KIO
#include <forwardingslavebase.h>
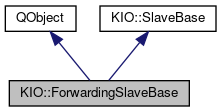
Public Member Functions | |
ForwardingSlaveBase (const QByteArray &protocol, const QByteArray &poolSocket, const QByteArray &appSocket) | |
virtual | ~ForwardingSlaveBase () |
virtual void | chmod (const KUrl &url, int permissions) |
virtual void | copy (const KUrl &src, const KUrl &dest, int permissions, JobFlags flags) |
virtual void | del (const KUrl &url, bool isfile) |
virtual void | get (const KUrl &url) |
virtual void | listDir (const KUrl &url) |
virtual void | mimetype (const KUrl &url) |
virtual void | mkdir (const KUrl &url, int permissions) |
virtual void | put (const KUrl &url, int permissions, JobFlags flags) |
virtual void | rename (const KUrl &src, const KUrl &dest, JobFlags flags) |
virtual void | setModificationTime (const KUrl &url, const QDateTime &mtime) |
virtual void | stat (const KUrl &url) |
virtual void | symlink (const QString &target, const KUrl &dest, JobFlags flags) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
SlaveBase (const QByteArray &protocol, const QByteArray &pool_socket, const QByteArray &app_socket) | |
virtual | ~SlaveBase () |
MetaData | allMetaData () const |
bool | cacheAuthentication (const AuthInfo &info) |
bool | canResume (KIO::filesize_t offset) |
void | canResume () |
bool | checkCachedAuthentication (AuthInfo &info) |
virtual void | chown (const KUrl &url, const QString &owner, const QString &group) |
virtual void | close () |
virtual void | closeConnection () |
KConfigGroup * | config () |
void | connected () |
void | connectSlave (const QString &path) |
int | connectTimeout () |
void | data (const QByteArray &data) |
void | dataReq () |
void | disconnectSlave () |
virtual void | dispatch (int command, const QByteArray &data) |
void | dispatchLoop () |
virtual void | dispatchOpenCommand (int command, const QByteArray &data) |
void | dropNetwork (const QString &host=QString()) |
void | error (int _errid, const QString &_text) |
void | errorPage () |
void | exit () |
void | finished () |
bool | hasMetaData (const QString &key) const |
void | infoMessage (const QString &msg) |
void | listEntries (const UDSEntryList &_entry) |
void | listEntry (const UDSEntry &_entry, bool ready) |
void | lookupHost (const QString &host) |
int | messageBox (MessageBoxType type, const QString &text, const QString &caption=QString(), const QString &buttonYes=i18n("&Yes"), const QString &buttonNo=i18n("&No")) |
int | messageBox (const QString &text, MessageBoxType type, const QString &caption=QString(), const QString &buttonYes=i18n("&Yes"), const QString &buttonNo=i18n("&No"), const QString &dontAskAgainName=QString()) |
QString | metaData (const QString &key) const |
void | mimeType (const QString &_type) |
virtual void | multiGet (const QByteArray &data) |
void | needSubUrlData () |
virtual void | open (const KUrl &url, QIODevice::OpenMode mode) |
virtual void | openConnection () |
void | opened () |
bool | openPasswordDialog (KIO::AuthInfo &info, const QString &errorMsg=QString()) |
void | position (KIO::filesize_t _pos) |
void | processedPercent (float percent) |
void | processedSize (KIO::filesize_t _bytes) |
int | proxyConnectTimeout () |
virtual void | read (KIO::filesize_t size) |
int | readData (QByteArray &buffer) |
int | readTimeout () |
void | redirection (const KUrl &_url) |
KRemoteEncoding * | remoteEncoding () |
virtual void | reparseConfiguration () |
bool | requestNetwork (const QString &host=QString()) |
int | responseTimeout () |
virtual void | seek (KIO::filesize_t offset) |
void | sendAndKeepMetaData () |
void | sendMetaData () |
virtual void | setHost (const QString &host, quint16 port, const QString &user, const QString &pass) |
void | setKillFlag () |
virtual void | setLinkDest (const KUrl &url, const QString &target) |
void | setMetaData (const QString &key, const QString &value) |
virtual void | setSubUrl (const KUrl &url) |
void | setTimeoutSpecialCommand (int timeout, const QByteArray &data=QByteArray()) |
virtual void | slave_status () |
void | slaveStatus (const QString &host, bool connected) |
virtual void | special (const QByteArray &data) |
void | speed (unsigned long _bytes_per_second) |
void | statEntry (const UDSEntry &_entry) |
void | totalSize (KIO::filesize_t _bytes) |
int | waitForAnswer (int expected1, int expected2, QByteArray &data, int *pCmd=0) |
int | waitForHostInfo (QHostInfo &info) |
void | warning (const QString &msg) |
bool | wasKilled () const |
virtual void | write (const QByteArray &data) |
void | written (KIO::filesize_t _bytes) |
Protected Member Functions | |
virtual void | prepareUDSEntry (KIO::UDSEntry &entry, bool listing=false) const |
KUrl | processedUrl () const |
KUrl | requestedUrl () const |
virtual bool | rewriteUrl (const KUrl &url, KUrl &newURL)=0 |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
virtual void | virtual_hook (int id, void *data) |
Additional Inherited Members | |
![]() | |
enum | MessageBoxType { QuestionYesNo = 1, WarningYesNo = 2, WarningContinueCancel = 3, WarningYesNoCancel = 4, Information = 5, SSLMessageBox = 6 } |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
enum | VirtualFunctionId { AppConnectionMade = 0 } |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
MetaData | mIncomingMetaData |
MetaData | mOutgoingMetaData |
QByteArray | mProtocol |
![]() | |
objectName | |
Detailed Description
This class should be used as a base for ioslaves acting as a forwarder to other ioslaves.
It has been designed to support only local filesystem like ioslaves.
If the resulting ioslave should be a simple proxy, you only need to implement the ForwardingSlaveBase::rewriteUrl() method.
For more advanced behavior, the classic ioslave methods should be reimplemented, because their default behavior in this class is to forward using the ForwardingSlaveBase::rewriteUrl() method.
A possible code snippet for an advanced stat() behavior would look like this in the child class:
Of course in this case, you surely need to reimplement listDir() and get() accordingly.
If you want view on directories to be correctly refreshed when something changes on a forwarded URL, you'll need a companion kded module to emit the KDirNotify Files*() D-Bus signals.
This class was initially used for media:/ ioslave. This ioslave code and the MediaDirNotify class of its companion kded module can be a good source of inspiration.
Definition at line 88 of file forwardingslavebase.h.
Constructor & Destructor Documentation
KIO::ForwardingSlaveBase::ForwardingSlaveBase | ( | const QByteArray & | protocol, |
const QByteArray & | poolSocket, | ||
const QByteArray & | appSocket | ||
) |
Definition at line 73 of file forwardingslavebase.cpp.
|
virtual |
Definition at line 82 of file forwardingslavebase.cpp.
Member Function Documentation
|
virtual |
Change permissions on url
The slave emits ERR_DOES_NOT_EXIST or ERR_CANNOT_CHMOD.
Reimplemented from KIO::SlaveBase.
Definition at line 320 of file forwardingslavebase.cpp.
|
virtual |
Copy src
into dest
.
By default, copy() is only called when copying a file from yourproto://host/path to yourproto://host/otherpath.
If you set copyFromFile=true then copy() will also be called when moving a file from file:///path to yourproto://host/otherpath. Otherwise such a copy would have to be done the slow way (get+put). See also KProtocolManager::canCopyFromFile().
If you set copyToFile=true then copy() will also be called when moving a file from yourproto: to file:. See also KProtocolManager::canCopyToFile().
If the slave returns an error ERR_UNSUPPORTED_ACTION, the job will ask for get + put instead.
- Parameters
-
src where to copy the file from (decoded) dest where to copy the file to (decoded) permissions may be -1. In this case no special permission mode is set. flags We support Overwrite here
Don't forget to set the modification time of dest
to be the modification time of src
.
Reimplemented from KIO::SlaveBase.
Definition at line 356 of file forwardingslavebase.cpp.
Delete a file or directory.
- Parameters
-
url file/directory to delete isfile if true, a file should be deleted. if false, a directory should be deleted.
By default, del() on a directory should FAIL if the directory is not empty. However, if metadata("recurse") == "true", then the slave can do a recursive deletion. This behavior is only invoked if the slave specifies deleteRecursive=true in its protocol file.
Reimplemented from KIO::SlaveBase.
Definition at line 381 of file forwardingslavebase.cpp.
|
virtual |
get, aka read.
- Parameters
-
url the full url for this request. Host, port and user of the URL can be assumed to be the same as in the last setHost() call.
The slave should first "emit" the mimetype by calling mimeType(), and then "emit" the data using the data() method.
The reason why we need get() to emit the mimetype is: when pasting a URL in krunner, or konqueror's location bar, we have to find out what is the mimetype of that URL. Rather than doing it with a call to mimetype(), then the app or part would have to do a second request to the same server, this is done like this: get() is called, and when it emits the mimetype, the job is put on hold and the right app or part is launched. When that app or part calls get(), the slave is magically reused, and the download can now happen. All with a single call to get() in the slave. This mechanism is also described in KIO::get().
Reimplemented from KIO::SlaveBase.
Definition at line 168 of file forwardingslavebase.cpp.
|
virtual |
Lists the contents of url
.
The slave should emit ERR_CANNOT_ENTER_DIRECTORY if it doesn't exist, if we don't have enough permissions. It should also emit totalFiles as soon as it knows how many files it will list. You should not list files if the path in url
is empty, but redirect to a non-empty path instead.
Reimplemented from KIO::SlaveBase.
Definition at line 242 of file forwardingslavebase.cpp.
|
virtual |
Finds mimetype for one file or directory.
This method should either emit 'mimeType' or it should send a block of data big enough to be able to determine the mimetype.
If the slave doesn't reimplement it, a get will be issued, i.e. the whole file will be downloaded before determining the mimetype on it - this is obviously not a good thing in most cases.
Reimplemented from KIO::SlaveBase.
Definition at line 224 of file forwardingslavebase.cpp.
|
virtual |
Create a directory.
- Parameters
-
url path to the directory to create permissions the permissions to set after creating the directory (-1 if no permissions to be set) The slave emits ERR_COULD_NOT_MKDIR if failure.
Reimplemented from KIO::SlaveBase.
Definition at line 260 of file forwardingslavebase.cpp.
|
protectedvirtual |
Allow to modify a UDSEntry before it's sent to the ioslave endpoint.
This is the default implementation working in most cases, but sometimes you could make use of more forwarding black magic (for example dynamically transform any desktop file into a fake directory...)
- Parameters
-
entry the UDSEntry to post-process listing indicate if this entry it created during a listDir operation
Definition at line 105 of file forwardingslavebase.cpp.
|
protected |
Return the URL being processed by the ioslave Only access it inside prepareUDSEntry()
Definition at line 158 of file forwardingslavebase.cpp.
|
virtual |
put, i.e.
write data into a file.
- Parameters
-
url where to write the file permissions may be -1. In this case no special permission mode is set. flags We support Overwrite here. Hopefully, we're going to support Resume in the future, too. If the file indeed already exists, the slave should NOT apply the permissions change to it. The support for resuming using .part files is done by calling canResume().
IMPORTANT: Use the "modified" metadata in order to set the modification time of the file.
- See also
- canResume()
Reimplemented from KIO::SlaveBase.
Definition at line 186 of file forwardingslavebase.cpp.
|
virtual |
Rename oldname
into newname
.
If the slave returns an error ERR_UNSUPPORTED_ACTION, the job will ask for copy + del instead.
Important: the slave must implement the logic "if the destination already exists, error ERR_DIR_ALREADY_EXIST or ERR_FILE_ALREADY_EXIST". For performance reasons no stat is done in the destination before hand, the slave must do it.
By default, rename() is only called when renaming (moving) from yourproto://host/path to yourproto://host/otherpath.
If you set renameFromFile=true then rename() will also be called when moving a file from file:///path to yourproto://host/otherpath. Otherwise such a move would have to be done the slow way (copy+delete). See KProtocolManager::canRenameFromFile() for more details.
If you set renameToFile=true then rename() will also be called when moving a file from yourproto: to file:. See KProtocolManager::canRenameToFile() for more details.
- Parameters
-
src where to move the file from dest where to move the file to flags We support Overwrite here
Reimplemented from KIO::SlaveBase.
Definition at line 278 of file forwardingslavebase.cpp.
|
protected |
Return the URL asked to the ioslave Only access it inside prepareUDSEntry()
Definition at line 163 of file forwardingslavebase.cpp.
|
protectedpure virtual |
Rewrite an url to its forwarded counterpart.
It should return true if everything was ok, and false otherwise.
If a problem is detected it's up to this method to trigger error() before returning. Returning false silently cancels the current slave operation.
- Parameters
-
url The URL as given during the slave call newURL The new URL to forward the slave call to
- Returns
- true if the given url could be correctly rewritten
|
virtual |
Sets the modification time for For instance this is what CopyJob uses to set mtime on dirs at the end of a copy.
It could also be used to set the mtime on any file, in theory. The usual implementation on unix is to call utime(path, &myutimbuf). The slave emits ERR_DOES_NOT_EXIST or ERR_CANNOT_SETTIME
Reimplemented from KIO::SlaveBase.
Definition at line 338 of file forwardingslavebase.cpp.
|
virtual |
Finds all details for one file or directory.
The information returned is the same as what listDir returns, but only for one file or directory. Call statEntry() after creating the appropriate UDSEntry for this url.
You can use the "details" metadata to optimize this method to only do as much work as needed by the application. By default details is 2 (all details wanted, including modification time, size, etc.), details==1 is used when deleting: we don't need all the information if it takes too much time, no need to follow symlinks etc. details==0 is used for very simple probing: we'll only get the answer "it's a file or a directory (or a symlink), or it doesn't exist".
Reimplemented from KIO::SlaveBase.
Definition at line 206 of file forwardingslavebase.cpp.
|
virtual |
Creates a symbolic link named dest
, pointing to target
, which may be a relative or an absolute path.
- Parameters
-
target The string that will become the "target" of the link (can be relative) dest The symlink to create. flags We support Overwrite here
Reimplemented from KIO::SlaveBase.
Definition at line 301 of file forwardingslavebase.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:55 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.