kmail
KMail::NetworkAccount Class Reference
#include <networkaccount.h>
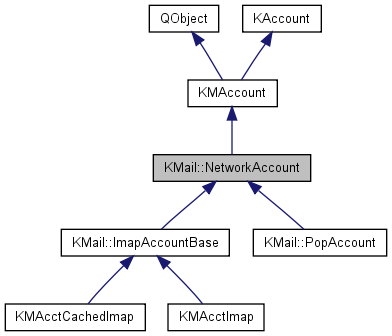
Public Member Functions | |
QString | auth () const |
virtual void | clearPasswd () |
virtual KURL | getUrl () const |
QString | host () const |
virtual void | init () |
virtual void | killAllJobs (bool disconnectSlave=false)=0 |
QString | login () const |
virtual bool | mailCheckCanProceed () const |
QString | passwd () const |
unsigned short int | port () const |
virtual void | pseudoAssign (const KMAccount *a) |
virtual void | readConfig (KConfig &config) |
void | readPassword () |
virtual void | setAuth (const QString &auth) |
virtual void | setCheckingMail (bool checking) |
virtual void | setHost (const QString &host) |
virtual void | setLogin (const QString &login) |
virtual void | setPasswd (const QString &passwd, bool storeInConfig=false) |
virtual void | setPort (unsigned short int port) |
virtual void | setSieveConfig (const KMail::SieveConfig &config) |
virtual void | setStorePasswd (bool store) |
virtual void | setUseSSL (bool use) |
virtual void | setUseTLS (bool use) |
KMail::SieveConfig | sieveConfig () const |
KIO::Slave * | slave () const |
virtual KIO::MetaData | slaveConfig () const |
bool | storePasswd () const |
bool | useSSL () const |
bool | useTLS () const |
virtual void | writeConfig (KConfig &config) |
virtual | ~NetworkAccount () |
Static Public Member Functions | |
static void | resetConnectionList (NetworkAccount *acct) |
Protected Member Functions | |
virtual unsigned short int | defaultPort () const =0 |
NetworkAccount (AccountManager *parent, const QString &name, uint id) | |
virtual QString | protocol () const =0 |
Protected Attributes | |
bool | mAskAgain: 1 |
QString | mAuth |
QString | mHost |
QString | mLogin |
QString | mPasswd |
bool | mPasswdDirty |
unsigned short int | mPort |
KMail::SieveConfig | mSieveConfig |
QGuardedPtr< KIO::Slave > | mSlave |
bool | mStorePasswd: 1 |
bool | mStorePasswdInConfig |
bool | mUseSSL: 1 |
bool | mUseTLS: 1 |
Detailed Description
Definition at line 46 of file networkaccount.h.
Constructor & Destructor Documentation
KMail::NetworkAccount::NetworkAccount | ( | AccountManager * | parent, | |
const QString & | name, | |||
uint | id | |||
) | [protected] |
Definition at line 52 of file networkaccount.cpp.
KMail::NetworkAccount::~NetworkAccount | ( | ) | [virtual] |
Definition at line 67 of file networkaccount.cpp.
Member Function Documentation
QString KMail::NetworkAccount::auth | ( | ) | const [inline] |
void KMail::NetworkAccount::clearPasswd | ( | ) | [virtual] |
Set the password to "" (empty string).
Reimplemented from KMAccount.
Definition at line 110 of file networkaccount.cpp.
virtual unsigned short int KMail::NetworkAccount::defaultPort | ( | ) | const [protected, pure virtual] |
Implemented in KMail::ImapAccountBase, and KMail::PopAccount.
KURL KMail::NetworkAccount::getUrl | ( | ) | const [virtual] |
QString KMail::NetworkAccount::host | ( | ) | const [inline] |
void KMail::NetworkAccount::init | ( | void | ) | [virtual] |
Set the config options to a decent state.
Reimplemented from KMAccount.
Reimplemented in KMail::ImapAccountBase, KMAcctCachedImap, and KMail::PopAccount.
Definition at line 71 of file networkaccount.cpp.
virtual void KMail::NetworkAccount::killAllJobs | ( | bool | disconnectSlave = false |
) | [pure virtual] |
Kill all jobs that are currently in progress.
Implemented in KMail::ImapAccountBase, KMAcctCachedImap, KMAcctImap, and KMail::PopAccount.
QString KMail::NetworkAccount::login | ( | ) | const [inline] |
bool KMail::NetworkAccount::mailCheckCanProceed | ( | ) | const [virtual] |
Determines whether the account can be checked, currently.
Reimplementations can use this to prevent mailchecks due to exceeded connection limits, or because a network link iis down.
- Returns:
- whether mail checks can proceed
Reimplemented from KMAccount.
Definition at line 341 of file networkaccount.cpp.
QString KMail::NetworkAccount::passwd | ( | ) | const |
unsigned short int KMail::NetworkAccount::port | ( | ) | const [inline] |
virtual QString KMail::NetworkAccount::protocol | ( | ) | const [protected, pure virtual] |
Implemented in KMail::ImapAccountBase, and KMail::PopAccount.
void KMail::NetworkAccount::pseudoAssign | ( | const KMAccount * | a | ) | [virtual] |
A weak assignment operator.
Reimplemented from KMAccount.
Reimplemented in KMail::ImapAccountBase, KMAcctCachedImap, KMAcctImap, and KMail::PopAccount.
Definition at line 276 of file networkaccount.cpp.
void KMail::NetworkAccount::readConfig | ( | KConfig & | config | ) | [virtual] |
Read config file entries.
This method is called by the account manager when a new account is created. The config group is already properly set by the caller.
Reimplemented from KMAccount.
Reimplemented in KMail::ImapAccountBase, KMAcctCachedImap, KMAcctImap, and KMail::PopAccount.
Definition at line 150 of file networkaccount.cpp.
void KMail::NetworkAccount::readPassword | ( | ) |
Read password from wallet, used for on-demand wallet opening.
Definition at line 292 of file networkaccount.cpp.
void KMail::NetworkAccount::resetConnectionList | ( | NetworkAccount * | acct | ) | [static] |
void KMail::NetworkAccount::setAuth | ( | const QString & | auth | ) | [virtual] |
Definition at line 114 of file networkaccount.cpp.
void KMail::NetworkAccount::setCheckingMail | ( | bool | checking | ) | [virtual] |
void KMail::NetworkAccount::setHost | ( | const QString & | host | ) | [virtual] |
Definition at line 124 of file networkaccount.cpp.
void KMail::NetworkAccount::setLogin | ( | const QString & | login | ) | [virtual] |
Definition at line 92 of file networkaccount.cpp.
void KMail::NetworkAccount::setPasswd | ( | const QString & | passwd, | |
bool | storeInConfig = false | |||
) | [virtual] |
Definition at line 102 of file networkaccount.cpp.
void KMail::NetworkAccount::setPort | ( | unsigned short int | port | ) | [virtual] |
Definition at line 128 of file networkaccount.cpp.
void KMail::NetworkAccount::setSieveConfig | ( | const KMail::SieveConfig & | config | ) | [virtual] |
Definition at line 140 of file networkaccount.cpp.
void KMail::NetworkAccount::setStorePasswd | ( | bool | store | ) | [virtual] |
Definition at line 118 of file networkaccount.cpp.
void KMail::NetworkAccount::setUseSSL | ( | bool | use | ) | [virtual] |
Definition at line 132 of file networkaccount.cpp.
void KMail::NetworkAccount::setUseTLS | ( | bool | use | ) | [virtual] |
Definition at line 136 of file networkaccount.cpp.
KMail::SieveConfig KMail::NetworkAccount::sieveConfig | ( | ) | const [inline] |
KIO::Slave* KMail::NetworkAccount::slave | ( | ) | const [inline] |
MetaData KMail::NetworkAccount::slaveConfig | ( | ) | const [virtual] |
Configure the slave by adding to the meta data map.
Reimplemented in KMail::ImapAccountBase, and KMail::PopAccount.
Definition at line 270 of file networkaccount.cpp.
bool KMail::NetworkAccount::storePasswd | ( | ) | const [inline] |
- Returns:
- whether to store the password in the config file
Definition at line 77 of file networkaccount.h.
bool KMail::NetworkAccount::useSSL | ( | ) | const [inline] |
bool KMail::NetworkAccount::useTLS | ( | ) | const [inline] |
void KMail::NetworkAccount::writeConfig | ( | KConfig & | config | ) | [virtual] |
Write all account information to given config file.
The config group is already properly set by the caller.
Reimplemented from KMAccount.
Reimplemented in KMail::ImapAccountBase, KMAcctCachedImap, and KMail::PopAccount.
Definition at line 198 of file networkaccount.cpp.
Member Data Documentation
bool KMail::NetworkAccount::mAskAgain [protected] |
Definition at line 136 of file networkaccount.h.
QString KMail::NetworkAccount::mAuth [protected] |
Definition at line 131 of file networkaccount.h.
QString KMail::NetworkAccount::mHost [protected] |
Definition at line 131 of file networkaccount.h.
QString KMail::NetworkAccount::mLogin [protected] |
Definition at line 131 of file networkaccount.h.
QString KMail::NetworkAccount::mPasswd [protected] |
Definition at line 131 of file networkaccount.h.
bool KMail::NetworkAccount::mPasswdDirty [protected] |
Definition at line 137 of file networkaccount.h.
unsigned short int KMail::NetworkAccount::mPort [protected] |
Definition at line 132 of file networkaccount.h.
Definition at line 129 of file networkaccount.h.
QGuardedPtr<KIO::Slave> KMail::NetworkAccount::mSlave [protected] |
Definition at line 130 of file networkaccount.h.
bool KMail::NetworkAccount::mStorePasswd [protected] |
Definition at line 133 of file networkaccount.h.
bool KMail::NetworkAccount::mStorePasswdInConfig [protected] |
Definition at line 137 of file networkaccount.h.
bool KMail::NetworkAccount::mUseSSL [protected] |
Definition at line 134 of file networkaccount.h.
bool KMail::NetworkAccount::mUseTLS [protected] |
Definition at line 135 of file networkaccount.h.
The documentation for this class was generated from the following files: