kmail
KMFolderMbox Class Reference
#include <kmfoldermbox.h>
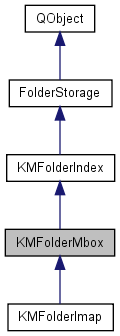
Public Member Functions | |
virtual int | addMsg (KMMessage *msg, int *index_return=0) |
virtual int | canAccess () |
int | compact (unsigned int startIndex, int nbMessages, FILE *tmpFile, off_t &offs, bool &done) |
virtual int | compact (bool silent) |
virtual int | create () |
virtual Q_INT64 | doFolderSize () const |
virtual KMFolderType | folderType () const |
DwString | getDwString (int idx) |
virtual IndexStatus | indexStatus () |
bool | isLocked () const |
virtual bool | isReadOnly () const |
KMFolderMbox (KMFolder *folder, const char *name=0) | |
virtual int | open (const char *owner) |
virtual void | reallyDoClose (const char *owner) |
void | setLockType (LockType ltype=FCNTL) |
void | setProcmailLockFileName (const QString &) |
virtual void | sync () |
virtual | ~KMFolderMbox () |
Static Public Member Functions | |
static QByteArray | escapeFrom (const DwString &str) |
Protected Member Functions | |
virtual int | createIndexFromContents () |
virtual FolderJob * | doCreateJob (QPtrList< KMMessage > &msgList, const QString &sets, FolderJob::JobType jt, KMFolder *folder) const |
virtual FolderJob * | doCreateJob (KMMessage *msg, FolderJob::JobType jt, KMFolder *folder, QString partSpecifier, const AttachmentStrategy *as) const |
virtual int | expungeContents () |
virtual int | lock () |
virtual KMMessage * | readMsg (int idx) |
virtual int | removeContents () |
virtual int | unlock () |
Detailed Description
Definition at line 47 of file kmfoldermbox.h.
Constructor & Destructor Documentation
KMFolderMbox::KMFolderMbox | ( | KMFolder * | folder, | |
const char * | name = 0 | |||
) |
Usually a parent is given.
But in some cases there is no fitting parent object available. Then the name of the folder is used as the absolute path to the folder file.
Definition at line 75 of file kmfoldermbox.cpp.
KMFolderMbox::~KMFolderMbox | ( | ) | [virtual] |
Definition at line 86 of file kmfoldermbox.cpp.
Member Function Documentation
int KMFolderMbox::addMsg | ( | KMMessage * | msg, | |
int * | index_return = 0 | |||
) | [virtual] |
Add the given message to the folder.
Usually the message is added at the end of the folder. Returns zero on success and an errno error code on failure. The index of the new message is stored in index_return if given. Please note that the message is added as is to the folder and the folder takes ownership of the message (deleting it in the destructor).
Implements FolderStorage.
Reimplemented in KMFolderImap.
Definition at line 929 of file kmfoldermbox.cpp.
int KMFolderMbox::canAccess | ( | ) | [virtual] |
Check folder for permissions Returns zero if readable and writable.
Implements FolderStorage.
Definition at line 198 of file kmfoldermbox.cpp.
int KMFolderMbox::compact | ( | unsigned int | startIndex, | |
int | nbMessages, | |||
FILE * | tmpFile, | |||
off_t & | offs, | |||
bool & | done | |||
) |
Remove some deleted messages from the folder.
Returns zero on success and an errno on failure. This is only for use from MboxCompactionJob.
Definition at line 1145 of file kmfoldermbox.cpp.
int KMFolderMbox::compact | ( | bool | silent | ) | [virtual] |
Remove deleted messages from the folder.
Returns zero on success and an errno on failure.
Implements FolderStorage.
Reimplemented in KMFolderImap.
Definition at line 1222 of file kmfoldermbox.cpp.
int KMFolderMbox::create | ( | ) | [virtual] |
Implements FolderStorage.
Reimplemented in KMFolderImap.
Definition at line 210 of file kmfoldermbox.cpp.
int KMFolderMbox::createIndexFromContents | ( | ) | [protected, virtual] |
Create index file from messages file and fill the message-info list mMsgList.
Returns 0 on success and an errno value (see fopen) on failure.
Implements KMFolderIndex.
Definition at line 540 of file kmfoldermbox.cpp.
FolderJob * KMFolderMbox::doCreateJob | ( | QPtrList< KMMessage > & | msgList, | |
const QString & | sets, | |||
FolderJob::JobType | jt, | |||
KMFolder * | folder | |||
) | const [protected, virtual] |
Implements FolderStorage.
Reimplemented in KMFolderImap.
Definition at line 446 of file kmfoldermbox.cpp.
FolderJob * KMFolderMbox::doCreateJob | ( | KMMessage * | msg, | |
FolderJob::JobType | jt, | |||
KMFolder * | folder, | |||
QString | partSpecifier, | |||
const AttachmentStrategy * | as | |||
) | const [protected, virtual] |
These two methods actually create the jobs.
They have to be implemented in all folders.
- See also:
- createJob
Implements FolderStorage.
Reimplemented in KMFolderImap.
Definition at line 436 of file kmfoldermbox.cpp.
Q_INT64 KMFolderMbox::doFolderSize | ( | ) | const [virtual] |
QByteArray KMFolderMbox::escapeFrom | ( | const DwString & | str | ) | [static] |
Definition at line 865 of file kmfoldermbox.cpp.
int KMFolderMbox::expungeContents | ( | ) | [protected, virtual] |
Called by KMFolder::expunge() to delete the actual contents.
At the time of the call the folder has already been closed, and the various index files deleted. Returns 0 on success.
Implements FolderStorage.
Reimplemented in KMFolderImap.
Definition at line 1261 of file kmfoldermbox.cpp.
virtual KMFolderType KMFolderMbox::folderType | ( | ) | const [inline, virtual] |
Returns the type of this folder.
Reimplemented from FolderStorage.
Reimplemented in KMFolderImap.
Definition at line 61 of file kmfoldermbox.h.
DwString KMFolderMbox::getDwString | ( | int | idx | ) | [virtual] |
Read a message and return it as a string.
Implements FolderStorage.
Definition at line 904 of file kmfoldermbox.cpp.
KMFolderIndex::IndexStatus KMFolderMbox::indexStatus | ( | ) | [virtual] |
Tests whether the contents of this folder is newer than the index.
Should return IndexTooOld if the index is older than the contents. Should return IndexMissing if there is contents but no index. Should return IndexOk if the folder doesn't exist anymore "physically" or if the index is not older than the contents.
Implements KMFolderIndex.
Definition at line 522 of file kmfoldermbox.cpp.
bool KMFolderMbox::isLocked | ( | ) | const [inline] |
virtual bool KMFolderMbox::isReadOnly | ( | ) | const [inline, virtual] |
Is the folder read-only?
Implements FolderStorage.
Reimplemented in KMFolderImap.
Definition at line 101 of file kmfoldermbox.h.
int KMFolderMbox::lock | ( | ) | [protected, virtual] |
Lock mail folder files.
Called by open(). Returns 0 on success and an errno error code on failure.
Definition at line 303 of file kmfoldermbox.cpp.
int KMFolderMbox::open | ( | const char * | owner | ) | [virtual] |
Open folder for access.
Does nothing if the folder is already opened. To reopen a folder call close() first. Returns zero on success and an error code equal to the c-library fopen call otherwise (errno).
Implements FolderStorage.
Definition at line 95 of file kmfoldermbox.cpp.
KMMessage * KMFolderMbox::readMsg | ( | int | idx | ) | [protected, virtual] |
Load message from file and store it at given index.
Returns 0 on failure.
Implements FolderStorage.
Definition at line 813 of file kmfoldermbox.cpp.
void KMFolderMbox::reallyDoClose | ( | const char * | owner | ) | [virtual] |
Implements FolderStorage.
Reimplemented in KMFolderImap.
Definition at line 259 of file kmfoldermbox.cpp.
int KMFolderMbox::removeContents | ( | ) | [protected, virtual] |
Called by KMFolder::remove() to delete the actual contents.
At the time of the call the folder has already been closed, and the various index files deleted. Returns 0 on success.
Implements FolderStorage.
Definition at line 1253 of file kmfoldermbox.cpp.
void KMFolderMbox::setLockType | ( | LockType | ltype = FCNTL |
) |
Definition at line 1241 of file kmfoldermbox.cpp.
void KMFolderMbox::setProcmailLockFileName | ( | const QString & | fname | ) |
Definition at line 1247 of file kmfoldermbox.cpp.
void KMFolderMbox::sync | ( | ) | [virtual] |
int KMFolderMbox::unlock | ( | ) | [protected, virtual] |
Unlock mail folder files.
Called by close(). Returns 0 on success and an errno error code on failure.
Definition at line 455 of file kmfoldermbox.cpp.
The documentation for this class was generated from the following files: