kmail
KMFolderSearch Class Reference
#include <kmfoldersearch.h>
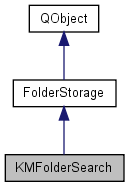
Public Slots | |
void | addSerNum (Q_UINT32 serNum) |
void | removeSerNum (Q_UINT32 serNum) |
void | slotSearchExamineMsgDone (KMFolder *, Q_UINT32 serNum, const KMSearchPattern *, bool) |
virtual int | updateIndex () |
Public Member Functions | |
virtual int | addMsg (KMMessage *msg, int *index_return=0) |
virtual int | canAccess () |
virtual int | compact (bool) |
virtual int | create () |
virtual int | find (const KMMsgBase *msg) const |
virtual KMFolderType | folderType () const |
DwString | getDwString (int idx) |
virtual KMMessage * | getMsg (int idx) |
virtual KMMsgBase * | getMsgBase (int idx) |
virtual const KMMsgBase * | getMsgBase (int idx) const |
virtual void | ignoreJobsForMessage (KMMessage *) |
virtual QString | indexLocation () const |
virtual bool | isMoveable () const |
virtual bool | isReadOnly () const |
KMFolderSearch (KMFolder *folder, const char *name=0) | |
virtual int | open (const char *owner) |
virtual void | reallyDoClose (const char *owner) |
const KMSearch * | search () const |
Q_UINT32 | serNum (int idx) |
void | setSearch (KMSearch *search) |
void | stopSearch () |
virtual void | sync () |
virtual void | tryReleasingFolder (KMFolder *folder) |
virtual int | writeIndex (bool createEmptyIndex=false) |
virtual | ~KMFolderSearch () |
Protected Slots | |
void | examineAddedMessage (KMFolder *folder, Q_UINT32 serNum) |
void | examineChangedMessage (KMFolder *folder, Q_UINT32 serNum, int delta) |
void | examineInvalidatedFolder (KMFolder *folder) |
void | examineRemovedFolder (KMFolder *folder) |
void | examineRemovedMessage (KMFolder *folder, Q_UINT32 serNum) |
void | executeSearch () |
void | propagateHeaderChanged (KMFolder *folder, int idx) |
bool | readSearch () |
void | searchFinished (bool success) |
Protected Member Functions | |
virtual void | clearIndex (bool autoDelete=true, bool syncDict=false) |
virtual int | count (bool cache=false) const |
virtual FolderJob * | doCreateJob (QPtrList< KMMessage > &msgList, const QString &sets, FolderJob::JobType jt, KMFolder *folder) const |
virtual FolderJob * | doCreateJob (KMMessage *msg, FolderJob::JobType jt, KMFolder *folder, QString partSpecifier, const AttachmentStrategy *as) const |
virtual int | expungeContents () |
virtual bool | readIndex () |
virtual KMMessage * | readMsg (int idx) |
virtual int | removeContents () |
virtual KMMsgInfo * | setIndexEntry (int idx, KMMessage *msg) |
virtual KMMsgBase * | takeIndexEntry (int idx) |
virtual void | truncateIndex () |
Detailed Description
Definition at line 109 of file kmfoldersearch.h.
Constructor & Destructor Documentation
KMFolderSearch::KMFolderSearch | ( | KMFolder * | folder, | |
const char * | name = 0 | |||
) |
Definition at line 316 of file kmfoldersearch.cpp.
KMFolderSearch::~KMFolderSearch | ( | ) | [virtual] |
Definition at line 378 of file kmfoldersearch.cpp.
Member Function Documentation
int KMFolderSearch::addMsg | ( | KMMessage * | msg, | |
int * | index_return = 0 | |||
) | [virtual] |
Add the given message to the folder.
Usually the message is added at the end of the folder. Returns zero on success and an errno error code on failure. The index of the new message is stored in index_return if given. Please note that the message is added as is to the folder and the folder takes ownership of the message (deleting it in the destructor).
Implements FolderStorage.
Definition at line 495 of file kmfoldersearch.cpp.
void KMFolderSearch::addSerNum | ( | Q_UINT32 | serNum | ) | [slot] |
Definition at line 441 of file kmfoldersearch.cpp.
int KMFolderSearch::canAccess | ( | ) | [virtual] |
Check folder for permissions Returns zero if readable and writable.
Implements FolderStorage.
Definition at line 530 of file kmfoldersearch.cpp.
void KMFolderSearch::clearIndex | ( | bool | autoDelete = true , |
|
bool | syncDict = false | |||
) | [protected, virtual] |
int KMFolderSearch::compact | ( | bool | silent | ) | [virtual] |
Remove deleted messages from the folder.
Returns zero on success and an errno on failure. A statusbar message will inform the user that the compaction worked, unless silent
is set.
Implements FolderStorage.
Definition at line 616 of file kmfoldersearch.cpp.
int KMFolderSearch::count | ( | bool | cache = false |
) | const [protected, virtual] |
Number of messages in this folder.
Reimplemented from FolderStorage.
Definition at line 893 of file kmfoldersearch.cpp.
int KMFolderSearch::create | ( | ) | [virtual] |
Create a new folder with the name of this object and open it.
Returns zero on success and an error code equal to the c-library fopen call otherwise.
Implements FolderStorage.
Definition at line 578 of file kmfoldersearch.cpp.
FolderJob * KMFolderSearch::doCreateJob | ( | KMMessage * | msg, | |
FolderJob::JobType | jt, | |||
KMFolder * | folder, | |||
QString | partSpecifier, | |||
const AttachmentStrategy * | as | |||
) | const [protected, virtual] |
These two methods actually create the jobs.
They have to be implemented in all folders.
- See also:
- createJob
Implements FolderStorage.
Definition at line 627 of file kmfoldersearch.cpp.
void KMFolderSearch::examineAddedMessage | ( | KMFolder * | folder, | |
Q_UINT32 | serNum | |||
) | [protected, slot] |
Definition at line 934 of file kmfoldersearch.cpp.
void KMFolderSearch::examineChangedMessage | ( | KMFolder * | folder, | |
Q_UINT32 | serNum, | |||
int | delta | |||
) | [protected, slot] |
Definition at line 1034 of file kmfoldersearch.cpp.
void KMFolderSearch::examineInvalidatedFolder | ( | KMFolder * | folder | ) | [protected, slot] |
Definition at line 1053 of file kmfoldersearch.cpp.
void KMFolderSearch::examineRemovedFolder | ( | KMFolder * | folder | ) | [protected, slot] |
Definition at line 1083 of file kmfoldersearch.cpp.
void KMFolderSearch::examineRemovedMessage | ( | KMFolder * | folder, | |
Q_UINT32 | serNum | |||
) | [protected, slot] |
Definition at line 1016 of file kmfoldersearch.cpp.
void KMFolderSearch::executeSearch | ( | ) | [protected, slot] |
Definition at line 421 of file kmfoldersearch.cpp.
int KMFolderSearch::expungeContents | ( | ) | [protected, virtual] |
Called by KMFolder::expunge() to delete the actual contents.
At the time of the call the folder has already been closed, and the various index files deleted. Returns 0 on success.
Implements FolderStorage.
Definition at line 887 of file kmfoldersearch.cpp.
int KMFolderSearch::find | ( | const KMMsgBase * | msg | ) | const [virtual] |
Returns the index of the given message or -1 if not found.
Implements FolderStorage.
Definition at line 700 of file kmfoldersearch.cpp.
virtual KMFolderType KMFolderSearch::folderType | ( | ) | const [inline, virtual] |
Returns the type of this folder.
Reimplemented from FolderStorage.
Definition at line 118 of file kmfoldersearch.h.
DwString KMFolderSearch::getDwString | ( | int | idx | ) | [virtual] |
Read a message and returns a DwString.
Implements FolderStorage.
Definition at line 787 of file kmfoldersearch.cpp.
KMMessage * KMFolderSearch::getMsg | ( | int | idx | ) | [virtual] |
Read message at given index.
Indexing starts at zero
Reimplemented from FolderStorage.
Definition at line 667 of file kmfoldersearch.cpp.
KMMsgBase * KMFolderSearch::getMsgBase | ( | int | idx | ) | [virtual] |
const KMMsgBase * KMFolderSearch::getMsgBase | ( | int | idx | ) | const [virtual] |
Provides access to the basic message fields that are also stored in the index.
Whenever you only need subject, from, date, status you should use this method instead of getMsg() because getMsg() will load the message if necessary and this method does not.
Implements FolderStorage.
Definition at line 643 of file kmfoldersearch.cpp.
void KMFolderSearch::ignoreJobsForMessage | ( | KMMessage * | msg | ) | [virtual] |
Removes and deletes all jobs associated with the particular message.
Reimplemented from FolderStorage.
Definition at line 681 of file kmfoldersearch.cpp.
QString KMFolderSearch::indexLocation | ( | ) | const [virtual] |
Returns full path to index file.
Implements FolderStorage.
Definition at line 713 of file kmfoldersearch.cpp.
virtual bool KMFolderSearch::isMoveable | ( | ) | const [inline, virtual] |
Returns true if this folder can be moved.
Reimplemented from FolderStorage.
Definition at line 133 of file kmfoldersearch.h.
bool KMFolderSearch::isReadOnly | ( | ) | const [virtual] |
Is the folder read-only?
Implements FolderStorage.
Definition at line 622 of file kmfoldersearch.cpp.
int KMFolderSearch::open | ( | const char * | owner | ) | [virtual] |
Open folder for access.
open() and close() use reference counting. Returns zero on success and an error code equal to the c-library fopen call otherwise (errno).
- See also:
- KMFolderOpener
Implements FolderStorage.
Definition at line 510 of file kmfoldersearch.cpp.
void KMFolderSearch::propagateHeaderChanged | ( | KMFolder * | folder, | |
int | idx | |||
) | [protected, slot] |
Definition at line 1092 of file kmfoldersearch.cpp.
bool KMFolderSearch::readIndex | ( | ) | [protected, virtual] |
Read index file and fill the message-info list mMsgList.
Implements FolderStorage.
Definition at line 801 of file kmfoldersearch.cpp.
KMMessage * KMFolderSearch::readMsg | ( | int | idx | ) | [protected, virtual] |
Load message from file and store it at given index.
Returns 0 on failure.
Implements FolderStorage.
Definition at line 792 of file kmfoldersearch.cpp.
bool KMFolderSearch::readSearch | ( | ) | [protected, slot] |
Definition at line 502 of file kmfoldersearch.cpp.
void KMFolderSearch::reallyDoClose | ( | const char * | owner | ) | [virtual] |
int KMFolderSearch::removeContents | ( | ) | [protected, virtual] |
Called by KMFolder::remove() to delete the actual contents.
At the time of the call the folder has already been closed, and the various index files deleted. Returns 0 on success.
Implements FolderStorage.
Definition at line 879 of file kmfoldersearch.cpp.
void KMFolderSearch::removeSerNum | ( | Q_UINT32 | serNum | ) | [slot] |
Definition at line 475 of file kmfoldersearch.cpp.
const KMSearch * KMFolderSearch::search | ( | ) | const |
Definition at line 429 of file kmfoldersearch.cpp.
void KMFolderSearch::searchFinished | ( | bool | success | ) | [protected, slot] |
Definition at line 434 of file kmfoldersearch.cpp.
Q_UINT32 KMFolderSearch::serNum | ( | int | idx | ) | [inline] |
Definition at line 186 of file kmfoldersearch.h.
void KMFolderSearch::setSearch | ( | KMSearch * | search | ) |
Definition at line 387 of file kmfoldersearch.cpp.
void KMFolderSearch::slotSearchExamineMsgDone | ( | KMFolder * | folder, | |
Q_UINT32 | serNum, | |||
const KMSearchPattern * | pattern, | |||
bool | matches | |||
) | [slot] |
Definition at line 970 of file kmfoldersearch.cpp.
void KMFolderSearch::stopSearch | ( | ) | [inline] |
Definition at line 125 of file kmfoldersearch.h.
void KMFolderSearch::sync | ( | ) | [virtual] |
KMMsgBase * KMFolderSearch::takeIndexEntry | ( | int | idx | ) | [protected, virtual] |
void KMFolderSearch::truncateIndex | ( | ) | [protected, virtual] |
void KMFolderSearch::tryReleasingFolder | ( | KMFolder * | ) | [virtual] |
Try releasing folder
if possible, something is attempting an exclusive access to it.
Currently used for KMFolderSearch and the background tasks like expiry.
Reimplemented from FolderStorage.
Definition at line 1131 of file kmfoldersearch.cpp.
int KMFolderSearch::updateIndex | ( | ) | [virtual, slot] |
Incrementally update the index if possible else call writeIndex.
Implements FolderStorage.
Definition at line 726 of file kmfoldersearch.cpp.
int KMFolderSearch::writeIndex | ( | bool | createEmptyIndex = false |
) | [virtual] |
Write index to index-file.
Returns 0 on success and errno error on failure.
Implements FolderStorage.
Definition at line 736 of file kmfoldersearch.cpp.
The documentation for this class was generated from the following files: