kmail
KMMsgBase Class Reference
#include <kmmsgbase.h>
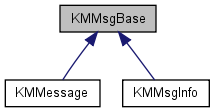
Public Types | |
enum | MsgPartType { MsgNoPart = 0, MsgFromPart = 1, MsgSubjectPart = 2, MsgToPart = 3, MsgReplyToIdMD5Part = 4, MsgIdMD5Part = 5, MsgXMarkPart = 6, MsgOffsetPart = 7, MsgLegacyStatusPart = 8, MsgSizePart = 9, MsgDatePart = 10, MsgFilePart = 11, MsgCryptoStatePart = 12, MsgMDNSentPart = 13, MsgReplyToAuxIdMD5Part = 14, MsgStrippedSubjectMD5Part = 15, MsgStatusPart = 16, MsgSizeServerPart = 17, MsgUIDPart = 18 } |
Public Member Functions | |
const uchar * | asIndexString (int &len) const |
void | assign (const KMMsgBase *other) |
virtual KMMsgAttachmentState | attachmentState () const |
QString | cleanSubject () const |
QString | cleanSubject (const QStringList &prefixRegExps, bool replace, const QString &newPrefix) const |
virtual time_t | date (void) const =0 |
virtual QString | dateStr (void) const |
virtual bool | dirty (void) const |
virtual bool | enableUndo () |
virtual KMMsgEncryptionState | encryptionState () const =0 |
virtual QString | fileName (void) const =0 |
virtual off_t | folderOffset (void) const =0 |
QString | forwardSubject () const |
virtual QString | fromStrip (void) const =0 |
off_t | getLongPart (MsgPartType) const |
virtual unsigned long | getMsgSerNum () const |
QString | getStringPart (MsgPartType) const |
virtual short | indexLength () const |
virtual off_t | indexOffset () const |
virtual void | initStrippedSubjectMD5 ()=0 |
virtual bool | isDeleted (void) const |
virtual bool | isForwarded (void) const |
virtual bool | isHam (void) const |
virtual bool | isIgnored (void) const |
virtual bool | isImportant (void) const |
virtual bool | isMessage (void) const |
virtual bool | isNew (void) const |
virtual bool | isOfUnknownStatus (void) const |
virtual bool | isOld (void) const |
virtual bool | isQueued (void) const |
virtual bool | isRead (void) const |
virtual bool | isReplied (void) const |
virtual bool | isSent (void) const |
virtual bool | isSpam (void) const |
virtual bool | isTodo (void) const |
virtual bool | isUnread (void) const |
virtual bool | isWatched (void) const |
KMMsgBase (const KMMsgBase &other) | |
KMMsgBase (KMFolder *p=0) | |
virtual KMMsgMDNSentState | mdnSentState () const =0 |
virtual QString | msgIdMD5 (void) const =0 |
virtual size_t | msgSize (void) const =0 |
virtual size_t | msgSizeServer (void) const =0 |
KMMsgBase & | operator= (const KMMsgBase &other) |
KMFolder * | parent () const |
QString | replySubject () const |
virtual QString | replyToAuxIdMD5 () const =0 |
virtual QString | replyToIdMD5 (void) const =0 |
virtual void | setDate (time_t aUnixTime)=0 |
virtual void | setDate (const QCString &aStrDate) |
void | setDirty (bool b) |
virtual void | setEnableUndo (bool enable) |
virtual void | setEncryptionState (const KMMsgEncryptionState, int idx=-1) |
virtual void | setEncryptionStateChar (QChar status, int idx=-1) |
virtual void | setFileName (const QString &filename)=0 |
virtual void | setFolderOffset (off_t offs)=0 |
virtual void | setIndexLength (short len) |
virtual void | setIndexOffset (off_t off) |
virtual void | setMDNSentState (KMMsgMDNSentState status, int idx=-1) |
virtual void | setMsgSize (size_t sz)=0 |
virtual void | setMsgSizeServer (size_t sz)=0 |
void | setParent (KMFolder *p) |
virtual void | setSignatureState (const KMMsgSignatureState, int idx=-1) |
virtual void | setSignatureStateChar (QChar status, int idx=-1) |
virtual void | setStatus (const char *statusField, const char *xstatusField=0) |
virtual void | setStatus (const KMMsgStatus status, int idx=-1) |
virtual void | setSubject (const QString &)=0 |
virtual void | setUID (ulong uid)=0 |
virtual void | setXMark (const QString &)=0 |
virtual KMMsgSignatureState | signatureState () const =0 |
virtual KMMsgStatus | status (void) const =0 |
QString | statusToSortRank () |
KMFolderIndex * | storage () const |
virtual QString | strippedSubjectMD5 () const =0 |
virtual QString | subject (void) const =0 |
virtual bool | subjectIsPrefixed () const =0 |
bool | syncIndexString () const |
virtual void | toggleStatus (const KMMsgStatus status, int idx=-1) |
virtual QString | toStrip (void) const =0 |
virtual ulong | UID (void) const =0 |
virtual QString | xmark (void) const =0 |
virtual | ~KMMsgBase () |
Static Public Member Functions | |
static QCString | autoDetectCharset (const QCString &encoding, const QStringList &encodingList, const QString &text) |
static QString | base64EncodedMD5 (const char *aStr, int len=-1) |
static QString | base64EncodedMD5 (const QCString &aStr) |
static QString | base64EncodedMD5 (const QString &aStr, bool utf8=false) |
static const QTextCodec * | codecForName (const QCString &_str) |
static QString | decodeRFC2047String (const QCString &aStr, const QCString prefCharset="") |
static QString | decodeRFC2231String (const QCString &aStr) |
static QCString | encodeRFC2047Quoted (const QCString &aStr, bool base64) |
static QCString | encodeRFC2047String (const QString &aStr, const QCString &charset) |
static QCString | encodeRFC2231String (const QString &aStr, const QCString &charset) |
static QCString | extractRFC2231HeaderField (const QCString &aStr, const QCString &field) |
static void | readConfig () |
static QString | replacePrefixes (const QString &str, const QStringList &prefixRegExps, bool replace, const QString &newPrefix) |
static QString | skipKeyword (const QString &str, QChar sepChar=':', bool *keywordFound=0) |
static QCString | statusToStr (const KMMsgStatus status) |
static QString | stripOffPrefixes (const QString &str) |
static QStringList | supportedEncodings (bool usAscii) |
static QCString | toUsAscii (const QString &_str, bool *ok=0) |
Protected Attributes | |
bool | mDirty |
bool | mEnableUndo |
short | mIndexLength |
off_t | mIndexOffset |
KMLegacyMsgStatus | mLegacyStatus |
KMFolder * | mParent |
KMMsgStatus | mStatus |
Detailed Description
Definition at line 136 of file kmmsgbase.h.
Member Enumeration Documentation
- Enumerator:
Definition at line 438 of file kmmsgbase.h.
Constructor & Destructor Documentation
KMMsgBase::KMMsgBase | ( | KMFolder * | p = 0 |
) |
Definition at line 73 of file kmmsgbase.cpp.
KMMsgBase::~KMMsgBase | ( | ) | [virtual] |
Definition at line 81 of file kmmsgbase.cpp.
KMMsgBase::KMMsgBase | ( | const KMMsgBase & | other | ) |
Member Function Documentation
const uchar * KMMsgBase::asIndexString | ( | int & | len | ) | const |
Return contents as index string.
This string is of indexStringLength() size
Definition at line 1324 of file kmmsgbase.cpp.
void KMMsgBase::assign | ( | const KMMsgBase * | other | ) |
KMMsgAttachmentState KMMsgBase::attachmentState | ( | ) | const [virtual] |
QCString KMMsgBase::autoDetectCharset | ( | const QCString & | encoding, | |
const QStringList & | encodingList, | |||
const QString & | text | |||
) | [static] |
Find out preferred charset for 'text'.
First encoding
is tried and if that one is not suitable, the encodings in encodingList
are tried.
Definition at line 1010 of file kmmsgbase.cpp.
QString KMMsgBase::base64EncodedMD5 | ( | const char * | aStr, | |
int | len = -1 | |||
) | [static] |
Definition at line 1001 of file kmmsgbase.cpp.
Definition at line 996 of file kmmsgbase.cpp.
Calculate the base64 encoded md5sum (sans the trailing equal signs).
If utf8
is false, uses QString::latin1() to calculate the md5sum of, else uses QString::utf8()
Definition at line 988 of file kmmsgbase.cpp.
QString KMMsgBase::cleanSubject | ( | ) | const |
Return this mails subject, with all "forward" and "reply" prefixes removed.
Definition at line 1460 of file kmmsgbase.cpp.
QString KMMsgBase::cleanSubject | ( | const QStringList & | prefixRegExps, | |
bool | replace, | |||
const QString & | newPrefix | |||
) | const |
Check for prefixes prefixRegExps
in subject().
If none is found, newPrefix
+ ' ' is prepended to the subject and the resulting string is returned. If replace
is true, any sequence of whitespace-delimited prefixes at the beginning of subject() is replaced by newPrefix
Definition at line 1467 of file kmmsgbase.cpp.
const QTextCodec * KMMsgBase::codecForName | ( | const QCString & | _str | ) | [static] |
Return a QTextCodec for the specified charset.
This function is a bit more tolerant, than QTextCodec::codecForName
Definition at line 566 of file kmmsgbase.cpp.
virtual time_t KMMsgBase::date | ( | void | ) | const [pure virtual] |
QString KMMsgBase::dateStr | ( | void | ) | const [virtual] |
QString KMMsgBase::decodeRFC2047String | ( | const QCString & | aStr, | |
const QCString | prefCharset = "" | |||
) | [static] |
This function handles both encodings described in RFC2047: Base64 ("=?iso-8859-1?b?...?=") and quoted-printable.
Definition at line 650 of file kmmsgbase.cpp.
virtual bool KMMsgBase::dirty | ( | void | ) | const [inline, virtual] |
Returns TRUE if changed since last folder-sync.
Reimplemented in KMMsgInfo.
Definition at line 268 of file kmmsgbase.h.
virtual bool KMMsgBase::enableUndo | ( | ) | [inline, virtual] |
Encode given string as described in RFC2047: using quoted-printable.
Definition at line 766 of file kmmsgbase.cpp.
Encode given string as described in RFC2231 (parameters in MIME headers).
Definition at line 859 of file kmmsgbase.cpp.
virtual KMMsgEncryptionState KMMsgBase::encryptionState | ( | ) | const [pure virtual] |
QCString KMMsgBase::extractRFC2231HeaderField | ( | const QCString & | aStr, | |
const QCString & | field | |||
) | [static] |
Extract a given param from the RFC2231-encoded header field, in particular concatenate possibly multiple entries, which are given as paramname*0=.
.; paramname*1=..; ... or paramname*0*=..; paramname*1*=..; ... and return their value as one string. That string will still be encoded
Definition at line 952 of file kmmsgbase.cpp.
virtual QString KMMsgBase::fileName | ( | void | ) | const [pure virtual] |
virtual off_t KMMsgBase::folderOffset | ( | void | ) | const [pure virtual] |
QString KMMsgBase::forwardSubject | ( | ) | const |
Return this mails subject, formatted for "forward" mails.
Definition at line 1476 of file kmmsgbase.cpp.
virtual QString KMMsgBase::fromStrip | ( | void | ) | const [pure virtual] |
off_t KMMsgBase::getLongPart | ( | MsgPartType | t | ) | const |
unsigned long KMMsgBase::getMsgSerNum | ( | ) | const [virtual] |
QString KMMsgBase::getStringPart | ( | MsgPartType | t | ) | const |
virtual short KMMsgBase::indexLength | ( | ) | const [inline, virtual] |
Definition at line 309 of file kmmsgbase.h.
virtual off_t KMMsgBase::indexOffset | ( | ) | const [inline, virtual] |
Definition at line 305 of file kmmsgbase.h.
virtual void KMMsgBase::initStrippedSubjectMD5 | ( | ) | [pure virtual] |
bool KMMsgBase::isDeleted | ( | void | ) | const [virtual] |
bool KMMsgBase::isForwarded | ( | void | ) | const [virtual] |
bool KMMsgBase::isHam | ( | void | ) | const [virtual] |
bool KMMsgBase::isIgnored | ( | void | ) | const [virtual] |
bool KMMsgBase::isImportant | ( | void | ) | const [virtual] |
bool KMMsgBase::isMessage | ( | void | ) | const [virtual] |
Returns TRUE if object is a real message (not KMMsgInfo or KMMsgBase).
Reimplemented in KMMessage.
Definition at line 119 of file kmmsgbase.cpp.
bool KMMsgBase::isNew | ( | void | ) | const [virtual] |
bool KMMsgBase::isOfUnknownStatus | ( | void | ) | const [virtual] |
bool KMMsgBase::isOld | ( | void | ) | const [virtual] |
bool KMMsgBase::isQueued | ( | void | ) | const [virtual] |
bool KMMsgBase::isRead | ( | void | ) | const [virtual] |
bool KMMsgBase::isReplied | ( | void | ) | const [virtual] |
bool KMMsgBase::isSent | ( | void | ) | const [virtual] |
bool KMMsgBase::isSpam | ( | void | ) | const [virtual] |
bool KMMsgBase::isTodo | ( | void | ) | const [virtual] |
bool KMMsgBase::isUnread | ( | void | ) | const [virtual] |
Returns TRUE if status unread.
Note that new messages are not unread.
Definition at line 356 of file kmmsgbase.cpp.
bool KMMsgBase::isWatched | ( | void | ) | const [virtual] |
virtual KMMsgMDNSentState KMMsgBase::mdnSentState | ( | ) | const [pure virtual] |
virtual QString KMMsgBase::msgIdMD5 | ( | void | ) | const [pure virtual] |
virtual size_t KMMsgBase::msgSize | ( | void | ) | const [pure virtual] |
virtual size_t KMMsgBase::msgSizeServer | ( | void | ) | const [pure virtual] |
KMFolder* KMMsgBase::parent | ( | void | ) | const [inline] |
void KMMsgBase::readConfig | ( | void | ) | [static] |
Reads config settings from group "Composer" and sets all internal variables (e.g.
indent-prefix, etc.)
Reimplemented in KMMessage.
Definition at line 1404 of file kmmsgbase.cpp.
QString KMMsgBase::replacePrefixes | ( | const QString & | str, | |
const QStringList & | prefixRegExps, | |||
bool | replace, | |||
const QString & | newPrefix | |||
) | [static] |
Check for prefixes prefixRegExps
in str
.
If none is found, newPrefix
+ ' ' is prepended to str
and the resulting string is returned. If replace
is true, any sequence of whitespace-delimited prefixes at the beginning of str
is replaced by newPrefix
.
Definition at line 1427 of file kmmsgbase.cpp.
QString KMMsgBase::replySubject | ( | ) | const |
Return this mails subject, formatted for "reply" mails.
Definition at line 1481 of file kmmsgbase.cpp.
virtual QString KMMsgBase::replyToAuxIdMD5 | ( | ) | const [pure virtual] |
virtual QString KMMsgBase::replyToIdMD5 | ( | void | ) | const [pure virtual] |
virtual void KMMsgBase::setDate | ( | time_t | aUnixTime | ) | [pure virtual] |
void KMMsgBase::setDate | ( | const QCString & | aStrDate | ) | [virtual] |
void KMMsgBase::setDirty | ( | bool | b | ) | [inline] |
virtual void KMMsgBase::setEnableUndo | ( | bool | enable | ) | [inline, virtual] |
Definition at line 383 of file kmmsgbase.h.
void KMMsgBase::setEncryptionState | ( | const | KMMsgEncryptionState, | |
int | idx = -1 | |||
) | [virtual] |
Set encryption status of the message and mark dirty.
Optional optimization: idx
may specify the index of this message within the parent folder.
Reimplemented in KMMessage, and KMMsgInfo.
Definition at line 300 of file kmmsgbase.cpp.
void KMMsgBase::setEncryptionStateChar | ( | QChar | status, | |
int | idx = -1 | |||
) | [virtual] |
Set encryption status of the message and mark dirty.
Optional optimization: idx
may specify the index of this message within the parent folder.
Definition at line 308 of file kmmsgbase.cpp.
virtual void KMMsgBase::setFileName | ( | const QString & | filename | ) | [pure virtual] |
virtual void KMMsgBase::setFolderOffset | ( | off_t | offs | ) | [pure virtual] |
virtual void KMMsgBase::setIndexLength | ( | short | len | ) | [inline, virtual] |
virtual void KMMsgBase::setIndexOffset | ( | off_t | off | ) | [inline, virtual] |
void KMMsgBase::setMDNSentState | ( | KMMsgMDNSentState | status, | |
int | idx = -1 | |||
) | [virtual] |
Set "MDN sent" status of the message.
Reimplemented in KMMessage, and KMMsgInfo.
Definition at line 333 of file kmmsgbase.cpp.
virtual void KMMsgBase::setMsgSize | ( | size_t | sz | ) | [pure virtual] |
virtual void KMMsgBase::setMsgSizeServer | ( | size_t | sz | ) | [pure virtual] |
void KMMsgBase::setParent | ( | KMFolder * | p | ) | [inline] |
void KMMsgBase::setSignatureState | ( | const | KMMsgSignatureState, | |
int | idx = -1 | |||
) | [virtual] |
Set signature status of the message and mark dirty.
Optional optimization: idx
may specify the index of this message within the parent folder.
Reimplemented in KMMessage, and KMMsgInfo.
Definition at line 325 of file kmmsgbase.cpp.
void KMMsgBase::setSignatureStateChar | ( | QChar | status, | |
int | idx = -1 | |||
) | [virtual] |
Set signature status of the message and mark dirty.
Optional optimization: idx
may specify the index of this message within the parent folder.
Definition at line 339 of file kmmsgbase.cpp.
void KMMsgBase::setStatus | ( | const char * | statusField, | |
const char * | xstatusField = 0 | |||
) | [virtual] |
void KMMsgBase::setStatus | ( | const KMMsgStatus | status, | |
int | idx = -1 | |||
) | [virtual] |
Set status and mark dirty.
Optional optimization: idx
may specify the index of this message within the parent folder.
Reimplemented in KMMessage, and KMMsgInfo.
Definition at line 153 of file kmmsgbase.cpp.
virtual void KMMsgBase::setSubject | ( | const QString & | ) | [pure virtual] |
virtual void KMMsgBase::setUID | ( | ulong | uid | ) | [pure virtual] |
virtual void KMMsgBase::setXMark | ( | const QString & | ) | [pure virtual] |
virtual KMMsgSignatureState KMMsgBase::signatureState | ( | ) | const [pure virtual] |
QString KMMsgBase::skipKeyword | ( | const QString & | str, | |
QChar | sepChar = ':' , |
|||
bool * | keywordFound = 0 | |||
) | [static] |
Skip leading keyword if keyword has given character at it's end (e.g.
':' or ',') and skip the then following blanks (if any) too. If keywordFound is specified it will be TRUE if a keyword was skipped and FALSE otherwise.
Definition at line 538 of file kmmsgbase.cpp.
virtual KMMsgStatus KMMsgBase::status | ( | void | ) | const [pure virtual] |
QString KMMsgBase::statusToSortRank | ( | ) |
QCString KMMsgBase::statusToStr | ( | const KMMsgStatus | status | ) | [static] |
KMFolderIndex * KMMsgBase::storage | ( | ) | const |
Returns str
with all "forward" and "reply" prefixes stripped off.
Definition at line 1419 of file kmmsgbase.cpp.
virtual QString KMMsgBase::strippedSubjectMD5 | ( | ) | const [pure virtual] |
virtual QString KMMsgBase::subject | ( | void | ) | const [pure virtual] |
virtual bool KMMsgBase::subjectIsPrefixed | ( | ) | const [pure virtual] |
QStringList KMMsgBase::supportedEncodings | ( | bool | usAscii | ) | [static] |
bool KMMsgBase::syncIndexString | ( | ) | const |
void KMMsgBase::toggleStatus | ( | const KMMsgStatus | status, | |
int | idx = -1 | |||
) | [virtual] |
Definition at line 124 of file kmmsgbase.cpp.
virtual QString KMMsgBase::toStrip | ( | void | ) | const [pure virtual] |
Convert all non-ascii characters to question marks If ok is non-null, *ok will be set to true if all characters where ascii, *ok will be set to false otherwise.
Definition at line 576 of file kmmsgbase.cpp.
virtual ulong KMMsgBase::UID | ( | void | ) | const [pure virtual] |
virtual QString KMMsgBase::xmark | ( | void | ) | const [pure virtual] |
Member Data Documentation
bool KMMsgBase::mDirty [protected] |
Definition at line 430 of file kmmsgbase.h.
bool KMMsgBase::mEnableUndo [protected] |
Definition at line 431 of file kmmsgbase.h.
short KMMsgBase::mIndexLength [protected] |
Definition at line 429 of file kmmsgbase.h.
off_t KMMsgBase::mIndexOffset [protected] |
Definition at line 428 of file kmmsgbase.h.
KMLegacyMsgStatus KMMsgBase::mLegacyStatus [mutable, protected] |
Definition at line 435 of file kmmsgbase.h.
KMFolder* KMMsgBase::mParent [protected] |
Definition at line 427 of file kmmsgbase.h.
KMMsgStatus KMMsgBase::mStatus [mutable, protected] |
Definition at line 432 of file kmmsgbase.h.
The documentation for this class was generated from the following files: