KCalCore Library
#include <memorycalendar.h>
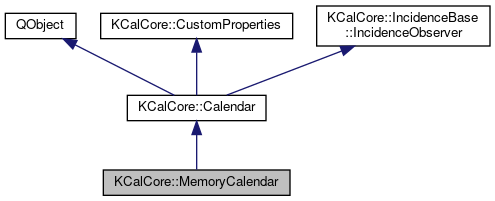
Public Types | |
typedef QSharedPointer < MemoryCalendar > | Ptr |
![]() | |
typedef QSharedPointer< Calendar > | Ptr |
Protected Member Functions | |
virtual void | virtual_hook (int id, void *data) |
![]() | |
void | appendAlarms (Alarm::List &alarms, const Incidence::Ptr &incidence, const KDateTime &from, const KDateTime &to) const |
void | appendRecurringAlarms (Alarm::List &alarms, const Incidence::Ptr &incidence, const KDateTime &from, const KDateTime &to) const |
virtual void | customPropertyUpdated () |
bool | deletionTracking () const |
virtual void | doSetTimeSpec (const KDateTime::Spec &timeSpec) |
void | notifyIncidenceAdded (const Incidence::Ptr &incidence) |
void | notifyIncidenceAdditionCanceled (const Incidence::Ptr &incidence) |
void | notifyIncidenceChanged (const Incidence::Ptr &incidence) |
void | notifyIncidenceDeleted (const Incidence::Ptr &incidence) |
void | setDeletionTracking (bool enable) |
void | setObserversEnabled (bool enabled) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
virtual void | customPropertyUpdate () |
Additional Inherited Members | |
![]() | |
void | filterChanged () |
![]() | |
static Incidence::Ptr | createException (const Incidence::Ptr &incidence, const KDateTime &recurrenceId, bool thisAndFuture=false) |
static Incidence::List | mergeIncidenceList (const Event::List &events, const Todo::List &todos, const Journal::List &journals) |
static Event::List | sortEvents (const Event::List &eventList, EventSortField sortField, SortDirection sortDirection) |
static Journal::List | sortJournals (const Journal::List &journalList, JournalSortField sortField, SortDirection sortDirection) |
static Todo::List | sortTodos (const Todo::List &todoList, TodoSortField sortField, SortDirection sortDirection) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
This class provides a calendar stored in memory.
Definition at line 46 of file memorycalendar.h.
Member Typedef Documentation
A shared pointer to a MemoryCalendar.
Definition at line 53 of file memorycalendar.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a calendar with a specified time zone timeZoneid
.
The time specification is used as the default for creating or modifying incidences in the Calendar. The time specification does not alter existing incidences.
The constructor also calls setViewTimeSpec(timeSpec
).
- Parameters
-
timeSpec time specification
|
explicit |
Construct Calendar object using a time zone ID.
The time zone ID is used as the default for creating or modifying incidences in the Calendar. The time zone does not alter existing incidences.
The constructor also calls setViewTimeZoneId(timeZoneId
).
- Parameters
-
timeZoneId is a string containing a time zone ID, which is assumed to be valid. If no time zone is found, the viewing time specification is set to local clock time. Example: "Europe/Berlin"
KCalCore::MemoryCalendar::~MemoryCalendar | ( | ) |
Destroys the calendar.
Member Function Documentation
|
virtual |
Inserts an Event into the calendar.
- Parameters
-
event is a pointer to the Event to insert.
- Returns
- true if the Event was successfully inserted; false otherwise.
- See also
- deleteEvent()
Implements KCalCore::Calendar.
|
virtual |
Inserts an Incidence into the calendar.
- Parameters
-
incidence is a pointer to the Incidence to insert.
- Returns
- true if the Incidence was successfully inserted; false otherwise.
- See also
- deleteIncidence()
Reimplemented from KCalCore::Calendar.
|
virtual |
Inserts a Journal into the calendar.
- Parameters
-
journal is a pointer to the Journal to insert.
- Returns
- true if the Journal was successfully inserted; false otherwise.
- See also
- deleteJournal()
Implements KCalCore::Calendar.
|
virtual |
Inserts a Todo into the calendar.
- Parameters
-
todo is a pointer to the Todo to insert.
- Returns
- true if the Todo was successfully inserted; false otherwise.
- See also
- deleteTodo()
Implements KCalCore::Calendar.
|
virtual |
Returns a list of Alarms within a time range for this Calendar.
- Parameters
-
from is the starting timestamp. to is the ending timestamp.
- Returns
- the list of Alarms for the for the specified time range.
Implements KCalCore::Calendar.
Alarm::List KCalCore::MemoryCalendar::alarmsTo | ( | const KDateTime & | to | ) | const |
Return a list of Alarms that occur before the specified timestamp.
- Parameters
-
to is the ending timestamp.
- Returns
- the list of Alarms occurring before the specified KDateTime.
|
virtual |
|
virtual |
Removes all Events from the calendar.
- See also
- deleteEvent() TODO_KDE5: Remove these methods. They are dangerous and don't add value.
Implements KCalCore::Calendar.
|
virtual |
|
virtual |
|
virtual |
Returns the deleted Event associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string. recurrenceId is possible recurrenceId of event, default is null
- Returns
- a pointer to the deleted Event. A null pointer is returned if no such deleted Event exists, or if deletion tracking is disabled.
- See also
- deletionTracking()
Implements KCalCore::Calendar.
|
virtual |
Returns a sorted, unfiltered list of all deleted Events for this Calendar.
- Parameters
-
sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered deleted Events sorted as specified. An empty list is returned if deletion tracking is disabled.
- See also
- deletionTracking()
Implements KCalCore::Calendar.
|
virtual |
Returns the deleted Journal associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string. recurrenceId is possible recurrenceId of journal, default is null
- Returns
- a pointer to the deleted Journal. A null pointer is returned if no such deleted Journal exists or if deletion tracking is disabled.
- See also
- deletionTracking()
Implements KCalCore::Calendar.
|
virtual |
Returns a sorted, unfiltered list of all deleted Journals for this Calendar.
- Parameters
-
sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered deleted Journals sorted as specified. An empty list is returned if deletion tracking is disabled.
- See also
- deletionTracking()
Implements KCalCore::Calendar.
|
virtual |
Returns the deleted Todo associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string. recurrenceId is possible recurrenceId of todo, default is null
- Returns
- a pointer to the deleted Todo. A null pointer is returned if no such deleted Todo exists or if deletion tracking is disabled.
- See also
- deletionTracking()
Implements KCalCore::Calendar.
|
virtual |
Returns a sorted, unfiltered list of all deleted Todos for this Calendar.
- Parameters
-
sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered deleted Todos sorted as specified. An empty list is returned if deletion tracking is disabled.
- See also
- deletionTracking()
Implements KCalCore::Calendar.
|
virtual |
Removes an Event from the calendar.
- Parameters
-
event is a pointer to the Event to remove.
- Returns
- true if the Event was successfully remove; false otherwise.
- See also
- addEvent(), deleteAllEvents()
Implements KCalCore::Calendar.
|
virtual |
Delete all events that are instances of recurring event event
.
- Parameters
-
event is a pointer to a deleted Event
- Returns
- true if delete was successful; false otherwise
Implements KCalCore::Calendar.
|
virtual |
Removes an Incidence from the calendar.
- Parameters
-
incidence is a pointer to the Incidence to remove.
- Returns
- true if the Incidence was successfully removed; false otherwise.
- See also
- addIncidence()
Reimplemented from KCalCore::Calendar.
|
virtual |
Delete all incidences that are instances of recurring incidence incidence
.
- Parameters
-
incidence is a pointer to a deleted Incidence
- Returns
- true if delete was successful; false otherwise
Implements KCalCore::Calendar.
|
virtual |
Removes a Journal from the calendar.
- Parameters
-
journal is a pointer to the Journal to remove.
- Returns
- true if the Journal was successfully removed; false otherwise.
- See also
- addJournal(), deleteAllJournals()
Implements KCalCore::Calendar.
|
virtual |
Delete all journals that are instances of recurring journal journal
.
- Parameters
-
journal is a pointer to a deleted Journal
- Returns
- true if delete was successful; false otherwise
Implements KCalCore::Calendar.
|
virtual |
Removes a Todo from the calendar.
- Parameters
-
todo is a pointer to the Todo to remove.
- Returns
- true if the Todo was successfully removed; false otherwise.
- See also
- addTodo(), deleteAllTodos()
Implements KCalCore::Calendar.
|
virtual |
Delete all to-dos that are instances of recurring to-do todo
.
- Parameters
-
todo is a pointer to a deleted Todo
- Returns
- true if delete was successful; false otherwise
Implements KCalCore::Calendar.
|
virtual |
Returns the Event associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string. recurrenceId is possible recurrenceId of event, default is null
Implements KCalCore::Calendar.
|
virtual |
Returns a sorted, unfiltered list of all possible instances for this recurring Event.
- Parameters
-
event event to check for. Caller guarantees it's of type Event. sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered event instances sorted as specified.
Implements KCalCore::Calendar.
|
virtual |
The IncidenceObserver interface.
This function is called before any changes are made.
- Parameters
-
uid is the string containing the incidence uid. recurrenceId is possible recurrenceid of incidence.
Implements KCalCore::IncidenceBase::IncidenceObserver.
|
virtual |
The Observer interface.
So far not implemented.
- Parameters
-
uid is the UID for the Incidence that has been updated. recurrenceId is possible recurrenceid of incidence.
Reimplemented from KCalCore::Calendar.
Incidence::Ptr KCalCore::MemoryCalendar::instance | ( | const QString & | identifier | ) | const |
|
virtual |
Returns the Journal associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string. recurrenceId is possible recurrenceId of journal, default is null
Implements KCalCore::Calendar.
|
virtual |
- Parameters
-
journal journal to check for. Caller guarantees it's of type Journal. sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered journal instances sorted as specified.
Implements KCalCore::Calendar.
|
virtual |
Returns a sorted, unfiltered list of all Events for this Calendar.
- Parameters
-
sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered Events sorted as specified.
Implements KCalCore::Calendar.
|
virtual |
Returns an unfiltered list of all Events occurring within a date range.
- Parameters
-
start is the starting date end is the ending date timeSpec time zone etc. to interpret start
andend
, or the calendar's default time spec if none is specifiedinclusive if true only Events which are completely included within the date range are returned.
- Returns
- the list of unfiltered Events occurring within the specified date range.
Implements KCalCore::Calendar.
|
virtual |
Returns an unfiltered list of all Events which occur on the given date.
- Parameters
-
date request unfiltered Event list for this QDate only. timeSpec time zone etc. to interpret date
, or the calendar's default time spec if none is specifiedsortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of unfiltered Events occurring on the specified QDate.
Implements KCalCore::Calendar.
|
virtual |
Returns an unfiltered list of all Events which occur on the given timestamp.
- Parameters
-
dt request unfiltered Event list for this KDateTime only.
- Returns
- the list of unfiltered Events occurring on the specified timestamp.
Implements KCalCore::Calendar.
|
virtual |
Returns a sorted, unfiltered list of all Journals for this Calendar.
- Parameters
-
sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered Journals sorted as specified.
Implements KCalCore::Calendar.
|
virtual |
Returns an unfiltered list of all Journals for on the specified date.
- Parameters
-
date request unfiltered Journals for this QDate only.
- Returns
- the list of unfiltered Journals for the specified date.
Implements KCalCore::Calendar.
|
virtual |
Returns a sorted, unfiltered list of all Todos for this Calendar.
- Parameters
-
sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered Todos sorted as specified.
Implements KCalCore::Calendar.
|
virtual |
Returns an unfiltered list of all Todos occurring within a date range.
- Parameters
-
start is the starting date end is the ending date timespec time zone etc. to interpret start
andend
, or the calendar's default time spec if none is specifiedinclusive if true only Todos which are completely included within the date range are returned.
- Returns
- the list of unfiltered Todos occurring within the specified date range.
Implements KCalCore::Calendar.
|
virtual |
Returns an unfiltered list of all Todos which due on the specified date.
- Parameters
-
date request unfiltered Todos due on this QDate.
- Returns
- the list of unfiltered Todos due on the specified date.
Implements KCalCore::Calendar.
|
virtual |
Returns the Todo associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string. recurrenceId is possible recurrenceId of todo, default is null
Implements KCalCore::Calendar.
|
virtual |
Returns a sorted, unfiltered list of all possible instances for this recurring Todo.
- Parameters
-
todo todo to check for. Caller guarantees it's of type Todo. sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered todo instances sorted as specified.
Implements KCalCore::Calendar.
|
protectedvirtual |
Standard trick to add virtuals later.
- Parameters
-
id is any integer unique to this class which we will use to identify the method to be called. data is a pointer to some glob of data, typically a struct. TODO_KDE5: change from int to VirtualHook type.
Reimplemented from KCalCore::Calendar.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:36:53 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.