KIO
#include <kbookmarkmanager.h>
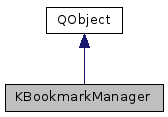
Public Slots | |
void | notifyChanged (const QString &groupAddress, const QDBusMessage &msg) |
void | notifyCompleteChange (const QString &caller) |
void | notifyConfigChanged () |
void | slotEditBookmarks () |
void | slotEditBookmarksAtAddress (const QString &address) |
Signals | |
void | bookmarkCompleteChange (QString caller) |
void | bookmarkConfigChanged () |
void | bookmarksChanged (QString groupAddress) |
void | changed (const QString &groupAddress, const QString &caller) |
void | configChanged () |
void | error (const QString &errorMessage) |
Public Member Functions | |
~KBookmarkManager () | |
bool | autoErrorHandlingEnabled () const |
void | emitChanged () |
void | emitChanged (const KBookmarkGroup &group) |
void | emitConfigChanged () |
KBookmark | findByAddress (const QString &address) |
QDomDocument | internalDocument () const |
QString | path () const |
KBookmarkGroup | root () const |
bool | save (bool toolbarCache=true) const |
bool | saveAs (const QString &filename, bool toolbarCache=true) const |
void | setAutoErrorHandlingEnabled (bool enable, QWidget *parent) |
void | setEditorOptions (const QString &caption, bool browser) |
void | setUpdate (bool update) |
KBookmarkGroup | toolbar () |
bool | updateAccessMetadata (const QString &url) |
void | updateFavicon (const QString &url, const QString &faviconurl) |
Static Public Member Functions | |
static KBookmarkManager * | createTempManager () |
static KBookmarkManager * | managerForExternalFile (const QString &bookmarksFile) |
static KBookmarkManager * | managerForFile (const QString &bookmarksFile, const QString &dbusObjectName) |
static KBookmarkManager * | userBookmarksManager () |
Detailed Description
This class implements the reading/writing of bookmarks in XML.
The bookmarks file is read and written using the XBEL standard (http://pyxml.sourceforge.net/topics/xbel/)
A sample file looks like this :
Definition at line 65 of file kbookmarkmanager.h.
Constructor & Destructor Documentation
KBookmarkManager::~KBookmarkManager | ( | ) |
Destructor.
Definition at line 308 of file kbookmarkmanager.cc.
Member Function Documentation
bool KBookmarkManager::autoErrorHandlingEnabled | ( | ) | const |
Check whether auto error handling is enabled.
If enabled, it will show an error dialog to the user when an error occurs. It is turned on by default.
- Returns
- true if auto error handling is enabled, false otherwise
- Note
- dialogs will only be displayed if the current thread is the gui thread
- Since
- 4.6
- See also
- setAutoErrorHandlingEnabled()
Definition at line 317 of file kbookmarkmanager.cc.
|
signal |
Signal send over DBUS.
|
signal |
Signal send over DBUS.
|
signal |
Signal send over DBUS.
Signals that the group (or any of its children) with the address groupAddress
(e.g.
"/4/5") has been modified by the caller caller
. connect to this
|
signal |
Signals that the config changed.
|
static |
only used for KBookmarkBar
Definition at line 196 of file kbookmarkmanager.cc.
void KBookmarkManager::emitChanged | ( | ) |
Saves the bookmark file and notifies everyone.
Definition at line 545 of file kbookmarkmanager.cc.
void KBookmarkManager::emitChanged | ( | const KBookmarkGroup & | group | ) |
Saves the bookmark file and notifies everyone.
- Parameters
-
group the parent of all changed bookmarks
Definition at line 551 of file kbookmarkmanager.cc.
void KBookmarkManager::emitConfigChanged | ( | ) |
Definition at line 564 of file kbookmarkmanager.cc.
|
signal |
Emitted when an error occurs.
Contains the translated error message.
- Since
- 4.6
- Returns
- the bookmark designated by
address
- Parameters
-
address the address belonging to the bookmark you're looking for tolerate when true tries to find the most tolerable bookmark position
- See also
- KBookmark::address
Definition at line 515 of file kbookmarkmanager.cc.
QDomDocument KBookmarkManager::internalDocument | ( | ) | const |
Definition at line 333 of file kbookmarkmanager.cc.
|
static |
Returns a KBookmarkManager, which will use KDirWatch for change detection This is important when sharing bookmarks with other Desktops.
- Parameters
-
bookmarksFile full path to the bookmarks file
- Since
- 4.1
Definition at line 172 of file kbookmarkmanager.cc.
|
static |
This static function will return an instance of the KBookmarkManager, responsible for the given bookmarksFile
.
If you do not instantiate this class either natively or in a derived class, then it will return an object with the default behaviors. If you wish to use different behaviors, you must derive your own class and instantiate it before this method is ever called.
- Parameters
-
bookmarksFile full path to the bookmarks file, Use ~/.kde/share/apps/konqueror/bookmarks.xml for the konqueror bookmarks dbusObjectName a unique name that represents this bookmark collection, usually your kinstance (e.g. kapplication) name. This is "konqueror" for the konqueror bookmarks, "kfile" for KFileDialog bookmarks, etc. The final DBus object path is /KBookmarkManager/dbusObjectName An empty dbusObjectName disables the registration to dbus (used for temporary managers)
Definition at line 150 of file kbookmarkmanager.cc.
|
slot |
Emit the changed signal for the group whose address is given.
- See also
- KBookmark::address() Called by the process that saved the file after a small change (new bookmark or new folder). Does not send signal over DBUS to the other Bookmark Managers You probably want to call emitChanged()
Definition at line 591 of file kbookmarkmanager.cc.
|
slot |
Reparse the whole bookmarks file and notify about the change Doesn't send signal over DBUS to the other Bookmark Managers You probably want to use emitChanged()
Definition at line 569 of file kbookmarkmanager.cc.
|
slot |
Definition at line 583 of file kbookmarkmanager.cc.
QString KBookmarkManager::path | ( | ) | const |
This will return the path that this manager is using to read the bookmarks.
- Returns
- the path containing the bookmarks
Definition at line 459 of file kbookmarkmanager.cc.
KBookmarkGroup KBookmarkManager::root | ( | ) | const |
This will return the root bookmark.
It is used to iterate through the bookmarks manually. It is mostly used internally.
- Returns
- the root (top-level) bookmark
Definition at line 464 of file kbookmarkmanager.cc.
Save the bookmarks to an XML file on disk.
You should use emitChanged() instead of this function, it saves and notifies everyone that the file has changed. Only use this if you don't want the emitChanged signal.
- Parameters
-
toolbarCache iff true save a cache of the toolbar folder, too
- Returns
- true if saving was successful
Definition at line 396 of file kbookmarkmanager.cc.
Save the bookmarks to the given XML file on disk.
- Parameters
-
filename full path to the desired bookmarks file location toolbarCache iff true save a cache of the toolbar folder, too
- Returns
- true if saving was successful
Definition at line 401 of file kbookmarkmanager.cc.
Enable or disable auto error handling is enabled.
If enabled, it will show an error dialog to the user when an error occurs. It is turned on by default. If disabled, the application should react on the error() signal.
- Parameters
-
enable true to enable auto error handling, false to disable parent the parent widget for the error dialogs, can be 0 for top-level
- Since
- 4.6
- See also
- autoErrorHandlingEnabled()
Definition at line 322 of file kbookmarkmanager.cc.
Set options with which slotEditBookmarks called keditbookmarks this can be used to change the appearance of the keditbookmarks in order to provide a slightly differing outer shell depending on the bookmarks file / app which calls it.
- Parameters
-
caption the –caption string, for instance "Konsole" browser iff false display no browser specific menu items in keditbookmarks :: –nobrowser
Definition at line 608 of file kbookmarkmanager.cc.
void KBookmarkManager::setUpdate | ( | bool | update | ) |
Set the update flag.
Defaults to true.
- Parameters
-
update if true then KBookmarkManager will listen to DBUS update requests.
Definition at line 328 of file kbookmarkmanager.cc.
|
slot |
Definition at line 614 of file kbookmarkmanager.cc.
|
slot |
Definition at line 627 of file kbookmarkmanager.cc.
KBookmarkGroup KBookmarkManager::toolbar | ( | ) |
This returns the root of the toolbar menu.
In the XML, this is the group with the attribute toolbar=yes
- Returns
- the toolbar group
Definition at line 469 of file kbookmarkmanager.cc.
Update access time stamps for a given url.
- Parameters
-
url the viewed url
- Returns
- true if any metadata was modified (bookmarks file is not saved automatically)
Definition at line 642 of file kbookmarkmanager.cc.
Definition at line 656 of file kbookmarkmanager.cc.
|
static |
Returns a pointer to the user's main (konqueror) bookmark collection.
Definition at line 669 of file kbookmarkmanager.cc.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.