kig
#include <cubic_imp.h>
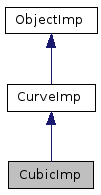
Public Types | |
typedef CurveImp | Parent |
![]() | |
typedef ObjectImp | Parent |
Public Member Functions | |
CubicImp (const CubicCartesianData &data) | |
~CubicImp () | |
QString | cartesianEquationString (const KigDocument &) const |
bool | contains (const Coordinate &p, int width, const KigWidget &) const |
bool | containsPoint (const Coordinate &p, const KigDocument &doc) const |
CubicImp * | copy () const |
const CubicCartesianData | data () const |
void | draw (KigPainter &p) const |
bool | equals (const ObjectImp &rhs) const |
double | getParam (const Coordinate &point, const KigDocument &) const |
const Coordinate | getPoint (double param, const KigDocument &) const |
const Coordinate | getPoint (double param) const |
const char * | iconForProperty (int which) const |
const ObjectImpType * | impRequirementForProperty (int which) const |
bool | inRect (const Rect &r, int width, const KigWidget &) const |
bool | internalContainsPoint (const Coordinate &p, double threshold) const |
bool | isPropertyDefinedOnOrThroughThisImp (int which) const |
bool | isVerticalCubic () const |
int | numberOfProperties () const |
const QByteArrayList | properties () const |
const QByteArrayList | propertiesInternalNames () const |
ObjectImp * | property (int which, const KigDocument &w) const |
Rect | surroundingRect () const |
ObjectImp * | transform (const Transformation &) const |
const ObjectImpType * | type () const |
void | visit (ObjectImpVisitor *vtor) const |
![]() | |
Coordinate | attachPoint () const |
QString | cartesianEquationString (const KigDocument &w) const |
![]() | |
virtual | ~ObjectImp () |
virtual bool | canFillInNextEscape () const |
virtual void | fillInNextEscape (QString &s, const KigDocument &) const |
int | getPropGid (const char *pname) const |
int | getPropLid (int propgid) const |
const char * | getPropName (int propgid) const |
bool | inherits (const ObjectImpType *t) const |
virtual bool | isCache () const |
bool | valid () const |
Static Public Member Functions | |
static const ObjectImpType * | stype () |
![]() | |
static const ObjectImpType * | stype () |
![]() | |
static const ObjectImpType * | stype () |
Additional Inherited Members | |
![]() | |
double | getDist (double param, const Coordinate &p, const KigDocument &doc) const |
double | getParamofmin (double a, double b, const Coordinate &p, const KigDocument &doc) const |
![]() | |
ObjectImp () | |
Detailed Description
An ObjectImp representing a cubic.
Definition at line 29 of file cubic_imp.h.
Member Typedef Documentation
typedef CurveImp CubicImp::Parent |
Definition at line 34 of file cubic_imp.h.
Constructor & Destructor Documentation
CubicImp::CubicImp | ( | const CubicCartesianData & | data | ) |
Definition at line 33 of file cubic_imp.cc.
CubicImp::~CubicImp | ( | ) |
Definition at line 38 of file cubic_imp.cc.
Member Function Documentation
QString CubicImp::cartesianEquationString | ( | const KigDocument & | ) | const |
Definition at line 423 of file cubic_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 56 of file cubic_imp.cc.
|
virtual |
Return whether this Curve contains the given point.
This is implemented as a numerical approximation. Implementations can/should use the value test_threshold in common.h as a threshold value.
Implements CurveImp.
Definition at line 369 of file cubic_imp.cc.
|
virtual |
Returns a copy of this ObjectImp.
The copy is an exact copy. Changes to the copy don't affect the original.
Implements CurveImp.
Definition at line 67 of file cubic_imp.cc.
const CubicCartesianData CubicImp::data | ( | ) | const |
Return the cartesian representation of this cubic.
Definition at line 331 of file cubic_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 51 of file cubic_imp.cc.
|
virtual |
Returns true if this ObjectImp is equal to rhs.
This function checks whether rhs is of the same ObjectImp type, and whether it contains the same data as this ObjectImp.
It is used e.g. by the KigCommand stuff to see what the user has changed during a move.
Implements ObjectImp.
Definition at line 341 of file cubic_imp.cc.
|
virtual |
Reimplemented from CurveImp.
Definition at line 72 of file cubic_imp.cc.
|
virtual |
Implements CurveImp.
Definition at line 154 of file cubic_imp.cc.
const Coordinate CubicImp::getPoint | ( | double | param | ) | const |
Definition at line 159 of file cubic_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 306 of file cubic_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 299 of file cubic_imp.cc.
Implements ObjectImp.
Definition at line 61 of file cubic_imp.cc.
bool CubicImp::internalContainsPoint | ( | const Coordinate & | p, |
double | threshold | ||
) | const |
Definition at line 374 of file cubic_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 400 of file cubic_imp.cc.
bool CubicImp::isVerticalCubic | ( | ) | const |
Definition at line 405 of file cubic_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 272 of file cubic_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 290 of file cubic_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 277 of file cubic_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 318 of file cubic_imp.cc.
|
static |
Definition at line 352 of file cubic_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 416 of file cubic_imp.cc.
|
virtual |
Return this ObjectImp, transformed by the transformation t.
Implements ObjectImp.
Definition at line 42 of file cubic_imp.cc.
|
virtual |
Returns the lowermost ObjectImpType that this object is an instantiation of.
E.g. if you want to get a string containing the internal name of the type of an object, you can do:
Implements ObjectImp.
Definition at line 347 of file cubic_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 336 of file cubic_imp.cc.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:35:40 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.