akregator
#include <mainwidget.h>
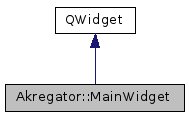
Public Types | |
enum | ViewMode { NormalView =0, WidescreenView, CombinedView } |
Signals | |
void | signalArticlesSelected (const QList< Akregator::Article > &) |
void | signalUnreadCountChanged (int) |
Public Member Functions | |
MainWidget (Akregator::Part *part, QWidget *parent, ActionManagerImpl *actionManager, const char *name) | |
~MainWidget () | |
void | addFeedToGroup (const QString &url, const QString &group) |
boost::shared_ptr< FeedList > | allFeedsList () |
QDomDocument | feedListToOPML () |
void | importFeedList (const QDomDocument &doc) |
bool | isNetworkAvailable () |
void | readProperties (const KConfigGroup &config) |
void | saveProperties (KConfigGroup &config) |
void | saveSettings () |
void | setFeedList (const boost::shared_ptr< FeedList > &feedList) |
ViewMode | viewMode () const |
Protected Slots | |
void | slotDeleteExpiredArticles () |
void | slotDoIntervalFetches () |
void | slotFetchingStarted () |
void | slotFetchingStopped () |
void | slotFramesChanged () |
void | slotMouseButtonPressed (int button, const KUrl &) |
void | slotOpenArticleInBrowser (const Akregator::Article &article) |
Protected Member Functions | |
void | addFeed (const QString &url, TreeNode *after, Folder *parent, bool autoExec=true) |
void | sendArticle (bool attach=false) |
Detailed Description
This is the main widget of the view, containing tree view, article list, viewer etc.
Definition at line 68 of file mainwidget.h.
Member Enumeration Documentation
Enumerator | |
---|---|
NormalView | |
WidescreenView | |
CombinedView |
Definition at line 119 of file mainwidget.h.
Constructor & Destructor Documentation
Akregator::MainWidget::MainWidget | ( | Akregator::Part * | part, |
QWidget * | parent, | ||
ActionManagerImpl * | actionManager, | ||
const char * | name | ||
) |
constructor
- Parameters
-
part the Akregator::Part which contains this widget parent parent widget name the name of the widget (QWidget )
Definition at line 104 of file mainwidget.cpp.
Akregator::MainWidget::~MainWidget | ( | ) |
destructor.
Note that cleanups should be done in slotOnShutdown(), so we don't risk accessing self-deleting objects after deletion.
Definition at line 95 of file mainwidget.cpp.
Member Function Documentation
|
protected |
Definition at line 669 of file mainwidget.cpp.
void Akregator::MainWidget::addFeedToGroup | ( | const QString & | url, |
const QString & | group | ||
) |
Add a feed to a group.
- Parameters
-
url The URL of the feed to add. group The name of the folder into which the feed is added. If the group does not exist, it is created. The feed is added as the last member of the group.
Definition at line 473 of file mainwidget.cpp.
|
inline |
Definition at line 107 of file mainwidget.h.
|
slot |
Definition at line 1172 of file mainwidget.cpp.
QDomDocument Akregator::MainWidget::feedListToOPML | ( | ) |
- Returns
- the displayed Feed List in OPML format
Definition at line 464 of file mainwidget.cpp.
void MainWidget::importFeedList | ( | const QDomDocument & | doc | ) |
Adds the feeds in doc
to the "Imported Folder".
- Parameters
-
doc the DOM tree (OPML) of the feeds to import
Definition at line 414 of file mainwidget.cpp.
bool MainWidget::isNetworkAvailable | ( | ) |
Definition at line 1183 of file mainwidget.cpp.
void Akregator::MainWidget::readProperties | ( | const KConfigGroup & | config | ) |
session management
Definition at line 1138 of file mainwidget.cpp.
void Akregator::MainWidget::saveProperties | ( | KConfigGroup & | config | ) |
Definition at line 1163 of file mainwidget.cpp.
void Akregator::MainWidget::saveSettings | ( | ) |
saves settings.
Make sure that the Settings singleton is not destroyed yet when saveSettings is called
Definition at line 342 of file mainwidget.cpp.
|
protected |
Definition at line 368 of file mainwidget.cpp.
void Akregator::MainWidget::setFeedList | ( | const boost::shared_ptr< FeedList > & | feedList | ) |
Definition at line 423 of file mainwidget.cpp.
|
signal |
emitted when the articles selected changed
|
signal |
emitted when the unread count of "All Feeds" was changed
|
slot |
deletes the currently selected article
Definition at line 982 of file mainwidget.cpp.
|
slot |
the article selection has changed
Definition at line 814 of file mainwidget.cpp.
|
slot |
toggles the keep flag of the currently selected article
Definition at line 1036 of file mainwidget.cpp.
|
slot |
switches view mode to combined view
Definition at line 542 of file mainwidget.cpp.
|
slot |
copies the link of current article to clipboard
Definition at line 941 of file mainwidget.cpp.
|
protectedslot |
Definition at line 459 of file mainwidget.cpp.
|
protectedslot |
Definition at line 768 of file mainwidget.cpp.
|
slot |
adds a new feed to the feed tree
Definition at line 650 of file mainwidget.cpp.
|
slot |
adds a feed group to the feed tree
Definition at line 681 of file mainwidget.cpp.
|
slot |
calls the properties dialog for feeds, starts renaming for feed groups
Definition at line 705 of file mainwidget.cpp.
|
slot |
removes the currently selected feed (ask for confirmation)
Definition at line 691 of file mainwidget.cpp.
|
slot |
called when URLs are dropped into the tree view
Definition at line 959 of file mainwidget.cpp.
|
slot |
starts fetching of all feeds in the tree
Definition at line 792 of file mainwidget.cpp.
|
slot |
fetches the currently selected feed
Definition at line 782 of file mainwidget.cpp.
|
protectedslot |
Definition at line 800 of file mainwidget.cpp.
|
protectedslot |
Definition at line 807 of file mainwidget.cpp.
|
protectedslot |
Definition at line 1030 of file mainwidget.cpp.
|
slot |
marks all articles in all feeds in the tree as read
Definition at line 747 of file mainwidget.cpp.
|
slot |
marks all articles in the currently selected feed as read
Definition at line 754 of file mainwidget.cpp.
|
protectedslot |
special behaviour in article list view (TODO: move code there?)
Definition at line 856 of file mainwidget.cpp.
|
slot |
displays a URL in the status bar when the user moves the mouse over a link
Definition at line 1133 of file mainwidget.cpp.
|
slot |
Definition at line 570 of file mainwidget.cpp.
|
slot |
Definition at line 586 of file mainwidget.cpp.
|
slot |
Definition at line 600 of file mainwidget.cpp.
|
slot |
Definition at line 554 of file mainwidget.cpp.
|
slot |
Definition at line 1188 of file mainwidget.cpp.
|
slot |
selects the next unread article in the article list
Definition at line 717 of file mainwidget.cpp.
|
slot |
selected tree node has changed
Definition at line 616 of file mainwidget.cpp.
|
slot |
switches view mode to normal view
Definition at line 496 of file mainwidget.cpp.
|
slot |
Definition at line 317 of file mainwidget.cpp.
|
protectedslot |
opens the link of an article in the external browser
Definition at line 908 of file mainwidget.cpp.
|
slot |
opens the homepage of the currently selected feed
Definition at line 883 of file mainwidget.cpp.
|
slot |
opens current article in new tab, background/foreground depends on settings TODO: use selected instead of current?
Definition at line 1203 of file mainwidget.cpp.
|
slot |
Definition at line 1209 of file mainwidget.cpp.
|
slot |
opens the current article (currentItem) in external browser TODO: use selected instead of current?
Definition at line 900 of file mainwidget.cpp.
|
slot |
selects the previous unread article in the article list
Definition at line 732 of file mainwidget.cpp.
|
slot |
reloads all open tabs
Definition at line 1177 of file mainwidget.cpp.
|
slot |
Definition at line 355 of file mainwidget.cpp.
|
inlineslot |
Definition at line 226 of file mainwidget.h.
|
inlineslot |
Definition at line 225 of file mainwidget.h.
|
slot |
marks the currenctly selected article as read after a user-set delay
Definition at line 1120 of file mainwidget.cpp.
|
slot |
marks the currently selected article as new
Definition at line 1115 of file mainwidget.cpp.
|
slot |
marks the currently selected article as read
Definition at line 1080 of file mainwidget.cpp.
|
slot |
marks the currently selected article as unread
Definition at line 1110 of file mainwidget.cpp.
|
slot |
emits signalUnreadCountChanged(int)
Definition at line 763 of file mainwidget.cpp.
|
slot |
reads the currently selected articles using KTTSD
Definition at line 1085 of file mainwidget.cpp.
|
slot |
toggles the visibility of the filter bar
Definition at line 965 of file mainwidget.cpp.
|
slot |
switches view mode to widescreen view
Definition at line 519 of file mainwidget.cpp.
|
inline |
Definition at line 123 of file mainwidget.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:58:14 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.