kmail
FolderStorage Class Reference
The FolderStorage class is the bass class for the storage related aspects of a collection of mail (a folder). More...
#include <folderstorage.h>
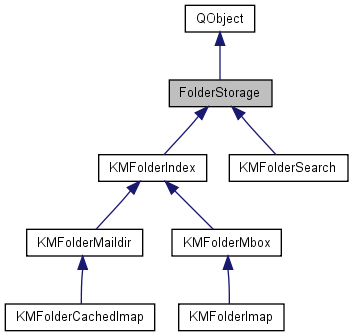
Public Types | |
enum | ChildrenState { HasChildren, HasNoChildren, ChildrenUnknown } |
Public Slots | |
virtual void | reallyAddCopyOfMsg (KMMessage *aMsg) |
virtual void | reallyAddMsg (KMMessage *aMsg) |
void | slotEmitChangedTimer () |
virtual int | updateIndex ()=0 |
Signals | |
void | changed () |
void | cleared () |
void | closed (KMFolder *) |
void | contentsTypeChanged (KMail::FolderContentsType type) |
void | expunged (KMFolder *) |
void | folderSizeChanged () |
void | invalidated (KMFolder *) |
void | locationChanged (const QString &, const QString &) |
void | msgAdded (KMFolder *, Q_UINT32 sernum) |
void | msgAdded (int idx) |
void | msgChanged (KMFolder *, Q_UINT32 sernum, int delta) |
void | msgHeaderChanged (KMFolder *, int) |
void | msgRemoved (KMFolder *) |
void | msgRemoved (int idx, QString msgIdMD5) |
void | msgRemoved (KMFolder *, Q_UINT32 sernum) |
void | nameChanged () |
void | numUnreadMsgsChanged (KMFolder *) |
void | readOnlyChanged (KMFolder *) |
void | removed (KMFolder *, bool) |
void | searchDone (KMFolder *, Q_UINT32, const KMSearchPattern *, bool) |
void | searchResult (KMFolder *, QValueList< Q_UINT32 >, const KMSearchPattern *, bool complete) |
void | statusMsg (const QString &) |
Public Member Functions | |
virtual KMAccount * | account () const |
void | addJob (FolderJob *) const |
virtual int | addMsg (QPtrList< KMMessage > &, QValueList< int > &index_return) |
virtual int | addMsg (KMMessage *msg, int *index_return=0)=0 |
virtual int | addMsgKeepUID (KMMessage *msg, int *index_return=0) |
bool | autoCreateIndex () const |
virtual int | canAccess ()=0 |
virtual bool | canAddMsgNow (KMMessage *aMsg, int *aIndex_ret) |
void | close (const char *owner, bool force=false) |
virtual int | compact (bool silent)=0 |
bool | compactable () const |
KMail::FolderContentsType | contentsType () const |
virtual void | correctUnreadMsgsCount () |
virtual int | count (bool cache=false) const |
virtual int | countUnread () |
virtual int | create ()=0 |
virtual FolderJob * | createJob (QPtrList< KMMessage > &msgList, const QString &sets, FolderJob::JobType jt=FolderJob::tGetMessage, KMFolder *folder=0) const |
virtual FolderJob * | createJob (KMMessage *msg, FolderJob::JobType jt=FolderJob::tGetMessage, KMFolder *folder=0, QString partSpecifier=QString::null, const AttachmentStrategy *as=0) const |
void | deregisterFromMessageDict () |
bool | dirty () const |
void | emitMsgAddedSignals (int idx) |
virtual int | expunge () |
virtual int | expungeOldMsg (int days) |
virtual QString | fileName () const |
int | find (const KMMessage *msg) const |
virtual int | find (const KMMsgBase *msg) const =0 |
KMFolder * | folder () const |
Q_INT64 | folderSize () const |
FolderStorage (KMFolder *folder, const char *name=0) | |
virtual KMFolderType | folderType () const |
virtual DwString | getDwString (int idx)=0 |
virtual KMMessage * | getMsg (int idx) |
virtual KMMsgBase * | getMsgBase (int idx)=0 |
virtual const KMMsgBase * | getMsgBase (int idx) const =0 |
virtual ChildrenState | hasChildren () const |
virtual void | ignoreJobsForMessage (KMMessage *) |
virtual QString | indexLocation () const =0 |
virtual bool | isCloseToQuota () const |
virtual bool | isMessage (int idx) |
virtual bool | isMoveable () const |
bool | isOpened () const |
virtual bool | isReadOnly () const =0 |
QString | label () const |
QString | location () const |
virtual void | markNewAsUnread () |
virtual void | markUnreadAsRead () |
virtual int | moveMsg (QPtrList< KMMessage >, int *index_return=0) |
virtual int | moveMsg (KMMessage *msg, int *index_return=0) |
virtual void | msgStatusChanged (const KMMsgStatus oldStatus, const KMMsgStatus newStatus, int idx) |
bool | needsCompacting () const |
virtual bool | noChildren () const |
virtual bool | noContent () const |
virtual int | open (const char *owner)=0 |
virtual KMMsgBase * | operator[] (int idx) |
virtual const KMMsgBase * | operator[] (int idx) const |
virtual void | quiet (bool beQuiet) |
virtual void | readConfig () |
virtual KMMessage * | readTemporaryMsg (int idx) |
virtual void | reallyDoClose (const char *owner)=0 |
void | registerWithMessageDict () |
virtual void | remove () |
void | removeJobs () |
virtual void | removeMsg (const QPtrList< KMMessage > &msgList, bool imapQuiet=false) |
virtual void | removeMsg (const QPtrList< KMMsgBase > &msgList, bool imapQuiet=false) |
virtual void | removeMsg (int i, bool imapQuiet=false) |
virtual int | rename (const QString &newName, KMFolderDir *aParent=0) |
virtual void | search (const KMSearchPattern *, Q_UINT32 serNum) |
virtual void | search (const KMSearchPattern *) |
virtual void | setAutoCreateIndex (bool) |
virtual void | setContentsType (KMail::FolderContentsType type, bool quiet=false) |
void | setDirty (bool f) |
virtual void | setHasChildren (ChildrenState state) |
virtual void | setNeedsCompacting (bool f) |
virtual void | setNoChildren (bool aNoChildren) |
virtual void | setNoContent (bool aNoContent) |
virtual void | setStatus (QValueList< int > &ids, KMMsgStatus status, bool toggle=false) |
virtual void | setStatus (int idx, KMMsgStatus status, bool toggle=false) |
virtual void | sync ()=0 |
virtual void | take (QPtrList< KMMessage > msgList) |
virtual KMMessage * | take (int idx) |
virtual KMFolder * | trashFolder () const |
virtual void | tryReleasingFolder (KMFolder *) |
virtual KMMsgInfo * | unGetMsg (int idx) |
virtual void | updateChildrenState () |
virtual void | writeConfig () |
virtual int | writeIndex (bool createEmptyIndex=false)=0 |
virtual | ~FolderStorage () |
Static Public Member Functions | |
static QString | dotEscape (const QString &) |
Protected Types | |
enum | { mDirtyTimerInterval = 600000 } |
Protected Slots | |
virtual void | removeJob (QObject *) |
void | slotProcessNextSearchBatch () |
Protected Member Functions | |
int | appendToFolderIdsFile (int idx=-1) |
virtual void | clearIndex (bool autoDelete=true, bool syncDict=false)=0 |
virtual FolderJob * | doCreateJob (QPtrList< KMMessage > &msgList, const QString &sets, FolderJob::JobType jt, KMFolder *folder) const =0 |
virtual FolderJob * | doCreateJob (KMMessage *msg, FolderJob::JobType jt, KMFolder *folder, QString partSpecifier, const AttachmentStrategy *as) const =0 |
virtual Q_INT64 | doFolderSize () const |
virtual int | expungeContents ()=0 |
virtual void | fillMessageDict () |
void | headerOfMsgChanged (const KMMsgBase *, int idx) |
void | invalidateFolder () |
KMMsgDictREntry * | rDict () const |
void | readFolderIdsFile () |
virtual bool | readIndex ()=0 |
virtual KMMessage * | readMsg (int idx)=0 |
virtual int | removeContents ()=0 |
void | replaceMsgSerNum (unsigned long sernum, KMMsgBase *msg, int idx) |
virtual KMMsgInfo * | setIndexEntry (int idx, KMMessage *msg)=0 |
void | setRDict (KMMsgDictREntry *rentry) const |
virtual KMMsgBase * | takeIndexEntry (int idx)=0 |
int | touchFolderIdsFile () |
virtual void | truncateIndex ()=0 |
int | writeFolderIdsFile () const |
Protected Attributes | |
bool | mAutoCreateIndex:1 |
bool | mChanged:1 |
bool | mCompactable:1 |
KMail::FolderContentsType | mContentsType |
bool | mConvertToUtf8:1 |
int | mCurrentSearchedMsg |
bool | mDirty:1 |
QTimer * | mDirtyTimer |
QTimer * | mEmitChangedTimer |
bool | mExportsSernums:1 |
bool | mFilesLocked:1 |
KMFolder * | mFolder |
int | mGuessedUnreadMsgs |
ChildrenState | mHasChildren |
QPtrList< FolderJob > | mJobList |
bool | mNoChildren:1 |
bool | mNoContent:1 |
int | mOpenCount |
int | mQuiet |
KMMsgDictREntry * | mRDict |
const KMSearchPattern * | mSearchPattern |
Q_INT64 | mSize |
int | mTotalMsgs |
int | mUnreadMsgs |
bool | mWriteConfigEnabled:1 |
bool | needsCompact:1 |
Detailed Description
The FolderStorage class is the bass class for the storage related aspects of a collection of mail (a folder).
Accounts
The accounts (of KMail) that are fed into the folder are represented as the children of the folder. They are only stored here during runtime to have a reference for which accounts point to a specific folder.Definition at line 79 of file folderstorage.h.
Member Enumeration Documentation
anonymous enum [protected] |
Definition at line 118 of file folderstorage.h.
Constructor & Destructor Documentation
FolderStorage::FolderStorage | ( | KMFolder * | folder, | |
const char * | name = 0 | |||
) |
Usually a parent is given.
But in some cases there is no fitting parent object available. Then the name of the folder is used as the absolute path to the folder file.
Definition at line 63 of file folderstorage.cpp.
FolderStorage::~FolderStorage | ( | ) | [virtual] |
Definition at line 93 of file folderstorage.cpp.
Member Function Documentation
KMAccount * FolderStorage::account | ( | ) | const [virtual] |
Reimplemented in KMFolderCachedImap, and KMFolderImap.
Definition at line 1171 of file folderstorage.cpp.
void FolderStorage::addJob | ( | FolderJob * | job | ) | const |
Add job for this folder.
This is done automatically by createJob. This method is public only for other kind of FolderJob like ExpireJob.
Definition at line 119 of file folderstorage.cpp.
int FolderStorage::addMsg | ( | QPtrList< KMMessage > & | msgList, | |
QValueList< int > & | index_return | |||
) | [virtual] |
Adds the given messages to the folder.
Behaviour is identical to addMsg(msg)
Reimplemented in KMFolderImap.
Definition at line 1149 of file folderstorage.cpp.
virtual int FolderStorage::addMsg | ( | KMMessage * | msg, | |
int * | index_return = 0 | |||
) | [pure virtual] |
Add the given message to the folder.
Usually the message is added at the end of the folder. Returns zero on success and an errno error code on failure. The index of the new message is stored in index_return if given. Please note that the message is added as is to the folder and the folder takes ownership of the message (deleting it in the destructor).
Implemented in KMFolderCachedImap, KMFolderImap, KMFolderMaildir, KMFolderMbox, and KMFolderSearch.
virtual int FolderStorage::addMsgKeepUID | ( | KMMessage * | msg, | |
int * | index_return = 0 | |||
) | [inline, virtual] |
(Note(bo): This needs to be fixed better at a later point.
) This is overridden by dIMAP because addMsg strips the X-UID header from the mail.
Reimplemented in KMFolderCachedImap.
Definition at line 197 of file folderstorage.h.
int FolderStorage::appendToFolderIdsFile | ( | int | idx = -1 |
) | [protected] |
Append message to end of message serial number file.
Definition at line 1000 of file folderstorage.cpp.
bool FolderStorage::autoCreateIndex | ( | ) | const [inline] |
Returns TRUE if a table of contents file is automatically created.
Definition at line 314 of file folderstorage.h.
virtual int FolderStorage::canAccess | ( | ) | [pure virtual] |
Check folder for permissions Returns zero if readable and writable.
Implemented in KMFolderMaildir, KMFolderMbox, and KMFolderSearch.
bool FolderStorage::canAddMsgNow | ( | KMMessage * | aMsg, | |
int * | aIndex_ret | |||
) | [virtual] |
Returns FALSE, if the message has to be retrieved from an IMAP account first.
In this case this function does this and cares for the rest
Definition at line 301 of file folderstorage.cpp.
void FolderStorage::changed | ( | ) | [signal] |
Emitted when the status, name, or associated accounts of this folder changed.
void FolderStorage::cleared | ( | ) | [signal] |
Emitted when the contents of a folder have been cleared (new search in a search folder, for example).
virtual void FolderStorage::clearIndex | ( | bool | autoDelete = true , |
|
bool | syncDict = false | |||
) | [protected, pure virtual] |
Implemented in KMFolderIndex, and KMFolderSearch.
void FolderStorage::close | ( | const char * | owner, | |
bool | force = false | |||
) |
Close folder.
open() and close() use reference counting. If force
is true the files are closed regardless of reference count, and the reference count will be set to zero.
Definition at line 102 of file folderstorage.cpp.
void FolderStorage::closed | ( | KMFolder * | ) | [signal] |
Emitted when the folder was closed and ticket owners have to reopen.
virtual int FolderStorage::compact | ( | bool | silent | ) | [pure virtual] |
Remove deleted messages from the folder.
Returns zero on success and an errno on failure. A statusbar message will inform the user that the compaction worked, unless silent
is set.
Implemented in KMFolderImap, KMFolderMaildir, KMFolderMbox, and KMFolderSearch.
bool FolderStorage::compactable | ( | ) | const [inline] |
KMail::FolderContentsType FolderStorage::contentsType | ( | ) | const [inline] |
- Returns:
- the type of contents held in this folder (mail, calendar, etc.)
Definition at line 401 of file folderstorage.h.
void FolderStorage::contentsTypeChanged | ( | KMail::FolderContentsType | type | ) | [signal] |
Emitted when the contents type (calendar, mail, tasks, .
.) of the folderstorage changes.
void FolderStorage::correctUnreadMsgsCount | ( | ) | [virtual] |
A cludge to help make sure the count of unread messges is kept in sync.
Definition at line 944 of file folderstorage.cpp.
int FolderStorage::count | ( | bool | cache = false |
) | const [virtual] |
Number of messages in this folder.
Reimplemented in KMFolderIndex, and KMFolderSearch.
Definition at line 807 of file folderstorage.cpp.
int FolderStorage::countUnread | ( | ) | [virtual] |
virtual int FolderStorage::create | ( | ) | [pure virtual] |
Create a new folder with the name of this object and open it.
Returns zero on success and an error code equal to the c-library fopen call otherwise.
Implemented in KMFolderCachedImap, KMFolderImap, KMFolderMaildir, KMFolderMbox, and KMFolderSearch.
FolderJob * FolderStorage::createJob | ( | QPtrList< KMMessage > & | msgList, | |
const QString & | sets, | |||
FolderJob::JobType | jt = FolderJob::tGetMessage , |
|||
KMFolder * | folder = 0 | |||
) | const [virtual] |
Definition at line 598 of file folderstorage.cpp.
FolderJob * FolderStorage::createJob | ( | KMMessage * | msg, | |
FolderJob::JobType | jt = FolderJob::tGetMessage , |
|||
KMFolder * | folder = 0 , |
|||
QString | partSpecifier = QString::null , |
|||
const AttachmentStrategy * | as = 0 | |||
) | const [virtual] |
These methods create respective FolderJob (You should derive FolderJob for each derived KMFolder).
Definition at line 587 of file folderstorage.cpp.
void FolderStorage::deregisterFromMessageDict | ( | ) |
Triggers deregistration from the message dict, which will cause the dict to ask the FolderStorage to write the relevant data structures to disk.
Definition at line 957 of file folderstorage.cpp.
bool FolderStorage::dirty | ( | ) | const [inline] |
Returns TRUE if the table of contents is dirty.
This happens when a message is deleted from the folder. The toc will then be re-created when the folder is closed.
Definition at line 323 of file folderstorage.h.
virtual FolderJob* FolderStorage::doCreateJob | ( | QPtrList< KMMessage > & | msgList, | |
const QString & | sets, | |||
FolderJob::JobType | jt, | |||
KMFolder * | folder | |||
) | const [protected, pure virtual] |
Implemented in KMFolderCachedImap, KMFolderImap, KMFolderMaildir, KMFolderMbox, and KMFolderSearch.
virtual FolderJob* FolderStorage::doCreateJob | ( | KMMessage * | msg, | |
FolderJob::JobType | jt, | |||
KMFolder * | folder, | |||
QString | partSpecifier, | |||
const AttachmentStrategy * | as | |||
) | const [protected, pure virtual] |
These two methods actually create the jobs.
They have to be implemented in all folders.
- See also:
- createJob
Implemented in KMFolderCachedImap, KMFolderImap, KMFolderMaildir, KMFolderMbox, and KMFolderSearch.
virtual Q_INT64 FolderStorage::doFolderSize | ( | ) | const [inline, protected, virtual] |
void FolderStorage::emitMsgAddedSignals | ( | int | idx | ) |
Called by derived classes implementation of addMsg.
Emits msgAdded signals
Restart always the timer or not. BTW we get a kmheaders refresh each 3 seg.?
Definition at line 284 of file folderstorage.cpp.
int FolderStorage::expunge | ( | ) | [virtual] |
Delete entire folder.
Forces a close *but* opens the folder again afterwards. Returns errno(3) error code or zero on success. see KMFolder::expungeContents
Definition at line 770 of file folderstorage.cpp.
virtual int FolderStorage::expungeContents | ( | ) | [protected, pure virtual] |
Called by KMFolder::expunge() to delete the actual contents.
At the time of the call the folder has already been closed, and the various index files deleted. Returns 0 on success.
Implemented in KMFolderImap, KMFolderMaildir, KMFolderMbox, and KMFolderSearch.
void FolderStorage::expunged | ( | KMFolder * | ) | [signal] |
Emitted after an expunge.
If not quiet, changed() will be emmitted first.
int FolderStorage::expungeOldMsg | ( | int | days | ) | [virtual] |
Delete messages in the folder that are older than days.
Return the number of deleted messages.
Definition at line 254 of file folderstorage.cpp.
QString FolderStorage::fileName | ( | ) | const [virtual] |
Returns the filename of the folder (reimplemented in KMFolderImap).
Reimplemented in KMFolderImap.
Definition at line 143 of file folderstorage.cpp.
virtual void FolderStorage::fillMessageDict | ( | ) | [inline, protected, virtual] |
Inserts messages into the message dictionary.
The messages will get new serial numbers. This is only used on newly appeared folders, where there is no .ids file yet, or when that has been invalidated.
Reimplemented in KMFolderIndex.
Definition at line 545 of file folderstorage.h.
int FolderStorage::find | ( | const KMMessage * | msg | ) | const |
virtual int FolderStorage::find | ( | const KMMsgBase * | msg | ) | const [pure virtual] |
Returns the index of the given message or -1 if not found.
Implemented in KMFolderIndex, and KMFolderSearch.
KMFolder* FolderStorage::folder | ( | void | ) | const [inline] |
Definition at line 92 of file folderstorage.h.
Q_INT64 FolderStorage::folderSize | ( | ) | const |
void FolderStorage::folderSizeChanged | ( | ) | [signal] |
Emitted when the folder's size changes.
virtual KMFolderType FolderStorage::folderType | ( | ) | const [inline, virtual] |
Returns the type of this folder.
Reimplemented in KMFolderCachedImap, KMFolderImap, KMFolderMaildir, KMFolderMbox, and KMFolderSearch.
Definition at line 95 of file folderstorage.h.
virtual DwString FolderStorage::getDwString | ( | int | idx | ) | [pure virtual] |
Read a message and returns a DwString.
Implemented in KMFolderMaildir, KMFolderMbox, and KMFolderSearch.
KMMessage * FolderStorage::getMsg | ( | int | idx | ) | [virtual] |
Read message at given index.
Indexing starts at zero
Reimplemented in KMFolderImap, and KMFolderSearch.
Definition at line 477 of file folderstorage.cpp.
virtual KMMsgBase* FolderStorage::getMsgBase | ( | int | idx | ) | [pure virtual] |
Implemented in KMFolderIndex, and KMFolderSearch.
virtual const KMMsgBase* FolderStorage::getMsgBase | ( | int | idx | ) | const [pure virtual] |
Provides access to the basic message fields that are also stored in the index.
Whenever you only need subject, from, date, status you should use this method instead of getMsg() because getMsg() will load the message if necessary and this method does not.
Implemented in KMFolderIndex, and KMFolderSearch.
virtual ChildrenState FolderStorage::hasChildren | ( | ) | const [inline, virtual] |
Returns if the folder has children, has no children or we don't know.
Definition at line 125 of file folderstorage.h.
void FolderStorage::headerOfMsgChanged | ( | const KMMsgBase * | aMsg, | |
int | idx | |||
) | [protected] |
Tell the folder that a header field that is usually used for the index (subject, from, .
..) has changed of given message. This method is usually called from within KMMessage::setSubject/set...
Definition at line 885 of file folderstorage.cpp.
void FolderStorage::ignoreJobsForMessage | ( | KMMessage * | msg | ) | [virtual] |
Removes and deletes all jobs associated with the particular message.
Reimplemented in KMFolderImap, and KMFolderSearch.
Definition at line 1051 of file folderstorage.cpp.
virtual QString FolderStorage::indexLocation | ( | ) | const [pure virtual] |
void FolderStorage::invalidated | ( | KMFolder * | ) | [signal] |
Emitted when the serial numbers of this folder were invalidated.
void FolderStorage::invalidateFolder | ( | ) | [protected] |
Called when serial numbers for a folder are invalidated, invalidates/recreates data structures dependent on the serial numbers for this folder.
Definition at line 974 of file folderstorage.cpp.
bool FolderStorage::isCloseToQuota | ( | ) | const [virtual] |
Return whether the folder is close to its quota limit, which can be reflected in the UI.
Reimplemented in KMFolderCachedImap.
Definition at line 845 of file folderstorage.cpp.
bool FolderStorage::isMessage | ( | int | idx | ) | [virtual] |
Checks if the message is already "gotten" with getMsg.
Definition at line 578 of file folderstorage.cpp.
bool FolderStorage::isMoveable | ( | ) | const [virtual] |
Returns true if this folder can be moved.
Reimplemented in KMFolderCachedImap, KMFolderImap, and KMFolderSearch.
Definition at line 1164 of file folderstorage.cpp.
bool FolderStorage::isOpened | ( | ) | const [inline] |
Test if folder is opened, i.e.
its reference count is greater than zero.
Definition at line 279 of file folderstorage.h.
virtual bool FolderStorage::isReadOnly | ( | ) | const [pure virtual] |
Is the folder read-only?
Implemented in KMFolderCachedImap, KMFolderImap, KMFolderMaildir, KMFolderMbox, and KMFolderSearch.
QString FolderStorage::label | ( | ) | const |
Returns the label of the folder for visualization.
Definition at line 802 of file folderstorage.cpp.
QString FolderStorage::location | ( | ) | const |
Emitted when the location on disk of the folder changes.
This is used by all code which uses the locatio on disk of the folder storage ( or the cache storage ) as an identifier for the folder.
void FolderStorage::markNewAsUnread | ( | ) | [virtual] |
void FolderStorage::markUnreadAsRead | ( | ) | [virtual] |
Definition at line 627 of file folderstorage.cpp.
int FolderStorage::moveMsg | ( | KMMessage * | msg, | |
int * | index_return = 0 | |||
) | [virtual] |
Detaches the given message from it's current folder and adds it to this folder.
Returns zero on success and an errno error code on failure. The index of the new message is stored in index_return if given.
Definition at line 608 of file folderstorage.cpp.
void FolderStorage::msgAdded | ( | KMFolder * | , | |
Q_UINT32 | sernum | |||
) | [signal] |
void FolderStorage::msgAdded | ( | int | idx | ) | [signal] |
Emitted when a message is added from the folder.
void FolderStorage::msgChanged | ( | KMFolder * | , | |
Q_UINT32 | sernum, | |||
int | delta | |||
) | [signal] |
Emitted, when the status of a message is changed.
void FolderStorage::msgHeaderChanged | ( | KMFolder * | , | |
int | ||||
) | [signal] |
Emitted when a field of the header of a specific message changed.
void FolderStorage::msgRemoved | ( | KMFolder * | ) | [signal] |
void FolderStorage::msgRemoved | ( | int | idx, | |
QString | msgIdMD5 | |||
) | [signal] |
Emitted after a message is removed from the folder.
void FolderStorage::msgRemoved | ( | KMFolder * | , | |
Q_UINT32 | sernum | |||
) | [signal] |
Emitted before a message is removed from the folder.
void FolderStorage::msgStatusChanged | ( | const KMMsgStatus | oldStatus, | |
const KMMsgStatus | newStatus, | |||
int | idx | |||
) | [virtual] |
Called by KMMsgBase::setStatus when status of a message has changed required to keep the number unread messages variable current.
Reimplemented in KMFolderMaildir.
Definition at line 851 of file folderstorage.cpp.
void FolderStorage::nameChanged | ( | ) | [signal] |
Emitted when the name of the folder changes.
bool FolderStorage::needsCompacting | ( | ) | const [inline] |
Returns TRUE if the folder contains deleted messages.
Definition at line 329 of file folderstorage.h.
virtual bool FolderStorage::noChildren | ( | ) | const [inline, virtual] |
virtual bool FolderStorage::noContent | ( | ) | const [inline, virtual] |
Returns, if the folder can't contain mails, but only subfolder.
Definition at line 106 of file folderstorage.h.
void FolderStorage::numUnreadMsgsChanged | ( | KMFolder * | ) | [signal] |
Emitted when number of unread messages has changed.
virtual int FolderStorage::open | ( | const char * | owner | ) | [pure virtual] |
Open folder for access.
open() and close() use reference counting. Returns zero on success and an error code equal to the c-library fopen call otherwise (errno).
- See also:
- KMFolderOpener
Implemented in KMFolderMaildir, KMFolderMbox, and KMFolderSearch.
virtual KMMsgBase* FolderStorage::operator[] | ( | int | idx | ) | [inline, virtual] |
virtual const KMMsgBase* FolderStorage::operator[] | ( | int | idx | ) | const [inline, virtual] |
void FolderStorage::quiet | ( | bool | beQuiet | ) | [virtual] |
If set to quiet the folder will not emit msgAdded(idx) signal.
This is necessary because adding the messages to the listview one by one as they come in ( as happens on msgAdded(idx) ) is very slow for large ( >10000 ) folders. For pop, where whole bodies are downloaded this is not an issue, but for imap, where we only download headers it becomes a bottleneck. We therefore set the folder quiet() and rebuild the listview completely once the complete folder has been checked.
Definition at line 205 of file folderstorage.cpp.
KMMsgDictREntry* FolderStorage::rDict | ( | ) | const [inline, protected] |
void FolderStorage::readConfig | ( | void | ) | [virtual] |
Read the config file.
Reimplemented in KMFolderCachedImap, and KMFolderImap.
Definition at line 905 of file folderstorage.cpp.
void FolderStorage::readFolderIdsFile | ( | ) | [protected] |
Read the on-disk cache of serial numbers of messages in this store and fill those into the global message dict, such that the messages we hold can be looked up there.
Definition at line 963 of file folderstorage.cpp.
virtual bool FolderStorage::readIndex | ( | ) | [protected, pure virtual] |
Read index file and fill the message-info list mMsgList.
Implemented in KMFolderIndex, and KMFolderSearch.
virtual KMMessage* FolderStorage::readMsg | ( | int | idx | ) | [protected, pure virtual] |
Load message from file and store it at given index.
Returns 0 on failure.
Implemented in KMFolderMaildir, KMFolderMbox, and KMFolderSearch.
void FolderStorage::readOnlyChanged | ( | KMFolder * | ) | [signal] |
Emitted when the readonly status of the folder changes.
KMMessage * FolderStorage::readTemporaryMsg | ( | int | idx | ) | [virtual] |
Load message from file and do NOT store it, only return it.
This is equivalent to, but faster than, getMsg+unGetMsg WARNING: the caller has to delete the returned value!
Definition at line 523 of file folderstorage.cpp.
void FolderStorage::reallyAddCopyOfMsg | ( | KMMessage * | aMsg | ) | [virtual, slot] |
Add a copy of the message to the folder after it has been retrieved from an IMAP server.
Definition at line 344 of file folderstorage.cpp.
void FolderStorage::reallyAddMsg | ( | KMMessage * | aMsg | ) | [virtual, slot] |
Add the message to the folder after it has been retrieved from an IMAP server.
Definition at line 323 of file folderstorage.cpp.
virtual void FolderStorage::reallyDoClose | ( | const char * | owner | ) | [pure virtual] |
Implemented in KMFolderCachedImap, KMFolderImap, KMFolderMaildir, KMFolderMbox, and KMFolderSearch.
void FolderStorage::registerWithMessageDict | ( | ) |
Triggers registration with the message dict, which will cause the dict to ask the FolderStorage to fill itself into it.
Definition at line 951 of file folderstorage.cpp.
void FolderStorage::remove | ( | ) | [virtual] |
Removes the folder physically from disk and empties the contents of the folder in memory.
Note that the folder is closed during this process, whether there are others using it or not.
- See also:
- KMFolder::removeContents
Reimplemented in KMFolderCachedImap, and KMFolderImap.
Definition at line 742 of file folderstorage.cpp.
virtual int FolderStorage::removeContents | ( | ) | [protected, pure virtual] |
Called by KMFolder::remove() to delete the actual contents.
At the time of the call the folder has already been closed, and the various index files deleted. Returns 0 on success.
Implemented in KMFolderMaildir, KMFolderMbox, and KMFolderSearch.
void FolderStorage::removed | ( | KMFolder * | , | |
bool | ||||
) | [signal] |
Emitted when a folder was removed.
void FolderStorage::removeJob | ( | QObject * | job | ) | [protected, virtual, slot] |
Definition at line 126 of file folderstorage.cpp.
void FolderStorage::removeJobs | ( | ) |
Definition at line 1073 of file folderstorage.cpp.
void FolderStorage::removeMsg | ( | const QPtrList< KMMsgBase > & | msgList, | |
bool | imapQuiet = false | |||
) | [virtual] |
Definition at line 358 of file folderstorage.cpp.
void FolderStorage::removeMsg | ( | int | i, | |
bool | imapQuiet = false | |||
) | [virtual] |
Remove (first occurrence of) given message from the folder.
Reimplemented in KMFolderCachedImap, KMFolderImap, and KMFolderMaildir.
Definition at line 380 of file folderstorage.cpp.
int FolderStorage::rename | ( | const QString & | newName, | |
KMFolderDir * | aParent = 0 | |||
) | [virtual] |
Physically rename the folder.
Returns zero on success and an errno on failure.
Reimplemented in KMFolderCachedImap, and KMFolderImap.
Definition at line 652 of file folderstorage.cpp.
void FolderStorage::replaceMsgSerNum | ( | unsigned long | sernum, | |
KMMsgBase * | msg, | |||
int | idx | |||
) | [protected] |
Replaces the serial number for the message msg
at index idx
with sernum
.
Definition at line 1012 of file folderstorage.cpp.
void FolderStorage::search | ( | const KMSearchPattern * | pattern, | |
Q_UINT32 | serNum | |||
) | [virtual] |
Check if the message matches the search criteria The end is signaled with searchDone().
Reimplemented in KMFolderImap.
Definition at line 1141 of file folderstorage.cpp.
void FolderStorage::search | ( | const KMSearchPattern * | pattern | ) | [virtual] |
Search for messages The end is signaled with searchDone().
Reimplemented in KMFolderImap.
Definition at line 1113 of file folderstorage.cpp.
void FolderStorage::searchDone | ( | KMFolder * | , | |
Q_UINT32 | , | |||
const KMSearchPattern * | , | |||
bool | ||||
) | [signal] |
Emitted when a search for a single message is completed The serial number and a bool matching yes/no is included.
void FolderStorage::searchResult | ( | KMFolder * | , | |
QValueList< Q_UINT32 > | , | |||
const KMSearchPattern * | , | |||
bool | complete | |||
) | [signal] |
Emitted when a search delivers results The matching serial numbers are included If complete
is true the search is done.
void FolderStorage::setAutoCreateIndex | ( | bool | autoIndex | ) | [virtual] |
Allow/disallow automatic creation of a table of contents file.
Default is TRUE.
Definition at line 151 of file folderstorage.cpp.
void FolderStorage::setContentsType | ( | KMail::FolderContentsType | type, | |
bool | quiet = false | |||
) | [virtual] |
Set the type of contents held in this folder (mail, calendar, etc.).
Definition at line 1103 of file folderstorage.cpp.
void FolderStorage::setDirty | ( | bool | f | ) |
virtual void FolderStorage::setHasChildren | ( | ChildrenState | state | ) | [inline, virtual] |
virtual KMMsgInfo* FolderStorage::setIndexEntry | ( | int | idx, | |
KMMessage * | msg | |||
) | [protected, pure virtual] |
Implemented in KMFolderIndex, and KMFolderSearch.
virtual void FolderStorage::setNeedsCompacting | ( | bool | f | ) | [inline, virtual] |
Definition at line 330 of file folderstorage.h.
void FolderStorage::setNoChildren | ( | bool | aNoChildren | ) | [virtual] |
virtual void FolderStorage::setNoContent | ( | bool | aNoContent | ) | [inline, virtual] |
void FolderStorage::setRDict | ( | KMMsgDictREntry * | rentry | ) | const [protected] |
Sets the reverse-dictionary for this folder.
const, because the mRDict is mutable, since it is not part of the (conceptually) const-relevant state of the object.
Definition at line 1018 of file folderstorage.cpp.
void FolderStorage::setStatus | ( | QValueList< int > & | ids, | |
KMMsgStatus | status, | |||
bool | toggle = false | |||
) | [virtual] |
Set the status of the message(s) in the QValueList ids
to status
.
Reimplemented in KMFolderCachedImap, and KMFolderImap.
Definition at line 1043 of file folderstorage.cpp.
void FolderStorage::setStatus | ( | int | idx, | |
KMMsgStatus | status, | |||
bool | toggle = false | |||
) | [virtual] |
Set the status of the message at index idx
to status
.
Reimplemented in KMFolderCachedImap, and KMFolderImap.
Definition at line 1030 of file folderstorage.cpp.
void FolderStorage::slotEmitChangedTimer | ( | ) | [slot] |
void FolderStorage::slotProcessNextSearchBatch | ( | ) | [protected, slot] |
void FolderStorage::statusMsg | ( | const QString & | ) | [signal] |
Emmited to display a message somewhere in a status line.
virtual void FolderStorage::sync | ( | ) | [pure virtual] |
KMMessage * FolderStorage::take | ( | int | idx | ) | [virtual] |
Detach message from this folder.
Usable to call addMsg() afterwards. Loads the message if it is not loaded up to now.
Reimplemented in KMFolderCachedImap, KMFolderImap, and KMFolderMaildir.
Definition at line 423 of file folderstorage.cpp.
virtual KMMsgBase* FolderStorage::takeIndexEntry | ( | int | idx | ) | [protected, pure virtual] |
Implemented in KMFolderIndex, and KMFolderSearch.
int FolderStorage::touchFolderIdsFile | ( | ) | [protected] |
virtual KMFolder* FolderStorage::trashFolder | ( | ) | const [inline, virtual] |
If this folder has a special trash folder set, return it.
Otherwise return 0.
Reimplemented in KMFolderCachedImap, and KMFolderImap.
Definition at line 384 of file folderstorage.h.
virtual void FolderStorage::truncateIndex | ( | ) | [protected, pure virtual] |
Implemented in KMFolderIndex, and KMFolderSearch.
virtual void FolderStorage::tryReleasingFolder | ( | KMFolder * | ) | [inline, virtual] |
Try releasing folder
if possible, something is attempting an exclusive access to it.
Currently used for KMFolderSearch and the background tasks like expiry.
Reimplemented in KMFolderSearch.
Definition at line 273 of file folderstorage.h.
KMMsgInfo * FolderStorage::unGetMsg | ( | int | idx | ) | [virtual] |
Replace KMMessage with KMMsgInfo and delete KMMessage.
Definition at line 553 of file folderstorage.cpp.
void FolderStorage::updateChildrenState | ( | ) | [virtual] |
virtual int FolderStorage::updateIndex | ( | ) | [pure virtual, slot] |
Incrementally update the index if possible else call writeIndex.
Implemented in KMFolderIndex, and KMFolderSearch.
void FolderStorage::writeConfig | ( | ) | [virtual] |
Write the config file.
Reimplemented in KMFolderCachedImap, and KMFolderImap.
Definition at line 926 of file folderstorage.cpp.
int FolderStorage::writeFolderIdsFile | ( | ) | const [protected] |
virtual int FolderStorage::writeIndex | ( | bool | createEmptyIndex = false |
) | [pure virtual] |
Write index to index-file.
Returns 0 on success and errno error on failure.
Implemented in KMFolderIndex, and KMFolderSearch.
Member Data Documentation
bool FolderStorage::mAutoCreateIndex [protected] |
bool FolderStorage::mChanged [protected] |
Definition at line 600 of file folderstorage.h.
bool FolderStorage::mCompactable [protected] |
bool FolderStorage::mConvertToUtf8 [protected] |
Definition at line 622 of file folderstorage.h.
int FolderStorage::mCurrentSearchedMsg [protected] |
Definition at line 641 of file folderstorage.h.
bool FolderStorage::mDirty [protected] |
if the index is dirty it will be recreated upon close()
Definition at line 607 of file folderstorage.h.
QTimer* FolderStorage::mDirtyTimer [protected] |
Definition at line 629 of file folderstorage.h.
QTimer* FolderStorage::mEmitChangedTimer [protected] |
Definition at line 639 of file folderstorage.h.
bool FolderStorage::mExportsSernums [protected] |
Has this storage exported its serial numbers to the global message dict for lookup?
Definition at line 605 of file folderstorage.h.
bool FolderStorage::mFilesLocked [protected] |
TRUE if the files of the folder are locked (writable).
Definition at line 609 of file folderstorage.h.
KMFolder* FolderStorage::mFolder [protected] |
Definition at line 637 of file folderstorage.h.
int FolderStorage::mGuessedUnreadMsgs [protected] |
Definition at line 612 of file folderstorage.h.
ChildrenState FolderStorage::mHasChildren [protected] |
Definition at line 632 of file folderstorage.h.
QPtrList<FolderJob> FolderStorage::mJobList [mutable, protected] |
bool FolderStorage::mNoChildren [protected] |
Definition at line 621 of file folderstorage.h.
bool FolderStorage::mNoContent [protected] |
Definition at line 620 of file folderstorage.h.
int FolderStorage::mOpenCount [protected] |
Definition at line 596 of file folderstorage.h.
int FolderStorage::mQuiet [protected] |
Definition at line 599 of file folderstorage.h.
KMMsgDictREntry* FolderStorage::mRDict [mutable, protected] |
const KMSearchPattern* FolderStorage::mSearchPattern [protected] |
Definition at line 642 of file folderstorage.h.
Q_INT64 FolderStorage::mSize [protected] |
Definition at line 614 of file folderstorage.h.
int FolderStorage::mTotalMsgs [protected] |
Definition at line 613 of file folderstorage.h.
int FolderStorage::mUnreadMsgs [protected] |
bool FolderStorage::mWriteConfigEnabled [protected] |
Definition at line 615 of file folderstorage.h.
bool FolderStorage::needsCompact [protected] |
sven: true if on destruct folder needs to be compacted.
Definition at line 617 of file folderstorage.h.
The documentation for this class was generated from the following files: