rocs/RocsCore
#include <DataStructure.h>
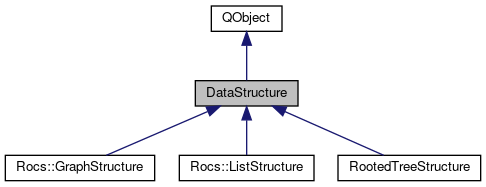
Public Slots | |
void | add_property (const QString &name, const QVariant &value) |
virtual DataList | addDataList (DataList dataList) |
virtual DataList | addDataList (QList< QPair< QString, QPointF > > dataList, int dataType) |
void | addDynamicProperty (const QString &property, const QVariant &value=QVariant(0)) |
virtual GroupPtr | addGroup (const QString &name) |
virtual DataPtr | createData (const QString &name, int dataType) |
virtual DataPtr | createData (const QString &name, const QPointF &point, int dataType) |
virtual PointerPtr | createPointer (DataPtr from, DataPtr to, int pointerType) |
DataPtr | getData (int uniqueIdentifier) |
virtual void | remove (DataPtr data) |
virtual void | remove (PointerPtr pointer) |
virtual void | remove (GroupPtr group) |
void | remove () |
void | remove_property (const QString &name) |
void | removeDynamicProperty (const QString &property) |
void | renameDynamicProperty (const QString &oldName, const QString &newName) |
void | setName (const QString &s) |
Signals | |
void | changed () |
void | dataCreated (DataPtr n) |
void | dataPositionChanged (const QPointF) |
void | nameChanged (const QString &name) |
void | pointerCreated (PointerPtr e) |
void | scriptError (const QString &message) |
Public Member Functions | |
virtual void | cleanUpBeforeConvert () |
const DataList | dataList (int dataType) const |
DataList | dataListAll () const |
Document * | document () const |
QScriptEngine * | engine () const |
virtual DataStructurePtr | getDataStructure () const |
const QList< GroupPtr > | groups () const |
bool | isDataVisible (int dataType) const |
bool | isPointerVisible (int pointerType) const |
QString | name () const |
virtual QMap< QString, QString > | pluginProperties () const |
PointerList | pointerListAll () const |
const PointerList | pointers (int pointerType) const |
bool | readOnly () const |
QScriptValue | scriptValue () const |
virtual void | setEngine (QScriptEngine *engine) |
virtual void | setPluginProperty (const QString &, const QString &) |
void | setReadOnly (bool r) |
void | updateData (DataPtr data) |
void | updatePointer (PointerPtr pointer) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static DataStructurePtr | create (Document *parent=0) |
static DataStructurePtr | create (DataStructurePtr other, Document *parent=0) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
DataStructure (Document *parent=0) | |
virtual | ~DataStructure () |
DataPtr | addData (DataPtr data) |
PointerPtr | addPointer (PointerPtr pointer) |
int | generateUniqueIdentifier () |
virtual void | importStructure (DataStructurePtr other) |
void | initialize () |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Static Protected Member Functions | |
template<typename T > | |
static DataStructurePtr | create (Document *parent=0) |
template<typename T > | |
static DataStructurePtr | create (DataStructurePtr other, Document *parent=0) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Properties | |
QString | name |
![]() | |
objectName | |
Detailed Description
Definition at line 43 of file DataStructure.h.
Constructor & Destructor Documentation
|
protected |
Default constructor.
- Parameters
-
parent the parent document of the data structure
Definition at line 109 of file DataStructure.cpp.
|
protectedvirtual |
Default destructor.
Definition at line 144 of file DataStructure.cpp.
Member Function Documentation
add the property named name
to this Data structure.
- Parameters
-
name is an identifier to property. value is the initial value of property.
Definition at line 505 of file DataStructure.cpp.
Definition at line 311 of file DataStructure.cpp.
- Deprecated:
- This method only calls addData for each element
Definition at line 348 of file DataStructure.cpp.
|
virtualslot |
Definition at line 358 of file DataStructure.cpp.
|
slot |
Definition at line 481 of file DataStructure.cpp.
Definition at line 468 of file DataStructure.cpp.
|
protected |
Definition at line 372 of file DataStructure.cpp.
|
signal |
|
virtual |
clear data that only is useful for a type of data structure and that cannot be converted to others
Definition at line 580 of file DataStructure.cpp.
|
static |
Definition at line 68 of file DataStructure.cpp.
|
static |
Definition at line 73 of file DataStructure.cpp.
|
inlinestaticprotected |
Definition at line 282 of file DataStructure.h.
|
inlinestaticprotected |
Definition at line 288 of file DataStructure.h.
Reimplemented in Rocs::GraphStructure, Rocs::ListStructure, and RootedTreeStructure.
Definition at line 300 of file DataStructure.cpp.
|
virtualslot |
Definition at line 339 of file DataStructure.cpp.
|
virtualslot |
Creates new pointer from data element "from" to data element "to" of given type "pointerType".
The pointer type must exist.
- Parameters
-
from data element where the pointer starts to data element where the pointer ends pointerType is the type of this pointer
Reimplemented in Rocs::GraphStructure, Rocs::ListStructure, and RootedTreeStructure.
Definition at line 393 of file DataStructure.cpp.
|
signal |
const DataList DataStructure::dataList | ( | int | dataType | ) | const |
Gives list of data elements of specified type if type exists.
If dataType does not exists, returns empty list.
- Parameters
-
dataType is type of data elements to be returned
- Returns
- DataList of data elements of specified type
Definition at line 149 of file DataStructure.cpp.
DataList DataStructure::dataListAll | ( | ) | const |
Gives list of all data elements of all existing types.
- Returns
- DataList of data elements
Definition at line 159 of file DataStructure.cpp.
|
signal |
Document * DataStructure::document | ( | ) | const |
Definition at line 570 of file DataStructure.cpp.
QScriptEngine * DataStructure::engine | ( | ) | const |
Definition at line 565 of file DataStructure.cpp.
|
protected |
Definition at line 295 of file DataStructure.cpp.
|
slot |
Access data element by its unique identifier.
Operation has amortized access time of O(1) (worst case O(n)).
- Parameters
-
uniqueIdentifier the unique identifier of the data element
Definition at line 411 of file DataStructure.cpp.
|
virtual |
Definition at line 103 of file DataStructure.cpp.
Definition at line 575 of file DataStructure.cpp.
|
protectedvirtual |
overwrites the current DataStructure with all values (Data and Pointer) from the given datastructure object.
- Parameters
-
other the data structure that shall be imported
- Returns
- void
Reimplemented in Rocs::GraphStructure, RootedTreeStructure, and Rocs::ListStructure.
Definition at line 116 of file DataStructure.cpp.
|
protected |
Definition at line 83 of file DataStructure.cpp.
bool DataStructure::isDataVisible | ( | int | dataType | ) | const |
Returns true if data elements of specified data type are visible, otherwise false.
- Parameters
-
dataType for that visibility information are returned
bool DataStructure::isPointerVisible | ( | int | pointerType | ) | const |
Returns true if pointers of specified pointer type are visible, otherwise false.
- Parameters
-
pointerType for that visibility information are returned
QString DataStructure::name | ( | ) | const |
|
signal |
Gives a map with plugin specific properties of the data structure.
- Returns
- map keys are property names, values are property values.
Reimplemented in Rocs::GraphStructure.
Definition at line 546 of file DataStructure.cpp.
|
signal |
PointerList DataStructure::pointerListAll | ( | ) | const |
Gives list all pointers of all existing types.
- Returns
- PointerList of pointers
Definition at line 179 of file DataStructure.cpp.
const PointerList DataStructure::pointers | ( | int | pointerType | ) | const |
Gives list of pointers of specified type if type exists.
If pointerType does not exists, returns empty list.
- Parameters
-
pointerType is type of pointers to be returned
- Returns
- PointerList of pointers of specified type
Definition at line 169 of file DataStructure.cpp.
bool DataStructure::readOnly | ( | ) | const |
Definition at line 551 of file DataStructure.cpp.
|
virtualslot |
Remove data
from data structure and (if necessary) destroys the data object.
It is valid to call this method more than once for the same data object.
- Parameters
-
data the data element that shall be removed
Reimplemented in Rocs::ListStructure.
Definition at line 428 of file DataStructure.cpp.
|
virtualslot |
Remove pointer
from data structure and (if necessary) destroys the pointer object.
It is valid to call this method more than once for the same pointer object.
- Parameters
-
pointer the pointer that shall be removed
Reimplemented in Rocs::ListStructure.
Definition at line 447 of file DataStructure.cpp.
|
virtualslot |
Remove group
from data structure and (if necessary) destroys the group object.
It is valid to call this method more than once for the same group object.
- Parameters
-
group the group that shall be removed
Definition at line 461 of file DataStructure.cpp.
|
slot |
if this datastructure shall be deleted, call ONLY this function
Definition at line 271 of file DataStructure.cpp.
|
slot |
remove the string named name
from this data structure.
- Parameters
-
name is the name of property to be removed.
Definition at line 510 of file DataStructure.cpp.
|
slot |
Definition at line 490 of file DataStructure.cpp.
Definition at line 495 of file DataStructure.cpp.
|
signal |
QScriptValue DataStructure::scriptValue | ( | ) | const |
Definition at line 561 of file DataStructure.cpp.
|
virtual |
Reimplemented in RootedTreeStructure.
Definition at line 515 of file DataStructure.cpp.
|
slot |
Definition at line 475 of file DataStructure.cpp.
Set plugin specific properties of data structure.
- Parameters
-
identifier is the unique identifier for this property value is the to be set value for the property
Reimplemented in Rocs::GraphStructure.
Definition at line 585 of file DataStructure.cpp.
void DataStructure::setReadOnly | ( | bool | r | ) |
Definition at line 265 of file DataStructure.cpp.
void DataStructure::updateData | ( | DataPtr | data | ) |
Updates registration of data in internal reference list.
- Parameters
-
data that is set with new type
Definition at line 247 of file DataStructure.cpp.
void DataStructure::updatePointer | ( | PointerPtr | pointer | ) |
Updates registration of pointer in internal reference list.
- Parameters
-
pointer that is set with new type
Definition at line 256 of file DataStructure.cpp.
Property Documentation
|
readwrite |
Definition at line 46 of file DataStructure.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.