step/stepcore
#include <world.h>
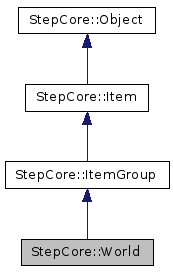
Additional Inherited Members | |
![]() | |
virtual ObjectErrors * | createObjectErrors () |
![]() | |
QString | _name |
Detailed Description
Contains multiple Item, Solver and general properties such as time.
- Todo:
- Redesign to avoid variable copying (scatter/gatherVariables)
Constructor & Destructor Documentation
StepCore::World::World | ( | const World & | world | ) |
StepCore::World::~World | ( | ) |
Member Function Documentation
|
inline |
void StepCore::World::clear | ( | ) |
|
inline |
Get current CollisionSolver.
|
inline |
Get current ConstraintSolver.
int StepCore::World::doEvolve | ( | double | delta | ) |
Integrate.
- Parameters
-
delta Integration interval
- Returns
- the same as Solver::doEvolve
- Todo:
- Provide error message
|
inline |
|
inline |
|
inline |
|
inline |
Object * StepCore::World::object | ( | const QString & | name | ) |
Add new item to the world.
Remove item from the world (you should delete item youself) Delete item from the world (it actually deletes item) Finds item in items() Get item by its index Get item by its name Get object (item, solver, *Solver or worls itself) by its name
CollisionSolver * StepCore::World::removeCollisionSolver | ( | ) |
Get current CollisionSolver and remove it from world.
ConstraintSolver * StepCore::World::removeConstraintSolver | ( | ) |
Get current ConstraintSolver and remove it from world.
Solver * StepCore::World::removeSolver | ( | ) |
void StepCore::World::setCollisionSolver | ( | CollisionSolver * | collisionSolver | ) |
Set new CollisionSolver (and delete the old one)
void StepCore::World::setConstraintSolver | ( | ConstraintSolver * | constraintSolver | ) |
Set new ConstraintSolver (and delete the old one)
|
inline |
|
inline |
void StepCore::World::setSolver | ( | Solver * | solver | ) |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:43:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.