kstars
#include <skymesh.h>
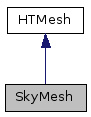
Public Member Functions | |
void | aperture (SkyPoint *center, double radius, MeshBufNum_t bufNum=DRAW_BUF) |
int | debug () |
void | debug (int debug) |
void | draw (QPainter &psky, MeshBufNum_t bufNum=DRAW_BUF) |
DrawID | drawID () |
int | incDrawID () |
Trixel | index (const SkyPoint *p) |
void | index (const QPointF &p1, const QPointF &p2, const QPointF &p3) |
void | index (const QPointF &p1, const QPointF &p2, const QPointF &p3, const QPointF &p4) |
void | index (const SkyPoint *center, double radius, MeshBufNum_t bufNum=DRAW_BUF) |
void | index (const SkyPoint *p1, const SkyPoint *p2) |
void | index (const SkyPoint *p1, const SkyPoint *p2, const SkyPoint *p3) |
void | index (const SkyPoint *p1, const SkyPoint *p2, const SkyPoint *p3, const SkyPoint *p4) |
const IndexHash & | indexLine (SkyList *points) |
const IndexHash & | indexLine (SkyList *points, IndexHash *skip) |
const IndexHash & | indexPoly (SkyList *points) |
const IndexHash & | indexPoly (const QPolygonF *points) |
Trixel | indexStar (StarObject *star) |
void | indexStar (StarObject *star1, StarObject *star2) |
const IndexHash & | indexStarLine (SkyList *points) |
bool | inDraw () |
void | inDraw (bool inDraw) |
void | setKSNumbers (KSNumbers *num) |
const SkyRegion & | skyRegion (const SkyPoint &p1, const SkyPoint &p2) |
![]() | |
HTMesh (int level, int buildLevel, int numBuffers=1) | |
~HTMesh () | |
Trixel | index (double ra, double dec) const |
void | intersect (double ra, double dec, double radius, BufNum bufNum=0) |
void | intersect (double ra1, double dec1, double ra2, double dec2, BufNum bufNum=0) |
void | intersect (double ra1, double dec1, double ra2, double dec2, double ra3, double dec3, BufNum bufNum=0) |
void | intersect (double ra1, double dec1, double ra2, double dec2, double ra3, double dec3, double ra4, double dec4, BufNum bufNum=0) |
int | intersectSize (BufNum bufNum=0) |
int | level () const |
MeshBuffer * | meshBuffer (BufNum bufNum=0) |
void | setDebug (int debug) |
int | size () const |
void | vertices (Trixel id, double *ra1, double *dec1, double *ra2, double *dec2, double *ra3, double *dec3) |
Static Public Member Functions | |
static SkyMesh * | Create (int level) |
static SkyMesh * | Instance () |
static SkyMesh * | Instance (int level) |
Protected Member Functions | |
SkyMesh (int level) | |
SkyMesh (SkyMesh &skyMesh) | |
Detailed Description
Constructor & Destructor Documentation
|
protected |
Definition at line 58 of file skymesh.cpp.
|
protected |
Member Function Documentation
void SkyMesh::aperture | ( | SkyPoint * | center, |
double | radius, | ||
MeshBufNum_t | bufNum = DRAW_BUF |
||
) |
finds the set of trixels that cover the circular aperture specified after first performing a reverse precession correction on the center so we don't have to re-index objects simply due to precession.
The drawID also gets incremented which is useful for drawing extended objects. Typically a safety factor of about one degree is added to the radius to account for proper motion, refraction and other imperfections.
- Parameters
-
center Center of the aperture radius Radius of the aperture in degrees bufNum Buffer to use
- Note
- See HTMesh.h for more
Definition at line 66 of file skymesh.cpp.
|
static |
Definition at line 36 of file skymesh.cpp.
void SkyMesh::draw | ( | QPainter & | psky, |
MeshBufNum_t | bufNum = DRAW_BUF |
||
) |
Definition at line 340 of file skymesh.cpp.
Definition at line 93 of file skymesh.cpp.
void SkyMesh::index | ( | const QPointF & | p1, |
const QPointF & | p2, | ||
const QPointF & | p3 | ||
) |
Definition at line 144 of file skymesh.cpp.
void SkyMesh::index | ( | const QPointF & | p1, |
const QPointF & | p2, | ||
const QPointF & | p3, | ||
const QPointF & | p4 | ||
) |
Definition at line 151 of file skymesh.cpp.
void SkyMesh::index | ( | const SkyPoint * | center, |
double | radius, | ||
MeshBufNum_t | bufNum = DRAW_BUF |
||
) |
Definition at line 114 of file skymesh.cpp.
Definition at line 123 of file skymesh.cpp.
Definition at line 129 of file skymesh.cpp.
void SkyMesh::index | ( | const SkyPoint * | p1, |
const SkyPoint * | p2, | ||
const SkyPoint * | p3, | ||
const SkyPoint * | p4 | ||
) |
Definition at line 136 of file skymesh.cpp.
Definition at line 159 of file skymesh.cpp.
Definition at line 190 of file skymesh.cpp.
Definition at line 241 of file skymesh.cpp.
const IndexHash & SkyMesh::indexPoly | ( | const QPolygonF * | points | ) |
Definition at line 289 of file skymesh.cpp.
Trixel SkyMesh::indexStar | ( | StarObject * | star | ) |
Definition at line 98 of file skymesh.cpp.
void SkyMesh::indexStar | ( | StarObject * | star1, |
StarObject * | star2 | ||
) |
Definition at line 105 of file skymesh.cpp.
Definition at line 164 of file skymesh.cpp.
|
static |
Definition at line 48 of file skymesh.cpp.
|
static |
returns the instance of SkyMesh corresponding to the given level
- Returns
- the instance of SkyMesh at the given level, or NULL if it has not yet been created.
Definition at line 53 of file skymesh.cpp.
returns the sky region needed to cover the rectangle defined by two SkyPoints p1 and p2
Definition at line 372 of file skymesh.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:36:23 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.