotRemoveFolderResult (KIO::Job *job) |
void | slotStatResult (KIO::Job *job) |
Protected Member Functions | |
void | checkFolders (const QStringList &folderNames, const QString &ns) |
virtual FolderJob * | doCreateJob (QPtrList< KMMessage > &msgList, const QString &sets, FolderJob::JobType jt, KMFolder *folder) const |
virtual FolderJob * | doCreateJob (KMMessage *msg, FolderJob::JobType jt, KMFolder *folder, QString partSpecifier, const AttachmentStrategy *as) const |
virtual int | expungeContents () |
KMFolderImap * | findParent (const QString &path, const QString &name) |
void | finishMailCheck (const char *func, imapState state) |
void | getMessagesResult (KIO::Job *job, bool lastSet) |
void | initInbox () |
void | setChildrenState (QString attributes) |
Protected Attributes | |
QGuardedPtr< KMAcctImap > | mAccount |
bool | mCheckFlags |
bool | mCheckMail |
imapState | mContentState |
QString | mImapPath |
bool | mIsSelected |
ulong | mLastUid |
bool | mReadOnly |
imapState | mSubfolderState |
QString | mUidValidity |
unsigned int | mUserRights |
Detailed Description
Definition at line 77 of file kmfolderimap.h.
Member Enumeration Documentation
Definition at line 87 of file kmfolderimap.h.
Constructor & Destructor Documentation
KMFolderImap::KMFolderImap | ( | KMFolder * | folder, | |
const char * | name = 0 | |||
) |
Usually a parent is given.
But in some cases there is no fitting parent object available. Then the name of the folder is used as the absolute path to the folder file.
Definition at line 63 of file kmfolderimap.cpp.
KMFolderImap::~KMFolderImap | ( | ) | [virtual] |
Definition at line 87 of file kmfolderimap.cpp.
Member Function Documentation
KMAcctImap * KMFolderImap::account | ( | ) | const [virtual] |
int KMFolderImap::addMsg | ( | QPtrList< KMMessage > & | msgList, | |
QValueList< int > & | index_return | |||
) | [virtual, slot] |
Adds the given messages to the folder.
Behaviour is identical to addMsg(msg)
Reimplemented from FolderStorage.
Definition at line 398 of file kmfolderimap.cpp.
int KMFolderImap::addMsg | ( | KMMessage * | msg, | |
int * | index_return = 0 | |||
) | [virtual, slot] |
Add the given message to the folder.
Usually the message is added at the end of the folder. Returns zero on success and an errno error code on failure. The index of the new message is stored in index_return if given. Please note that the message is added as is to the folder and the folder takes ownership of the message (deleting it in the destructor).
Reimplemented from KMFolderMbox.
Definition at line 388 of file kmfolderimap.cpp.
Definition at line 353 of file kmfolderimap.cpp.
void KMFolderImap::addMsgQuiet | ( | KMMessage * | aMsg | ) | [virtual, slot] |
Add a message to a folder after is has been added on an IMAP server.
Definition at line 326 of file kmfolderimap.cpp.
bool KMFolderImap::autoExpunge | ( | ) |
Automatically expunge deleted messages when leaving the folder.
Definition at line 1799 of file kmfolderimap.cpp.
static QString KMFolderImap::cacheLocation | ( | ) | [inline, static] |
Definition at line 83 of file kmfolderimap.h.
void KMFolderImap::checkFolders | ( | const QStringList & | folderNames, | |
const QString & | ns | |||
) | [protected] |
See if all folders are still present on server, otherwise delete them.
Definition at line 948 of file kmfolderimap.cpp.
void KMFolderImap::checkValidity | ( | ) | [protected, slot] |
virtual int KMFolderImap::compact | ( | bool | silent | ) | [inline, virtual] |
Remove deleted messages from the folder.
Returns zero on success and an errno on failure.
Reimplemented from KMFolderMbox.
Definition at line 211 of file kmfolderimap.h.
int KMFolderImap::create | ( | ) | [virtual] |
void KMFolderImap::createFolder | ( | const QString & | name, | |
const QString & | imapPath = QString::null , |
|||
bool | askUser = true | |||
) |
Create a new subfolder You may specify the root imap path or this folder will be used If you set askUser to false and the server can only handle folders that contain messages _or_ folders the new folder is set to "contains messages" by default.
Definition at line 1720 of file kmfolderimap.cpp.
Definition at line 1792 of file kmfolderimap.cpp.
void KMFolderImap::deleted | ( | KMFolderImap * | ) | [signal] |
Emitted, when the account is deleted.
Definition at line 1847 of file kmfolderimap.cpp.
void KMFolderImap::deleteMessage | ( | KMMessage * | msg | ) |
void KMFolderImap::directoryListingFinished | ( | KMFolderImap * | ) | [signal] |
Emitted at the end of the directory listing.
FolderJob * KMFolderImap::doCreateJob | ( | KMMessage * | msg, | |
FolderJob::JobType | jt, | |||
KMFolder * | folder, | |||
QString | partSpecifier, | |||
const AttachmentStrategy * | as | |||
) | const [protected, virtual] |
These two methods actually create the jobs.
They have to be implemented in all folders.
- See also:
- createJob
Reimplemented from KMFolderMbox.
Definition at line 1645 of file kmfolderimap.cpp.
Encode the given string in a filename save 7 bit string.
Definition at line 1784 of file kmfolderimap.cpp.
int KMFolderImap::expungeContents | ( | ) | [protected, virtual] |
Called by KMFolder::expunge() to delete the actual contents.
At the time of the call the folder has already been closed, and the various index files deleted. Returns 0 on success.
Reimplemented from KMFolderMbox.
Definition at line 2234 of file kmfolderimap.cpp.
void KMFolderImap::expungeFolder | ( | KMFolderImap * | aFolder, | |
bool | quiet | |||
) |
virtual QString KMFolderImap::fileName | ( | ) | const [inline, virtual] |
Return the filename of the folder (reimplemented from KFolder).
Reimplemented from FolderStorage.
Definition at line 247 of file kmfolderimap.h.
KMFolderImap * KMFolderImap::findParent | ( | const QString & | path, | |
const QString & | name | |||
) | [protected] |
void KMFolderImap::finishMailCheck | ( | const char * | func, | |
imapState | state | |||
) | [protected] |
Definition at line 2426 of file kmfolderimap.cpp.
void KMFolderImap::flagsToStatus | ( | KMMsgBase * | msg, | |
int | flags, | |||
bool | newMsg = TRUE , |
|||
int | supportedFalgs = 31 | |||
) | [static, slot] |
Convert IMAP flags to a message status.
- Parameters:
-
newMsg specifies whether unseen messages are new or unread
Definition at line 1423 of file kmfolderimap.cpp.
void KMFolderImap::folderComplete | ( | KMFolderImap * | folder, | |
bool | success | |||
) | [signal] |
void KMFolderImap::folderCreationResult | ( | const QString & | name, | |
bool | success | |||
) | [signal] |
Emitted when a folder creation has finished.
- Parameters:
-
name The name of the folder that should have been created. success True if the folder was created, false otherwise.
virtual KMFolderType KMFolderImap::folderType | ( | ) | const [inline, virtual] |
Returns the type of this folder.
Reimplemented from KMFolderMbox.
Definition at line 107 of file kmfolderimap.h.
void KMFolderImap::getAndCheckFolder | ( | bool | force = FALSE |
) |
virtual imapState KMFolderImap::getContentState | ( | ) | [inline, virtual] |
Definition at line 94 of file kmfolderimap.h.
void KMFolderImap::getFolder | ( | bool | force = FALSE |
) |
void KMFolderImap::getMessagesResult | ( | KIO::Job * | job, | |
bool | lastSet | |||
) | [protected] |
Definition at line 1689 of file kmfolderimap.cpp.
KMMessage * KMFolderImap::getMsg | ( | int | idx | ) | [virtual] |
Read message at given index.
Indexing starts at zero
Reimplemented from FolderStorage.
Definition at line 138 of file kmfolderimap.cpp.
virtual imapState KMFolderImap::getSubfolderState | ( | ) | [inline, virtual] |
Definition at line 97 of file kmfolderimap.h.
void KMFolderImap::getUids | ( | const QPtrList< KMMessage > & | msgList, | |
QValueList< ulong > & | uids | |||
) |
void KMFolderImap::getUids | ( | QValueList< int > & | ids, | |
QValueList< ulong > & | uids | |||
) |
void KMFolderImap::ignoreJobsForMessage | ( | KMMessage * | msg | ) | [virtual] |
Removes and deletes all jobs associated with the particular message.
Reimplemented from FolderStorage.
Definition at line 1508 of file kmfolderimap.cpp.
QString KMFolderImap::imapPath | ( | ) | const [inline] |
Definition at line 112 of file kmfolderimap.h.
bool KMFolderImap::includeInMailCheck | ( | ) | [inline] |
void KMFolderImap::initializeFrom | ( | KMFolderImap * | parent, | |
QString | path, | |||
QString | mimeType | |||
) |
Initialize this storage from another one.
Used when creating a child folder
Definition at line 993 of file kmfolderimap.cpp.
void KMFolderImap::initInbox | ( | ) | [protected] |
virtual bool KMFolderImap::isAutoExpire | ( | ) | const [inline, virtual] |
bool KMFolderImap::isMoveable | ( | ) | const [virtual] |
Returns true if this folder can be moved.
Reimplemented from FolderStorage.
Definition at line 2388 of file kmfolderimap.cpp.
bool KMFolderImap::isReadOnly | ( | ) | const [inline, virtual] |
Is the folder readonly?
Reimplemented from KMFolderMbox.
Definition at line 296 of file kmfolderimap.h.
bool KMFolderImap::isSelected | ( | ) | [inline] |
Definition at line 230 of file kmfolderimap.h.
ulong KMFolderImap::lastUid | ( | ) |
bool KMFolderImap::listDirectory | ( | ) | [virtual] |
List a directory and add the contents to kmfoldermgr It uses a ListJob to get the folders returns false if the connection failed.
Definition at line 739 of file kmfolderimap.cpp.
QStringList KMFolderImap::makeSets | ( | const QStringList & | uids, | |
bool | sort = true | |||
) | [static] |
Definition at line 1977 of file kmfolderimap.cpp.
QStringList KMFolderImap::makeSets | ( | QValueList< ulong > & | uids, | |
bool | sort = true | |||
) | [static] |
int KMFolderImap::permanentFlags | ( | ) | const [inline] |
Returns the IMAP flags that can be stored on the server.
Definition at line 322 of file kmfolderimap.h.
bool KMFolderImap::processNewMail | ( | bool | interactive | ) |
Refresh the number of unseen mails Returns false in an error condition.
Definition at line 2094 of file kmfolderimap.cpp.
void KMFolderImap::readConfig | ( | void | ) | [virtual] |
Read the config file.
Reimplemented from FolderStorage.
Definition at line 191 of file kmfolderimap.cpp.
void KMFolderImap::reallyDoClose | ( | const char * | owner | ) | [virtual] |
Closes and cancels all pending jobs.
Reimplemented from KMFolderMbox.
Definition at line 109 of file kmfolderimap.cpp.
void KMFolderImap::reallyGetFolder | ( | const QString & | startUid = QString::null |
) | [protected, slot] |
void KMFolderImap::remove | ( | ) | [virtual] |
Remove the IMAP folder on the server and if successful also locally.
Reimplemented from FolderStorage.
Definition at line 230 of file kmfolderimap.cpp.
void KMFolderImap::removeMsg | ( | int | i, | |
bool | quiet = FALSE | |||
) | [virtual] |
Remove (first occurrence of) given message from the folder.
Reimplemented from FolderStorage.
Definition at line 277 of file kmfolderimap.cpp.
int KMFolderImap::rename | ( | const QString & | newName, | |
KMFolderDir * | aParent = 0 | |||
) | [virtual] |
Physically rename the folder.
Returns zero on success and an errno on failure.
Reimplemented from FolderStorage.
Definition at line 317 of file kmfolderimap.cpp.
void KMFolderImap::saveMsgMetaData | ( | KMMessage * | msg, | |
ulong | uid = 0 | |||
) |
Save the metadata for the UID If the UID is not supplied the one from the message is taken.
Definition at line 2407 of file kmfolderimap.cpp.
void KMFolderImap::search | ( | const KMSearchPattern * | pattern, | |
Q_UINT32 | serNum | |||
) | [virtual] |
Check if the message matches the search criteria The end is signaled with searchDone().
Reimplemented from FolderStorage.
Definition at line 2366 of file kmfolderimap.cpp.
void KMFolderImap::search | ( | const KMSearchPattern * | pattern | ) | [virtual] |
Search for messages The actual search is done in slotSearch and the end is signaled with searchDone().
Reimplemented from FolderStorage.
Definition at line 2342 of file kmfolderimap.cpp.
void KMFolderImap::seenFlagToStatus | ( | KMMsgBase * | msg, | |
int | flags, | |||
bool | newMsg = true | |||
) | [static, slot] |
Convert IMAP seen flag to a message status.
- Parameters:
-
newMsg specifies whether unseen messages are new or unread
Definition at line 1456 of file kmfolderimap.cpp.
void KMFolderImap::sendFolderComplete | ( | bool | success | ) | [inline] |
const ulong KMFolderImap::serNumForUID | ( | ulong | uid | ) |
Get the serial number for the given UID (if available).
Definition at line 2395 of file kmfolderimap.cpp.
void KMFolderImap::setAccount | ( | KMAcctImap * | acct | ) |
void KMFolderImap::setAlreadyRemoved | ( | bool | removed | ) |
Mark the folder as already removed from the server If set to true the folder will only be deleted locally This will recursively be applied to all children.
Definition at line 2309 of file kmfolderimap.cpp.
void KMFolderImap::setCheckingValidity | ( | bool | val | ) | [inline] |
Definition at line 283 of file kmfolderimap.h.
void KMFolderImap::setChildrenState | ( | QString | attributes | ) | [protected] |
Definition at line 1003 of file kmfolderimap.cpp.
virtual void KMFolderImap::setContentState | ( | imapState | state | ) | [inline, virtual] |
Definition at line 95 of file kmfolderimap.h.
void KMFolderImap::setImapPath | ( | const QString & | path | ) |
void KMFolderImap::setIncludeInMailCheck | ( | bool | check | ) |
Definition at line 2300 of file kmfolderimap.cpp.
void KMFolderImap::setSelected | ( | bool | selected | ) | [inline] |
Tell the folder, this it is selected and shall also display new mails, not only their number, when checking for mail.
Definition at line 229 of file kmfolderimap.h.
void KMFolderImap::setStatus | ( | QValueList< int > & | _ids, | |
KMMsgStatus | status, | |||
bool | toggle | |||
) | [virtual] |
Change the status of several messages indicated by ids
.
Reimplemented from FolderStorage.
Definition at line 1888 of file kmfolderimap.cpp.
void KMFolderImap::setStatus | ( | int | idx, | |
KMMsgStatus | status, | |||
bool | toggle | |||
) | [virtual] |
Change the status of the message indicated by index
Overloaded function for the following one.
Reimplemented from FolderStorage.
Definition at line 1882 of file kmfolderimap.cpp.
void KMFolderImap::setSubfolderState | ( | imapState | state | ) | [virtual] |
Definition at line 2281 of file kmfolderimap.cpp.
void KMFolderImap::setUidValidity | ( | const QString & | validity | ) | [inline] |
void KMFolderImap::setUserRights | ( | unsigned int | userRights | ) |
Set the user's rights on this folder - called by getUserRights.
Definition at line 2264 of file kmfolderimap.cpp.
void KMFolderImap::slotCheckNamespace | ( | const QStringList & | subfolderNames, | |
const QStringList & | subfolderPaths, | |||
const QStringList & | subfolderMimeTypes, | |||
const QStringList & | subfolderAttributes, | |||
const ImapAccountBase::jobData & | jobData | |||
) | [slot] |
Connected to slotListNamespaces creates/removes namespace folders.
Definition at line 668 of file kmfolderimap.cpp.
void KMFolderImap::slotCheckValidityResult | ( | KIO::Job * | job | ) | [protected, slot] |
Definition at line 1107 of file kmfolderimap.cpp.
void KMFolderImap::slotCompleteMailCheckProgress | ( | ) | [protected, slot] |
notify the progress item that the mail check for this folder is done.
Definition at line 2271 of file kmfolderimap.cpp.
void KMFolderImap::slotCopyMsgResult | ( | KMail::FolderJob * | job | ) | [slot] |
Connected to the result signal of the copy/move job.
Definition at line 516 of file kmfolderimap.cpp.
void KMFolderImap::slotCreateFolderResult | ( | KIO::Job * | job | ) | [protected, slot] |
void KMFolderImap::slotCreatePendingFolders | ( | int | errorCode, | |
const QString & | errorMsg | |||
) | [protected, slot] |
Is connected when there are folders to be created on startup and the account is still connecting.
Once the account emits the connected signal this slot is called and the folders created.
Definition at line 2327 of file kmfolderimap.cpp.
void KMFolderImap::slotGetLastMessagesResult | ( | KIO::Job * | job | ) | [protected, slot] |
Definition at line 1706 of file kmfolderimap.cpp.
void KMFolderImap::slotGetMessagesData | ( | KIO::Job * | job, | |
const QByteArray & | data | |||
) | [protected, slot] |
Definition at line 1521 of file kmfolderimap.cpp.
void KMFolderImap::slotGetMessagesResult | ( | KIO::Job * | job | ) | [protected, slot] |
void KMFolderImap::slotListFolderEntries | ( | KIO::Job * | job, | |
const KIO::UDSEntryList & | uds | |||
) | [protected, slot] |
Definition at line 1373 of file kmfolderimap.cpp.
void KMFolderImap::slotListFolderResult | ( | KIO::Job * | job | ) | [protected, slot] |
void KMFolderImap::slotListNamespaces | ( | ) | [protected, slot] |
void KMFolderImap::slotListResult | ( | const QStringList & | subfolderNames, | |
const QStringList & | subfolderPaths, | |||
const QStringList & | subfolderMimeTypes, | |||
const QStringList & | subfolderAttributes, | |||
const ImapAccountBase::jobData & | jobData | |||
) | [slot] |
Connected to ListJob::receivedFolders creates/removes folders.
Definition at line 774 of file kmfolderimap.cpp.
void KMFolderImap::slotProcessNewMail | ( | int | errorCode, | |
const QString & | errorMsg | |||
) | [protected, slot] |
Is called when the slave is connected and triggers a newmail check.
Definition at line 2082 of file kmfolderimap.cpp.
void KMFolderImap::slotRemoveFolderResult | ( | KIO::Job * | job | ) | [protected, slot] |
Remove the folder also locally, if removing on the server succeeded.
Definition at line 261 of file kmfolderimap.cpp.
void KMFolderImap::slotSearchDone | ( | Q_UINT32 | serNum, | |
const KMSearchPattern * | pattern, | |||
bool | matches | |||
) | [slot] |
Called from the SearchJob when the message was searched.
Definition at line 2381 of file kmfolderimap.cpp.
void KMFolderImap::slotSearchDone | ( | QValueList< Q_UINT32 > | serNums, | |
const KMSearchPattern * | pattern, | |||
bool | complete | |||
) | [slot] |
Called from the SearchJob when the folder is done or messages where found.
Definition at line 2358 of file kmfolderimap.cpp.
void KMFolderImap::slotSimpleData | ( | KIO::Job * | job, | |
const QByteArray & | data | |||
) | [slot] |
void KMFolderImap::slotStatResult | ( | KIO::Job * | job | ) | [protected, slot] |
QPtrList< KMMessage > KMFolderImap::splitMessageList | ( | const QString & | set, | |
QPtrList< KMMessage > & | msgList | |||
) | [static] |
splits the message list according to sets.
Modifies the .
Definition at line 550 of file kmfolderimap.cpp.
QValueList< ulong > KMFolderImap::splitSets | ( | const QString | uids | ) | [static] |
QString KMFolderImap::statusToFlags | ( | KMMsgStatus | status, | |
int | supportedFalgs | |||
) | [static] |
KMMessage * KMFolderImap::take | ( | int | idx | ) | [virtual, slot] |
Detach message from this folder.
Usable to call addMsg() afterwards. Loads the message if it is not loaded up to now.
Reimplemented from FolderStorage.
Definition at line 588 of file kmfolderimap.cpp.
KMFolder * KMFolderImap::trashFolder | ( | ) | const [virtual] |
Return the trash folder.
Reimplemented from FolderStorage.
Definition at line 131 of file kmfolderimap.cpp.
QString KMFolderImap::uidValidity | ( | ) | [inline] |
Definition at line 119 of file kmfolderimap.h.
unsigned int KMFolderImap::userRights | ( | ) | const [inline] |
The user's rights on this folder - see bitfield in ACLJobs namespace.
- Returns:
- 0 when not known yet
Definition at line 302 of file kmfolderimap.h.
QTextCodec * KMFolderImap::utf7Codec | ( | ) | [static] |
Definition at line 1776 of file kmfolderimap.cpp.
void KMFolderImap::writeConfig | ( | void | ) | [virtual] |
Write the config file.
Reimplemented from FolderStorage.
Definition at line 215 of file kmfolderimap.cpp.
Member Data Documentation
QGuardedPtr<KMAcctImap> KMFolderImap::mAccount [mutable, protected] |
Definition at line 514 of file kmfolderimap.h.
bool KMFolderImap::mCheckFlags [protected] |
Definition at line 511 of file kmfolderimap.h.
bool KMFolderImap::mCheckMail [protected] |
Definition at line 513 of file kmfolderimap.h.
imapState KMFolderImap::mContentState [protected] |
Definition at line 509 of file kmfolderimap.h.
QString KMFolderImap::mImapPath [protected] |
Definition at line 507 of file kmfolderimap.h.
bool KMFolderImap::mIsSelected [protected] |
Definition at line 510 of file kmfolderimap.h.
ulong KMFolderImap::mLastUid [protected] |
Definition at line 508 of file kmfolderimap.h.
bool KMFolderImap::mReadOnly [protected] |
imapState KMFolderImap::mSubfolderState [protected] |
Definition at line 509 of file kmfolderimap.h.
QString KMFolderImap::mUidValidity [protected] |
Definition at line 516 of file kmfolderimap.h.
unsigned int KMFolderImap::mUserRights [protected] |
Definition at line 517 of file kmfolderimap.h.
The documentation for this class was generated from the following files: