KDECore
#include <k3reverseresolver.h>
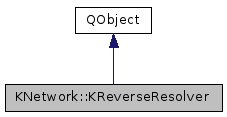
Public Types | |
enum | Flags { NumericHost = 0x01, NumericService = 0x02, NodeNameOnly = 0x04, Datagram = 0x08, NumericScope = 0x10, ResolutionRequired = 0x20 } |
Signals | |
void | finished (const KNetwork::KReverseResolver &obj) |
Public Member Functions | |
KReverseResolver (const KSocketAddress &addr, int flags=0, QObject *parent=0L) | |
virtual | ~KReverseResolver () |
const KSocketAddress & | address () const |
virtual bool | event (QEvent *) |
bool | failure () const |
bool | isRunning () const |
QString | node () const |
QString | service () const |
bool | start () |
bool | success () const |
Static Public Member Functions | |
static bool | resolve (const KSocketAddress &addr, QString &node, QString &serv, int flags=0) |
static bool | resolve (const struct sockaddr *sa, quint16 salen, QString &node, QString &serv, int flags=0) |
Detailed Description
Run a reverse-resolution on a socket address.
This class is provided as a counterpart to KResolver in such a way as it produces a reverse resolution: it resolves a socket address from its binary representations into a textual representation.
Most users will use the static functions resolve(), which work both synchronously (blocking) and asynchronously (non-blocking).
- Deprecated:
- Use KSocketFactory or KLocalSocket instead
Definition at line 51 of file k3reverseresolver.h.
Member Enumeration Documentation
Flags for the reverse resolution.
These flags are used by the reverse resolution functions for setting resolution parameters. The possible values are:
- NumericHost: don't try to resolve the host address to a text form. Instead, convert the address to its numeric textual representation.
- NumericService: the same as NumericHost, but for the service name
- NodeNameOnly: returns the node name only (i.e., not the Fully Qualified Domain Name)
- Datagram: in case of ambiguity in the service name, prefer the name associated with the datagram protocol
- NumericScope: for those addresses which have the concept of scope, resolve using the numeric value instead of the proper scope name.
- ResolutionRequired: normally, when resolving, if the name resolution fails, the process normally converts the numeric address into its presentation forms. This flag causes the function to return with error instead.
Enumerator | |
---|---|
NumericHost | |
NumericService | |
NodeNameOnly | |
Datagram | |
NumericScope | |
ResolutionRequired |
Definition at line 75 of file k3reverseresolver.h.
Constructor & Destructor Documentation
|
explicit |
Constructs this object to resolve the given socket address.
- Parameters
-
addr the address to resolve flags the flags to use, see Flags parent the parent object (see QObject)
Definition at line 115 of file k3reverseresolver.cpp.
|
virtual |
Destructor.
Definition at line 122 of file k3reverseresolver.cpp.
Member Function Documentation
const KSocketAddress & KReverseResolver::address | ( | ) | const |
Returns the socket address which was subject to resolution.
Definition at line 154 of file k3reverseresolver.cpp.
|
virtual |
Overrides event handling.
Definition at line 176 of file k3reverseresolver.cpp.
bool KReverseResolver::failure | ( | ) | const |
This function returns true if the processing has finished with failure, false if it's still running or succeeded.
Definition at line 139 of file k3reverseresolver.cpp.
|
signal |
This signal is emitted when the resolution has finished.
- Parameters
-
obj this class, which contains the results
bool KReverseResolver::isRunning | ( | ) | const |
This function returns 'true' if the processing is still running.
Definition at line 129 of file k3reverseresolver.cpp.
QString KReverseResolver::node | ( | ) | const |
Returns the resolved node name, if the resolution has finished successfully, or QString() otherwise.
Definition at line 144 of file k3reverseresolver.cpp.
|
static |
Resolves a socket address to its textual representation.
FIXME!! How can we do this in a non-blocking manner!?
This function is used to resolve a socket address from its binary representation to a textual form, even if numeric only.
- Parameters
-
addr the socket address to be resolved node the QString where we will store the resolved node serv the QString where we will store the resolved service flags flags to be used for this resolution.
- Returns
- true if the resolution succeeded, false if not
- See also
- ReverseFlags for the possible values for
flags
Definition at line 196 of file k3reverseresolver.cpp.
|
static |
Resolves a socket address to its textual representation.
FIXME!! How can we do this in a non-blocking manner!?
This function behaves just like the above one, except it takes a sockaddr structure and its size as parameters.
- Parameters
-
sa the sockaddr structure containing the address to be resolved salen the length of the sockaddr structure node the QString where we will store the resolved node serv the QString where we will store the resolved service flags flags to be used for this resolution.
- Returns
- true if the resolution succeeded, false if not
- See also
- ReverseFlags for the possible values for
flags
Definition at line 209 of file k3reverseresolver.cpp.
QString KReverseResolver::service | ( | ) | const |
Returns the resolved service name, if the resolution has finished successfully, or QString() otherwise.
Definition at line 149 of file k3reverseresolver.cpp.
bool KReverseResolver::start | ( | ) |
Starts the resolution.
This function returns 'true' if the resolution has started successfully.
Definition at line 159 of file k3reverseresolver.cpp.
bool KReverseResolver::success | ( | ) | const |
This function returns true if the processing has finished with success, false if it's still running or failed.
Definition at line 134 of file k3reverseresolver.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.