Kate
#include <katevinormalmode.h>
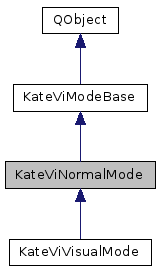
Protected Types | |
enum | PasteLocation { AtCurrentPosition, AfterCurrentPosition } |
Protected Member Functions | |
Cursor | cursorPosAtEndOfPaste (const Cursor &pasteLocation, const QString &pastedText) |
void | executeCommand (const KateViCommand *cmd) |
QRegExp | generateMatchingItemRegex () |
OperationMode | getOperationMode () const |
virtual void | goToPos (const KateViRange &r) |
KTextEditor::MovingRange *& | highlightedYankForDocument () |
void | highlightYank (const KateViRange &range) |
void | initializeCommands () |
void | joinLines (unsigned int from, unsigned int to) const |
bool | motionWillBeUsedWithCommand () |
bool | paste (KateViNormalMode::PasteLocation pasteLocation, bool isgPaste, bool isIndentedPaste) |
void | reformatLines (unsigned int from, unsigned int to) const |
void | resetParser () |
void | shrinkRangeAroundCursor (KateViRange &toShrink, const KateViRange &rangeToShrinkTo) |
KateViRange | textObjectComma (bool inner) |
bool | waitingForRegisterOrCharToSearch () |
![]() | |
void | addJump (KTextEditor::Cursor cursor) |
void | addToNumberUnderCursor (int count) |
bool | deleteRange (KateViRange &r, OperationMode mode=LineWise, bool addToRegister=true) |
KateDocument * | doc () const |
void | fillRegister (const QChar ®, const QString &text, OperationMode flag=CharWise) |
int | findLineStartingWitchChar (const QChar &c, unsigned int count, bool forward=true) const |
Cursor | findNextWordStart (int fromLine, int fromColumn, bool onlyCurrentLine=false) const |
Cursor | findNextWORDStart (int fromLine, int fromColumn, bool onlyCurrentLine=false) const |
KateViRange | findPatternForMotion (const QString &pattern, bool backwards, bool caseSensitive, const Cursor &startFrom, int count=1) const |
Cursor | findPrevWordEnd (int fromLine, int fromColumn, bool onlyCurrentLine=false) const |
Cursor | findPrevWORDEnd (int fromLine, int fromColumn, bool onlyCurrentLine=false) const |
Cursor | findPrevWordStart (int fromLine, int fromColumn, bool onlyCurrentLine=false) const |
Cursor | findPrevWORDStart (int fromLine, int fromColumn, bool onlyCurrentLine=false) const |
KateViRange | findSurrounding (const QRegExp &c1, const QRegExp &c2, bool inner=false) const |
KateViRange | findSurroundingBrackets (const QChar &c1, const QChar &c2, bool inner, const QChar &nested1, const QChar &nested2) const |
KateViRange | findSurroundingQuotes (const QChar &c, bool inner=false) const |
Cursor | findWordEnd (int fromLine, int fromColumn, bool onlyCurrentLine=false) const |
Cursor | findWORDEnd (int fromLine, int fromColumn, bool onlyCurrentLine=false) const |
const QChar | getCharAtVirtualColumn (QString &line, int virtualColumn, int tabWidht) const |
const QChar | getCharUnderCursor () const |
QChar | getChosenRegister (const QChar &defaultReg) const |
unsigned int | getCount () const |
const QString | getLine (int lineNumber=-1) const |
KTextEditor::Cursor | getNextJump (KTextEditor::Cursor) |
KTextEditor::Cursor | getPrevJump (KTextEditor::Cursor) |
const QString | getRange (KateViRange &r, OperationMode mode=LineWise) const |
QString | getRegisterContent (const QChar ®) |
OperationMode | getRegisterFlag (const QChar ®) const |
const Range | getWordRangeUnderCursor () const |
const QString | getWordUnderCursor () const |
KateViRange | goLineDown () |
KateViRange | goLineUp () |
KateViRange | goLineUpDown (int lines) |
KateViRange | goVisualLineUpDown (int lines) |
bool | isCounted () |
unsigned int | linesDisplayed () |
void | scrollViewLines (int l) |
bool | startInsertMode () |
bool | startNormalMode () |
bool | startReplaceMode () |
bool | startVisualBlockMode () |
bool | startVisualLineMode () |
bool | startVisualMode () |
void | switchView (Direction direction=Next) |
void | updateCursor (const Cursor &c) const |
void | yankToClipBoard (QChar chosen_register, QString text) |
Detailed Description
Commands for the vi normal mode.
Definition at line 49 of file katevinormalmode.h.
Member Enumeration Documentation
|
protected |
Enumerator | |
---|---|
AtCurrentPosition | |
AfterCurrentPosition |
Definition at line 287 of file katevinormalmode.h.
Constructor & Destructor Documentation
KateViNormalMode::KateViNormalMode | ( | KateViInputModeManager * | viInputModeManager, |
KateView * | view, | ||
KateViewInternal * | viewInternal | ||
) |
Definition at line 53 of file katevinormalmode.cpp.
|
virtual |
Definition at line 98 of file katevinormalmode.cpp.
Member Function Documentation
void KateViNormalMode::addCurrentPositionToJumpList | ( | ) |
Definition at line 547 of file katevinormalmode.cpp.
void KateViNormalMode::beginMonitoringDocumentChanges | ( | ) |
Definition at line 485 of file katevinormalmode.cpp.
bool KateViNormalMode::commandAbort | ( | ) |
Definition at line 1602 of file katevinormalmode.cpp.
bool KateViNormalMode::commandAddToNumber | ( | ) |
Definition at line 1668 of file katevinormalmode.cpp.
bool KateViNormalMode::commandAlignLine | ( | ) |
Definition at line 1645 of file katevinormalmode.cpp.
bool KateViNormalMode::commandAlignLines | ( | ) |
Definition at line 1655 of file katevinormalmode.cpp.
bool KateViNormalMode::commandAppendToBlock | ( | ) |
Definition at line 1697 of file katevinormalmode.cpp.
bool KateViNormalMode::commandCentreViewOnCursor | ( | ) |
Definition at line 1592 of file katevinormalmode.cpp.
bool KateViNormalMode::commandChange | ( | ) |
Definition at line 1105 of file katevinormalmode.cpp.
bool KateViNormalMode::commandChangeCase | ( | ) |
Definition at line 910 of file katevinormalmode.cpp.
bool KateViNormalMode::commandChangeCaseRange | ( | ) |
Definition at line 984 of file katevinormalmode.cpp.
bool KateViNormalMode::commandChangeLine | ( | ) |
Definition at line 1156 of file katevinormalmode.cpp.
bool KateViNormalMode::commandChangeToEOL | ( | ) |
Definition at line 1144 of file katevinormalmode.cpp.
bool KateViNormalMode::commandCollapseLocal | ( | ) |
Definition at line 1839 of file katevinormalmode.cpp.
bool KateViNormalMode::commandCollapseToplevelNodes | ( | ) |
Definition at line 1805 of file katevinormalmode.cpp.
bool KateViNormalMode::commandDelete | ( | ) |
Definition at line 753 of file katevinormalmode.cpp.
bool KateViNormalMode::commandDeleteChar | ( | ) |
Definition at line 1324 of file katevinormalmode.cpp.
bool KateViNormalMode::commandDeleteCharBackward | ( | ) |
Definition at line 1349 of file katevinormalmode.cpp.
bool KateViNormalMode::commandDeleteLine | ( | ) |
Definition at line 720 of file katevinormalmode.cpp.
bool KateViNormalMode::commandDeleteToEOL | ( | ) |
Definition at line 759 of file katevinormalmode.cpp.
bool KateViNormalMode::commandEnterInsertMode | ( | ) |
enter insert mode at the cursor position
Definition at line 559 of file katevinormalmode.cpp.
bool KateViNormalMode::commandEnterInsertModeAppend | ( | ) |
enter insert mode after the current character
Definition at line 570 of file katevinormalmode.cpp.
bool KateViNormalMode::commandEnterInsertModeAppendEOL | ( | ) |
start insert mode after the last character of the line
Definition at line 596 of file katevinormalmode.cpp.
bool KateViNormalMode::commandEnterInsertModeBeforeFirstNonBlankInLine | ( | ) |
Definition at line 607 of file katevinormalmode.cpp.
bool KateViNormalMode::commandEnterInsertModeLast | ( | ) |
enter insert mode at the last insert position
Definition at line 627 of file katevinormalmode.cpp.
bool KateViNormalMode::commandEnterReplaceMode | ( | ) |
Definition at line 715 of file katevinormalmode.cpp.
bool KateViNormalMode::commandEnterVisualBlockMode | ( | ) |
Definition at line 648 of file katevinormalmode.cpp.
bool KateViNormalMode::commandEnterVisualLineMode | ( | ) |
Definition at line 638 of file katevinormalmode.cpp.
bool KateViNormalMode::commandEnterVisualMode | ( | ) |
Definition at line 693 of file katevinormalmode.cpp.
bool KateViNormalMode::commandExpandAll | ( | ) |
Definition at line 1849 of file katevinormalmode.cpp.
bool KateViNormalMode::commandExpandLocal | ( | ) |
Definition at line 1857 of file katevinormalmode.cpp.
bool KateViNormalMode::commandFormatLine | ( | ) |
Definition at line 1790 of file katevinormalmode.cpp.
bool KateViNormalMode::commandFormatLines | ( | ) |
Definition at line 1799 of file katevinormalmode.cpp.
bool KateViNormalMode::commandGoToNextJump | ( | ) |
Definition at line 1721 of file katevinormalmode.cpp.
bool KateViNormalMode::commandGoToPrevJump | ( | ) |
Definition at line 1728 of file katevinormalmode.cpp.
bool KateViNormalMode::commandgPaste | ( | ) |
Definition at line 1302 of file katevinormalmode.cpp.
bool KateViNormalMode::commandgPasteBefore | ( | ) |
Definition at line 1309 of file katevinormalmode.cpp.
bool KateViNormalMode::commandIndentedPaste | ( | ) |
Definition at line 1314 of file katevinormalmode.cpp.
bool KateViNormalMode::commandIndentedPasteBefore | ( | ) |
Definition at line 1319 of file katevinormalmode.cpp.
bool KateViNormalMode::commandIndentLine | ( | ) |
Definition at line 1502 of file katevinormalmode.cpp.
bool KateViNormalMode::commandIndentLines | ( | ) |
Definition at line 1524 of file katevinormalmode.cpp.
bool KateViNormalMode::commandJoinLines | ( | ) |
Definition at line 1051 of file katevinormalmode.cpp.
bool KateViNormalMode::commandMakeLowercase | ( | ) |
Definition at line 820 of file katevinormalmode.cpp.
bool KateViNormalMode::commandMakeLowercaseLine | ( | ) |
Definition at line 845 of file katevinormalmode.cpp.
bool KateViNormalMode::commandMakeUppercase | ( | ) |
Definition at line 863 of file katevinormalmode.cpp.
bool KateViNormalMode::commandMakeUppercaseLine | ( | ) |
Definition at line 892 of file katevinormalmode.cpp.
bool KateViNormalMode::commandOpenNewLineOver | ( | ) |
Definition at line 1024 of file katevinormalmode.cpp.
bool KateViNormalMode::commandOpenNewLineUnder | ( | ) |
Definition at line 1004 of file katevinormalmode.cpp.
bool KateViNormalMode::commandPaste | ( | ) |
Definition at line 1286 of file katevinormalmode.cpp.
bool KateViNormalMode::commandPasteBefore | ( | ) |
Definition at line 1292 of file katevinormalmode.cpp.
bool KateViNormalMode::commandPrependToBlock | ( | ) |
Definition at line 1682 of file katevinormalmode.cpp.
bool KateViNormalMode::commandPrintCharacterCode | ( | ) |
Definition at line 1609 of file katevinormalmode.cpp.
bool KateViNormalMode::commandRedo | ( | ) |
Definition at line 1486 of file katevinormalmode.cpp.
bool KateViNormalMode::commandRepeatLastChange | ( | ) |
Definition at line 1630 of file katevinormalmode.cpp.
bool KateViNormalMode::commandReplaceCharacter | ( | ) |
Definition at line 1375 of file katevinormalmode.cpp.
bool KateViNormalMode::commandReplayMacro | ( | ) |
Definition at line 1821 of file katevinormalmode.cpp.
bool KateViNormalMode::commandReselectVisual | ( | ) |
Definition at line 658 of file katevinormalmode.cpp.
bool KateViNormalMode::commandScrollHalfPageDown | ( | ) |
Definition at line 1583 of file katevinormalmode.cpp.
bool KateViNormalMode::commandScrollHalfPageUp | ( | ) |
Definition at line 1573 of file katevinormalmode.cpp.
bool KateViNormalMode::commandScrollPageDown | ( | ) |
Definition at line 1553 of file katevinormalmode.cpp.
bool KateViNormalMode::commandScrollPageUp | ( | ) |
Definition at line 1563 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSearchBackward | ( | ) |
Definition at line 1450 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSearchForward | ( | ) |
Definition at line 1465 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSetMark | ( | ) |
Definition at line 1492 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSplitHoriz | ( | ) |
Definition at line 1760 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSplitVert | ( | ) |
Definition at line 1765 of file katevinormalmode.cpp.
bool KateViNormalMode::commandStartRecordingMacro | ( | ) |
Definition at line 1814 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSubstituteChar | ( | ) |
Definition at line 1188 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSubstituteLine | ( | ) |
Definition at line 1198 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSubtractFromNumber | ( | ) |
Definition at line 1675 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSwitchToCmdLine | ( | ) |
Definition at line 1416 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSwitchToDownView | ( | ) |
Definition at line 1740 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSwitchToLeftView | ( | ) |
Definition at line 1735 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSwitchToNextTab | ( | ) |
Definition at line 1770 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSwitchToNextView | ( | ) |
Definition at line 1755 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSwitchToPrevTab | ( | ) |
Definition at line 1780 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSwitchToRightView | ( | ) |
Definition at line 1750 of file katevinormalmode.cpp.
bool KateViNormalMode::commandSwitchToUpView | ( | ) |
Definition at line 1745 of file katevinormalmode.cpp.
bool KateViNormalMode::commandToggleRegionVisibility | ( | ) |
Definition at line 1867 of file katevinormalmode.cpp.
bool KateViNormalMode::commandToOtherEnd | ( | ) |
Definition at line 703 of file katevinormalmode.cpp.
bool KateViNormalMode::commandUndo | ( | ) |
Definition at line 1480 of file katevinormalmode.cpp.
bool KateViNormalMode::commandUnindentLine | ( | ) |
Definition at line 1513 of file katevinormalmode.cpp.
bool KateViNormalMode::commandUnindentLines | ( | ) |
Definition at line 1539 of file katevinormalmode.cpp.
bool KateViNormalMode::commandYank | ( | ) |
Definition at line 1204 of file katevinormalmode.cpp.
bool KateViNormalMode::commandYankLine | ( | ) |
Definition at line 1223 of file katevinormalmode.cpp.
bool KateViNormalMode::commandYankToEOL | ( | ) |
Definition at line 1243 of file katevinormalmode.cpp.
|
protected |
Definition at line 3620 of file katevinormalmode.cpp.
|
protected |
Definition at line 510 of file katevinormalmode.cpp.
|
protected |
Definition at line 3473 of file katevinormalmode.cpp.
|
protected |
Definition at line 3505 of file katevinormalmode.cpp.
|
protectedvirtual |
Definition at line 493 of file katevinormalmode.cpp.
|
virtual |
parses a key stroke to check if it's a valid (part of) a command
- Returns
- true if a command was completed and executed, false otherwise
Implements KateViModeBase.
Definition at line 109 of file katevinormalmode.cpp.
|
protected |
Definition at line 3781 of file katevinormalmode.cpp.
|
protected |
Definition at line 3753 of file katevinormalmode.cpp.
|
protected |
Definition at line 3288 of file katevinormalmode.cpp.
|
protected |
Definition at line 3636 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionDown | ( | ) |
Definition at line 1882 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionDownToFirstNonBlank | ( | ) |
Definition at line 1949 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionFindChar | ( | ) |
Definition at line 2208 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionFindCharBackward | ( | ) |
Definition at line 2239 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionFindNext | ( | ) |
Definition at line 2422 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionFindPrev | ( | ) |
Definition at line 2403 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionLeft | ( | ) |
Definition at line 1892 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionPageDown | ( | ) |
Definition at line 1921 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionPageUp | ( | ) |
Definition at line 1935 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionRepeatlastTF | ( | ) |
Definition at line 2356 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionRepeatlastTFBackward | ( | ) |
Definition at line 2379 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionRight | ( | ) |
Definition at line 1906 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToAfterParagraph | ( | ) |
Definition at line 2884 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToBeforeParagraph | ( | ) |
Definition at line 2856 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToChar | ( | ) |
Definition at line 2277 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToCharBackward | ( | ) |
Definition at line 2315 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToColumn0 | ( | ) |
Definition at line 2186 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToEndOfPrevWord | ( | ) |
Definition at line 2117 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToEndOfPrevWORD | ( | ) |
Definition at line 2144 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToEndOfWord | ( | ) |
Definition at line 2078 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToEndOfWORD | ( | ) |
Definition at line 2095 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToEOL | ( | ) |
Definition at line 2170 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToFirstCharacterOfLine | ( | ) |
Definition at line 2195 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToFirstLineOfWindow | ( | ) |
Definition at line 2792 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToIncrementalSearchMatch | ( | ) |
Definition at line 2915 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToLastLineOfWindow | ( | ) |
Definition at line 2829 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToLineFirst | ( | ) |
Definition at line 2442 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToLineLast | ( | ) |
Definition at line 2455 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToMark | ( | ) |
Definition at line 2488 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToMarkLine | ( | ) |
Definition at line 2515 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToMatchingItem | ( | ) |
Definition at line 2527 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToMiddleLineOfWindow | ( | ) |
Definition at line 2811 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToNextBraceBlockEnd | ( | ) |
Definition at line 2705 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToNextBraceBlockStart | ( | ) |
Definition at line 2649 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToNextOccurrence | ( | ) |
Definition at line 2760 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToNextVisualLine | ( | ) |
Definition at line 2848 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToPreviousBraceBlockEnd | ( | ) |
Definition at line 2736 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToPreviousBraceBlockStart | ( | ) |
Definition at line 2680 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToPrevOccurrence | ( | ) |
Definition at line 2775 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToPrevVisualLine | ( | ) |
Definition at line 2852 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionToScreenColumn | ( | ) |
Definition at line 2473 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionUp | ( | ) |
Definition at line 1887 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionUpToFirstNonBlank | ( | ) |
Definition at line 1963 of file katevinormalmode.cpp.
|
inlineprotected |
Definition at line 306 of file katevinormalmode.h.
KateViRange KateViNormalMode::motionWordBackward | ( | ) |
Definition at line 2011 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionWORDBackward | ( | ) |
Definition at line 2056 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionWordForward | ( | ) |
Definition at line 1977 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::motionWORDForward | ( | ) |
Definition at line 2034 of file katevinormalmode.cpp.
|
protected |
Definition at line 3526 of file katevinormalmode.cpp.
|
protected |
Definition at line 3649 of file katevinormalmode.cpp.
|
virtual |
Reimplemented in KateViVisualMode.
Definition at line 478 of file katevinormalmode.cpp.
|
protected |
(re)set to start configuration.
This is done when a command is completed executed or when a command is aborted
Definition at line 452 of file katevinormalmode.cpp.
|
protected |
Definition at line 3656 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectABackQuote | ( | ) |
Definition at line 3170 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectABracket | ( | ) |
Definition at line 3193 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectAComma | ( | ) |
Definition at line 3276 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectACurlyBracket | ( | ) |
Definition at line 3205 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectAInequalitySign | ( | ) |
Definition at line 3264 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectAParen | ( | ) |
Definition at line 3181 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectAQuoteDouble | ( | ) |
Definition at line 3150 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectAQuoteSingle | ( | ) |
Definition at line 3160 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectAWord | ( | ) |
Definition at line 2927 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectAWORD | ( | ) |
Definition at line 3039 of file katevinormalmode.cpp.
|
protected |
Definition at line 3720 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectInnerBackQuote | ( | ) |
Definition at line 3175 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectInnerBracket | ( | ) |
Definition at line 3199 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectInnerComma | ( | ) |
Definition at line 3281 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectInnerCurlyBracket | ( | ) |
Definition at line 3211 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectInnerInequalitySign | ( | ) |
Definition at line 3270 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectInnerParen | ( | ) |
Definition at line 3187 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectInnerQuoteDouble | ( | ) |
Definition at line 3155 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectInnerQuoteSingle | ( | ) |
Definition at line 3165 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectInnerWord | ( | ) |
Definition at line 3003 of file katevinormalmode.cpp.
KateViRange KateViNormalMode::textObjectInnerWORD | ( | ) |
Definition at line 3115 of file katevinormalmode.cpp.
|
protected |
Definition at line 3788 of file katevinormalmode.cpp.
Member Data Documentation
|
protected |
Definition at line 305 of file katevinormalmode.h.
|
protected |
Definition at line 301 of file katevinormalmode.h.
|
protected |
Definition at line 318 of file katevinormalmode.h.
|
protected |
Definition at line 314 of file katevinormalmode.h.
|
protected |
Definition at line 298 of file katevinormalmode.h.
|
protected |
Definition at line 343 of file katevinormalmode.h.
|
protected |
Definition at line 325 of file katevinormalmode.h.
|
protected |
Definition at line 320 of file katevinormalmode.h.
|
protected |
Definition at line 299 of file katevinormalmode.h.
|
protected |
Definition at line 338 of file katevinormalmode.h.
|
protected |
Definition at line 337 of file katevinormalmode.h.
|
protected |
Definition at line 311 of file katevinormalmode.h.
|
protected |
Definition at line 345 of file katevinormalmode.h.
|
protected |
Definition at line 332 of file katevinormalmode.h.
|
protected |
Definition at line 297 of file katevinormalmode.h.
|
protected |
Definition at line 315 of file katevinormalmode.h.
|
protected |
Definition at line 310 of file katevinormalmode.h.
|
protected |
Definition at line 313 of file katevinormalmode.h.
|
protected |
Definition at line 303 of file katevinormalmode.h.
Definition at line 329 of file katevinormalmode.h.
|
protected |
Definition at line 304 of file katevinormalmode.h.
|
protected |
Definition at line 330 of file katevinormalmode.h.
|
protected |
Definition at line 316 of file katevinormalmode.h.
|
protected |
Definition at line 306 of file katevinormalmode.h.
|
protected |
Definition at line 302 of file katevinormalmode.h.
|
protected |
Definition at line 335 of file katevinormalmode.h.
|
protected |
Definition at line 347 of file katevinormalmode.h.
|
protected |
Definition at line 326 of file katevinormalmode.h.
|
protected |
Definition at line 322 of file katevinormalmode.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.