KHTML
#include <khtml_part.h>
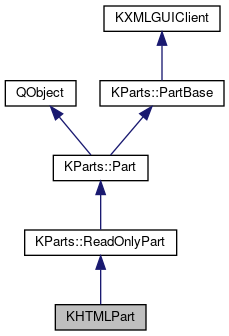
Public Types | |
enum | CaretDisplayPolicy { CaretVisible, CaretInvisible, CaretBlink } |
enum | DNSPrefetch { DNSPrefetchDisabled =0, DNSPrefetchEnabled, DNSPrefetchOnlyWWWAndSLD } |
enum | FindOptions { FindLinksOnly = 1 * KFind::MinimumUserOption, FindNoPopups = 2 * KFind::MinimumUserOption } |
enum | FormNotification { NoNotification = 0, Before, Only, Unused =255 } |
enum | GUIProfile { DefaultGUI, BrowserViewGUI } |
![]() | |
enum | ReverseStateChange |
Public Slots | |
QVariant | executeScript (const QString &script) |
void | setActiveNode (const DOM::Node &node) |
void | setCaretMode (bool enable) |
void | setCaretVisible (bool show) |
void | setEditable (bool enable) |
void | stopAnimations () |
void | submitFormProxy (const char *action, const QString &url, const QByteArray &formData, const QString &target, const QString &contentType=QString(), const QString &boundary=QString()) |
![]() |
Signals | |
void | caretPositionChanged (const DOM::Node &node, long offset) |
void | configurationChanged () |
void | docCreated () |
void | formSubmitNotification (const char *action, const QString &url, const QByteArray &formData, const QString &target, const QString &contentType, const QString &boundary) |
void | nodeActivated (const DOM::Node &) |
void | onURL (const QString &url) |
void | popupMenu (const QString &url, const QPoint &point) |
void | selectionChanged () |
![]() | |
void | canceled (const QString &errMsg) |
void | completed () |
void | completed (bool pendingAction) |
void | started (KIO::Job *) |
void | urlChanged (const KUrl &url) |
![]() | |
void | setStatusBarText (const QString &text) |
void | setWindowCaption (const QString &caption) |
Protected Types | |
enum | PageSecurity { NotCrypted, Encrypted, Mixed } |
Protected Slots | |
virtual void | slotFinished (KJob *) |
![]() | |
void | slotWidgetDestroyed () |
Properties | |
bool | dndEnabled |
DNSPrefetch | dnsPrefetch |
QString | encoding |
bool | javaEnabled |
bool | javaScriptEnabled |
QString | lastModified |
bool | metaRefreshEnabled |
bool | modified |
bool | pluginsEnabled |
![]() | |
KUrl | url |
![]() | |
objectName | |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
static QString | findMostRecentXMLFile (const QStringList &files, QString &doc) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
This class is khtml's main class.
It features an almost complete web browser, and html renderer.
The easiest way to use this class (if you just want to display an HTML page at some URL) is the following:
Java and JavaScript are enabled by default depending on the user's settings. If you do not need them, and especially if you display unfiltered data from untrusted sources, it is strongly recommended to turn them off. In that case, you should also turn off the automatic redirect and plugins:
You may also wish to disable external references. This will prevent KHTML from loading images, frames, etc, or redirecting to external sites.
Some apps want to write their HTML code directly into the widget instead of opening an url. You can do this in the following way:
You can do as many calls to write() as you wish. There are two write() methods, one accepting a QString and one accepting a char
*
argument. You should use one or the other (but not both) since the method using the char
*
argument does an additional decoding step to convert the written data to Unicode.
It is also possible to write content to the HTML part using the standard streaming API from KParts::ReadOnlyPart. The usage of the API is similar to that of the begin(), write(), end() process described above as the following example shows:
HTML Browser Widget
Definition at line 206 of file khtml_part.h.
Member Enumeration Documentation
Enumeration for displaying the caret.
Enumerator | |
---|---|
CaretVisible |
caret is displayed |
CaretInvisible |
caret is not displayed |
CaretBlink |
caret toggles between visible and invisible |
Definition at line 586 of file khtml_part.h.
DNS Prefetching Mode enumeration.
- DNSPrefetchDisabled do not prefetch hostnames
- DNSPrefetchEnabled always prefetch hostnames
- DNSPrefetchOnlyWWWAndSLD only do DNS prefetching for bare SLD and www sub-domain
Enumerator | |
---|---|
DNSPrefetchDisabled | |
DNSPrefetchEnabled | |
DNSPrefetchOnlyWWWAndSLD |
Definition at line 281 of file khtml_part.h.
Extra Find options that can be used when calling the extended findText().
Enumerator | |
---|---|
FindLinksOnly | |
FindNoPopups |
Definition at line 787 of file khtml_part.h.
Enumerator | |
---|---|
NoNotification | |
Before | |
Only | |
Unused |
Definition at line 1106 of file khtml_part.h.
Enumerator | |
---|---|
DefaultGUI | |
BrowserViewGUI |
Definition at line 272 of file khtml_part.h.
|
protected |
Enumerator | |
---|---|
NotCrypted | |
Encrypted | |
Mixed |
Definition at line 1277 of file khtml_part.h.
Constructor & Destructor Documentation
KHTMLPart::KHTMLPart | ( | QWidget * | parentWidget = 0 , |
QObject * | parent = 0 , |
||
GUIProfile | prof = DefaultGUI |
||
) |
Constructs a new KHTMLPart.
KHTML basically consists of two objects: The KHTMLPart itself, holding the document data (DOM document), and the KHTMLView, derived from QScrollArea, in which the document content is rendered in. You can specify two different parent objects for a KHTMLPart, one parent for the KHTMLPart document and one parent for the KHTMLView. If the second parent
argument is 0L, then parentWidget
is used as parent for both objects, the part and the view.
Definition at line 181 of file khtml_part.cpp.
KHTMLPart::KHTMLPart | ( | KHTMLView * | view, |
QObject * | parent = 0 , |
||
GUIProfile | prof = DefaultGUI |
||
) |
Constructs a new KHTMLPart.
This constructor is useful if you wish to subclass KHTMLView. If the view
passed as first argument to the constructor was built with a null KHTMLPart pointer, then the newly created KHTMLPart will be assigned as the view's part.
Therefore, you might either initialize the view as part of the initialization list of your derived KHTMLPart class constructor:
Or separately build the KHTMLView beforehand:
Definition at line 190 of file khtml_part.cpp.
|
virtual |
Destructor.
Definition at line 532 of file khtml_part.cpp.
Member Function Documentation
DOM::Node KHTMLPart::activeNode | ( | ) | const |
Returns the node that has the keyboard focus.
Definition at line 6933 of file khtml_part.cpp.
bool KHTMLPart::autoloadImages | ( | ) | const |
Returns whether images contained in the document are loaded automatically or not.
- Note
- that the returned information is unrelieable as long as no begin() was called.
Definition at line 1478 of file khtml_part.cpp.
KUrl KHTMLPart::backgroundURL | ( | ) | const |
Returns the URL for the background Image (used by save background)
Definition at line 3931 of file khtml_part.cpp.
KUrl KHTMLPart::baseURL | ( | ) | const |
Definition at line 2514 of file khtml_part.cpp.
Clears the widget and prepares it for new content.
If you want url() to return for example "file:/tmp/test.html", you can use the following code:
- Parameters
-
url is the url of the document to be displayed. Even if you are generating the HTML on the fly, it may be useful to specify a directory so that any pixmaps are found. xOffset is the initial horizontal scrollbar value. Usually you don't want to use this. yOffset is the initial vertical scrollbar value. Usually you don't want to use this.
All child frames and the old document are removed if you call this method.
Definition at line 1993 of file khtml_part.cpp.
KParts::BrowserExtension * KHTMLPart::browserExtension | ( | ) | const |
Returns a pointer to the KParts::BrowserExtension.
Definition at line 1049 of file khtml_part.cpp.
KParts::BrowserHostExtension * KHTMLPart::browserHostExtension | ( | ) | const |
Definition at line 1054 of file khtml_part.cpp.
KHTMLPart::CaretDisplayPolicy KHTMLPart::caretDisplayPolicyNonFocused | ( | ) | const |
Returns the current caret policy when the view is not focused.
Definition at line 2930 of file khtml_part.cpp.
|
signal |
This signal is emitted whenever the caret position has been changed.
The signal transmits the position the DOM::Range way, the node and the zero-based offset within this node.
- Parameters
-
node node which the caret is in. This can be null if the caret has been deactivated. offset offset within the node. If the node is null, the offset is meaningless.
|
virtual |
Stops loading the document and kills all data requests (for images, etc.)
Reimplemented from KParts::ReadOnlyPart.
Definition at line 922 of file khtml_part.cpp.
returns a KUrl object for the given url.
Use when you know what you're doing.
Definition at line 2522 of file khtml_part.cpp.
|
signal |
Emitted whenever the configuration has changed.
|
protectedvirtual |
This method is called when a new embedded object (include html frames) is to be created.
Reimplement it if you want to add support for certain embeddable objects without registering them in the KDE wide registry system (KSyCoCa) . Another reason for re-implementing this method could be if you want to derive from KTHMLPart and also want all html frame objects to be a object of your derived type, in which case you should return a new instance for the mimetype 'text/html' .
Definition at line 4608 of file khtml_part.cpp.
KParts::ReadOnlyPart * KHTMLPart::currentFrame | ( | ) | const |
Return the current frame (the one that has focus) Not necessarily a direct child of ours, framesets can be nested.
Returns "this" if this part isn't a frameset.
Definition at line 5299 of file khtml_part.cpp.
|
protectedvirtual |
Reimplemented from KParts::Part.
Definition at line 6087 of file khtml_part.cpp.
bool KHTMLPart::dndEnabled | ( | ) | const |
Returns whether Dragn'n'Drop support is enabled or not.
DNSPrefetch KHTMLPart::dnsPrefetch | ( | ) | const |
Returns currently set DNS prefetching mode.
See DNSPrefetch
enum for explanation of values.
- Note
- Always returns
DNSPrefetchDisabled
if setOnlyLocalReferences() mode is enabled.
- Since
- 4.2
|
signal |
|
protectedvirtual |
Implements the streaming API of KParts::ReadOnlyPart.
Definition at line 2187 of file khtml_part.cpp.
DOM::Document KHTMLPart::document | ( | ) | const |
Returns a reference to the DOM document.
Definition at line 1012 of file khtml_part.cpp.
QString KHTMLPart::documentSource | ( | ) | const |
Returns the content of the source document.
Definition at line 1017 of file khtml_part.cpp.
Implements the streaming API of KParts::ReadOnlyPart.
Definition at line 2170 of file khtml_part.cpp.
|
protectedvirtual |
Implements the streaming API of KParts::ReadOnlyPart.
Definition at line 2181 of file khtml_part.cpp.
DOM::Editor * KHTMLPart::editor | ( | ) | const |
Returns the instance of the attached html editor interface.
Definition at line 3500 of file khtml_part.cpp.
QString KHTMLPart::encoding | ( | ) | const |
Returns the encoding the page currently uses.
Note that the encoding might be different from the charset.
|
virtual |
Call this after your last call to write().
Definition at line 2138 of file khtml_part.cpp.
Same as executeScript( const QString & ) except with the Node parameter specifying the 'this' value.
Definition at line 1326 of file khtml_part.cpp.
Execute the specified snippet of JavaScript code.
Returns true
if JavaScript was enabled, no error occurred and the code returned true
itself or false
otherwise.
- Deprecated:
- , use executeString( DOM::Node(), script)
Definition at line 1321 of file khtml_part.cpp.
Finds a frame by name.
Returns 0L if frame can't be found.
Definition at line 5283 of file khtml_part.cpp.
KHTMLPart * KHTMLPart::findFrameParent | ( | KParts::ReadOnlyPart * | callingPart, |
const QString & | f, | ||
khtml::ChildFrame ** | childFrame = 0 |
||
) |
Recursively finds the part containing the frame with name f
and checks if it is accessible by callingPart
Returns 0L if no suitable frame can't be found.
Returns parent part if a suitable frame was found and frame info in *childFrame
Definition at line 5190 of file khtml_part.cpp.
KParts::ReadOnlyPart * KHTMLPart::findFramePart | ( | const QString & | f | ) |
Finds a frame by name.
Returns 0L if frame can't be found.
Definition at line 5293 of file khtml_part.cpp.
void KHTMLPart::findText | ( | ) |
Starts a new search by popping up a dialog asking the user what he wants to search for.
Definition at line 3053 of file khtml_part.cpp.
void KHTMLPart::findText | ( | const QString & | str, |
long | options, | ||
QWidget * | parent = 0 , |
||
KFindDialog * | findDialog = 0 |
||
) |
Starts a new search, but bypasses the user dialog.
- Parameters
-
str The string to search for. options Find options. parent Parent used for centering popups like "string not found". findDialog Optionally, you can supply your own dialog.
Definition at line 3060 of file khtml_part.cpp.
void KHTMLPart::findTextBegin | ( | ) |
Initiates a text search.
Definition at line 2969 of file khtml_part.cpp.
Finds the next occurrence of a string set by findText()
- Parameters
-
reverse if true
, revert seach direction (only if no find dialog is used)
- Returns
true
if a new match was found.
Definition at line 3068 of file khtml_part.cpp.
int KHTMLPart::fontScaleFactor | ( | ) | const |
Returns the current font scale factor.
Definition at line 5886 of file khtml_part.cpp.
bool KHTMLPart::forcePermitLocalImages | ( | ) | const |
If true, local image files will be loaded even when forbidden by the Kiosk/KAuthorized policies ( default false
).
See setForcePermitLocalImages.
- Since
- 4.6
Definition at line 2803 of file khtml_part.cpp.
KHTMLPart::FormNotification KHTMLPart::formNotification | ( | ) | const |
Determine if signal should be emitted before, instead or never when a submitForm() happens.
KDE5 remove me
Definition at line 7328 of file khtml_part.cpp.
|
signal |
If form notification is on, this will be emitted either for a form submit or before the form submit according to the setting.
KDE4 remove me
Returns whether a frame with the specified name is exists or not.
In contrast to the findFrame method this one also returns true
if the frame is defined but no displaying component has been found/loaded, yet.
Definition at line 5314 of file khtml_part.cpp.
KJSProxy * KHTMLPart::framejScript | ( | KParts::ReadOnlyPart * | framePart | ) |
Returns child frame framePart its script interpreter.
Definition at line 5336 of file khtml_part.cpp.
QStringList KHTMLPart::frameNames | ( | ) | const |
Returns a list of names of all frame (including iframe) objects of the current document.
Note that this method is not working recursively for sub-frames.
Definition at line 6033 of file khtml_part.cpp.
QList< KParts::ReadOnlyPart * > KHTMLPart::frames | ( | ) | const |
Definition at line 6046 of file khtml_part.cpp.
Finds the anchor named name
.
If the anchor is found, the widget scrolls to the closest position. Returns if
the anchor has been found.
Definition at line 2708 of file khtml_part.cpp.
|
protectedvirtual |
Internal reimplementation of KParts::Part::guiActivateEvent .
Reimplemented from KParts::ReadOnlyPart.
Definition at line 6626 of file khtml_part.cpp.
bool KHTMLPart::hasSelection | ( | ) | const |
Has the user selected anything?
Call selectedText() to retrieve the selected text.
- Returns
true
if there is text selected.
Definition at line 3256 of file khtml_part.cpp.
void KHTMLPart::hide | ( | ) |
DOM::HTMLDocument KHTMLPart::htmlDocument | ( | ) | const |
Returns a reference to the DOM HTML document (for non-HTML documents, returns null)
Definition at line 1004 of file khtml_part.cpp.
presents a detailed error message to the user.
errorCode
kio error code, eg KIO::ERR_SERVER_TIMEOUT. text
kio additional information text. url
the url that triggered the error.
Definition at line 1807 of file khtml_part.cpp.
|
inline |
Access to internal APIs. Don't use outside
Definition at line 1937 of file khtml_part.h.
bool KHTMLPart::inProgress | ( | ) | const |
Definition at line 7447 of file khtml_part.cpp.
bool KHTMLPart::isCaretMode | ( | ) | const |
Returns whether caret mode is on/off.
Definition at line 2884 of file khtml_part.cpp.
bool KHTMLPart::isEditable | ( | ) | const |
Returns true
if the document is editable, false
otherwise.
Definition at line 2904 of file khtml_part.cpp.
bool KHTMLPart::isModified | ( | ) | const |
Checks whether the page contains unsubmitted form changes.
- Returns
true
if form changes exist
Definition at line 7344 of file khtml_part.cpp.
bool KHTMLPart::isPointInsideSelection | ( | int | x, |
int | y | ||
) |
Returns whether the given point is inside the current selection.
The coordinates are content-coordinates.
Definition at line 6122 of file khtml_part.cpp.
bool KHTMLPart::javaEnabled | ( | ) | const |
Return true
if Java applet support is enabled, false
if disabled.
bool KHTMLPart::jScriptEnabled | ( | ) | const |
Returns true
if Javascript support is enabled or false
otherwise.
Definition at line 1106 of file khtml_part.cpp.
KJS::Interpreter * KHTMLPart::jScriptInterpreter | ( | ) |
Returns the JavaScript interpreter the part is using.
This method is mainly intended for applications which embed and extend the part and provides a mechanism for adding additional native objects to the interpreter (or removing the built-ins).
One thing people using this method to add things to the interpreter must consider, is that when you start writing new content to the part, the interpreter is cleared. This includes both use of the begin( const KUrl &, int, int ) method, and the openUrl( const KUrl & ) method. If you want your objects to have a longer lifespan, then you must retain a KJS::Object yourself to ensure that the reference count of your custom objects never reaches 0. You will also need to re-add your bindings every time this happens - one way to detect the need for this is to connect to the docCreated() signal, another is to reimplement the begin() method.
Definition at line 1083 of file khtml_part.cpp.
QString KHTMLPart::jsDefaultStatusBarText | ( | ) | const |
Called by KJS.
Returns the DefaultStatusBarText assigned via window.defaultStatus
Definition at line 5938 of file khtml_part.cpp.
QString KHTMLPart::jsStatusBarText | ( | ) | const |
Called by KJS.
Returns the StatusBarText assigned via window.status
Definition at line 5933 of file khtml_part.cpp.
|
protectedvirtual |
Eventhandler for the khtml::DrawContentsEvent.
Definition at line 6622 of file khtml_part.cpp.
|
protectedvirtual |
Eventhandler for the khtml::MouseDoubleClickEvent.
Definition at line 6341 of file khtml_part.cpp.
|
protectedvirtual |
Eventhandler for the khtml::MouseMouseMoveEvent.
Definition at line 6550 of file khtml_part.cpp.
|
protectedvirtual |
Eventhandler of the khtml::MousePressEvent.
Definition at line 6295 of file khtml_part.cpp.
|
protectedvirtual |
Eventhandler for the khtml::MouseMouseReleaseEvent.
Definition at line 6561 of file khtml_part.cpp.
QString KHTMLPart::lastModified | ( | ) | const |
Last-modified date (in raw string format), if received in the [HTTP] headers.
Will pre-resolve name
according to dnsPrefetch current settings Returns true
if the name will be pre-resolved.
Otherwise returns false.
Definition at line 3460 of file khtml_part.cpp.
bool KHTMLPart::metaRefreshEnabled | ( | ) | const |
Returns true
if automatic forwarding is enabled.
bool KHTMLPart::nextAnchor | ( | ) |
Go to the next anchor.
This is useful to navigate from outside the navigator
Definition at line 2755 of file khtml_part.cpp.
|
signal |
This signal is emitted when an element retrieves the keyboard focus.
Note that the signal argument can be a null node if no element is active, meaning a node has explicitly been deactivated without a new one becoming active.
DOM::Node KHTMLPart::nodeUnderMouse | ( | ) | const |
Returns the Node
currently under the mouse.
The returned node may be a shared node (e. g. an <area> node if the mouse is hovering over an image map).
Definition at line 5692 of file khtml_part.cpp.
DOM::Node KHTMLPart::nonSharedNodeUnderMouse | ( | ) | const |
Returns the Node
currently under the mouse that is not shared.
The returned node is always the node that is physically under the mouse pointer (irrespective of logically overlying elements like, e. g., <area> on image maps).
Definition at line 5697 of file khtml_part.cpp.
bool KHTMLPart::onlyLocalReferences | ( | ) | const |
Returns whether only file:/ or data:/ references are allowed to be loaded ( default false
).
See setOnlyLocalReferences.
Definition at line 2793 of file khtml_part.cpp.
|
signal |
Emitted if the cursor is moved over an URL.
|
protectedvirtual |
Internal empty reimplementation of KParts::ReadOnlyPart::openFile .
Reimplemented from KParts::ReadOnlyPart.
Definition at line 1628 of file khtml_part.cpp.
Opens the specified URL url
.
Reimplemented from KParts::ReadOnlyPart::openUrl .
The format of the error url is that two variables are passed in the query: error = int kio error code, errText = QString error text from kio and the URL where the error happened is passed as a sub URL.
Reimplemented from KParts::ReadOnlyPart.
Definition at line 665 of file khtml_part.cpp.
QString KHTMLPart::pageReferrer | ( | ) | const |
Referrer used to obtain this page.
Definition at line 5948 of file khtml_part.cpp.
Paints the HTML page to a QPainter.
See KHTMLView::paint for details
Definition at line 2194 of file khtml_part.cpp.
KHTMLPart * KHTMLPart::parentPart | ( | ) |
Returns a pointer to the parent KHTMLPart if the part is a frame in an HTML frameset.
Returns 0L otherwise.
Definition at line 5356 of file khtml_part.cpp.
KParts::PartManager * KHTMLPart::partManager | ( | ) |
Returns a reference to the partmanager instance which manages html frame objects.
Definition at line 4661 of file khtml_part.cpp.
Definition at line 6988 of file khtml_part.cpp.
bool KHTMLPart::pluginsEnabled | ( | ) | const |
Returns true
if plugins are enabled, false
if disabled.
Emitted when the user clicks the right mouse button on the document.
See KParts::BrowserExtension for two more popupMenu signals emitted by khtml, with much more information in the signal.
Loads a script into the script cache.
Definition at line 6973 of file khtml_part.cpp.
Loads a style sheet into the stylesheet cache.
Definition at line 6968 of file khtml_part.cpp.
bool KHTMLPart::prevAnchor | ( | ) |
Go to previous anchor.
Definition at line 2764 of file khtml_part.cpp.
QString KHTMLPart::referrer | ( | ) | const |
Referrer used for links in this page.
Definition at line 5943 of file khtml_part.cpp.
bool KHTMLPart::restored | ( | ) | const |
Definition at line 6983 of file khtml_part.cpp.
|
virtual |
Restores the KHTMLPart's previously saved state (including child frame objects) from the provided QDataStream.
- See also
- saveState()
This is called from the restoreState() method of the browserExtension() .
Definition at line 5482 of file khtml_part.cpp.
|
virtual |
Saves the KHTMLPart's complete state (including child frame objects) to the provided QDataStream.
This is called from the saveState() method of the browserExtension().
Definition at line 5394 of file khtml_part.cpp.
Schedules a redirection after delay
seconds.
Definition at line 2560 of file khtml_part.cpp.
void KHTMLPart::selectAll | ( | ) |
Marks all text in the document as selected.
Definition at line 6746 of file khtml_part.cpp.
|
virtual |
Returns the text the user has marked.
Definition at line 3098 of file khtml_part.cpp.
QString KHTMLPart::selectedTextAsHTML | ( | ) | const |
Return the text the user has marked.
This is guaranteed to be valid xml, and to contain the <html> and <body> tags.
FIXME probably should make virtual for 4.0 ?
Definition at line 3080 of file khtml_part.cpp.
DOM::Range KHTMLPart::selection | ( | ) | const |
Returns the selected part of the HTML.
Definition at line 3261 of file khtml_part.cpp.
void KHTMLPart::selection | ( | DOM::Node & | startNode, |
long & | startOffset, | ||
DOM::Node & | endNode, | ||
long & | endOffset | ||
) | const |
Returns the selected part of the HTML by returning the starting and end position.
If there is no selection, both nodes and offsets are equal.
- Parameters
-
startNode returns node selection starts in startOffset returns offset within starting node endNode returns node selection ends in endOffset returns offset within end node.
Definition at line 3266 of file khtml_part.cpp.
|
signal |
This signal is emitted when the selection changes.
|
slot |
Sets the focused node of the document to the specified node.
If the node is a form control, the control will receive focus in the same way that it would if the user had clicked on it or tabbed to it with the keyboard. For most other types of elements, there is no visual indication of whether or not they are focused.
See activeNode
- Parameters
-
node The node to focus
Definition at line 6919 of file khtml_part.cpp.
void KHTMLPart::setAlwaysHonourDoctype | ( | bool | b = true | ) |
Sets whether the document's Doctype should always be used to determine the parsing mode for the document.
Without this, parsing will be forced to strict mode when using the write( const QString &str ) method for backward compatibility reasons.
Definition at line 2114 of file khtml_part.cpp.
void KHTMLPart::setAutoloadImages | ( | bool | enable | ) |
Specifies whether images contained in the document should be loaded automatically or not.
- Note
- Request will be ignored if called before begin().
Definition at line 1450 of file khtml_part.cpp.
void KHTMLPart::setCaretDisplayPolicyNonFocused | ( | CaretDisplayPolicy | policy | ) |
Sets the caret display policy when the view is not focused.
Whenever the caret is in use, this property determines how the caret should be displayed when the document view is not focused.
The default policy is CaretInvisible.
- Parameters
-
policy new display policy
Definition at line 2942 of file khtml_part.cpp.
|
slot |
Enables/disables caret mode.
Enabling caret mode displays a caret which can be used to navigate the document using the keyboard only. Caret mode is switched off by default.
- Parameters
-
enable true
to enable,false
to disable caret mode.
Definition at line 2867 of file khtml_part.cpp.
Sets the caret to the given position.
If the given location is invalid, it will snap to the nearest valid location. Immediately afterwards a caretPositionChanged
signal containing the effective position is emitted
- Parameters
-
node node to set to offset zero-based offset within the node extendSelection If true
, a selection will be spanned from the last caret position to the given one. Otherwise, any existing selection will be deselected.
Definition at line 2913 of file khtml_part.cpp.
|
slot |
Sets the visibility of the caret.
This methods displays or hides the caret regardless of the current caret display policy (see setCaretDisplayNonFocused), and regardless of focus.
The caret will be shown/hidden only under at least one of the following conditions:
- the document is editable
- the document is in caret mode
- the document's currently focused element is editable
- Parameters
-
show true
to make visible,false
to hide.
Definition at line 2952 of file khtml_part.cpp.
void KHTMLPart::setDNDEnabled | ( | bool | b | ) |
Enables or disables Drag'n'Drop support.
A drag operation is started if the users drags a link.
Definition at line 6077 of file khtml_part.cpp.
void KHTMLPart::setDNSPrefetch | ( | DNSPrefetch | pmode | ) |
Sets whether DNS Names found in loaded documents'anchors should be pre-fetched (pre-resolved).
Note that calling this function will permanently override the User settings about DNS prefetch support. Not calling this function is the only way to let the default settings apply.
- Note
- This setting has no effect if setOnlyLocalReferences() mode is enabled.
- Parameters
-
pmode the mode to set. See DNSPrefetch enum for explanation of values.
- Since
- 4.2
Definition at line 1115 of file khtml_part.cpp.
|
slot |
Makes the document editable.
Setting this property to true
makes the document, and its subdocuments (such as frames, iframes, objects) editable as a whole. FIXME: insert more information about navigation, features etc. as seen fit
- Parameters
-
enable true
to set document editable,false
to set it read-only.
Definition at line 2889 of file khtml_part.cpp.
Sets the encoding the page uses.
This can be different from the charset. The widget will try to reload the current page in the new encoding, if url() is not empty.
Definition at line 2654 of file khtml_part.cpp.
void KHTMLPart::setFixedFont | ( | const QString & | name | ) |
Sets the fixed font style.
- Parameters
-
name The font name to use for fixed text, e.g. the <pre>
tag.
Definition at line 2778 of file khtml_part.cpp.
void KHTMLPart::setFontScaleFactor | ( | int | percent | ) |
Sets the scale factor to be applied to fonts.
The value is given in percent, larger values mean generally larger fonts.
The given value should be in the range of 20..300, values outside that range are not guaranteed to work. A value of 100 will disable all scaling of font sizes and show the page with the sizes determined via the given lengths in the stylesheets.
Definition at line 5863 of file khtml_part.cpp.
void KHTMLPart::setForcePermitLocalImages | ( | bool | enable | ) |
Security option.
If this is set to true, content loaded from external URLs will be permitted to access images on disk regardless of of the Kiosk policy. You should be careful in enabling this, as it may make it easier to fake any HTML-based chrome or to perform other such user-confusion attack. false
by default.
- Since
- 4.6
Definition at line 2808 of file khtml_part.cpp.
void KHTMLPart::setFormNotification | ( | KHTMLPart::FormNotification | fn | ) |
Determine if signal should be emitted before, instead or never when a submitForm() happens.
KDE5 remove me
Definition at line 7324 of file khtml_part.cpp.
void KHTMLPart::setJavaEnabled | ( | bool | enable | ) |
Enables/disables Java applet support.
Note that calling this function will permanently override the User settings about Java applet support. Not calling this function is the only way to let the default settings apply.
Definition at line 1363 of file khtml_part.cpp.
void KHTMLPart::setJScriptEnabled | ( | bool | enable | ) |
Enable/disable Javascript support.
Note that this will in either case permanently override the default usersetting. If you want to have the default UserSettings, don't call this method.
Definition at line 1097 of file khtml_part.cpp.
void KHTMLPart::setJSDefaultStatusBarText | ( | const QString & | text | ) |
Called by KJS.
Sets the DefaultStatusBarText assigned via window.defaultStatus
Definition at line 5928 of file khtml_part.cpp.
void KHTMLPart::setJSStatusBarText | ( | const QString & | text | ) |
Called by KJS.
Sets the StatusBarText assigned via window.status
Definition at line 5923 of file khtml_part.cpp.
void KHTMLPart::setMetaRefreshEnabled | ( | bool | enable | ) |
Enable/disable automatic forwarding by <meta http-equiv="refresh" ....
>
Definition at line 1128 of file khtml_part.cpp.
void KHTMLPart::setOnlyLocalReferences | ( | bool | enable | ) |
Security option.
Specify whether only file:/ or data:/ urls are allowed to be loaded without user confirmation by KHTML. ( for example referenced by stylesheets, images, scripts, subdocuments, embedded elements ).
This option is mainly intended for enabling the "mail reader mode", where you load untrusted content with a file:/ url.
Please note that enabling this option currently automatically disables Javascript, Java and Plugins support. This might change in the future if the security model is becoming more sophisticated, so don't rely on this behaviour.
( default false
- everything is loaded unless forbidden by KApplication::authorizeURLAction).
Definition at line 2798 of file khtml_part.cpp.
|
protected |
Definition at line 1657 of file khtml_part.cpp.
|
protected |
Definition at line 6998 of file khtml_part.cpp.
void KHTMLPart::setPluginsEnabled | ( | bool | enable | ) |
Enables or disables plugins, default is enabled.
Definition at line 1382 of file khtml_part.cpp.
void KHTMLPart::setSelection | ( | const DOM::Range & | r | ) |
Sets the current selection.
Definition at line 3275 of file khtml_part.cpp.
void KHTMLPart::setStandardFont | ( | const QString & | name | ) |
Sets the standard font style.
- Parameters
-
name The font name to use for standard text.
Definition at line 2773 of file khtml_part.cpp.
void KHTMLPart::setStatusMessagesEnabled | ( | bool | enable | ) |
Enable/disable statusbar messages.
When this class wants to set the statusbar text, it emits setStatusBarText(const QString & text) If you want to catch this for your own statusbar, note that it returns back a rich text string, starting with "<qt>". This you need to either pass this into your own QLabel or to strip out the tags before passing it to QStatusBar::message(const QString & message)
Definition at line 1078 of file khtml_part.cpp.
Shows or hides the suppressed popup indicator.
Definition at line 7369 of file khtml_part.cpp.
const KHTMLSettings * KHTMLPart::settings | ( | ) | const |
Definition at line 2508 of file khtml_part.cpp.
void KHTMLPart::setURLCursor | ( | const QCursor & | c | ) |
Sets the cursor to use when the cursor is on a link.
Definition at line 2783 of file khtml_part.cpp.
void KHTMLPart::setUserStyleSheet | ( | const KUrl & | url | ) |
Sets a user defined style sheet to be used on top of the HTML 4 default style sheet.
This gives a wide range of possibilities to change the layout of the page.
To have an effect this function has to be called after calling begin().
Definition at line 2696 of file khtml_part.cpp.
void KHTMLPart::setUserStyleSheet | ( | const QString & | styleSheet | ) |
Sets a user defined style sheet to be used on top of the HTML 4 default style sheet.
This gives a wide range of possibilities to change the layout of the page.
To have an effect this function has to be called after calling begin().
Definition at line 2702 of file khtml_part.cpp.
void KHTMLPart::setZoomFactor | ( | int | percent | ) |
Sets the Zoom factor.
The value is given in percent, larger values mean a generally larger font and larger page contents.
The given value should be in the range of 20..300, values outside that range are not guaranteed to work. A value of 100 will disable all zooming and show the page with the sizes determined via the given lengths in the stylesheets.
Definition at line 5778 of file khtml_part.cpp.
void KHTMLPart::show | ( | ) |
|
virtual |
Called when a certain error situation (i.e.
connection timed out) occurred. The default implementation either shows a KIO error dialog or loads a more verbose error description a as page, depending on the users configuration. job
is the job that signaled the error situation
Definition at line 1790 of file khtml_part.cpp.
|
protectedvirtualslot |
Called when the job downloading the page is finished.
Can be reimplemented, for instance to get metadata out of the job, but make sure to call KHTMLPart::slotFinished() too.
Definition at line 1907 of file khtml_part.cpp.
|
inlineprotectedvirtual |
Hook for adding code before a job is started.
This can be used to add metadata, like job->addMetaData("PropagateHttpHeader", "true") to get the HTTP headers.
Definition at line 1404 of file khtml_part.h.
bool KHTMLPart::statusMessagesEnabled | ( | ) | const |
Returns true
if status messages are enabled.
Definition at line 1092 of file khtml_part.cpp.
|
slot |
Stops all animated images on the current and child pages.
Definition at line 2200 of file khtml_part.cpp.
|
slot |
Definition at line 4687 of file khtml_part.cpp.
|
protectedvirtual |
Reimplemented from QObject.
Definition at line 3437 of file khtml_part.cpp.
KUrl KHTMLPart::toplevelURL | ( | ) |
Returns the toplevel (origin) URL of this document, even if this part is a frame or an iframe.
- Returns
- the actual original url.
Definition at line 7332 of file khtml_part.cpp.
|
protected |
Definition at line 5793 of file khtml_part.cpp.
QCursor KHTMLPart::urlCursor | ( | ) | const |
Returns the cursor which is used when the cursor is on a link.
Definition at line 2788 of file khtml_part.cpp.
|
protectedvirtual |
Definition at line 3690 of file khtml_part.cpp.
KHTMLView * KHTMLPart::view | ( | ) | const |
Returns a pointer to the HTML document's view.
Definition at line 1059 of file khtml_part.cpp.
|
virtual |
Writes another part of the HTML code to the widget.
You may call this function many times in sequence. But remember: The fewer calls you make, the faster the widget will be.
The HTML code is send through a decoder which decodes the stream to Unicode.
The len
parameter is needed for streams encoded in utf-16, since these can have \0 chars in them. In case the encoding you're using isn't utf-16, you can safely leave out the length parameter.
Attention: Don't mix calls to write( const char *) with calls to write( const QString & ).
The result might not be what you want.
Definition at line 2089 of file khtml_part.cpp.
|
virtual |
Writes another part of the HTML code to the widget.
You may call this function many times in sequence. But remember: The fewer calls you make, the faster the widget will be.
For historic and backward compatibility reasons, this method will force the use of strict mode for the document, unless setAlwaysHonourDoctype() has been called previously.
Definition at line 2119 of file khtml_part.cpp.
int KHTMLPart::zoomFactor | ( | ) | const |
Returns the current zoom factor.
Definition at line 5714 of file khtml_part.cpp.
Property Documentation
|
readwrite |
Definition at line 257 of file khtml_part.h.
|
readwrite |
Definition at line 259 of file khtml_part.h.
|
readwrite |
Definition at line 267 of file khtml_part.h.
|
readwrite |
Definition at line 256 of file khtml_part.h.
|
readwrite |
Definition at line 255 of file khtml_part.h.
|
read |
Definition at line 268 of file khtml_part.h.
|
readwrite |
Definition at line 269 of file khtml_part.h.
|
read |
Definition at line 266 of file khtml_part.h.
|
readwrite |
Definition at line 258 of file khtml_part.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:26:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.