kmail
KMail::PopAccount Class Reference
KMail account for pop mail account. More...
#include <popaccount.h>
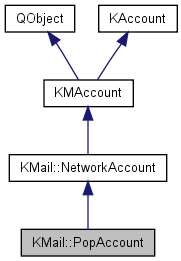
Detailed Description
KMail account for pop mail account.Definition at line 28 of file popaccount.h.
Member Enumeration Documentation
enum KMail::PopAccount::Stage [protected] |
Constructor & Destructor Documentation
KMail::PopAccount::~PopAccount | ( | ) | [virtual] |
Definition at line 87 of file popaccount.cpp.
KMail::PopAccount::PopAccount | ( | AccountManager * | owner, | |
const QString & | accountName, | |||
uint | id | |||
) | [protected] |
Definition at line 56 of file popaccount.cpp.
Member Function Documentation
void KMail::PopAccount::connectJob | ( | ) | [protected] |
Connect up the standard signals/slots for the KIO Jobs.
Definition at line 287 of file popaccount.cpp.
unsigned short int KMail::PopAccount::defaultPort | ( | ) | const [virtual] |
bool KMail::PopAccount::filterOnServer | ( | void | ) | const [inline] |
Shall messages be filter on the server (TRUE) or not (FALSE).
Definition at line 78 of file popaccount.h.
unsigned int KMail::PopAccount::filterOnServerCheckSize | ( | void | ) | const [inline] |
Size of messages which should be check on the pop server before download.
Definition at line 85 of file popaccount.h.
void KMail::PopAccount::init | ( | void | ) | [virtual] |
Set the config options to a decent state.
Reimplemented from KMail::NetworkAccount.
Definition at line 113 of file popaccount.cpp.
void KMail::PopAccount::killAllJobs | ( | bool | disconnectSlave = false |
) | [virtual] |
Kill all jobs that are currently in progress.
Implements KMail::NetworkAccount.
Definition at line 1081 of file popaccount.cpp.
bool KMail::PopAccount::leaveOnServer | ( | void | ) | const [inline] |
Shall messages be left on the server upon retreival (TRUE) or deleted (FALSE).
Definition at line 53 of file popaccount.h.
int KMail::PopAccount::leaveOnServerCount | ( | void | ) | const [inline] |
If value is positive, leave so many messages on the server.
Definition at line 65 of file popaccount.h.
int KMail::PopAccount::leaveOnServerDays | ( | void | ) | const [inline] |
If value is positive, leave mail on the server for so many days.
Definition at line 59 of file popaccount.h.
int KMail::PopAccount::leaveOnServerSize | ( | void | ) | const [inline] |
void KMail::PopAccount::processNewMail | ( | bool | interactive | ) | [virtual] |
Process new mail for this account if one arrived.
Returns TRUE if new mail has been found. Whether the mail is automatically loaded to an associated folder or not depends on the type of the account.
Implements KMAccount.
Definition at line 145 of file popaccount.cpp.
void KMail::PopAccount::processRemainingQueuedMessages | ( | ) | [protected] |
QString KMail::PopAccount::protocol | ( | ) | const [virtual] |
void KMail::PopAccount::pseudoAssign | ( | const KMAccount * | a | ) | [virtual] |
A weak assignment operator.
Reimplemented from KMail::NetworkAccount.
Definition at line 128 of file popaccount.cpp.
void KMail::PopAccount::readConfig | ( | KConfig & | config | ) | [virtual] |
Read config file entries.
This method is called by the account manager when a new account is created. The config group is already properly set by the caller.
Reimplemented from KMail::NetworkAccount.
Definition at line 215 of file popaccount.cpp.
void KMail::PopAccount::saveUidList | ( | ) | [protected] |
void KMail::PopAccount::setFilterOnServer | ( | bool | b | ) | [virtual] |
Definition at line 275 of file popaccount.cpp.
void KMail::PopAccount::setFilterOnServerCheckSize | ( | unsigned int | aSize | ) | [virtual] |
Definition at line 281 of file popaccount.cpp.
void KMail::PopAccount::setLeaveOnServer | ( | bool | b | ) | [virtual] |
Definition at line 251 of file popaccount.cpp.
void KMail::PopAccount::setLeaveOnServerCount | ( | int | count | ) | [virtual] |
Definition at line 263 of file popaccount.cpp.
void KMail::PopAccount::setLeaveOnServerDays | ( | int | days | ) | [virtual] |
Definition at line 257 of file popaccount.cpp.
void KMail::PopAccount::setLeaveOnServerSize | ( | int | size | ) | [virtual] |
Definition at line 269 of file popaccount.cpp.
void KMail::PopAccount::setUsePipelining | ( | bool | b | ) | [virtual] |
Definition at line 245 of file popaccount.cpp.
MetaData KMail::PopAccount::slaveConfig | ( | ) | const [virtual] |
Configure the slave by adding to the meta data map.
Reimplemented from KMail::NetworkAccount.
Definition at line 424 of file popaccount.cpp.
void KMail::PopAccount::slotAbortRequested | ( | ) | [protected, slot] |
void KMail::PopAccount::slotCancel | ( | ) | [protected, slot] |
void KMail::PopAccount::slotData | ( | KIO::Job * | job, | |
const QByteArray & | data | |||
) | [protected, slot] |
New data has arrived append it to the end of the current message.
Definition at line 877 of file popaccount.cpp.
void KMail::PopAccount::slotGetNextHdr | ( | ) | [protected, slot] |
If there are more headers to be downloaded then start a new kio job to get the next header.
Definition at line 1071 of file popaccount.cpp.
void KMail::PopAccount::slotGetNextMsg | ( | ) | [protected, slot] |
If there are more messages to be downloaded then start a new kio job to get the message whose id is at the head of the queue.
Definition at line 854 of file popaccount.cpp.
void KMail::PopAccount::slotJobFinished | ( | ) | [protected, slot] |
Called when a job is finished.
Basically a finite state machine for cycling through the Idle, List, Uidl, Retr, Quit stages
Definition at line 476 of file popaccount.cpp.
void KMail::PopAccount::slotMsgRetrieved | ( | KIO::Job * | , | |
const QString & | infoMsg | |||
) | [protected, slot] |
A messages has been retrieved successfully.
The next data belongs to the next message.
Definition at line 444 of file popaccount.cpp.
void KMail::PopAccount::slotProcessPendingMsgs | ( | ) | [protected, slot] |
Messages are downloaded in the background and then once every x seconds a batch of messages are processed.
Messages are processed in batches to reduce flicker (multiple refreshes of the qlistview of messages headers in a single second causes flicker) when using a fast pop server such as one on a lan.
Processing a message means applying KMAccount::processNewMsg to it and adding its UID to the list of seen UIDs
Definition at line 309 of file popaccount.cpp.
void KMail::PopAccount::slotResult | ( | KIO::Job * | ) | [protected, slot] |
Finished downloading the current kio job, either due to an error or because the job has been canceled or because the complete message has been downloaded.
Definition at line 1015 of file popaccount.cpp.
void KMail::PopAccount::slotSlaveError | ( | KIO::Slave * | aSlave, | |
int | error, | |||
const QString & | errorMsg | |||
) | [protected, slot] |
void KMail::PopAccount::startJob | ( | ) | [protected] |
Start a KIO Job to get a list of messages on the pop server.
Definition at line 367 of file popaccount.cpp.
QString KMail::PopAccount::type | ( | void | ) | const [virtual] |
bool KMail::PopAccount::usePipelining | ( | void | ) | const [inline] |
void KMail::PopAccount::writeConfig | ( | KConfig & | config | ) | [virtual] |
Write all account information to given config file.
The config group is already properly set by the caller.
Reimplemented from KMail::NetworkAccount.
Definition at line 230 of file popaccount.cpp.
Member Data Documentation
QByteArray KMail::PopAccount::curMsgData [protected] |
Definition at line 160 of file popaccount.h.
int KMail::PopAccount::curMsgLen [protected] |
Definition at line 163 of file popaccount.h.
QDataStream* KMail::PopAccount::curMsgStrm [protected] |
Definition at line 161 of file popaccount.h.
int KMail::PopAccount::dataCounter [protected] |
Definition at line 171 of file popaccount.h.
bool KMail::PopAccount::gotMsgs [protected] |
Definition at line 128 of file popaccount.h.
QPtrListIterator<KMPopHeaders> KMail::PopAccount::headerIt [protected] |
Definition at line 137 of file popaccount.h.
bool KMail::PopAccount::headers [protected] |
Definition at line 138 of file popaccount.h.
QPtrList<KMPopHeaders> KMail::PopAccount::headersOnServer [protected] |
Definition at line 136 of file popaccount.h.
QStringList KMail::PopAccount::idsOfForcedDeletes [protected] |
Definition at line 153 of file popaccount.h.
QStringList KMail::PopAccount::idsOfMsgs [protected] |
Definition at line 144 of file popaccount.h.
QStringList KMail::PopAccount::idsOfMsgsToDelete [protected] |
Definition at line 152 of file popaccount.h.
int KMail::PopAccount::indexOfCurrentMsg [protected] |
Definition at line 154 of file popaccount.h.
bool KMail::PopAccount::interactive [protected] |
Definition at line 168 of file popaccount.h.
KIO::SimpleJob* KMail::PopAccount::job [protected] |
Definition at line 132 of file popaccount.h.
QValueList<int> KMail::PopAccount::lensOfMsgs [protected] |
Definition at line 145 of file popaccount.h.
bool KMail::PopAccount::mFilterOnServer [protected] |
Definition at line 129 of file popaccount.h.
unsigned int KMail::PopAccount::mFilterOnServerCheckSize [protected] |
Definition at line 130 of file popaccount.h.
QMap<QString, bool> KMail::PopAccount::mHeaderDeleteUids [protected] |
Definition at line 140 of file popaccount.h.
QMap<QString, bool> KMail::PopAccount::mHeaderDownUids [protected] |
Definition at line 141 of file popaccount.h.
QMap<QString, bool> KMail::PopAccount::mHeaderLaterUids [protected] |
Definition at line 142 of file popaccount.h.
bool KMail::PopAccount::mLeaveOnServer [protected] |
Definition at line 124 of file popaccount.h.
int KMail::PopAccount::mLeaveOnServerCount [protected] |
Definition at line 126 of file popaccount.h.
int KMail::PopAccount::mLeaveOnServerDays [protected] |
Definition at line 125 of file popaccount.h.
int KMail::PopAccount::mLeaveOnServerSize [protected] |
Definition at line 127 of file popaccount.h.
QMap<QString, int> KMail::PopAccount::mMsgsPendingDownload [protected] |
Definition at line 134 of file popaccount.h.
bool KMail::PopAccount::mProcessing [protected] |
Definition at line 169 of file popaccount.h.
Definition at line 157 of file popaccount.h.
QValueList<KMMessage*> KMail::PopAccount::msgsAwaitingProcessing [protected] |
Definition at line 156 of file popaccount.h.
Definition at line 158 of file popaccount.h.
QDict<int> KMail::PopAccount::mSizeOfNextSeenMsgsDict [protected] |
Definition at line 151 of file popaccount.h.
QMap<QString, int> KMail::PopAccount::mTimeOfNextSeenMsgsMap [protected] |
Definition at line 150 of file popaccount.h.
QValueVector<int> KMail::PopAccount::mTimeOfSeenMsgsVector [protected] |
Definition at line 149 of file popaccount.h.
QMap<QString, QString> KMail::PopAccount::mUidForIdMap [protected] |
Definition at line 146 of file popaccount.h.
bool KMail::PopAccount::mUidlFinished [protected] |
Definition at line 170 of file popaccount.h.
QDict<int> KMail::PopAccount::mUidsOfNextSeenMsgsDict [protected] |
Definition at line 148 of file popaccount.h.
QDict<int> KMail::PopAccount::mUidsOfSeenMsgsDict [protected] |
Definition at line 147 of file popaccount.h.
bool KMail::PopAccount::mUsePipelining [protected] |
Definition at line 123 of file popaccount.h.
int KMail::PopAccount::numBytes [protected] |
Definition at line 167 of file popaccount.h.
int KMail::PopAccount::numBytesRead [protected] |
Definition at line 167 of file popaccount.h.
int KMail::PopAccount::numBytesToRead [protected] |
Definition at line 167 of file popaccount.h.
int KMail::PopAccount::numMsgBytesRead [protected] |
Definition at line 167 of file popaccount.h.
int KMail::PopAccount::numMsgs [protected] |
Definition at line 167 of file popaccount.h.
int KMail::PopAccount::processingDelay [protected] |
Definition at line 166 of file popaccount.h.
QTimer KMail::PopAccount::processMsgsTimer [protected] |
Definition at line 165 of file popaccount.h.
Stage KMail::PopAccount::stage [protected] |
Definition at line 164 of file popaccount.h.
The documentation for this class was generated from the following files: