KCalCore Library
#include <calendar.h>
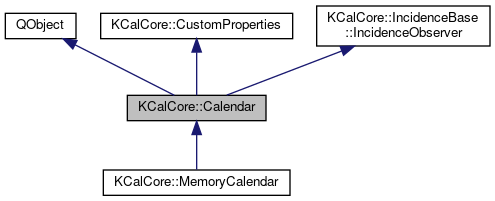
Classes | |
class | CalendarObserver |
Public Types | |
typedef QSharedPointer< Calendar > | Ptr |
Signals | |
void | filterChanged () |
Public Member Functions | |
Calendar (const KDateTime::Spec &timeSpec) | |
Calendar (const QString &timeZoneId) | |
virtual | ~Calendar () |
virtual bool | addEvent (const Event::Ptr &event)=0 |
virtual bool | addIncidence (const Incidence::Ptr &incidence) |
virtual bool | addJournal (const Journal::Ptr &journal)=0 |
bool | addNotebook (const QString ¬ebook, bool isVisible) |
virtual bool | addTodo (const Todo::Ptr &todo)=0 |
virtual Alarm::List | alarms (const KDateTime &from, const KDateTime &to) const =0 |
bool | batchAdding () const |
virtual bool | beginChange (const Incidence::Ptr &incidence) |
QStringList | categories () const |
virtual void | clearNotebookAssociations () |
virtual void | close ()=0 |
QString | defaultNotebook () const |
virtual void | deleteAllEvents ()=0 |
virtual void | deleteAllJournals ()=0 |
virtual void | deleteAllTodos ()=0 |
Incidence::Ptr | deleted (const QString &uid, const KDateTime &recurrenceId=KDateTime()) const |
virtual Event::Ptr | deletedEvent (const QString &uid, const KDateTime &recurrenceId=KDateTime()) const =0 |
virtual Event::List | deletedEvents (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) const =0 |
virtual Journal::Ptr | deletedJournal (const QString &uid, const KDateTime &recurrenceId=KDateTime()) const =0 |
virtual Journal::List | deletedJournals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending) const =0 |
virtual Todo::Ptr | deletedTodo (const QString &uid, const KDateTime &recurrenceId=KDateTime()) const =0 |
virtual Todo::List | deletedTodos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending) const =0 |
virtual bool | deleteEvent (const Event::Ptr &event)=0 |
virtual bool | deleteEventInstances (const Event::Ptr &event)=0 |
virtual bool | deleteIncidence (const Incidence::Ptr &incidence) |
virtual bool | deleteIncidenceInstances (const Incidence::Ptr &incidence)=0 |
virtual bool | deleteJournal (const Journal::Ptr &journal)=0 |
virtual bool | deleteJournalInstances (const Journal::Ptr &journal)=0 |
bool | deleteNotebook (const QString ¬ebook) |
virtual bool | deleteTodo (const Todo::Ptr &todo)=0 |
virtual bool | deleteTodoInstances (const Todo::Ptr &todo)=0 |
KCALCORE_DEPRECATED Incidence::Ptr | dissociateOccurrence (const Incidence::Ptr &incidence, const QDate &date, const KDateTime::Spec &spec, bool single=true) |
virtual Incidence::List | duplicates (const Incidence::Ptr &incidence) |
virtual void | endBatchAdding () |
virtual bool | endChange (const Incidence::Ptr &incidence) |
virtual Event::Ptr | event (const QString &uid, const KDateTime &recurrenceId=KDateTime()) const =0 |
virtual Event::List | eventInstances (const Incidence::Ptr &event, EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) const =0 |
virtual Event::List | events (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) const |
Event::List | events (const KDateTime &dt) const |
Event::List | events (const QDate &start, const QDate &end, const KDateTime::Spec &timeSpec=KDateTime::Spec(), bool inclusive=false) const |
Event::List | events (const QDate &date, const KDateTime::Spec &timeSpec=KDateTime::Spec(), EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) const |
CalFilter * | filter () const |
bool | hasValidNotebook (const QString ¬ebook) const |
Incidence::Ptr | incidence (const QString &uid, const KDateTime &recurrenceId=KDateTime()) const |
virtual Incidence::Ptr | incidenceFromSchedulingID (const QString &sid) const |
virtual Incidence::List | incidences () const |
virtual Incidence::List | incidences (const QDate &date) const |
virtual Incidence::List | incidences (const QString ¬ebook) const |
virtual Incidence::List | incidencesFromSchedulingID (const QString &sid) const |
virtual Incidence::List | instances (const Incidence::Ptr &incidence) const |
bool | isAncestorOf (const Incidence::Ptr &ancestor, const Incidence::Ptr &incidence) const |
bool | isModified () const |
virtual bool | isSaving () const |
bool | isVisible (const Incidence::Ptr &incidence) const |
virtual Journal::Ptr | journal (const QString &uid, const KDateTime &recurrenceId=KDateTime()) const =0 |
virtual Journal::List | journalInstances (const Incidence::Ptr &journal, JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending) const =0 |
virtual Journal::List | journals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending) const |
virtual Journal::List | journals (const QDate &date) const |
virtual QString | notebook (const Incidence::Ptr &incidence) const |
virtual QString | notebook (const QString &uid) const |
virtual QStringList | notebooks () const |
Person::Ptr | owner () const |
QString | productId () const |
virtual Event::List | rawEvents (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) const =0 |
virtual Event::List | rawEvents (const QDate &start, const QDate &end, const KDateTime::Spec &timeSpec=KDateTime::Spec(), bool inclusive=false) const =0 |
virtual Event::List | rawEventsForDate (const KDateTime &dt) const =0 |
virtual Event::List | rawEventsForDate (const QDate &date, const KDateTime::Spec &timeSpec=KDateTime::Spec(), EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) const =0 |
virtual Incidence::List | rawIncidences () const |
virtual Journal::List | rawJournals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending) const =0 |
virtual Journal::List | rawJournalsForDate (const QDate &date) const =0 |
virtual Todo::List | rawTodos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending) const =0 |
virtual Todo::List | rawTodos (const QDate &start, const QDate &end, const KDateTime::Spec ×pec=KDateTime::Spec(), bool inclusive=false) const =0 |
virtual Todo::List | rawTodosForDate (const QDate &date) const =0 |
void | registerObserver (CalendarObserver *observer) |
Incidence::List | relations (const QString &uid) const |
virtual bool | reload () |
virtual void | removeRelations (const Incidence::Ptr &incidence) |
virtual bool | save () |
bool | setDefaultNotebook (const QString ¬ebook) |
void | setFilter (CalFilter *filter) |
void | setModified (bool modified) |
virtual bool | setNotebook (const Incidence::Ptr &incidence, const QString ¬ebook) |
void | setOwner (const Person::Ptr &owner) |
void | setProductId (const QString &id) |
void | setTimeSpec (const KDateTime::Spec &timeSpec) |
void | setTimeZoneId (const QString &timeZoneId) |
void | setTimeZones (ICalTimeZones *zones) |
virtual void | setupRelations (const Incidence::Ptr &incidence) |
void | setViewTimeSpec (const KDateTime::Spec &timeSpec) const |
void | setViewTimeZoneId (const QString &timeZoneId) const |
void | shiftTimes (const KDateTime::Spec &oldSpec, const KDateTime::Spec &newSpec) |
virtual void | startBatchAdding () |
KDateTime::Spec | timeSpec () const |
QString | timeZoneId () const |
ICalTimeZones * | timeZones () const |
virtual Todo::Ptr | todo (const QString &uid, const KDateTime &recurrenceId=KDateTime()) const =0 |
virtual Todo::List | todoInstances (const Incidence::Ptr &todo, TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending) const =0 |
virtual Todo::List | todos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending) const |
virtual Todo::List | todos (const QDate &date) const |
virtual Todo::List | todos (const QDate &start, const QDate &end, const KDateTime::Spec ×pec=KDateTime::Spec(), bool inclusive=false) const |
void | unregisterObserver (CalendarObserver *observer) |
bool | updateNotebook (const QString ¬ebook, bool isVisible) |
KDateTime::Spec | viewTimeSpec () const |
QString | viewTimeZoneId () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &other) | |
virtual | ~CustomProperties () |
QMap< QByteArray, QString > | customProperties () const |
QString | customProperty (const QByteArray &app, const QByteArray &key) const |
QString | nonKDECustomProperty (const QByteArray &name) const |
QString | nonKDECustomPropertyParameters (const QByteArray &name) const |
CustomProperties & | operator= (const CustomProperties &other) |
bool | operator== (const CustomProperties &properties) const |
void | removeCustomProperty (const QByteArray &app, const QByteArray &key) |
void | removeNonKDECustomProperty (const QByteArray &name) |
void | setCustomProperties (const QMap< QByteArray, QString > &properties) |
void | setCustomProperty (const QByteArray &app, const QByteArray &key, const QString &value) |
void | setNonKDECustomProperty (const QByteArray &name, const QString &value, const QString ¶meters=QString()) |
![]() | |
virtual | ~IncidenceObserver () |
virtual void | incidenceUpdate (const QString &uid, const KDateTime &recurrenceId)=0 |
Static Public Member Functions | |
static Incidence::Ptr | createException (const Incidence::Ptr &incidence, const KDateTime &recurrenceId, bool thisAndFuture=false) |
static Incidence::List | mergeIncidenceList (const Event::List &events, const Todo::List &todos, const Journal::List &journals) |
static Event::List | sortEvents (const Event::List &eventList, EventSortField sortField, SortDirection sortDirection) |
static Journal::List | sortJournals (const Journal::List &journalList, JournalSortField sortField, SortDirection sortDirection) |
static Todo::List | sortTodos (const Todo::List &todoList, TodoSortField sortField, SortDirection sortDirection) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
Protected Member Functions | |
void | appendAlarms (Alarm::List &alarms, const Incidence::Ptr &incidence, const KDateTime &from, const KDateTime &to) const |
void | appendRecurringAlarms (Alarm::List &alarms, const Incidence::Ptr &incidence, const KDateTime &from, const KDateTime &to) const |
virtual void | customPropertyUpdated () |
bool | deletionTracking () const |
virtual void | doSetTimeSpec (const KDateTime::Spec &timeSpec) |
void | incidenceUpdated (const QString &uid, const KDateTime &recurrenceId) |
void | notifyIncidenceAdded (const Incidence::Ptr &incidence) |
void | notifyIncidenceAdditionCanceled (const Incidence::Ptr &incidence) |
void | notifyIncidenceChanged (const Incidence::Ptr &incidence) |
void | notifyIncidenceDeleted (const Incidence::Ptr &incidence) |
void | setDeletionTracking (bool enable) |
void | setObserversEnabled (bool enabled) |
virtual void | virtual_hook (int id, void *data) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
virtual void | customPropertyUpdate () |
Additional Inherited Members | |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Represents the main calendar class.
A calendar contains information like incidences (events, to-dos, journals), alarms, time zones, and other useful information.
This is an abstract base class defining the interface to a calendar. It is implemented by subclasses like MemoryCalendar, which use different methods to store and access the data.
Ownership of Incidences:
Incidence ownership is handled by the following policy: as soon as an incidence (or any other subclass of IncidenceBase) is added to the Calendar by an add...() method it is owned by the Calendar object. The Calendar takes care of deleting the incidence using the delete...() methods. All Incidences returned by the query functions are returned as pointers so that changes to the returned Incidences are immediately visible in the Calendar. Do Not attempt to 'delete' any Incidence object you get from Calendar – use the delete...() methods.
Definition at line 128 of file calendar.h.
Member Typedef Documentation
A shared pointer to a Calendar.
Definition at line 138 of file calendar.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a calendar with a specified time zone timeZoneid
.
The time specification is used as the default for creating or modifying incidences in the Calendar. The time specification does not alter existing incidences.
The constructor also calls setViewTimeSpec(timeSpec
).
- Parameters
-
timeSpec time specification
|
explicit |
Construct Calendar object using a time zone ID.
The time zone ID is used as the default for creating or modifying incidences in the Calendar. The time zone does not alter existing incidences.
The constructor also calls setViewTimeZoneId(timeZoneId
).
- Parameters
-
timeZoneId is a string containing a time zone ID, which is assumed to be valid. If no time zone is found, the viewing time specification is set to local clock time. Example: "Europe/Berlin"
|
virtual |
Destroys the calendar.
Member Function Documentation
|
pure virtual |
Inserts an Event into the calendar.
- Parameters
-
event is a pointer to the Event to insert.
- Returns
- true if the Event was successfully inserted; false otherwise.
- See also
- deleteEvent()
Implemented in KCalCore::MemoryCalendar.
|
virtual |
Inserts an Incidence into the calendar.
- Parameters
-
incidence is a pointer to the Incidence to insert.
- Returns
- true if the Incidence was successfully inserted; false otherwise.
- See also
- deleteIncidence()
Reimplemented in KCalCore::MemoryCalendar.
Definition at line 675 of file calendar.cpp.
|
pure virtual |
Inserts a Journal into the calendar.
- Parameters
-
journal is a pointer to the Journal to insert.
- Returns
- true if the Journal was successfully inserted; false otherwise.
- See also
- deleteJournal()
Implemented in KCalCore::MemoryCalendar.
bool KCalCore::Calendar::addNotebook | ( | const QString & | notebook, |
bool | isVisible | ||
) |
Add notebook information into calendar.
Is usually called by storages only.
- Parameters
-
notebook notebook uid isVisible notebook visibility
- Returns
- true if operation succeeded
- See also
- isVisible()
|
pure virtual |
Inserts a Todo into the calendar.
- Parameters
-
todo is a pointer to the Todo to insert.
- Returns
- true if the Todo was successfully inserted; false otherwise.
- See also
- deleteTodo()
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns a list of Alarms within a time range for this Calendar.
- Parameters
-
from is the starting timestamp. to is the ending timestamp.
- Returns
- the list of Alarms for the for the specified time range.
Implemented in KCalCore::MemoryCalendar.
|
protected |
Appends alarms of incidence in interval to list of alarms.
- Parameters
-
alarms is a List of Alarms to be appended onto. incidence is a pointer to an Incidence containing the Alarm to be appended. from is the lower range of the next Alarm repitition. to is the upper range of the next Alarm repitition.
Definition at line 1387 of file calendar.cpp.
|
protected |
Appends alarms of recurring events in interval to list of alarms.
- Parameters
-
alarms is a List of Alarms to be appended onto. incidence is a pointer to an Incidence containing the Alarm to be appended. from is the lower range of the next Alarm repitition. to is the upper range of the next Alarm repitition.
Definition at line 1404 of file calendar.cpp.
bool Calendar::batchAdding | ( | ) | const |
- Returns
- true if batch adding is in progress
Definition at line 1528 of file calendar.cpp.
|
virtual |
Flag that a change to a Calendar Incidence is starting.
- Parameters
-
incidence is a pointer to the Incidence that will be changing.
Definition at line 1370 of file calendar.cpp.
QStringList KCalCore::Calendar::categories | ( | ) | const |
Returns a list of all categories used by Incidences in this Calendar.
- Returns
- a QStringList containing all the categories.
|
virtual |
Clears notebook associations from hash-tables for incidences.
Called when in-memory content of the calendar is cleared.
|
pure virtual |
Clears out the current calendar, freeing all used memory etc.
Implemented in KCalCore::MemoryCalendar.
|
static |
Creates an exception for an occurrence from a recurring Incidence.
The returned exception is not automatically inserted into the calendar.
- Parameters
-
incidence is a pointer to a recurring Incidence. recurrenceId specifies the specific occurrence for which the exception applies. thisAndFuture specifies if the exception applies only this specific occcurrence or also to all future occurrences.
- Returns
- a pointer to a new exception incidence with
- Parameters
-
recurrenceId set.
- Since
- 4.11
Definition at line 701 of file calendar.cpp.
|
protectedvirtual |
CustomProperties::customPropertyUpdated()
Reimplemented from KCalCore::CustomProperties.
Definition at line 1332 of file calendar.cpp.
QString KCalCore::Calendar::defaultNotebook | ( | ) | const |
Get uid of default notebook.
- Returns
- notebook uid
|
pure virtual |
Removes all Events from the calendar.
- See also
- deleteEvent() TODO_KDE5: Remove these methods. They are dangerous and don't add value.
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Removes all Journals from the calendar.
- See also
- deleteJournal()
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Incidence::Ptr Calendar::deleted | ( | const QString & | uid, |
const KDateTime & | recurrenceId = KDateTime() |
||
) | const |
Returns the deleted Incidence associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string. recurrenceId is possible recurrenceid of incidence, default is null
Definition at line 830 of file calendar.cpp.
|
pure virtual |
Returns the deleted Event associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string. recurrenceId is possible recurrenceId of event, default is null
- Returns
- a pointer to the deleted Event. A null pointer is returned if no such deleted Event exists, or if deletion tracking is disabled.
- See also
- deletionTracking()
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns a sorted, unfiltered list of all deleted Events for this Calendar.
- Parameters
-
sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered deleted Events sorted as specified. An empty list is returned if deletion tracking is disabled.
- See also
- deletionTracking()
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns the deleted Journal associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string. recurrenceId is possible recurrenceId of journal, default is null
- Returns
- a pointer to the deleted Journal. A null pointer is returned if no such deleted Journal exists or if deletion tracking is disabled.
- See also
- deletionTracking()
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns a sorted, unfiltered list of all deleted Journals for this Calendar.
- Parameters
-
sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered deleted Journals sorted as specified. An empty list is returned if deletion tracking is disabled.
- See also
- deletionTracking()
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns the deleted Todo associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string. recurrenceId is possible recurrenceId of todo, default is null
- Returns
- a pointer to the deleted Todo. A null pointer is returned if no such deleted Todo exists or if deletion tracking is disabled.
- See also
- deletionTracking()
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns a sorted, unfiltered list of all deleted Todos for this Calendar.
- Parameters
-
sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered deleted Todos sorted as specified. An empty list is returned if deletion tracking is disabled.
- See also
- deletionTracking()
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Removes an Event from the calendar.
- Parameters
-
event is a pointer to the Event to remove.
- Returns
- true if the Event was successfully remove; false otherwise.
- See also
- addEvent(), deleteAllEvents()
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Delete all events that are instances of recurring event event
.
- Parameters
-
event is a pointer to a deleted Event
- Returns
- true if delete was successful; false otherwise
Implemented in KCalCore::MemoryCalendar.
|
virtual |
Removes an Incidence from the calendar.
- Parameters
-
incidence is a pointer to the Incidence to remove.
- Returns
- true if the Incidence was successfully removed; false otherwise.
- See also
- addIncidence()
Reimplemented in KCalCore::MemoryCalendar.
Definition at line 685 of file calendar.cpp.
|
pure virtual |
Delete all incidences that are instances of recurring incidence incidence
.
- Parameters
-
incidence is a pointer to a deleted Incidence
- Returns
- true if delete was successful; false otherwise
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Removes a Journal from the calendar.
- Parameters
-
journal is a pointer to the Journal to remove.
- Returns
- true if the Journal was successfully removed; false otherwise.
- See also
- addJournal(), deleteAllJournals()
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Delete all journals that are instances of recurring journal journal
.
- Parameters
-
journal is a pointer to a deleted Journal
- Returns
- true if delete was successful; false otherwise
Implemented in KCalCore::MemoryCalendar.
bool KCalCore::Calendar::deleteNotebook | ( | const QString & | notebook | ) |
Delete notebook information from calendar.
Is usually called by storages only.
- Parameters
-
notebook notebook uid
- Returns
- true if operation succeeded
- See also
- isVisible()
|
pure virtual |
Removes a Todo from the calendar.
- Parameters
-
todo is a pointer to the Todo to remove.
- Returns
- true if the Todo was successfully removed; false otherwise.
- See also
- addTodo(), deleteAllTodos()
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Delete all to-dos that are instances of recurring to-do todo
.
- Parameters
-
todo is a pointer to a deleted Todo
- Returns
- true if delete was successful; false otherwise
Implemented in KCalCore::MemoryCalendar.
|
protected |
Returns if deletion tracking is enabled.
Default is true.
- Since
- 4.11
Definition at line 1538 of file calendar.cpp.
Incidence::Ptr Calendar::dissociateOccurrence | ( | const Incidence::Ptr & | incidence, |
const QDate & | date, | ||
const KDateTime::Spec & | spec, | ||
bool | single = true |
||
) |
Dissociate an Incidence from a recurring Incidence.
By default, only one single Incidence for the specified date will be dissociated and returned. If single is false, then the recurrence will be split at date, the old Incidence will have its recurrence ending at date and the new Incidence will have all recurrences past the date.
- Parameters
-
incidence is a pointer to a recurring Incidence. date is the QDate within the recurring Incidence on which the dissociation will be performed. spec is the spec in which the date is formulated. single is a flag meaning that a new Incidence should be created from the recurring Incidences after date.
- Returns
- a pointer to a new recurring Incidence if single is false.
Definition at line 739 of file calendar.cpp.
|
protectedvirtual |
Let Calendar subclasses set the time specification.
- Parameters
-
timeSpec is the time specification (time zone, etc.) for viewing Incidence dates.
Definition at line 1267 of file calendar.cpp.
|
virtual |
List all possible duplicate incidences.
- Parameters
-
incidence is the incidence to check.
- Returns
- a list of duplicate incidences.
|
virtual |
Tells the Calendar that you stoped adding a batch of incidences.
- See also
- startBatchAdding()
Definition at line 1523 of file calendar.cpp.
|
virtual |
Flag that a change to a Calendar Incidence has completed.
- Parameters
-
incidence is a pointer to the Incidence that was changed.
Definition at line 1376 of file calendar.cpp.
|
pure virtual |
Returns the Event associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string. recurrenceId is possible recurrenceId of event, default is null
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns a sorted, unfiltered list of all possible instances for this recurring Event.
- Parameters
-
event event to check for. Caller guarantees it's of type Event. sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered event instances sorted as specified.
Implemented in KCalCore::MemoryCalendar.
|
virtual |
Returns a sorted, filtered list of all Events for this Calendar.
- Parameters
-
sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all filtered Events sorted as specified.
Definition at line 667 of file calendar.cpp.
Event::List Calendar::events | ( | const KDateTime & | dt | ) | const |
Returns a filtered list of all Events which occur on the given timestamp.
- Parameters
-
dt request filtered Event list for this KDateTime only.
- Returns
- the list of filtered Events occurring on the specified timestamp.
Definition at line 651 of file calendar.cpp.
Event::List Calendar::events | ( | const QDate & | start, |
const QDate & | end, | ||
const KDateTime::Spec & | timeSpec = KDateTime::Spec() , |
||
bool | inclusive = false |
||
) | const |
Returns a filtered list of all Events occurring within a date range.
- Parameters
-
start is the starting date. end is the ending date. timeSpec time zone etc. to interpret start
andend
, or the calendar's default time spec if none is specifiedinclusive if true only Events which are completely included within the date range are returned.
- Returns
- the list of filtered Events occurring within the specified date range.
Definition at line 658 of file calendar.cpp.
Event::List Calendar::events | ( | const QDate & | date, |
const KDateTime::Spec & | timeSpec = KDateTime::Spec() , |
||
EventSortField | sortField = EventSortUnsorted , |
||
SortDirection | sortDirection = SortDirectionAscending |
||
) | const |
Returns a sorted, filtered list of all Events which occur on the given date.
The Events are sorted according to sortField and sortDirection.
- Parameters
-
date request filtered Event list for this QDate only. timeSpec time zone etc. to interpret start
andend
, or the calendar's default time spec if none is specifiedsortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of sorted, filtered Events occurring on date.
Definition at line 641 of file calendar.cpp.
CalFilter* KCalCore::Calendar::filter | ( | ) | const |
Returns the calendar filter.
- See also
- setFilter()
|
signal |
Emitted when setFilter() is called.
- Since
- 4.11
bool KCalCore::Calendar::hasValidNotebook | ( | const QString & | notebook | ) | const |
Check if calendar knows about the given notebook.
This means that it will be saved by one of the attached storages.
- Parameters
-
notebook notebook uid
- Returns
- true if calendar has valid notebook
Incidence::Ptr Calendar::incidence | ( | const QString & | uid, |
const KDateTime & | recurrenceId = KDateTime() |
||
) | const |
Returns the Incidence associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string. recurrenceId is possible recurrenceid of incidence, default is null
Definition at line 813 of file calendar.cpp.
|
virtual |
Returns the Incidence associated with the given scheduling identifier.
- Parameters
-
sid is a unique scheduling identifier string.
Definition at line 859 of file calendar.cpp.
|
virtual |
Returns a filtered list of all Incidences for this Calendar.
- Returns
- the list of all filtered Incidences.
|
virtual |
|
virtual |
List all notebook incidences in the memory.
- Parameters
-
notebook is the notebook uid.
- Returns
- a list of incidences for the notebook.
|
virtual |
Searches all events and todos for an incidence with this scheduling identifier.
Returns a list of matching results.
- Parameters
-
sid is a unique scheduling identifier string.
Definition at line 846 of file calendar.cpp.
|
protectedvirtual |
The Observer interface.
So far not implemented.
- Parameters
-
uid is the UID for the Incidence that has been updated. recurrenceId is possible recurrenceid of incidence.
Implements KCalCore::IncidenceBase::IncidenceObserver.
Reimplemented in KCalCore::MemoryCalendar.
Definition at line 1248 of file calendar.cpp.
|
virtual |
Returns an unfiltered list of all exceptions of this recurring incidence.
- Parameters
-
incidence incidence to check
- Returns
- the list of all unfiltered exceptions.
bool Calendar::isAncestorOf | ( | const Incidence::Ptr & | ancestor, |
const Incidence::Ptr & | incidence | ||
) | const |
Checks if ancestor
is an ancestor of incidence
.
- Parameters
-
ancestor is the incidence we are testing to be an ancestor. incidence is the incidence we are testing to be descended from ancestor
.
Definition at line 1147 of file calendar.cpp.
bool Calendar::isModified | ( | ) | const |
Determine the calendar's modification status.
- Returns
- true if the calendar has been modified since open or last save.
- See also
- setModified()
Definition at line 1233 of file calendar.cpp.
|
virtual |
Determine if the calendar is currently being saved.
- Returns
- true if the calendar is currently being saved; false otherwise.
Definition at line 1217 of file calendar.cpp.
bool KCalCore::Calendar::isVisible | ( | const Incidence::Ptr & | incidence | ) | const |
Check if incidence is visible.
- Parameters
-
incidence is a pointer to the Incidence to check for visibility.
- Returns
- true if incidence is visible, false otherwise
|
pure virtual |
Returns the Journal associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string. recurrenceId is possible recurrenceId of journal, default is null
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns a sorted, unfiltered list of all instances for this recurring Journal.
- Parameters
-
journal journal to check for. Caller guarantees it's of type Journal. sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered journal instances sorted as specified.
Implemented in KCalCore::MemoryCalendar.
|
virtual |
Returns a sorted, filtered list of all Journals for this Calendar.
- Parameters
-
sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all filtered Journals sorted as specified.
Definition at line 1004 of file calendar.cpp.
|
virtual |
Returns a filtered list of all Journals for on the specified date.
- Parameters
-
date request filtered Journals for this QDate only.
- Returns
- the list of filtered Journals for the specified date.
Definition at line 1012 of file calendar.cpp.
|
static |
Create a merged list of Events, Todos, and Journals.
static
- Parameters
-
events is an Event list to merge. todos is a Todo list to merge. journals is a Journal list to merge.
- Returns
- a list of merged Incidences.
Definition at line 1348 of file calendar.cpp.
|
virtual |
Get incidence's notebook.
- Parameters
-
incidence incidence
- Returns
- notebook uid
Get incidence's notebook.
- Parameters
-
uid is a unique identifier string
- Returns
- notebook uid
|
virtual |
List all uids of notebooks currently in the memory.
- Returns
- list of uids of notebooks
|
protected |
Let Calendar subclasses notify that they inserted an Incidence.
- Parameters
-
incidence is a pointer to the Incidence object that was inserted.
Definition at line 1272 of file calendar.cpp.
|
protected |
Let Calendar subclasses notify that they canceled addition of an Incidence.
- Parameters
-
incidence is a pointer to the Incidence object that addition as canceled.
Definition at line 1317 of file calendar.cpp.
|
protected |
Let Calendar subclasses notify that they modified an Incidence.
- Parameters
-
incidence is a pointer to the Incidence object that was modified.
Definition at line 1287 of file calendar.cpp.
|
protected |
Let Calendar subclasses notify that they removed an Incidence.
- Parameters
-
incidence is a pointer to the Incidence object that was removed.
Definition at line 1302 of file calendar.cpp.
Person::Ptr KCalCore::Calendar::owner | ( | ) | const |
QString Calendar::productId | ( | ) | const |
Returns the calendar's Product ID.
- See also
- setProductId()
Definition at line 1342 of file calendar.cpp.
|
pure virtual |
Returns a sorted, unfiltered list of all Events for this Calendar.
- Parameters
-
sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered Events sorted as specified.
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns an unfiltered list of all Events occurring within a date range.
- Parameters
-
start is the starting date end is the ending date timeSpec time zone etc. to interpret start
andend
, or the calendar's default time spec if none is specifiedinclusive if true only Events which are completely included within the date range are returned.
- Returns
- the list of unfiltered Events occurring within the specified date range.
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns an unfiltered list of all Events which occur on the given timestamp.
- Parameters
-
dt request unfiltered Event list for this KDateTime only.
- Returns
- the list of unfiltered Events occurring on the specified timestamp.
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns a sorted, unfiltered list of all Events which occur on the given date.
The Events are sorted according to sortField and sortDirection.
- Parameters
-
date request unfiltered Event list for this QDate only timeSpec time zone etc. to interpret date
, or the calendar's default time spec if none is specifiedsortField specifies the EventSortField sortDirection specifies the SortDirection
- Returns
- the list of sorted, unfiltered Events occurring on
date
Implemented in KCalCore::MemoryCalendar.
|
virtual |
Returns an unfiltered list of all Incidences for this Calendar.
- Returns
- the list of all unfiltered Incidences.
|
pure virtual |
Returns a sorted, unfiltered list of all Journals for this Calendar.
- Parameters
-
sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered Journals sorted as specified.
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns an unfiltered list of all Journals for on the specified date.
- Parameters
-
date request unfiltered Journals for this QDate only.
- Returns
- the list of unfiltered Journals for the specified date.
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns a sorted, unfiltered list of all Todos for this Calendar.
- Parameters
-
sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered Todos sorted as specified.
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns an unfiltered list of all Todos occurring within a date range.
- Parameters
-
start is the starting date end is the ending date timespec time zone etc. to interpret start
andend
, or the calendar's default time spec if none is specifiedinclusive if true only Todos which are completely included within the date range are returned.
- Returns
- the list of unfiltered Todos occurring within the specified date range.
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns an unfiltered list of all Todos which due on the specified date.
- Parameters
-
date request unfiltered Todos due on this QDate.
- Returns
- the list of unfiltered Todos due on the specified date.
Implemented in KCalCore::MemoryCalendar.
void Calendar::registerObserver | ( | CalendarObserver * | observer | ) |
Registers an Observer for this Calendar.
- Parameters
-
observer is a pointer to an Observer object that will be watching this Calendar.
- See also
- unregisterObserver()
Definition at line 1195 of file calendar.cpp.
Incidence::List Calendar::relations | ( | const QString & | uid | ) | const |
Returns a list of incidences that have a relation of RELTYPE parent to incidence uid
.
- Parameters
-
uid The parent identifier whos children we want to obtain.
Definition at line 1159 of file calendar.cpp.
|
virtual |
Loads the calendar contents from storage.
This requires that the calendar has been previously loaded (initialized).
- Returns
- true if the reload was successful; otherwise false. Base implementation returns true.
Definition at line 1243 of file calendar.cpp.
|
virtual |
Removes all Relations from an Incidence.
- Parameters
-
incidence is a pointer to the Incidence to have a Relation removed.
Definition at line 1064 of file calendar.cpp.
|
virtual |
Syncs changes in memory to persistent storage.
- Returns
- true if the save was successful; false otherwise. Base implementation returns true.
Definition at line 1238 of file calendar.cpp.
bool KCalCore::Calendar::setDefaultNotebook | ( | const QString & | notebook | ) |
set DefaultNotebook information to calendar.
- Parameters
-
notebook notebook uid
- Returns
- true if operation was successful; false otherwise.
|
protected |
Enables or disabled deletion tracking.
Default is true.
- Since
- 4.11
Definition at line 1533 of file calendar.cpp.
void KCalCore::Calendar::setFilter | ( | CalFilter * | filter | ) |
void Calendar::setModified | ( | bool | modified | ) |
Sets if the calendar has been modified.
- Parameters
-
modified is true if the calendar has been modified since open or last save.
- See also
- isModified()
Definition at line 1222 of file calendar.cpp.
|
virtual |
Associate notebook for an incidence.
- Parameters
-
incidence incidence notebook notebook uid
- Returns
- true if the operation was successful; false otherwise.
|
protected |
Let Calendar subclasses notify that they enabled an Observer.
- Parameters
-
enabled if true tells the calendar that a subclass has enabled an Observer.
Definition at line 1382 of file calendar.cpp.
void KCalCore::Calendar::setOwner | ( | const Person::Ptr & | owner | ) |
void Calendar::setProductId | ( | const QString & | id | ) |
Sets the calendar Product ID to id
.
- Parameters
-
id is a string containing the Product ID.
- See also
- productId() const
Definition at line 1337 of file calendar.cpp.
void KCalCore::Calendar::setTimeSpec | ( | const KDateTime::Spec & | timeSpec | ) |
Sets the default time specification (time zone, etc.) used for creating or modifying incidences in the Calendar.
The method also calls setViewTimeSpec(timeSpec
).
- Parameters
-
timeSpec time specification
void KCalCore::Calendar::setTimeZoneId | ( | const QString & | timeZoneId | ) |
Sets the time zone ID used for creating or modifying incidences in the Calendar.
This method has no effect on existing incidences.
The method also calls setViewTimeZoneId(timeZoneId
).
- Parameters
-
timeZoneId is a string containing a time zone ID, which is assumed to be valid. The time zone ID is used to set the time zone for viewing Incidence date/times. If no time zone is found, the viewing time specification is set to local clock time. Example: "Europe/Berlin"
- See also
- setTimeSpec()
void KCalCore::Calendar::setTimeZones | ( | ICalTimeZones * | zones | ) |
Set the time zone collection used by the calendar.
- Parameters
-
zones time zones collection. Important: all time zones references in the calendar must be included in the collection.
|
virtual |
Setup Relations for an Incidence.
- Parameters
-
incidence is a pointer to the Incidence to have a Relation setup.
Definition at line 1021 of file calendar.cpp.
void KCalCore::Calendar::setViewTimeSpec | ( | const KDateTime::Spec & | timeSpec | ) | const |
Notes the time specification which the client application intends to use for viewing the incidences in this calendar.
This is simply a convenience method which makes a note of the new time zone so that it can be read back by viewTimeSpec(). The client application must convert date/time values to the desired time zone itself.
The time specification is not used in any way by the Calendar or its incidences; it is solely for use by the client application.
- Parameters
-
timeSpec time specification
- See also
- viewTimeSpec()
void KCalCore::Calendar::setViewTimeZoneId | ( | const QString & | timeZoneId | ) | const |
Notes the time zone Id which the client application intends to use for viewing the incidences in this calendar.
This is simply a convenience method which makes a note of the new time zone so that it can be read back by viewTimeId(). The client application must convert date/time values to the desired time zone itself.
The Id is not used in any way by the Calendar or its incidences. It is solely for use by the client application.
- Parameters
-
timeZoneId is a string containing a time zone ID, which is assumed to be valid. The time zone ID is used to set the time zone for viewing Incidence date/times. If no time zone is found, the viewing time specification is set to local clock time. Example: "Europe/Berlin"
- See also
- viewTimeZoneId()
void KCalCore::Calendar::shiftTimes | ( | const KDateTime::Spec & | oldSpec, |
const KDateTime::Spec & | newSpec | ||
) |
Shifts the times of all incidences so that they appear at the same clock time as before but in a new time zone.
The shift is done from a viewing time zone rather than from the actual incidence time zone.
For example, shifting an incidence whose start time is 09:00 America/New York, using an old viewing time zone (oldSpec
) of Europe/London, to a new time zone (newSpec
) of Europe/Paris, will result in the time being shifted from 14:00 (which is the London time of the incidence start) to 14:00 Paris time.
- Parameters
-
oldSpec the time specification which provides the clock times newSpec the new time specification
- See also
- isLocalTime()
|
static |
Sort a list of Events.
Private class that helps to provide binary compatibility between releases.
- Parameters
-
eventList is a pointer to a list of Events. sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- a list of Events sorted as specified.
Calendar::Calendar(const KDateTime::Spec &timeSpec) : d(new KCalCore::Calendar::Private) { d->mTimeSpec = timeSpec; d->mViewTimeSpec = timeSpec; }
Calendar::Calendar(const QString &timeZoneId) : d(new KCalCore::Calendar::Private) { setTimeZoneId(timeZoneId); }
Calendar::~Calendar() { delete d; }
Person::Ptr Calendar::owner() const { return d->mOwner; }
void Calendar::setOwner(const Person::Ptr &owner) { Q_ASSERT(owner); d->mOwner = owner; setModified(true); }
void Calendar::setTimeSpec(const KDateTime::Spec &timeSpec) { d->mTimeSpec = timeSpec; d->mBuiltInTimeZone = ICalTimeZone(); setViewTimeSpec(timeSpec);
doSetTimeSpec(d->mTimeSpec); }
KDateTime::Spec Calendar::timeSpec() const { return d->mTimeSpec; }
void Calendar::setTimeZoneId(const QString &timeZoneId) { d->mTimeSpec = d->timeZoneIdSpec(timeZoneId, false); d->mViewTimeSpec = d->mTimeSpec; d->mBuiltInViewTimeZone = d->mBuiltInTimeZone;
doSetTimeSpec(d->mTimeSpec); }
QString Calendar::timeZoneId() const { KTimeZone tz = d->mTimeSpec.timeZone(); return tz.isValid() ? tz.name() : QString(); }
void Calendar::setViewTimeSpec(const KDateTime::Spec &timeSpec) const { d->mViewTimeSpec = timeSpec; d->mBuiltInViewTimeZone = ICalTimeZone(); }
void Calendar::setViewTimeZoneId(const QString &timeZoneId) const { d->mViewTimeSpec = d->timeZoneIdSpec(timeZoneId, true); }
KDateTime::Spec Calendar::viewTimeSpec() const { return d->mViewTimeSpec; }
QString Calendar::viewTimeZoneId() const { KTimeZone tz = d->mViewTimeSpec.timeZone(); return tz.isValid() ? tz.name() : QString(); }
ICalTimeZones *Calendar::timeZones() const { return d->mTimeZones; }
void Calendar::setTimeZones(ICalTimeZones *zones) { if (!zones) { return; }
if (d->mTimeZones && (d->mTimeZones != zones)) { delete d->mTimeZones; d->mTimeZones = 0; } d->mTimeZones = zones; }
void Calendar::shiftTimes(const KDateTime::Spec &oldSpec, const KDateTime::Spec &newSpec) { setTimeSpec(newSpec);
int i, end; Event::List ev = events(); for (i = 0, end = ev.count(); i < end; ++i) { ev[i]->shiftTimes(oldSpec, newSpec); }
Todo::List to = todos(); for (i = 0, end = to.count(); i < end; ++i) { to[i]->shiftTimes(oldSpec, newSpec); }
Journal::List jo = journals(); for (i = 0, end = jo.count(); i < end; ++i) { jo[i]->shiftTimes(oldSpec, newSpec); } }
void Calendar::setFilter(CalFilter *filter) { if (filter) { d->mFilter = filter; } else { d->mFilter = d->mDefaultFilter; } emit filterChanged(); }
CalFilter *Calendar::filter() const { return d->mFilter; }
QStringList Calendar::categories() const { Incidence::List rawInc(rawIncidences()); QStringList cats, thisCats; : For now just iterate over all incidences. In the future, the list of categories should be built when reading the file. for (Incidence::List::ConstIterator i = rawInc.constBegin(); i != rawInc.constEnd(); ++i) { thisCats = (*i)->categories(); for (QStringList::ConstIterator si = thisCats.constBegin(); si != thisCats.constEnd(); ++si) { if (!cats.contains(*si)) { cats.append(*si); } } } return cats; }
Incidence::List Calendar::incidences(const QDate &date) const { return mergeIncidenceList(events(date), todos(date), journals(date)); }
Incidence::List Calendar::incidences() const { return mergeIncidenceList(events(), todos(), journals()); }
Incidence::List Calendar::rawIncidences() const { return mergeIncidenceList(rawEvents(), rawTodos(), rawJournals()); }
Incidence::List Calendar::instances(const Incidence::Ptr &incidence) const { if (incidence) { Event::List elist; Todo::List tlist; Journal::List jlist;
if (incidence->type() == Incidence::TypeEvent) { elist = eventInstances(incidence); } else if (incidence->type() == Incidence::TypeTodo) { tlist = todoInstances(incidence); } else if (incidence->type() == Incidence::TypeJournal) { jlist = journalInstances(incidence); } return mergeIncidenceList(elist, tlist, jlist); } else { return Incidence::List(); } }
Incidence::List Calendar::duplicates(const Incidence::Ptr &incidence) { if (incidence) { Incidence::List list; Incidence::List vals = values(d->mNotebookIncidences); Incidence::List::const_iterator it; for (it = vals.constBegin(); it != vals.constEnd(); ++it) { if (((incidence->dtStart() == (*it)->dtStart()) || (!incidence->dtStart().isValid() && !(*it)->dtStart().isValid())) && (incidence->summary() == (*it)->summary())) { list.append(*it); } } return list; } else { return Incidence::List(); } }
bool Calendar::addNotebook(const QString ¬ebook, bool isVisible) { if (d->mNotebooks.contains(notebook)) { return false; } else { d->mNotebooks.insert(notebook, isVisible); return true; } }
bool Calendar::updateNotebook(const QString ¬ebook, bool isVisible) { if (!d->mNotebooks.contains(notebook)) { return false; } else { d->mNotebooks.insert(notebook, isVisible); return true; } }
bool Calendar::deleteNotebook(const QString ¬ebook) { if (!d->mNotebooks.contains(notebook)) { return false; } else { return d->mNotebooks.remove(notebook); } }
bool Calendar::setDefaultNotebook(const QString ¬ebook) { if (!d->mNotebooks.contains(notebook)) { return false; } else { d->mDefaultNotebook = notebook; return true; } }
QString Calendar::defaultNotebook() const { return d->mDefaultNotebook; }
bool Calendar::hasValidNotebook(const QString ¬ebook) const { return d->mNotebooks.contains(notebook); }
bool Calendar::isVisible(const Incidence::Ptr &incidence) const { if (d->mIncidenceVisibility.contains(incidence)) { return d->mIncidenceVisibility[incidence]; } const QString nuid = notebook(incidence); bool rv; if (d->mNotebooks.contains(nuid)) { rv = d->mNotebooks.value(nuid); } else { NOTE returns true also for nonexisting notebooks for compatibility rv = true; } d->mIncidenceVisibility[incidence] = rv; return rv; }
void Calendar::clearNotebookAssociations() { d->mNotebookIncidences.clear(); d->mUidToNotebook.clear(); d->mIncidenceVisibility.clear(); }
bool Calendar::setNotebook(const Incidence::Ptr &inc, const QString ¬ebook) { if (!inc) { return false; }
if (!notebook.isEmpty() && !incidence(inc->uid(), inc->recurrenceId())) { kWarning() << "cannot set notebook until incidence has been added"; return false; }
if (d->mUidToNotebook.contains(inc->uid())) { QString old = d->mUidToNotebook.value(inc->uid()); if (!old.isEmpty() && notebook != old) { if (inc->hasRecurrenceId()) { kWarning() << "cannot set notebook for child incidences"; return false; } Move all possible children also. Incidence::List list = instances(inc); Incidence::List::Iterator it; for (it = list.begin(); it != list.end(); ++it) { d->mNotebookIncidences.remove(old, *it); d->mNotebookIncidences.insert(notebook, *it); } notifyIncidenceChanged(inc); // for removing from old notebook don not remove from mUidToNotebook to keep deleted incidences d->mNotebookIncidences.remove(old, inc); } } if (!notebook.isEmpty()) { d->mUidToNotebook.insert(inc->uid(), notebook); d->mNotebookIncidences.insert(notebook, inc); kDebug() << "setting notebook" << notebook << "for" << inc->uid(); notifyIncidenceChanged(inc); // for inserting into new notebook }
return true; }
QString Calendar::notebook(const Incidence::Ptr &incidence) const { if (incidence) { return d->mUidToNotebook.value(incidence->uid()); } else { return QString(); } }
QString Calendar::notebook(const QString &uid) const { return d->mUidToNotebook.value(uid); }
QStringList Calendar::notebooks() const { return d->mNotebookIncidences.uniqueKeys(); }
Incidence::List Calendar::incidences(const QString ¬ebook) const { if (notebook.isEmpty()) { return values(d->mNotebookIncidences); } else { return values(d->mNotebookIncidences, notebook); } }
/** static
Definition at line 594 of file calendar.cpp.
|
static |
Sort a list of Journals.
static
- Parameters
-
journalList is a pointer to a list of Journals. sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns
- a list of Journals sorted as specified.
Definition at line 970 of file calendar.cpp.
|
static |
Sort a list of Todos.
static
- Parameters
-
todoList is a pointer to a list of Todos. sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns
- a list of Todos sorted as specified.
Definition at line 874 of file calendar.cpp.
|
virtual |
Call this to tell the calendar that you're adding a batch of incidences.
So it doesn't, for example, ask the destination for each incidence.
- See also
- endBatchAdding()
Definition at line 1518 of file calendar.cpp.
KDateTime::Spec KCalCore::Calendar::timeSpec | ( | ) | const |
Get the time specification (time zone etc.) used for creating or modifying incidences in the Calendar.
- Returns
- time specification
QString KCalCore::Calendar::timeZoneId | ( | ) | const |
Returns the time zone ID used for creating or modifying incidences in the calendar.
- Returns
- the string containing the time zone ID, or empty string if the creation/modification time specification is not a time zone.
ICalTimeZones* KCalCore::Calendar::timeZones | ( | ) | const |
Returns the time zone collection used by the calendar.
- Returns
- the time zones collection.
- See also
- setLocalTime()
|
pure virtual |
Returns the Todo associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string. recurrenceId is possible recurrenceId of todo, default is null
Implemented in KCalCore::MemoryCalendar.
|
pure virtual |
Returns a sorted, unfiltered list of all possible instances for this recurring Todo.
- Parameters
-
todo todo to check for. Caller guarantees it's of type Todo. sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered todo instances sorted as specified.
Implemented in KCalCore::MemoryCalendar.
|
virtual |
Returns a sorted, filtered list of all Todos for this Calendar.
- Parameters
-
sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all filtered Todos sorted as specified.
Definition at line 946 of file calendar.cpp.
|
virtual |
Returns a filtered list of all Todos which are due on the specified date.
- Parameters
-
date request filtered Todos due on this QDate.
- Returns
- the list of filtered Todos due on the specified date.
Definition at line 954 of file calendar.cpp.
|
virtual |
Returns a filtered list of all Todos occurring within a date range.
- Parameters
-
start is the starting date end is the ending date timespec time zone etc. to interpret start
andend
, or the calendar's default time spec if none is specifiedinclusive if true only Todos which are completely included within the date range are returned.
- Returns
- the list of filtered Todos occurring within the specified date range.
Definition at line 961 of file calendar.cpp.
void Calendar::unregisterObserver | ( | CalendarObserver * | observer | ) |
Unregisters an Observer for this Calendar.
- Parameters
-
observer is a pointer to an Observer object that has been watching this Calendar.
- See also
- registerObserver()
Definition at line 1208 of file calendar.cpp.
bool KCalCore::Calendar::updateNotebook | ( | const QString & | notebook, |
bool | isVisible | ||
) |
Update notebook information in calendar.
Is usually called by storages only.
- Parameters
-
notebook notebook uid isVisible notebook visibility
- Returns
- true if operation succeeded
- See also
- isVisible()
KDateTime::Spec KCalCore::Calendar::viewTimeSpec | ( | ) | const |
Returns the time specification used for viewing the incidences in this calendar.
This simply returns the time specification last set by setViewTimeSpec().
- See also
- setViewTimeSpec().
QString KCalCore::Calendar::viewTimeZoneId | ( | ) | const |
Returns the time zone Id used for viewing the incidences in this calendar.
This simply returns the time specification last set by setViewTimeSpec().
- See also
- setViewTimeZoneId().
|
protectedvirtual |
Reimplemented from KCalCore::CustomProperties.
Reimplemented in KCalCore::MemoryCalendar.
Definition at line 1543 of file calendar.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:36:53 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.