kmail
KMAccount Class Reference
#include <kmaccount.h>
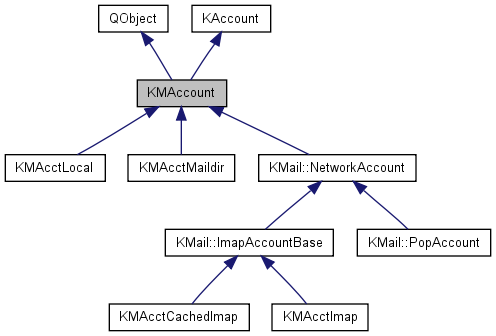
Public Types | |
enum | CheckStatus { CheckOK, CheckIgnored, CheckCanceled, CheckAborted, CheckError } |
Signals | |
virtual void | finishedCheck (bool newMail, CheckStatus status) |
virtual void | newMailsProcessed (const QMap< QString, int > &newInFolder) |
Public Member Functions | |
virtual void | cancelMailCheck () |
void | checkDone (bool newMail, CheckStatus status) |
bool | checkExclude (void) const |
bool | checkingMail () |
int | checkInterval () const |
virtual void | clearPasswd () |
int | defaultCheckInterval (void) const |
void | deleteFolderJobs () |
KMFolder * | folder (void) const |
bool | hasInbox () const |
uint | identityId () const |
virtual void | ignoreJobsForMessage (KMMessage *) |
virtual void | init () |
virtual void | invalidateIMAPFolders () |
virtual bool | mailCheckCanProceed () const |
ProgressItem * | mailCheckProgressItem () const |
virtual QString | name () const |
const QString & | precommand (void) const |
virtual void | processNewMail (bool interactive)=0 |
virtual void | pseudoAssign (const KMAccount *a) |
virtual void | readConfig (KConfig &config) |
void | readTimerConfig () |
bool | runPrecommand (const QString &precommand) |
virtual void | setCheckExclude (bool aExclude) |
virtual void | setCheckingMail (bool checking) |
virtual void | setCheckInterval (int aInterval) |
virtual void | setFolder (KMFolder *, bool addAccount=false) |
virtual void | setHasInbox (bool has) |
void | setIdentityId (uint identityId) |
virtual void | setName (const QString &) |
virtual void | setPrecommand (const QString &cmd) |
virtual void | setTrash (const QString &aTrash) |
QString | trash () const |
virtual QString | type () const |
virtual void | writeConfig (KConfig &config) |
virtual | ~KMAccount () |
Static Public Member Functions | |
static QString | decryptStr (const QString &inStr) |
static QString | encryptStr (const QString &inStr) |
static QString | importPassword (const QString &) |
Static Public Attributes | |
static const int | DefaultCheckInterval = 5 |
Protected Slots | |
virtual void | mailCheck () |
virtual void | precommandExited (bool) |
virtual void | sendReceipts () |
Protected Member Functions | |
void | addToNewInFolder (QString folderId, int num) |
virtual void | deinstallTimer () |
virtual void | installTimer () |
KMAccount (AccountManager *owner, const QString &accountName, uint id) | |
virtual bool | processNewMsg (KMMessage *msg) |
virtual void | sendReceipt (KMMessage *msg) |
Protected Attributes | |
bool | mCheckingMail: 1 |
bool | mExclude |
QGuardedPtr< KMAcctFolder > | mFolder |
bool | mHasInbox: 1 |
uint | mIdentityId |
int | mInterval |
QPtrList< FolderJob > | mJobList |
QGuardedPtr< ProgressItem > | mMailCheckProgressItem |
AccountManager * | mOwner |
QString | mPrecommand |
bool | mPrecommandSuccess |
QValueList< KMMessage * > | mReceipts |
QTimer * | mTimer |
QString | mTrash |
Detailed Description
Definition at line 73 of file kmaccount.h.
Member Enumeration Documentation
Definition at line 84 of file kmaccount.h.
Constructor & Destructor Documentation
KMAccount::~KMAccount | ( | ) | [virtual] |
Definition at line 111 of file kmaccount.cpp.
KMAccount::KMAccount | ( | AccountManager * | owner, | |
const QString & | accountName, | |||
uint | id | |||
) | [protected] |
Definition at line 86 of file kmaccount.cpp.
Member Function Documentation
void KMAccount::addToNewInFolder | ( | QString | folderId, | |
int | num | |||
) | [protected] |
Call this to increase the number of new messages in a folder for messages which are _not_ processed with processNewMsg().
- Parameters:
-
folderId the id of the folder num the number of new message in this folder
Definition at line 494 of file kmaccount.cpp.
virtual void KMAccount::cancelMailCheck | ( | ) | [inline, virtual] |
Abort all running mail checks.
Used when closing the last KMMainWin. Ensure that mail check can be restarted later, e.g. if reopening a mainwindow from a composer window.
Reimplemented in KMail::ImapAccountBase, KMAcctCachedImap, and KMAcctImap.
Definition at line 239 of file kmaccount.h.
void KMAccount::checkDone | ( | bool | newMail, | |
CheckStatus | status | |||
) |
Call this if the newmail-check ended.
- Parameters:
-
newMail true if new mail arrived status the status of the mail check
Definition at line 473 of file kmaccount.cpp.
bool KMAccount::checkExclude | ( | void | ) | const [inline] |
Definition at line 179 of file kmaccount.h.
bool KMAccount::checkingMail | ( | ) | [inline] |
Set/Get if this account is currently checking mail.
Reimplemented in KMail::ImapAccountBase.
Definition at line 224 of file kmaccount.h.
int KMAccount::checkInterval | ( | ) | const |
Definition at line 311 of file kmaccount.cpp.
void KMAccount::clearPasswd | ( | ) | [virtual] |
Set password to "" (empty string).
Reimplemented in KMail::NetworkAccount.
Definition at line 126 of file kmaccount.cpp.
Definition at line 200 of file kmaccount.h.
int KMAccount::defaultCheckInterval | ( | void | ) | const [inline] |
This can be used to provide a more complex calculation later if we want.
Definition at line 163 of file kmaccount.h.
void KMAccount::deinstallTimer | ( | ) | [protected, virtual] |
Definition at line 365 of file kmaccount.cpp.
void KMAccount::deleteFolderJobs | ( | ) |
Deletes the set of FolderJob associated with this account.
Definition at line 319 of file kmaccount.cpp.
Very primitive en/de-cryption so that the password is not readable in the config file.
But still very easy breakable.
Definition at line 424 of file kmaccount.cpp.
virtual void KMAccount::finishedCheck | ( | bool | newMail, | |
CheckStatus | status | |||
) | [virtual, signal] |
Emitted after the mail check is finished.
- Parameters:
-
newMail true if there was new mail status the status of the mail check
KMFolder* KMAccount::folder | ( | void | ) | const [inline] |
There can be exactly one folder that is fed by messages from an account.
Definition at line 123 of file kmaccount.h.
bool KMAccount::hasInbox | ( | ) | const [inline] |
uint KMAccount::identityId | ( | ) | const [inline] |
Definition at line 253 of file kmaccount.h.
void KMAccount::ignoreJobsForMessage | ( | KMMessage * | msg | ) | [virtual] |
delete jobs associated with this message
Reimplemented in KMAcctImap.
Definition at line 327 of file kmaccount.cpp.
Definition at line 436 of file kmaccount.cpp.
void KMAccount::init | ( | void | ) | [virtual] |
Set intelligent default values to the fields of the account.
Reimplemented in KMail::ImapAccountBase, KMAcctCachedImap, KMAcctLocal, KMAcctMaildir, KMail::NetworkAccount, and KMail::PopAccount.
Definition at line 103 of file kmaccount.cpp.
void KMAccount::installTimer | ( | ) | [protected, virtual] |
void KMAccount::invalidateIMAPFolders | ( | ) | [virtual] |
If this account is a disconnected IMAP account, invalidate it.
Reimplemented in KMAcctCachedImap.
Definition at line 454 of file kmaccount.cpp.
void KMAccount::mailCheck | ( | ) | [protected, virtual, slot] |
Definition at line 402 of file kmaccount.cpp.
virtual bool KMAccount::mailCheckCanProceed | ( | ) | const [inline, virtual] |
Determines whether the account can be checked, currently.
Reimplementations can use this to prevent mailchecks due to exceeded connection limits, or because a network link iis down.
- Returns:
- whether mail checks can proceed
Reimplemented in KMail::NetworkAccount.
Definition at line 219 of file kmaccount.h.
ProgressItem* KMAccount::mailCheckProgressItem | ( | ) | const [inline] |
Call ->progress( int foo ) on this to update the account's progress indicators.
Definition at line 245 of file kmaccount.h.
virtual QString KMAccount::name | ( | ) | const [inline, virtual] |
Account name (reimpl because of ambiguous QObject::name()).
Reimplemented from QObject.
Definition at line 103 of file kmaccount.h.
virtual void KMAccount::newMailsProcessed | ( | const QMap< QString, int > & | newInFolder | ) | [virtual, signal] |
Emitted after the mail check is finished.
- Parameters:
-
newInFolder number of new messages for each folder
const QString& KMAccount::precommand | ( | void | ) | const [inline] |
void KMAccount::precommandExited | ( | bool | success | ) | [protected, virtual, slot] |
Definition at line 395 of file kmaccount.cpp.
virtual void KMAccount::processNewMail | ( | bool | interactive | ) | [pure virtual] |
Process new mail for this account if one arrived.
Returns TRUE if new mail has been found. Whether the mail is automatically loaded to an associated folder or not depends on the type of the account.
Implemented in KMAcctCachedImap, KMAcctImap, KMAcctLocal, KMAcctMaildir, and KMail::PopAccount.
bool KMAccount::processNewMsg | ( | KMMessage * | msg | ) | [protected, virtual] |
Does filtering and storing in a folder for the given message.
Shall be called from within processNewMail() to process the new messages. Returns false if failed to add new message.
Definition at line 216 of file kmaccount.cpp.
void KMAccount::pseudoAssign | ( | const KMAccount * | a | ) | [virtual] |
A weak assignment operator.
Reimplemented in KMail::ImapAccountBase, KMAcctCachedImap, KMAcctImap, KMAcctLocal, KMAcctMaildir, KMail::NetworkAccount, and KMail::PopAccount.
Definition at line 459 of file kmaccount.cpp.
void KMAccount::readConfig | ( | KConfig & | config | ) | [virtual] |
Read config file entries.
This method is called by the account manager when a new account is created. The config group is already properly set by the caller.
Reimplemented in KMail::ImapAccountBase, KMAcctCachedImap, KMAcctImap, KMAcctLocal, KMAcctMaildir, KMail::NetworkAccount, and KMail::PopAccount.
Definition at line 145 of file kmaccount.cpp.
void KMAccount::readTimerConfig | ( | ) |
Definition at line 166 of file kmaccount.cpp.
bool KMAccount::runPrecommand | ( | const QString & | precommand | ) |
Runs the precommand.
If the precommand is empty, the method will just return success and not actually do anything
- Returns:
- True if successful, false otherwise
Definition at line 372 of file kmaccount.cpp.
void KMAccount::sendReceipt | ( | KMMessage * | msg | ) | [protected, virtual] |
Send receipt of message back to sender (confirming delivery).
Checks the config settings, calls
- See also:
- KMMessage::createDeliveryReceipt and queues the resulting message in
mReceipts
.
Definition at line 197 of file kmaccount.cpp.
void KMAccount::sendReceipts | ( | ) | [protected, virtual, slot] |
Definition at line 415 of file kmaccount.cpp.
void KMAccount::setCheckExclude | ( | bool | aExclude | ) | [virtual] |
Set/get whether account should be part of the accounts checked with "Check Mail".
Definition at line 341 of file kmaccount.cpp.
virtual void KMAccount::setCheckingMail | ( | bool | checking | ) | [inline, virtual] |
void KMAccount::setCheckInterval | ( | int | aInterval | ) | [virtual] |
Set/get interval for checking if new mail arrived (in minutes).
An interval of zero (or less) disables the automatic checking.
Definition at line 302 of file kmaccount.cpp.
void KMAccount::setFolder | ( | KMFolder * | aFolder, | |
bool | addAccount = false | |||
) | [virtual] |
virtual void KMAccount::setHasInbox | ( | bool | has | ) | [inline, virtual] |
Definition at line 206 of file kmaccount.h.
void KMAccount::setIdentityId | ( | uint | identityId | ) | [inline] |
void KMAccount::setName | ( | const QString & | aName | ) | [virtual] |
virtual void KMAccount::setPrecommand | ( | const QString & | cmd | ) | [inline, virtual] |
Definition at line 185 of file kmaccount.h.
virtual void KMAccount::setTrash | ( | const QString & | aTrash | ) | [inline, virtual] |
Definition at line 130 of file kmaccount.h.
QString KMAccount::trash | ( | ) | const [inline] |
virtual QString KMAccount::type | ( | void | ) | const [inline, virtual] |
Returns type of the account.
Reimplemented in KMAcctCachedImap, KMAcctImap, KMAcctLocal, KMAcctMaildir, and KMail::PopAccount.
Definition at line 93 of file kmaccount.h.
void KMAccount::writeConfig | ( | KConfig & | config | ) | [virtual] |
Write all account information to given config file.
The config group is already properly set by the caller.
Reimplemented in KMail::ImapAccountBase, KMAcctCachedImap, KMAcctLocal, KMAcctMaildir, KMail::NetworkAccount, and KMail::PopAccount.
Definition at line 178 of file kmaccount.cpp.
Member Data Documentation
const int KMAccount::DefaultCheckInterval = 5 [static] |
bool KMAccount::mCheckingMail [protected] |
Definition at line 318 of file kmaccount.h.
bool KMAccount::mExclude [protected] |
Definition at line 317 of file kmaccount.h.
QGuardedPtr<KMAcctFolder> KMAccount::mFolder [protected] |
bool KMAccount::mHasInbox [protected] |
Definition at line 322 of file kmaccount.h.
uint KMAccount::mIdentityId [protected] |
Definition at line 324 of file kmaccount.h.
int KMAccount::mInterval [protected] |
Definition at line 312 of file kmaccount.h.
QPtrList<FolderJob> KMAccount::mJobList [protected] |
QGuardedPtr<ProgressItem> KMAccount::mMailCheckProgressItem [protected] |
Definition at line 323 of file kmaccount.h.
AccountManager* KMAccount::mOwner [protected] |
Definition at line 309 of file kmaccount.h.
QString KMAccount::mPrecommand [protected] |
Definition at line 307 of file kmaccount.h.
bool KMAccount::mPrecommandSuccess [protected] |
Definition at line 319 of file kmaccount.h.
QValueList<KMMessage*> KMAccount::mReceipts [protected] |
Definition at line 320 of file kmaccount.h.
QTimer* KMAccount::mTimer [protected] |
Definition at line 311 of file kmaccount.h.
QString KMAccount::mTrash [protected] |
Definition at line 308 of file kmaccount.h.
The documentation for this class was generated from the following files: