kmail
KMail::AccountManager Class Reference
The account manager is responsible for creating accounts of various types via the factory method create() and for keeping track of them. More...
#include <accountmanager.h>
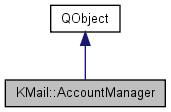
Public Slots | |
void | addToTotalNewMailCount (const QMap< QString, int > &newInFolder) |
void | intCheckMail (int, bool interactive=true) |
void | processNextCheck (bool newMail) |
void | singleCheckMail (KMAccount *, bool interactive=true) |
void | singleInvalidateIMAPFolders (KMAccount *) |
Signals | |
void | accountAdded (KMAccount *account) |
void | accountRemoved (KMAccount *account) |
void | checkedMail (bool newMail, bool interactive, const QMap< QString, int > &newInFolder) |
Public Member Functions | |
AccountManager () | |
void | add (KMAccount *account) |
void | cancelMailCheck () |
void | checkMail (bool interactive=true) |
KMAccount * | create (const QString &type, const QString &name=QString::null, uint id=0) |
KMAccount * | find (const uint id) const |
KMAccount * | findByName (const QString &name) const |
KMAccount * | first () |
const KMAccount * | first () const |
QStringList | getAccounts () const |
void | invalidateIMAPFolders () |
KMAccount * | next () |
const KMAccount * | next () const |
void | readConfig (void) |
void | readPasswords () |
bool | remove (KMAccount *) |
void | writeConfig (bool withSync=true) |
~AccountManager () |
Detailed Description
The account manager is responsible for creating accounts of various types via the factory method create() and for keeping track of them.Definition at line 35 of file accountmanager.h.
Constructor & Destructor Documentation
AccountManager::AccountManager | ( | ) |
Initializes the account manager.
readConfig() needs to be called in order to fill it with persisted account information from the config file.
Definition at line 33 of file accountmanager.cpp.
AccountManager::~AccountManager | ( | ) |
Definition at line 42 of file accountmanager.cpp.
Member Function Documentation
void KMail::AccountManager::accountAdded | ( | KMAccount * | account | ) | [signal] |
emitted when an account is added
void KMail::AccountManager::accountRemoved | ( | KMAccount * | account | ) | [signal] |
emitted when an account is removed
void AccountManager::add | ( | KMAccount * | account | ) |
this slot increases the count of new mails to show a total number after checking in multiple accounts.
Definition at line 366 of file accountmanager.cpp.
void AccountManager::cancelMailCheck | ( | ) |
void KMail::AccountManager::checkedMail | ( | bool | newMail, | |
bool | interactive, | |||
const QMap< QString, int > & | newInFolder | |||
) | [signal] |
Emitted if new mail has been collected.
- Parameters:
-
newMail true if there was new mail interactive true if the mail check was initiated by the user newInFolder number of new messages for each folder
void AccountManager::checkMail | ( | bool | interactive = true |
) |
KMAccount * AccountManager::create | ( | const QString & | type, | |
const QString & | name = QString::null , |
|||
uint | id = 0 | |||
) |
Create a new account of given type with given name.
Currently the types "local" for local mail folders and "pop" are supported.
Definition at line 208 of file accountmanager.cpp.
KMAccount * AccountManager::find | ( | const uint | id | ) | const |
Find account by id.
Returns 0 if account does not exist.
Definition at line 267 of file accountmanager.cpp.
Find account by name.
Returns 0 if account does not exist. Search is done case sensitive.
Definition at line 255 of file accountmanager.cpp.
KMAccount * AccountManager::first | ( | ) |
Definition at line 278 of file accountmanager.cpp.
const KMAccount* KMail::AccountManager::first | ( | ) | const [inline] |
QStringList AccountManager::getAccounts | ( | ) | const |
Definition at line 344 of file accountmanager.cpp.
void AccountManager::intCheckMail | ( | int | item, | |
bool | interactive = true | |||
) | [slot] |
Definition at line 354 of file accountmanager.cpp.
void AccountManager::invalidateIMAPFolders | ( | ) |
KMAccount * AccountManager::next | ( | ) |
Definition at line 289 of file accountmanager.cpp.
const KMAccount* KMail::AccountManager::next | ( | ) | const [inline] |
void AccountManager::processNextCheck | ( | bool | newMail | ) | [slot] |
Definition at line 136 of file accountmanager.cpp.
void AccountManager::readConfig | ( | void | ) |
void AccountManager::readPasswords | ( | ) |
bool AccountManager::remove | ( | KMAccount * | acct | ) |
Physically remove account.
Also deletes the given account object ! Returns FALSE and does nothing if the account cannot be removed.
Definition at line 299 of file accountmanager.cpp.
void AccountManager::singleCheckMail | ( | KMAccount * | account, | |
bool | interactive = true | |||
) | [slot] |
Definition at line 112 of file accountmanager.cpp.
void AccountManager::singleInvalidateIMAPFolders | ( | KMAccount * | account | ) | [slot] |
Definition at line 331 of file accountmanager.cpp.
void AccountManager::writeConfig | ( | bool | withSync = true |
) |
The documentation for this class was generated from the following files: