kmail
mailinglist-magic.cpp File Reference
#include "mailinglist-magic.h"
#include "kmmessage.h"
#include <kconfig.h>
#include <kurl.h>
#include <kdebug.h>
#include <qstringlist.h>
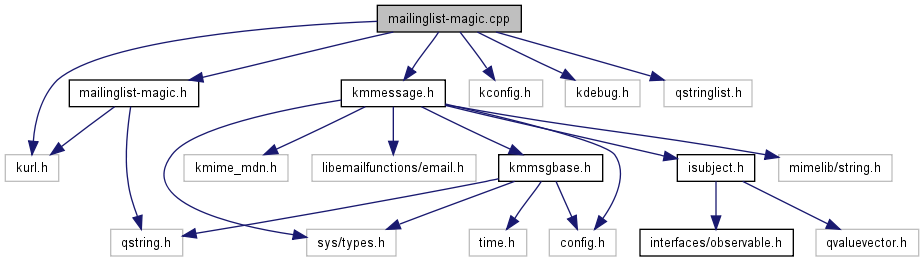
Go to the source code of this file.
Typedefs | |
typedef QString(* | MagicDetectorFunc )(const KMMessage *, QCString &, QString &) |
Functions | |
static QString | check_delivered_to (const KMMessage *message, QCString &header_name, QString &header_value) |
static QString | check_list_id (const KMMessage *message, QCString &header_name, QString &header_value) |
static QString | check_list_post (const KMMessage *message, QCString &header_name, QString &header_value) |
static QString | check_mailing_list (const KMMessage *message, QCString &header_name, QString &header_value) |
static QString | check_sender (const KMMessage *message, QCString &header_name, QString &header_value) |
static QString | check_x_beenthere (const KMMessage *message, QCString &header_name, QString &header_value) |
static QString | check_x_loop (const KMMessage *message, QCString &header_name, QString &header_value) |
static QString | check_x_mailing_list (const KMMessage *message, QCString &header_name, QString &header_value) |
static QString | check_x_ml_name (const KMMessage *message, QCString &header_name, QString &header_value) |
static QStringList | headerToAddress (const QString &header) |
Variables | |
MagicDetectorFunc | magic_detector [] |
static const int | num_detectors = sizeof (magic_detector) / sizeof (magic_detector[0]) |
Typedef Documentation
typedef QString(* MagicDetectorFunc)(const KMMessage *, QCString &, QString &) |
Definition at line 18 of file mailinglist-magic.cpp.
Function Documentation
static QString check_delivered_to | ( | const KMMessage * | message, | |
QCString & | header_name, | |||
QString & | header_value | |||
) | [static] |
Definition at line 65 of file mailinglist-magic.cpp.
static QString check_list_id | ( | const KMMessage * | message, | |
QCString & | header_name, | |||
QString & | header_value | |||
) | [static] |
Definition at line 102 of file mailinglist-magic.cpp.
static QString check_list_post | ( | const KMMessage * | message, | |
QCString & | header_name, | |||
QString & | header_value | |||
) | [static] |
Definition at line 127 of file mailinglist-magic.cpp.
static QString check_mailing_list | ( | const KMMessage * | message, | |
QCString & | header_name, | |||
QString & | header_value | |||
) | [static] |
Definition at line 147 of file mailinglist-magic.cpp.
static QString check_sender | ( | const KMMessage * | message, | |
QCString & | header_name, | |||
QString & | header_value | |||
) | [static] |
Definition at line 21 of file mailinglist-magic.cpp.
static QString check_x_beenthere | ( | const KMMessage * | message, | |
QCString & | header_name, | |||
QString & | header_value | |||
) | [static] |
Definition at line 50 of file mailinglist-magic.cpp.
static QString check_x_loop | ( | const KMMessage * | message, | |
QCString & | header_name, | |||
QString & | header_value | |||
) | [static] |
Definition at line 166 of file mailinglist-magic.cpp.
static QString check_x_mailing_list | ( | const KMMessage * | message, | |
QCString & | header_name, | |||
QString & | header_value | |||
) | [static] |
Definition at line 81 of file mailinglist-magic.cpp.
static QString check_x_ml_name | ( | const KMMessage * | message, | |
QCString & | header_name, | |||
QString & | header_value | |||
) | [static] |
Definition at line 183 of file mailinglist-magic.cpp.
static QStringList headerToAddress | ( | const QString & | header | ) | [static] |
Definition at line 212 of file mailinglist-magic.cpp.
Variable Documentation
Initial value:
{ check_list_id, check_list_post, check_sender, check_x_mailing_list, check_mailing_list, check_delivered_to, check_x_beenthere, check_x_loop, check_x_ml_name }
Definition at line 196 of file mailinglist-magic.cpp.
const int num_detectors = sizeof (magic_detector) / sizeof (magic_detector[0]) [static] |
Definition at line 209 of file mailinglist-magic.cpp.