KDEUI
#include <kkeysequencewidget.h>
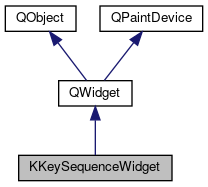
Public Types | |
enum | Validation { Validate = 0, NoValidate = 1 } |
Public Slots | |
void | applyStealShortcut () |
void | captureKeySequence () |
void | clearKeySequence () |
void | setKeySequence (const QKeySequence &seq, Validation val=NoValidate) |
Signals | |
void | keySequenceChanged (const QKeySequence &seq) |
void | stealShortcut (const QKeySequence &seq, KAction *action) |
Public Member Functions | |
KKeySequenceWidget (QWidget *parent=0) | |
virtual | ~KKeySequenceWidget () |
bool | isKeySequenceAvailable (const QKeySequence &seq) const |
QKeySequence | keySequence () const |
void | setCheckActionCollections (const QList< KActionCollection * > &actionCollections) |
void | setCheckActionList (const QList< QAction * > &checkList) |
void | setComponentName (const QString &componentName) |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
virtual int | heightForWidth (int w) const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
virtual QSize | minimumSizeHint () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
virtual QSize | sizeHint () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Configuration | |
enum | ShortcutType { None = 0x00, LocalShortcuts = 0x01, StandardShortcuts = 0x02, GlobalShortcuts = 0x03 } |
void | setCheckForConflictsAgainst (ShortcutTypes types) |
ShortcutTypes | checkForConflictsAgainst () const |
void | setMultiKeyShortcutsAllowed (bool) |
bool | multiKeyShortcutsAllowed () const |
void | setModifierlessAllowed (bool allow) |
bool | isModifierlessAllowed () |
void | setClearButtonShown (bool show) |
Additional Inherited Members | |
![]() | |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
QWidgetMapper * | wmapper () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
int | x11AppCells (int screen) |
Qt::HANDLE | x11AppColormap (int screen) |
bool | x11AppDefaultColormap (int screen) |
bool | x11AppDefaultVisual (int screen) |
int | x11AppDepth (int screen) |
Display * | x11AppDisplay () |
int | x11AppDpiX (int screen) |
int | x11AppDpiY (int screen) |
Qt::HANDLE | x11AppRootWindow (int screen) |
int | x11AppScreen () |
void * | x11AppVisual (int screen) |
void | x11SetAppDpiX (int dpi, int screen) |
void | x11SetAppDpiY (int dpi, int screen) |
![]() | |
typedef | RenderFlags |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | changeEvent (QEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEvent *event) |
virtual bool | event (QEvent *event) |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual void | paintEvent (QPaintEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual void | wheelEvent (QWheelEvent *event) |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QPaintDevice () | |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
A widget to input a QKeySequence.
This widget lets the user choose a QKeySequence, which is usually used as a shortcut key. The recording is initiated by calling captureKeySequence() or the user clicking into the widget.
The widgets provides support for conflict handling. See setCheckForConflictsAgainst() for more information.

Definition at line 51 of file kkeysequencewidget.h.
Member Enumeration Documentation
Enumerator | |
---|---|
None |
No checking for conflicts. |
LocalShortcuts |
Check with local shortcuts.
|
StandardShortcuts |
Check against standard shortcuts.
|
GlobalShortcuts |
Check against global shortcuts.
|
Definition at line 99 of file kkeysequencewidget.h.
An enum about validation when setting a key sequence.
- See also
- setKeySequence()
Enumerator | |
---|---|
Validate |
Validate key sequence. |
NoValidate |
Use key sequence without validation. |
Definition at line 75 of file kkeysequencewidget.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
Definition at line 251 of file kkeysequencewidget.cpp.
|
virtual |
Destructs the widget.
Definition at line 289 of file kkeysequencewidget.cpp.
Member Function Documentation
|
slot |
Actually remove the shortcut that the user wanted to steal, from the action that was using it.
This only applies to actions provided to us by setCheckActionCollections() and setCheckActionList().
Global and Standard Shortcuts have to be stolen immediately when the user gives his consent (technical reasons). That means those changes will be active even if you never call applyStealShortcut().
To be called before you apply your changes. No local shortcuts are stolen until this function is called.
Definition at line 398 of file kkeysequencewidget.cpp.
|
slot |
Capture a shortcut from the keyboard.
This call will only return once a key sequence has been captured or input was aborted. If a key sequence was input, keySequenceChanged() will be emitted.
- See also
- setModifierlessAllowed()
Definition at line 364 of file kkeysequencewidget.cpp.
ShortcutTypes KKeySequenceWidget::checkForConflictsAgainst | ( | ) | const |
|
slot |
Clear the key sequence.
Definition at line 392 of file kkeysequencewidget.cpp.
bool KKeySequenceWidget::isKeySequenceAvailable | ( | const QKeySequence & | seq | ) | const |
Checks whether the key sequence seq is available to grab.
The sequence is checked under the same rules as if it has been typed by the user. This method is useful if you get key sequences from another input source and want to check if it is save to set them.
- Since
- 4.2
Definition at line 329 of file kkeysequencewidget.cpp.
bool KKeySequenceWidget::isModifierlessAllowed | ( | ) |
- See also
- setModifierlessAllowed()
Definition at line 339 of file kkeysequencewidget.cpp.
QKeySequence KKeySequenceWidget::keySequence | ( | ) | const |
Return the currently selected key sequence.
Definition at line 370 of file kkeysequencewidget.cpp.
|
signal |
This signal is emitted when the current key sequence has changed, be it by user input or programmatically.
bool KKeySequenceWidget::multiKeyShortcutsAllowed | ( | ) | const |
void KKeySequenceWidget::setCheckActionCollections | ( | const QList< KActionCollection * > & | actionCollections | ) |
Set a list of action collections to check against for conflictuous shortcut.
- See also
- setCheckForConflictsAgainst()
If a KAction with a conflicting shortcut is found inside this list and its shortcut can be configured (KAction::isShortcutConfigurable() returns true) the user will be prompted whether to steal the shortcut from this action.
- Since
- 4.1
Definition at line 358 of file kkeysequencewidget.cpp.
- Deprecated:
- since 4.1 use setCheckActionCollections so that KKeySequenceWidget knows in which action collection to call the writeSettings method after stealing a shortcut from an action.
Definition at line 351 of file kkeysequencewidget.cpp.
void KKeySequenceWidget::setCheckForConflictsAgainst | ( | ShortcutTypes | types | ) |
Configure if the widget should check for conflicts with existing shortcuts.
When capturing a key sequence for local shortcuts you should check against GlobalShortcuts and your other local shortcuts. This is the default.
You have to provide the local actions to check against with setCheckActionCollections().
When capturing a key sequence for a global shortcut you should check against StandardShortcuts, GlobalShortcuts and your local shortcuts.
There are two ways to react to a user agreeing to steal a shortcut:
- Listen to the stealShortcut() signal and steal the shortcuts manually. It's your responsibility to save that change later when you think it is appropriate.
- Call applyStealShortcut and KKeySequenceWidget will steal the shortcut. This will save the actionCollections the shortcut is part of so make sure it doesn't inadvertly save some unwanted changes too. Read its documentation for some limitation when handling global shortcuts.
If you want to do the conflict checking yourself here are some code snippets for global ...
... and standard shortcuts
- Since
- 4.2
Definition at line 318 of file kkeysequencewidget.cpp.
void KKeySequenceWidget::setClearButtonShown | ( | bool | show | ) |
Set whether a small button to set an empty key sequence should be displayed next to the main input widget.
The default is to show the clear button.
Definition at line 345 of file kkeysequencewidget.cpp.
void KKeySequenceWidget::setComponentName | ( | const QString & | componentName | ) |
If the component using this widget supports shortcuts contexts, it has to set its component name so we can check conflicts correctly.
Definition at line 301 of file kkeysequencewidget.cpp.
|
slot |
Set the key sequence.
If val
== Validate, and the call is actually changing the key sequence, conflictuous shortcut will be checked.
Definition at line 377 of file kkeysequencewidget.cpp.
void KKeySequenceWidget::setModifierlessAllowed | ( | bool | allow | ) |
This only applies to user input, not to setShortcut().
Set whether to accept "plain" keys without modifiers (like Ctrl, Alt, Meta). Plain keys by our definition include letter and symbol keys and text editing keys (Return, Space, Tab, Backspace, Delete). "Special" keys like F1, Cursor keys, Insert, PageDown will always work.
Definition at line 323 of file kkeysequencewidget.cpp.
void KKeySequenceWidget::setMultiKeyShortcutsAllowed | ( | bool | allowed | ) |
Allow multikey shortcuts?
Definition at line 312 of file kkeysequencewidget.cpp.
|
signal |
This signal is emitted after the user agreed to steal a shortcut from an action.
This is only done for local shortcuts. So you can be sure action is one of the actions you provided with setCheckActionList() or setCheckActionCollections().
If you listen to that signal and don't call applyStealShortcut() you are supposed to steal the shortcut and save this change.
Property Documentation
|
readwrite |
Definition at line 65 of file kkeysequencewidget.h.
|
readwrite |
Definition at line 70 of file kkeysequencewidget.h.
|
readwrite |
Definition at line 60 of file kkeysequencewidget.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.