kmail
KMFolderIndex Class Reference
A FolderStorage with an index for faster access to often used message properties. More...
#include <kmfolderindex.h>
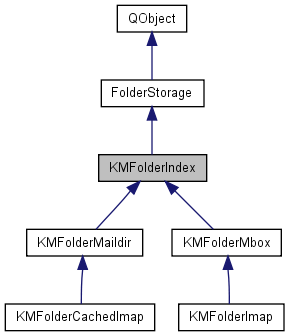
Public Types | |
enum | IndexStatus { IndexOk, IndexMissing, IndexTooOld } |
Public Slots | |
virtual int | updateIndex () |
Public Member Functions | |
virtual void | clearIndex (bool autoDelete=true, bool syncDict=false) |
virtual int | count (bool cache=false) const |
int | find (const KMMessage *msg) const |
virtual int | find (const KMMsgBase *msg) const |
virtual KMMsgBase * | getMsgBase (int idx) |
virtual const KMMsgBase * | getMsgBase (int idx) const |
virtual QString | indexLocation () const |
int | indexSizeOfLong () |
uchar * | indexStreamBasePtr () |
bool | indexSwapByteOrder () |
KMFolderIndex (KMFolder *folder, const char *name=0) | |
void | recreateIndex () |
int | serialIndexId () const |
virtual KMMsgInfo * | setIndexEntry (int idx, KMMessage *msg) |
virtual KMMsgBase * | takeIndexEntry (int idx) |
virtual void | truncateIndex () |
virtual int | writeIndex (bool createEmptyIndex=false) |
virtual | ~KMFolderIndex () |
Protected Member Functions | |
virtual int | createIndexFromContents ()=0 |
virtual void | fillMessageDict () |
virtual IndexStatus | indexStatus ()=0 |
bool | readIndex () |
bool | readIndexHeader (int *gv=0) |
bool | updateIndexStreamPtr (bool just_close=FALSE) |
Protected Attributes | |
off_t | mHeaderOffset |
int | mIndexId |
int | mIndexSizeOfLong |
FILE * | mIndexStream |
uchar * | mIndexStreamPtr |
int | mIndexStreamPtrLength |
bool | mIndexSwapByteOrder |
KMMsgList | mMsgList |
Detailed Description
A FolderStorage with an index for faster access to often used message properties.This class represents a message store which has an index for providing fast access to often used message properties, namely those displayed in the list of messages (KMHeaders).
Definition at line 37 of file kmfolderindex.h.
Member Enumeration Documentation
This enum indicates the status of the index file.
It's returned by indexStatus().
Definition at line 49 of file kmfolderindex.h.
Constructor & Destructor Documentation
KMFolderIndex::KMFolderIndex | ( | KMFolder * | folder, | |
const char * | name = 0 | |||
) |
Usually a parent is given.
But in some cases there is no fitting parent object available. Then the name of the folder is used as the absolute path to the folder file.
Definition at line 75 of file kmfolderindex.cpp.
KMFolderIndex::~KMFolderIndex | ( | ) | [virtual] |
Definition at line 88 of file kmfolderindex.cpp.
Member Function Documentation
void KMFolderIndex::clearIndex | ( | bool | autoDelete = true , |
|
bool | syncDict = false | |||
) | [virtual] |
int KMFolderIndex::count | ( | bool | cache = false |
) | const [virtual] |
Number of messages in this folder.
Reimplemented from FolderStorage.
Definition at line 298 of file kmfolderindex.cpp.
virtual int KMFolderIndex::createIndexFromContents | ( | ) | [protected, pure virtual] |
Create index file from messages file and fill the message-info list mMsgList.
Returns 0 on success and an errno value (see fopen) on failure.
Implemented in KMFolderCachedImap, KMFolderMaildir, and KMFolderMbox.
void KMFolderIndex::fillMessageDict | ( | ) | [protected, virtual] |
Inserts messages into the message dictionary by iterating over the message list.
The messages will get new serial numbers. This is only used on newly appeared folders, where there is no .ids file yet, or when that has been invalidated.
Reimplemented from FolderStorage.
Definition at line 464 of file kmfolderindex.cpp.
int KMFolderIndex::find | ( | const KMMessage * | msg | ) | const [inline] |
virtual int KMFolderIndex::find | ( | const KMMsgBase * | msg | ) | const [inline, virtual] |
Returns the index of the given message or -1 if not found.
Implements FolderStorage.
Definition at line 69 of file kmfolderindex.h.
virtual KMMsgBase* KMFolderIndex::getMsgBase | ( | int | idx | ) | [inline, virtual] |
virtual const KMMsgBase* KMFolderIndex::getMsgBase | ( | int | idx | ) | const [inline, virtual] |
Provides access to the basic message fields that are also stored in the index.
Whenever you only need subject, from, date, status you should use this method instead of getMsg() because getMsg() will load the message if necessary and this method does not.
Implements FolderStorage.
Definition at line 66 of file kmfolderindex.h.
QString KMFolderIndex::indexLocation | ( | ) | const [virtual] |
Returns full path to index file.
Implements FolderStorage.
Definition at line 93 of file kmfolderindex.cpp.
int KMFolderIndex::indexSizeOfLong | ( | ) | [inline] |
Definition at line 78 of file kmfolderindex.h.
KMFolderIndex::IndexStatus KMFolderIndex::indexStatus | ( | ) | [protected, pure virtual] |
Tests whether the contents of this folder is newer than the index.
Should return IndexTooOld if the index is older than the contents. Should return IndexMissing if there is contents but no index. Should return IndexOk if the folder doesn't exist anymore "physically" or if the index is not older than the contents.
Implemented in KMFolderMbox.
Definition at line 435 of file kmfolderindex.cpp.
uchar* KMFolderIndex::indexStreamBasePtr | ( | ) | [inline] |
Definition at line 75 of file kmfolderindex.h.
bool KMFolderIndex::indexSwapByteOrder | ( | ) | [inline] |
Definition at line 77 of file kmfolderindex.h.
bool KMFolderIndex::readIndex | ( | ) | [protected, virtual] |
Read index file and fill the message-info list mMsgList.
Implements FolderStorage.
Definition at line 213 of file kmfolderindex.cpp.
bool KMFolderIndex::readIndexHeader | ( | int * | gv = 0 |
) | [protected] |
Read index header.
Called from within readIndex().
Definition at line 307 of file kmfolderindex.cpp.
void KMFolderIndex::recreateIndex | ( | ) |
Definition at line 487 of file kmfolderindex.cpp.
int KMFolderIndex::serialIndexId | ( | ) | const [inline] |
virtual KMMsgBase* KMFolderIndex::takeIndexEntry | ( | int | idx | ) | [inline, virtual] |
void KMFolderIndex::truncateIndex | ( | ) | [virtual] |
int KMFolderIndex::updateIndex | ( | ) | [virtual, slot] |
Incrementally update the index if possible else call writeIndex.
Implements FolderStorage.
Definition at line 107 of file kmfolderindex.cpp.
bool KMFolderIndex::updateIndexStreamPtr | ( | bool | just_close = FALSE |
) | [protected] |
Definition at line 390 of file kmfolderindex.cpp.
int KMFolderIndex::writeIndex | ( | bool | createEmptyIndex = false |
) | [virtual] |
Write index to index-file.
Returns 0 on success and errno error on failure.
Implements FolderStorage.
Definition at line 123 of file kmfolderindex.cpp.
Member Data Documentation
off_t KMFolderIndex::mHeaderOffset [protected] |
int KMFolderIndex::mIndexId [protected] |
Definition at line 125 of file kmfolderindex.h.
int KMFolderIndex::mIndexSizeOfLong [protected] |
Definition at line 127 of file kmfolderindex.h.
FILE* KMFolderIndex::mIndexStream [protected] |
uchar* KMFolderIndex::mIndexStreamPtr [protected] |
Definition at line 124 of file kmfolderindex.h.
int KMFolderIndex::mIndexStreamPtrLength [protected] |
Definition at line 125 of file kmfolderindex.h.
bool KMFolderIndex::mIndexSwapByteOrder [protected] |
Definition at line 126 of file kmfolderindex.h.
KMMsgList KMFolderIndex::mMsgList [protected] |
The documentation for this class was generated from the following files: