KChatBase
#include <KChatBase>
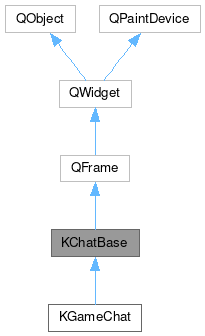
Public Types | |
enum | SendingIds { SendToAll = 0 } |
![]() | |
enum | Shadow |
enum | Shape |
enum | StyleMask |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
Public Slots | |
virtual void | addMessage (const QString &fromName, const QString &text) |
virtual void | addSystemMessage (const QString &fromName, const QString &text) |
void | setAcceptMessage (bool a) |
void | slotClear () |
Public Member Functions | |
KChatBase (QWidget *parent, KChatBaseModel *model=nullptr, KChatBaseItemDelegate *delegate=nullptr, bool noComboBox=false) | |
~KChatBase () override | |
virtual bool | acceptMessage () const |
bool | addSendingEntry (const QString &text, int id) |
void | changeSendingEntry (const QString &text, int id) |
void | clear () |
int | findIndex (int id) const |
virtual QString | fromName () const =0 |
bool | insertSendingEntry (const QString &text, int id, int index=-1) |
int | maxItems () const |
QFont | messageFont () const |
KChatBaseModel * | model () |
QFont | nameFont () const |
int | nextId () const |
virtual void | readConfig (KConfig *conf=nullptr) |
void | removeSendingEntry (int id) |
virtual void | saveConfig (KConfig *conf=nullptr) |
int | sendingEntry () const |
void | setBothFont (const QFont &font) |
void | setCompletionMode (KCompletion::CompletionMode mode) |
void | setMaxItems (int maxItems) |
void | setMessageFont (const QFont &font) |
void | setModel (KChatBaseModel *m) |
void | setNameFont (const QFont &font) |
void | setSendingEntry (int id) |
void | setSystemBothFont (const QFont &font) |
void | setSystemMessageFont (const QFont &font) |
void | setSystemNameFont (const QFont &font) |
QFont | systemMessageFont () const |
QFont | systemNameFont () const |
![]() | |
QFrame (QWidget *parent, Qt::WindowFlags f) | |
QRect | frameRect () const const |
Shadow | frameShadow () const const |
Shape | frameShape () const const |
int | frameStyle () const const |
int | frameWidth () const const |
int | lineWidth () const const |
int | midLineWidth () const const |
void | setFrameRect (const QRect &) |
void | setFrameShadow (Shadow) |
void | setFrameShape (Shape) |
void | setFrameStyle (int style) |
void | setLineWidth (int) |
void | setMidLineWidth (int) |
virtual QSize | sizeHint () const const override |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
virtual QSize | minimumSizeHint () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
Protected Member Functions | |
KChatBase (KChatBasePrivate &dd, QWidget *parent, bool noComboBox) | |
virtual QString | comboBoxItem (const QString &name) const |
QModelIndex | indexAt (QPoint pos) const |
virtual void | returnPressed (const QString &text)=0 |
![]() | |
virtual void | changeEvent (QEvent *ev) override |
virtual bool | event (QEvent *e) override |
virtual void | initStyleOption (QStyleOptionFrame *option) const const |
virtual void | paintEvent (QPaintEvent *) override |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEnterEvent *event) |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The base class for chat widgets.
This is the base class for both KChat and KGameChat. KGameChat is the class you want to use if you write a KGame based game as it will do most things for you. KChat is more or less the same but not KGame dependent
KChatBase provides a complete chat widget, featuring different sending means (e.g. "send to all", "send to player1", "send to group2" and so on - see addSendingEntry). It also provides full auto-completion capabilities (see KCompletion and KLineEdit) which defaults to disabled. The user can change this by right-clicking on the KLineEdit widget and selecting the desired behaviour. You can also change this manually by calling setCompletionMode.
To make KhatBase useful you have to overwrite at least returnPressed. Here you should send the message to all of your clients (or just some of them, depending on sendingEntry).
To add a message just call addMessage with the nickname of the player who sent the message and the message itself.
You probably don't want to use the abstract class KChatBase directly but use one of the derived classes KChat or KGameChat. The latter is the widget of choice if you develop a KGame application as you don't have to do anything but providing a KGame object. If you want to change the kind of elements displayed (using pixmaps for example), then you will also have to derive the KChatBaseModel and KChatBaseItemDelegate classes.
Definition at line 63 of file kchatbase.h.
Member Enumeration Documentation
◆ SendingIds
enum KChatBase::SendingIds |
Definition at line 85 of file kchatbase.h.
Constructor & Destructor Documentation
◆ KChatBase() [1/2]
|
explicit |
- Parameters
-
parent The parent widget for this widget. model delegate noComboBox If true then the combo box where the player can choose where to send messages to (either globally or just to some players) will not be added.
Definition at line 56 of file kchatbase.cpp.
◆ ~KChatBase()
|
override |
◆ KChatBase() [2/2]
|
protected |
Definition at line 61 of file kchatbase.cpp.
Member Function Documentation
◆ acceptMessage()
|
virtual |
- Returns
- True if this widget is able to send messages (see returnPressed) and false if not. The default implementation returns the value which has been set by setAcceptMessage (true by default)
Definition at line 125 of file kchatbase.cpp.
◆ addMessage
Add a text in the listbox.
See also signalSendMessage()
Maybe you want to replace this with a function that creates a nicer text than "fromName: text"
Update: the function layoutMessage is called by this now. This means that you will get user defined outlook on the messages :-)
- Parameters
-
fromName The player who sent this message text The text to be added
Definition at line 396 of file kchatbase.cpp.
◆ addSendingEntry()
bool KChatBase::addSendingEntry | ( | const QString & | text, |
int | id ) |
Adds a new entry in the combo box.
The default is "send to all players" only. This function is provided for convenience. You can also call insertSendingEntry with index = -1. See also nextId!
- Parameters
-
text The text of the new entry id An ID for this entry. This must be unique for this entry. It has nothing to do with the position of the entry in the combo box. See nextId
- Returns
- True if successful, otherwise false (e.g. if the id is already used)
Definition at line 139 of file kchatbase.cpp.
◆ addSystemMessage
This works just like addMessage but adds a system message.
layoutSystemMessage is used to generate the displayed item. System messages will have a different look than player messages.
You may wish to use this to display status information from your game.
Definition at line 403 of file kchatbase.cpp.
◆ changeSendingEntry()
void KChatBase::changeSendingEntry | ( | const QString & | text, |
int | id ) |
This changes a combo box entry.
- Parameters
-
text The new text of the entry id The ID of the item to be changed
Definition at line 204 of file kchatbase.cpp.
◆ clear()
void KChatBase::clear | ( | ) |
Clear all messages in the list.
Definition at line 297 of file kchatbase.cpp.
◆ comboBoxItem()
Replace to customize the combo box.
Default: i18n("Send to %1).arg(name)
- Parameters
-
name The name of the player
- Returns
- The string as it will be shown in the combo box
Definition at line 260 of file kchatbase.cpp.
◆ findIndex()
int KChatBase::findIndex | ( | int | id | ) | const |
- Returns
- The index of the combo box entry with the given id
Definition at line 227 of file kchatbase.cpp.
◆ fromName()
|
pure virtual |
- Returns
- The name that will be shown for messages from this widget. Either the string that was set by setFromName or the name of the player that was set by setFromPlayer
Implemented in KGameChat.
◆ indexAt()
|
protected |
Returns the model index of the message at the viewport coordinates point.
- Parameters
-
pos position to check index for
- Returns
- model index of message with coordinates pos
Definition at line 107 of file kchatbase.cpp.
◆ insertSendingEntry()
bool KChatBase::insertSendingEntry | ( | const QString & | text, |
int | id, | ||
int | index = -1 ) |
Inserts a new entry in the combo box.
- Parameters
-
text The entry id An ID for this entry. This must be unique for this entry. It has nothing to do with the position of the entry in the combo box!
- See also
- nextId
- Parameters
-
index The position of the entry. If -1 the entry will be added at the bottom
- Returns
- True if successful, otherwise false (e.g. if the id is already used)
Definition at line 148 of file kchatbase.cpp.
◆ maxItems()
int KChatBase::maxItems | ( | ) | const |
- Returns
- The maximum number of messages in the list. -1 is unlimited. See also setMaxItems
Definition at line 319 of file kchatbase.cpp.
◆ messageFont()
QFont KChatBase::messageFont | ( | ) | const |
This font should be used for a message.
layoutMessage sets the font of a message using KChatBaseItemDelegate::setMessageFont but if you replace layoutMessage with your own function you should use messageFont() yourself.
- Returns
- The font that is used for a message
Definition at line 333 of file kchatbase.cpp.
◆ model()
KChatBaseModel * KChatBase::model | ( | ) |
Definition at line 49 of file kchatbase.cpp.
◆ nameFont()
QFont KChatBase::nameFont | ( | ) | const |
This font should be used for the name (the "from: " part) of a message.
layoutMessage uses this to set the font using KChatBaseItemDelegate::setNameFont but if you want to overwrite layoutMessage you should do this yourself.
- Returns
- The font that is used for the name part of the message.
Definition at line 326 of file kchatbase.cpp.
◆ nextId()
int KChatBase::nextId | ( | ) | const |
- Returns
- An ID that has not yet been used in the combo box.
- See also
- addSendingEntry
Definition at line 234 of file kchatbase.cpp.
◆ readConfig()
|
virtual |
Read the configuration from a KConfig object.
If the pointer is NULL KGlobal::config() is used and the group is changed to "KChatBase". The current KConfig::group is restored after this call.
Definition at line 287 of file kchatbase.cpp.
◆ removeSendingEntry()
void KChatBase::removeSendingEntry | ( | int | id | ) |
Removes the entry with the ID id from the combo box.
Note that id is not the index of the entry!
- See also
- addSendingEntry
- Parameters
-
id The unique id of the entry
Definition at line 189 of file kchatbase.cpp.
◆ returnPressed()
|
protectedpure virtual |
This is called whenever the user pushed return ie wants to send a message.
Note that you MUST add the message to the widget when this function is called as it has already been added to the KCompletion object of the KLineEdit widget!
Must be implemented in derived classes
- Parameters
-
text The message to be sent
Implemented in KGameChat.
◆ saveConfig()
|
virtual |
Save the configuration of the dialog to a KConfig object.
If the supplied KConfig pointer is NULL then KGlobal::config() is used instead (and the group is changed to "KChatBase") butr the current group is restored at the end.
- Parameters
-
conf A pointer to the KConfig object to save the config to. If you use 0 then KGlobal::config() is used and the group is changed to "KChatBase" (the current group is restored at the end).
Definition at line 277 of file kchatbase.cpp.
◆ sendingEntry()
int KChatBase::sendingEntry | ( | ) | const |
- Returns
- The unique ID of the sending entry that has been selected.
- See also
- addSendingEntry
Note that the entry "send to all" always uses KChatBase::SendToAll, i.e. 0 as id!
Definition at line 173 of file kchatbase.cpp.
◆ setAcceptMessage
|
slot |
- Parameters
-
a If false this widget cannot send a message until setAcceptMessage(true) is called
Definition at line 132 of file kchatbase.cpp.
◆ setBothFont()
void KChatBase::setBothFont | ( | const QFont & | font | ) |
This sets both - nameFont and messageFont to font.
You probably want to use this if you don't wish to distinguish between these parts of a message.
- Parameters
-
font A font used for both nameFont and messageFont
Definition at line 368 of file kchatbase.cpp.
◆ setCompletionMode()
void KChatBase::setCompletionMode | ( | KCompletion::CompletionMode | mode | ) |
See KLineEdit::setCompletionMode.
Definition at line 270 of file kchatbase.cpp.
◆ setMaxItems()
void KChatBase::setMaxItems | ( | int | maxItems | ) |
Set the maximum number of items in the list.
If the number of item exceeds the maximum as many items are deleted (oldest first) as necessary. The number of items will never exceed this value.
- Parameters
-
maxItems the maximum number of items. -1 (default) for unlimited.
Definition at line 304 of file kchatbase.cpp.
◆ setMessageFont()
void KChatBase::setMessageFont | ( | const QFont & | font | ) |
Set the font that is used for the message part of a message.
- See also
- messageFont, setBothFont
Definition at line 361 of file kchatbase.cpp.
◆ setModel()
void KChatBase::setModel | ( | KChatBaseModel * | m | ) |
Definition at line 41 of file kchatbase.cpp.
◆ setNameFont()
void KChatBase::setNameFont | ( | const QFont & | font | ) |
Set the font that is used for the name part of a message.
See also nameFont and setBothFont
Definition at line 354 of file kchatbase.cpp.
◆ setSendingEntry()
void KChatBase::setSendingEntry | ( | int | id | ) |
This selects a combo box entry.
- Parameters
-
id The ID of the item to be selected
Definition at line 216 of file kchatbase.cpp.
◆ setSystemBothFont()
void KChatBase::setSystemBothFont | ( | const QFont & | font | ) |
Same as setBothFont but applies only to system messages.
- See also
- layoutSystemMessage
Definition at line 389 of file kchatbase.cpp.
◆ setSystemMessageFont()
void KChatBase::setSystemMessageFont | ( | const QFont & | font | ) |
Same as setMessageFont but applies only to system messages.
- See also
- layoutSystemMessage
Definition at line 382 of file kchatbase.cpp.
◆ setSystemNameFont()
void KChatBase::setSystemNameFont | ( | const QFont & | font | ) |
Same as setNameFont but applies only to system messages.
- See also
- layoutSystemMessage
Definition at line 375 of file kchatbase.cpp.
◆ slotClear
|
slot |
This clears all messages in the view.
Note that only the messages are cleared, not the sender names in the combo box!
Definition at line 265 of file kchatbase.cpp.
◆ systemMessageFont()
QFont KChatBase::systemMessageFont | ( | ) | const |
Same as systemMessageFont but applies only to system messages.
- See also
- layoutSystemMessage
Definition at line 347 of file kchatbase.cpp.
◆ systemNameFont()
QFont KChatBase::systemNameFont | ( | ) | const |
Same as systemNameFont but applies only to system messages.
- See also
- layoutSystemMessage
Definition at line 340 of file kchatbase.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 28 2025 11:53:10 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.