KIO
#include <kfiledialog.h>
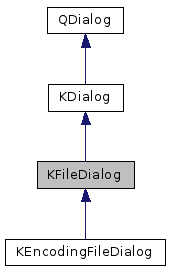
Public Types | |
enum | OperationMode { Other = 0, Opening, Saving } |
enum | Option { ConfirmOverwrite = 0x01, ShowInlinePreview = 0x02 } |
Public Slots | |
int | exec () |
Signals | |
void | fileHighlighted (const KUrl &) |
QT_MOC_COMPAT void | fileHighlighted (const QString &) |
void | fileSelected (const KUrl &) |
QT_MOC_COMPAT void | fileSelected (const QString &) |
void | filterChanged (const QString &filter) |
void | selectionChanged () |
Static Public Member Functions | |
static QString | getExistingDirectory (const KUrl &startDir=KUrl(), QWidget *parent=0, const QString &caption=QString()) |
static KUrl | getExistingDirectoryUrl (const KUrl &startDir=KUrl(), QWidget *parent=0, const QString &caption=QString()) |
static KUrl | getImageOpenUrl (const KUrl &startDir=KUrl(), QWidget *parent=0, const QString &caption=QString()) |
static QString | getOpenFileName (const KUrl &startDir=KUrl(), const QString &filter=QString(), QWidget *parent=0, const QString &caption=QString()) |
static QStringList | getOpenFileNames (const KUrl &startDir=KUrl(), const QString &filter=QString(), QWidget *parent=0, const QString &caption=QString()) |
static QString | getOpenFileNameWId (const KUrl &startDir, const QString &filter, WId parent_id, const QString &caption) |
static KUrl | getOpenUrl (const KUrl &startDir=KUrl(), const QString &filter=QString(), QWidget *parent=0, const QString &caption=QString()) |
static KUrl::List | getOpenUrls (const KUrl &startDir=KUrl(), const QString &filter=QString(), QWidget *parent=0, const QString &caption=QString()) |
static QString | getSaveFileName (const KUrl &startDir=KUrl(), const QString &filter=QString(), QWidget *parent=0, const QString &caption=QString()) |
static QString | getSaveFileName (const KUrl &startDir, const QString &filter, QWidget *parent, const QString &caption, Options options) |
static QString | getSaveFileNameWId (const KUrl &startDir, const QString &filter, WId parent_id, const QString &caption) |
static QString | getSaveFileNameWId (const KUrl &startDir, const QString &filter, WId parent_id, const QString &caption, Options options) |
static KUrl | getSaveUrl (const KUrl &startDir=KUrl(), const QString &filter=QString(), QWidget *parent=0, const QString &caption=QString()) |
static KUrl | getSaveUrl (const KUrl &startDir, const QString &filter, QWidget *parent, const QString &caption, Options options) |
static KUrl | getStartUrl (const KUrl &startDir, QString &recentDirClass) |
static void | setStartDir (const KUrl &directory) |
Protected Slots | |
virtual void | accept () |
virtual void | slotCancel () |
virtual void | slotOk () |
Protected Member Functions | |
virtual void | hideEvent (QHideEvent *event) |
virtual void | keyPressEvent (QKeyEvent *e) |
Detailed Description
Provides a user (and developer) friendly way to select files and directories.
The widget can be used as a drop in replacement for the QFileDialog widget, but has greater functionality and a nicer GUI.
You will usually want to use one of the static methods getOpenFileName(), getSaveFileName(), getOpenUrl() or for multiple files getOpenFileNames() or getOpenUrls().
The dialog has been designed to allow applications to customize it by subclassing. It uses geometry management to ensure that subclasses can easily add children that will be incorporated into the layout.
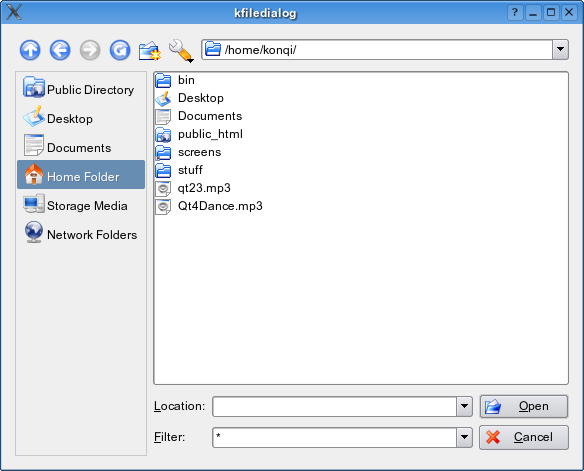
A file selection dialog.
Definition at line 68 of file kfiledialog.h.
Member Enumeration Documentation
Defines some default behavior of the filedialog.
E.g. in mode Opening
and Saving
, the selected files/urls will be added to the "recent documents" list. The Saving mode also implies setKeepLocation() being set.
Other
means that no default actions are performed.
- See also
- setOperationMode
- operationMode
Enumerator | |
---|---|
Other | |
Opening | |
Saving |
Definition at line 85 of file kfiledialog.h.
enum KFileDialog::Option |
Defines the options to use when calling getSave* functions.
- Since
- 4.4
Enumerator | |
---|---|
ConfirmOverwrite |
Confirm whether to overwrite file to save. |
ShowInlinePreview |
Always show an inline preview. |
Definition at line 91 of file kfiledialog.h.
Constructor & Destructor Documentation
KFileDialog::KFileDialog | ( | const KUrl & | startDir, |
const QString & | filter, | ||
QWidget * | parent, | ||
QWidget * | widget = 0 |
||
) |
Constructs a file dialog.
- Parameters
-
startDir Specifies the starting directory and/or initially selected file name, or a last used directory and optional file name using the kfiledialog:///
syntax. Refer to the KFileWidget documentation for more information on this parameter.filter A shell glob or a mimetype filter that specifies which files to display. For better consistency across applications, it is recommended to use a mimetype filter. See setFilter() and setMimeFilter() for details on how to use this argument. parent The parent widget of this dialog widget A widget, or a widget of widgets, for displaying custom data in the dialog. This can be used, for example, to display a check box with the caption "Open as read-only". When creating this widget, you don't need to specify a parent, since the widget's parent will be set automatically by KFileDialog.
- See also
- KFileWidget::KFileWidget()
Definition at line 253 of file kfiledialog.cpp.
KFileDialog::~KFileDialog | ( | ) |
Destructs the file dialog.
Definition at line 320 of file kfiledialog.cpp.
Member Function Documentation
|
protectedvirtualslot |
Definition at line 424 of file kfiledialog.cpp.
KActionCollection * KFileDialog::actionCollection | ( | ) | const |
- Returns
- a pointer to the action collection, holding all the used KActions.
Definition at line 882 of file kfiledialog.cpp.
KUrl KFileDialog::baseUrl | ( | ) | const |
- Returns
- the currently shown directory.
Definition at line 694 of file kfiledialog.cpp.
KPushButton * KFileDialog::cancelButton | ( | ) | const |
- Returns
- a pointer to the Cancel-Button in the filedialog. You may use it e.g. to set a custom text to it.
Definition at line 867 of file kfiledialog.cpp.
void KFileDialog::clearFilter | ( | ) |
Clears any mime- or namefilter.
Does not reload the directory.
Definition at line 363 of file kfiledialog.cpp.
QString KFileDialog::currentFilter | ( | ) | const |
Returns the current filter as entered by the user or one of the predefined set via setFilter().
- See also
- setFilter()
- filterChanged()
Definition at line 341 of file kfiledialog.cpp.
KMimeType::Ptr KFileDialog::currentFilterMimeType | ( | ) |
Returns the mimetype for the desired output format.
This is only valid if setMimeFilter() has been called previously.
- See also
- setFilterMimeType()
Definition at line 384 of file kfiledialog.cpp.
QString KFileDialog::currentMimeFilter | ( | ) | const |
The mimetype for the desired output format.
This is only valid if setMimeFilter() has been called previously.
- See also
- setMimeFilter()
Definition at line 372 of file kfiledialog.cpp.
|
slot |
Definition at line 965 of file kfiledialog.cpp.
|
signal |
Emitted when the user highlights a file.
- Since
- 4.4
|
signal |
- Deprecated:
- , connect to fileSelected(const KUrl&) instead
|
signal |
Emitted when the user selects a file.
It is only emitted in single- selection mode. The best way to get notified about selected file(s) is to connect to the okClicked() signal inherited from KDialog and call selectedFile(), selectedFiles(), selectedUrl() or selectedUrls().
- Since
- 4.4
|
signal |
- Deprecated:
- , connect to fileSelected(const KUrl&) instead
KAbstractFileWidget * KFileDialog::fileWidget | ( | ) |
Returns the KFileWidget that implements most of this file dialog.
If you link to libkfile you can cast this to a KFileWidget*.
Definition at line 959 of file kfiledialog.cpp.
|
signal |
Emitted when the filter changed, i.e.
the user entered an own filter or chose one of the predefined set via setFilter().
- Parameters
-
filter contains the new filter (only the extension part, not the explanation), i.e. "*.cpp" or "*.cpp *.cc".
- See also
- setFilter()
- currentFilter()
KFileFilterCombo * KFileDialog::filterWidget | ( | ) | const |
- Returns
- the combobox that contains the filters You need to link to libkfile to use this widget.
Definition at line 877 of file kfiledialog.cpp.
|
static |
Creates a modal directory-selection dialog and returns the selected directory (local only) or an empty string if none was chosen.
- Parameters
-
startDir Starting directory or kfiledialog:///
URL. Refer to the KFileWidget documentation for more information on this parameter.parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
- Returns
- the path to an existing local directory.
- See also
- KFileWidget::KFileWidget()
Definition at line 630 of file kfiledialog.cpp.
|
static |
Creates a modal directory-selection dialog and returns the selected directory or an empty string if none was chosen.
This version supports remote urls.
- Parameters
-
startDir Starting directory or kfiledialog:///
URL. Refer to the KFileWidget documentation for more information on this parameter.parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
- Returns
- the url to an existing directory (local or remote).
- See also
- KFileWidget::KFileWidget()
Definition at line 617 of file kfiledialog.cpp.
|
static |
Creates a modal file dialog with an image previewer and returns the selected url or an empty string if none was chosen.
- Parameters
-
startDir Starting directory or kfiledialog:///
URL. Refer to the KFileWidget documentation for more information on this parameter.parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
- See also
- KFileWidget::KFileWidget()
Definition at line 645 of file kfiledialog.cpp.
|
static |
Creates a modal file dialog and return the selected filename or an empty string if none was chosen.
Note that with this method the user must select an existing filename.
- Parameters
-
startDir Starting directory or kfiledialog:///
URL. Refer to the KFileWidget documentation for more information on this parameter.filter A shell glob or a mimetype filter that specifies which files to display. The preferred option is to set a list of mimetype names, see setMimeFilter() for details. Otherwise you can set the text to be displayed for the each glob, and provide multiple globs, see setFilter() for details. parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
- See also
- KFileWidget::KFileWidget()
Definition at line 464 of file kfiledialog.cpp.
|
static |
Creates a modal file dialog and returns the selected filenames or an empty list if none was chosen.
Note that with this method the user must select an existing filename.
- Parameters
-
startDir Starting directory or kfiledialog:///
URL. Refer to the KFileWidget documentation for more information on this parameter.filter A shell glob or a mimetype filter that specifies which files to display. The preferred option is to set a list of mimetype names, see setMimeFilter() for details. Otherwise you can set the text to be displayed for the each glob, and provide multiple globs, see setFilter() for details. parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
- See also
- KFileWidget::KFileWidget()
Definition at line 518 of file kfiledialog.cpp.
|
static |
Use this version only if you have no QWidget available as parent widget.
This can be the case if the parent widget is a widget in another process or if the parent widget is a non-Qt widget. For example, in a GTK program.
Definition at line 497 of file kfiledialog.cpp.
|
static |
Creates a modal file dialog and returns the selected URL or an empty string if none was chosen.
Note that with this method the user must select an existing URL.
- Parameters
-
startDir Starting directory or kfiledialog:///
URL. Refer to the KFileWidget documentation for more information on this parameter.filter A shell glob or a mimetype filter that specifies which files to display. The preferred option is to set a list of mimetype names, see setMimeFilter() for details. Otherwise you can set the text to be displayed for the each glob, and provide multiple globs, see setFilter() for details. parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
- See also
- KFileWidget::KFileWidget()
Definition at line 552 of file kfiledialog.cpp.
|
static |
Creates a modal file dialog and returns the selected URLs or an empty list if none was chosen.
Note that with this method the user must select an existing filename.
- Parameters
-
startDir Starting directory or kfiledialog:///
URL. Refer to the KFileWidget documentation for more information on this parameter.filter A shell glob or a mimetype filter that specifies which files to display. The preferred option is to set a list of mimetype names, see setMimeFilter() for details. Otherwise you can set the text to be displayed for the each glob, and provide multiple globs, see setFilter() for details. parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
- See also
- KFileWidget::KFileWidget()
Definition at line 578 of file kfiledialog.cpp.
|
static |
Creates a modal file dialog and returns the selected filename or an empty string if none was chosen.
Note that with this method the user need not select an existing filename.
- Parameters
-
startDir Starting directory or kfiledialog:///
URL. Refer to the KFileWidget documentation for more information on this parameter.filter A shell glob or a mimetype filter that specifies which files to display. The preferred option is to set a list of mimetype names, see setMimeFilter() for details. Otherwise you can set the text to be displayed for the each glob, and provide multiple globs, see setFilter() for details. parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
- See also
- KFileWidget::KFileWidget()
Definition at line 701 of file kfiledialog.cpp.
|
static |
Creates a modal file dialog and returns the selected filename or an empty string if none was chosen.
Note that with this method the user need not select an existing filename.
- Parameters
-
startDir Starting directory or kfiledialog:///
URL. Refer to the KFileWidget documentation for more information on this parameter.filter A shell glob or a mimetype filter that specifies which files to display. The preferred option is to set a list of mimetype names, see setMimeFilter() for details. Otherwise you can set the text to be displayed for the each glob, and provide multiple globs, see setFilter() for details. parent The widget the dialog will be centered on initially. caption The name of the dialog widget. options Dialog options.
- See also
- KFileWidget::KFileWidget()
- Since
- 4.4
Definition at line 710 of file kfiledialog.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 768 of file kfiledialog.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
- Since
- 4.4
Definition at line 777 of file kfiledialog.cpp.
|
static |
Creates a modal file dialog and returns the selected filename or an empty string if none was chosen.
Note that with this method the user need not select an existing filename.
- Parameters
-
startDir Starting directory or kfiledialog:///
URL. Refer to the KFileWidget documentation for more information on this parameter.filter A shell glob or a mimetype filter that specifies which files to display. The preferred option is to set a list of mimetype names, see setMimeFilter() for details. Otherwise you can set the text to be displayed for the each glob, and provide multiple globs, see setFilter() for details. parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
- See also
- KFileWidget::KFileWidget()
Definition at line 805 of file kfiledialog.cpp.
|
static |
Creates a modal file dialog and returns the selected filename or an empty string if none was chosen.
Note that with this method the user need not select an existing filename.
- Parameters
-
startDir Starting directory or kfiledialog:///
URL. Refer to the KFileWidget documentation for more information on this parameter.filter A shell glob or a mimetype filter that specifies which files to display. The preferred option is to set a list of mimetype names, see setMimeFilter() for details. Otherwise you can set the text to be displayed for the each glob, and provide multiple globs, see setFilter() for details. parent The widget the dialog will be centered on initially. caption The name of the dialog widget. options Dialog options.
- See also
- KFileWidget::KFileWidget()
- Since
- 4.4
Definition at line 813 of file kfiledialog.cpp.
This method implements the logic to determine the user's default directory to be listed.
E.g. the documents directory, home directory or a recently used directory.
- Parameters
-
startDir Starting directory or kfiledialog:///
URL. Refer to the KFileWidget documentation for more information on this parameter.recentDirClass If the kfiledialog:///
syntax is used, this will return the string to be passed to KRecentDirs::dir() and KRecentDirs::add().
- Returns
- The URL that should be listed by default (e.g. by KFileDialog or KDirSelectDialog).
- See also
- KFileWidget::KFileWidget()
- KFileWidget::getStartUrl( const KUrl& startDir, QString& recentDirClass );
Definition at line 941 of file kfiledialog.cpp.
|
protectedvirtual |
Reimplemented for saving the dialog geometry.
Definition at line 930 of file kfiledialog.cpp.
bool KFileDialog::keepsLocation | ( | ) | const |
- Returns
- whether the contents of the location edit are kept when changing directories.
Definition at line 894 of file kfiledialog.cpp.
|
protectedvirtual |
Reimplemented to animate the cancel button.
Definition at line 916 of file kfiledialog.cpp.
KUrlComboBox * KFileDialog::locationEdit | ( | ) | const |
- Returns
- the combobox used to type the filename or full location of the file. You need to link to libkfile to use this widget.
Definition at line 872 of file kfiledialog.cpp.
KFile::Modes KFileDialog::mode | ( | ) | const |
Returns the mode of the filedialog.
- See also
- setMode()
Definition at line 855 of file kfiledialog.cpp.
KPushButton * KFileDialog::okButton | ( | ) | const |
- Returns
- a pointer to the OK-Button in the filedialog. You may use it e.g. to set a custom text to it.
Definition at line 862 of file kfiledialog.cpp.
KFileDialog::OperationMode KFileDialog::operationMode | ( | ) | const |
- Returns
- the current operation mode, Opening, Saving or Other. Default is Other.
Definition at line 909 of file kfiledialog.cpp.
QString KFileDialog::selectedFile | ( | ) | const |
Returns the full path of the selected file in the local filesystem.
(Local files only)
Definition at line 680 of file kfiledialog.cpp.
QStringList KFileDialog::selectedFiles | ( | ) | const |
Returns a list of all selected local files.
Definition at line 687 of file kfiledialog.cpp.
KUrl KFileDialog::selectedUrl | ( | ) | const |
- Returns
- The selected fully qualified filename.
Definition at line 666 of file kfiledialog.cpp.
KUrl::List KFileDialog::selectedUrls | ( | ) | const |
- Returns
- The list of selected URLs.
Definition at line 673 of file kfiledialog.cpp.
|
signal |
Emitted when the user hilights one or more files in multiselection mode.
Note: fileHighlighted() or fileSelected() are not emitted in multiselection mode. You may use selectedItems() to ask for the current highlighted items.
- See also
- fileSelected
void KFileDialog::setConfirmOverwrite | ( | bool | enable | ) |
Sets whether the dialog should ask before accepting the selected file when KFileDialog::OperationMode is set to Saving.
In this case a KMessageBox appears for confirmation.
- Parameters
-
enable Set this to true to enable checking.
- Since
- 4.2
Definition at line 610 of file kfiledialog.cpp.
void KFileDialog::setFilter | ( | const QString & | filter | ) |
Sets the filter to be used to filter
.
The filter can be either set as a space-separated list of mimetypes, which is recommended, or as a list of shell globs separated by '\n'
.
If the filter contains an unescaped '/'
, a mimetype filter is assumed. If you would like a '/'
visible in your filter it can be escaped with a '\'
. You can specify multiple mimetypes like this (separated with space):
When showing the filter to the user, the mimetypes will be automatically translated into their description like `PNG image'. Multiple mimetypes will be automatically summarized to a filter item `All supported files'. To add a filter item for all files matching '*'
, add all/allfiles
as mimetype.
If the filter contains no unescaped '/'
, it is assumed that the filter contains conventional shell globs. Several filter items to select from can be separated by '\n'
. Every filter entry is defined through namefilter|text
to display. If no '
|' is found in the expression, just the namefilter is shown. Examples:
Note: The text to display is not parsed in any way. So, if you want to show the suffix to select by a specific filter, you must repeat it.
For better consistency across applications, it is recommended to use a mimetype filter.
- See also
- filterChanged
- setMimeFilter
Definition at line 332 of file kfiledialog.cpp.
void KFileDialog::setInlinePreviewShown | ( | bool | show | ) |
Forces the inline previews to be shown or hidden, depending on show
.
- Parameters
-
show Whether to show inline previews or not.
- Since
- 4.2
Definition at line 396 of file kfiledialog.cpp.
void KFileDialog::setKeepLocation | ( | bool | keep | ) |
Sets whether the filename/url should be kept when changing directories.
This is for example useful when having a predefined filename where the full path for that file is searched.
This is implicitly set when operationMode() is KFileDialog::Saving
getSaveFileName() and getSaveUrl() set this to true by default, so that you can type in the filename and change the directory without having to type the name again.
Definition at line 887 of file kfiledialog.cpp.
void KFileDialog::setLocationLabel | ( | const QString & | text | ) |
Sets the text to be displayed in front of the selection.
The default is "Location". Most useful if you want to make clear what the location is used for.
Definition at line 325 of file kfiledialog.cpp.
void KFileDialog::setMimeFilter | ( | const QStringList & | types, |
const QString & | defaultType = QString() |
||
) |
Sets the filter up to specify the output type.
- Parameters
-
types a list of mimetypes that can be used as output format defaultType the default mimetype to use as output format, if any. If defaultType
is set, it will be set as the current item. Otherwise, a first item showing all the mimetypes will be created. Typically,defaultType
should be empty for loading and set for saving.
Do not use in conjunction with setFilter()
Definition at line 348 of file kfiledialog.cpp.
void KFileDialog::setMode | ( | KFile::Modes | m | ) |
Sets the mode of the dialog.
The mode is defined as (in kfile.h):
You can OR the values, e.g.
Definition at line 847 of file kfiledialog.cpp.
void KFileDialog::setOperationMode | ( | KFileDialog::OperationMode | mode | ) |
Sets the operational mode of the filedialog to Saving
, Opening
or Other
.
This will set some flags that are specific to loading or saving files. E.g. setKeepLocation() makes mostly sense for a save-as dialog. So setOperationMode( KFileDialog::Saving ); sets setKeepLocation for example.
The mode Saving
, together with a default filter set via setMimeFilter() will make the filter combobox read-only.
The default mode is Opening
.
Call this method right after instantiating KFileDialog.
Definition at line 901 of file kfiledialog.cpp.
void KFileDialog::setPreviewWidget | ( | KPreviewWidgetBase * | w | ) |
Adds a preview widget and enters the preview mode.
In this mode the dialog is split and the right part contains your preview widget.
Ownership is transferred to KFileDialog. You need to create the preview-widget with "new", i.e. on the heap.
- Parameters
-
w The widget to be used for the preview.
Definition at line 389 of file kfiledialog.cpp.
void KFileDialog::setSelection | ( | const QString & | name | ) |
Sets the file name to preselect to name
.
This takes absolute URLs and relative file names.
Definition at line 454 of file kfiledialog.cpp.
|
static |
Used by KDirSelectDialog to share the dialog's start directory.
Definition at line 947 of file kfiledialog.cpp.
Sets the directory to view.
- Parameters
-
url URL to show. clearforward Indicates whether the forward queue should be cleared.
Definition at line 444 of file kfiledialog.cpp.
|
virtual |
- See also
- QWidget::sizeHint()
Definition at line 405 of file kfiledialog.cpp.
|
protectedvirtualslot |
Definition at line 436 of file kfiledialog.cpp.
|
protectedvirtualslot |
Definition at line 416 of file kfiledialog.cpp.
KToolBar * KFileDialog::toolBar | ( | ) | const |
Returns a pointer to the toolbar.
You can use this to insert custom items into it, e.g.:
Definition at line 954 of file kfiledialog.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:04 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.