rocs/RocsCore
#include <GraphStructure.h>
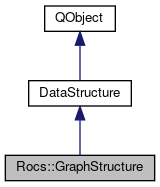
Public Types | |
enum | GRAPH_TYPE { Graph, Multigraph } |
Public Member Functions | |
GraphStructure (Document *parent=0) | |
~GraphStructure () | |
DataPtr | createData (const QString &name, int dataType) |
Q_INVOKABLE QScriptValue | createEdge (Data *fromRaw, Data *toRaw, int type) |
Q_INVOKABLE QScriptValue | createEdge (Data *fromRaw, Data *toRaw) |
Q_INVOKABLE QScriptValue | createNode (int type) |
Q_INVOKABLE QScriptValue | createNode () |
PointerPtr | createPointer (DataPtr from, DataPtr to, int pointerType) |
QMap< DataPtr, PointerList > | dijkstraShortestPaths (DataPtr from) |
Q_INVOKABLE QScriptValue | edges () |
Q_INVOKABLE QScriptValue | edges (int type) |
GRAPH_TYPE | graphType () const |
void | importStructure (DataStructurePtr other) |
bool | multigraph () const |
Q_INVOKABLE QScriptValue | nodes () |
Q_INVOKABLE QScriptValue | nodes (int type) |
QMap< QString, QString > | pluginProperties () const |
void | setPluginProperty (const QString &identifier, const QString &property) |
![]() | |
virtual void | cleanUpBeforeConvert () |
const DataList | dataList (int dataType) const |
DataList | dataListAll () const |
Document * | document () const |
QScriptEngine * | engine () const |
virtual DataStructurePtr | getDataStructure () const |
const QList< GroupPtr > | groups () const |
bool | isDataVisible (int dataType) const |
bool | isPointerVisible (int pointerType) const |
QString | name () const |
PointerList | pointerListAll () const |
const PointerList | pointers (int pointerType) const |
bool | readOnly () const |
QScriptValue | scriptValue () const |
virtual void | setEngine (QScriptEngine *engine) |
void | setReadOnly (bool r) |
void | updateData (DataPtr data) |
void | updatePointer (PointerPtr pointer) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static DataStructurePtr | create (Document *parent) |
static DataStructurePtr | create (DataStructurePtr other, Document *parent) |
![]() | |
static DataStructurePtr | create (Document *parent=0) |
static DataStructurePtr | create (DataStructurePtr other, Document *parent=0) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Properties | |
QString | name |
![]() | |
QString | name |
![]() | |
objectName | |
Additional Inherited Members | |
![]() | |
void | changed () |
void | dataCreated (DataPtr n) |
void | dataPositionChanged (const QPointF) |
void | nameChanged (const QString &name) |
void | pointerCreated (PointerPtr e) |
void | scriptError (const QString &message) |
![]() | |
DataStructure (Document *parent=0) | |
virtual | ~DataStructure () |
DataPtr | addData (DataPtr data) |
PointerPtr | addPointer (PointerPtr pointer) |
int | generateUniqueIdentifier () |
void | initialize () |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
template<typename T > | |
static DataStructurePtr | create (Document *parent=0) |
template<typename T > | |
static DataStructurePtr | create (DataStructurePtr other, Document *parent=0) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
Definition at line 29 of file GraphStructure.h.
Member Enumeration Documentation
Enumerator | |
---|---|
Graph | |
Multigraph |
Definition at line 35 of file GraphStructure.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 57 of file GraphStructure.cpp.
Rocs::GraphStructure::~GraphStructure | ( | ) |
Definition at line 91 of file GraphStructure.cpp.
Member Function Documentation
|
slot |
Creates a new edge from.
- Parameters
-
fromRaw to toRaw. fromRaw is origin of pointer toRaw is target of pointer
- Returns
- script value for the new pointer
Definition at line 227 of file GraphStructure.cpp.
|
slot |
Creates a new node with specified.
- Parameters
-
name. name of the new node
- Returns
- script value for the new node
Definition at line 217 of file GraphStructure.cpp.
|
slot |
Creates a new overlay edge from.
- Parameters
-
fromRaw to toRaw at overlay overlay. If the overlay does not exist no pointer is created. fromRaw is origin of pointer toRaw is target of pointer overlay is the overlay for the to created pointer
- Returns
- script value for the new pointer
Definition at line 235 of file GraphStructure.cpp.
|
static |
Definition at line 44 of file GraphStructure.cpp.
|
static |
Definition at line 49 of file GraphStructure.cpp.
Internal method to create new graph node.
Use
- See also
- Data::create(...) for creating new nodes.
- Parameters
-
name is the name of the node dataType is the type of this node, defaults to 0
- Returns
- the created node as DataPtr
Reimplemented from DataStructure.
Definition at line 450 of file GraphStructure.cpp.
QScriptValue Rocs::GraphStructure::createEdge | ( | Data * | fromRaw, |
Data * | toRaw, | ||
int | type | ||
) |
Creates a new edge edge from.
- Parameters
-
fromRaw to toRaw of pointer type type. If the specified pointer type does not exist, no pointer is created. fromRaw is origin of pointer toRaw is target of pointer overlay is the overlay for the to created pointer
- Returns
- script value for the new pointer
Definition at line 152 of file GraphStructure.cpp.
QScriptValue Rocs::GraphStructure::createEdge | ( | Data * | fromRaw, |
Data * | toRaw | ||
) |
Creates a new edge from.
- Parameters
-
fromRaw to toRaw. fromRaw is origin of pointer toRaw is target of pointer
- Returns
- script value for the new pointer
Definition at line 147 of file GraphStructure.cpp.
QScriptValue Rocs::GraphStructure::createNode | ( | int | type | ) |
Creates a new data element and return it.
If the specified data type is not registered, no data element is created.
- Parameters
-
type is the data type of the created node
- Returns
- script value for the new node
Definition at line 140 of file GraphStructure.cpp.
QScriptValue Rocs::GraphStructure::createNode | ( | ) |
Creates a new data element and return it.
- Returns
- script value for the new node
Definition at line 135 of file GraphStructure.cpp.
|
virtual |
Internal method to create new graph edge.
Use
- See also
- Pointer::create(...) for creating new edges.
- Parameters
-
from is the origin of the new edge to is the target of the new edge pointerType is the type of this edge, defaults to 0
- Returns
- the created edge as PointerPtr
Reimplemented from DataStructure.
Definition at line 423 of file GraphStructure.cpp.
|
slot |
Computes the Dijkstra's shortest path algorithm to compute the shortes path from.
- Parameters
-
from to to. Note: this shortest path algorithm works only for graphs with all edges values non-negative. For undirected graphs reverse edges are add automatically. The algorithm has time complexity O(V log V + E). from the node from which the computation starts to the node to which the shortest path shall be computed
- Returns
- the edge set of the shortest path
Definition at line 243 of file GraphStructure.cpp.
QMap< DataPtr, PointerList > Rocs::GraphStructure::dijkstraShortestPaths | ( | DataPtr | from | ) |
Computes the Dijkstra's shortest path algorithm to compute all shortest path distances from from
.
Note: this shortest path algorithm works only for graphs with all edges values non-negative. For undirected graphs reverse edges are add automatically. The algorithm has time complexity O(V log V + E).
- Parameters
-
from the node from which the computation starts
- Returns
- list is map with keys = target elements, values = path to target
Definition at line 294 of file GraphStructure.cpp.
|
slot |
Computes the Dijkstra's shortest path algorithm to compute all shortest path distances from from
.
If edge has value 0, the edge value is set to 1. Infinity is returned when there is no path between from
and another node in the graph. Note: this shortest path algorithm works only for graphs with all edges values non-negative. For undirected graphs reverse edges are added automatically. The algorithm has time complexity O(V log V + E).
- Parameters
-
from the node from which the computation starts
- Returns
- the edge set of the shortest path
Definition at line 262 of file GraphStructure.cpp.
QScriptValue Rocs::GraphStructure::edges | ( | ) |
Returns a list of all edges of the graph.
- Returns
- array containing the edges
Definition at line 115 of file GraphStructure.cpp.
QScriptValue Rocs::GraphStructure::edges | ( | int | type | ) |
Returns a list of all edges of given type
in the graph.
- Parameters
-
type is the overlay for the to created pointer
- Returns
- array containing the edges
Definition at line 126 of file GraphStructure.cpp.
Rocs::GraphStructure::GRAPH_TYPE Rocs::GraphStructure::graphType | ( | ) | const |
Returns type of the graph given by enum.
- See also
- GRAPH_TYPE.
- Returns
- graph type
Definition at line 413 of file GraphStructure.cpp.
|
virtual |
overwrites the current DataStructure with all values (Data and Pointer) from the given datastructure object.
- Parameters
-
other the data structure that shall be imported
- Returns
- void
Reimplemented from DataStructure.
Definition at line 63 of file GraphStructure.cpp.
|
slot |
Returns a list of all edges of the graph.
- Returns
- array containing the edges
Definition at line 201 of file GraphStructure.cpp.
|
slot |
Returns a list of all edges of given type
in the graph.
- Parameters
-
type is the overlay for the to created pointer
- Returns
- array containing the edges
Definition at line 209 of file GraphStructure.cpp.
|
slot |
Returns a list of all nodes of the graph.
- Returns
- array containing the nodes
Definition at line 185 of file GraphStructure.cpp.
|
slot |
Returns a list of all nodes of given type
in the graph.
- Parameters
-
type is the overlay for the to created pointer
- Returns
- array containing the nodes
Definition at line 193 of file GraphStructure.cpp.
bool Rocs::GraphStructure::multigraph | ( | ) | const |
Definition at line 418 of file GraphStructure.cpp.
QScriptValue Rocs::GraphStructure::nodes | ( | ) |
Returns a list of all nodes of the graph.
- Returns
- array containing the nodes
Definition at line 95 of file GraphStructure.cpp.
QScriptValue Rocs::GraphStructure::nodes | ( | int | type | ) |
Returns a list of all nodes of given type
in the graph.
- Parameters
-
type is the overlay for the to created pointer
- Returns
- array containing the nodes
Definition at line 106 of file GraphStructure.cpp.
|
slot |
Gives array of edges of specified overlay.
If overlay does not exist an empty array is returned.
- Parameters
-
overlay integer that identifies the overlay
- Returns
- QScriptValue array
Definition at line 177 of file GraphStructure.cpp.
Gives a map with plugin specific properties of the data structure.
Implementation of virtual method
- Returns
- map keys are property names, values are property values.
Reimplemented from DataStructure.
Definition at line 462 of file GraphStructure.cpp.
|
slot |
Setter for graph type.
No conversations are performed.
- Parameters
-
type as specified by enum GRAPH_TYPE
- Returns
- void
Definition at line 383 of file GraphStructure.cpp.
|
virtual |
Set plugin specific properties of data structure.
- Parameters
-
identifier is the unique identifier for this property value is the to be set value for the property
Reimplemented from DataStructure.
Definition at line 469 of file GraphStructure.cpp.
Property Documentation
|
readwrite |
Definition at line 32 of file GraphStructure.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.