KGame
#include <KGame/KGame>
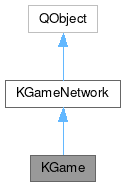
Public Types | |
enum | GamePolicy { PolicyUndefined = 0 , PolicyClean = 1 , PolicyDirty = 2 , PolicyLocal = 3 } |
enum | GameStatus { Init = 0 , Run = 1 , Pause = 2 , End = 3 , Abort = 4 , SystemPause = 5 , Intro = 6 , UserStatus = 7 } |
typedef QList< KPlayer * > | KGamePlayerList |
![]() | |
typedef | QObjectList |
Signals | |
void | signalClientJoinedGame (quint32 clientid, KGame *me) |
void | signalClientLeftGame (int clientID, int oldgamestatus, KGame *me) |
void | signalGameOver (int status, KPlayer *current, KGame *me) |
void | signalLoad (QDataStream &stream) |
void | signalLoadError (QDataStream &stream, bool network, int cookie, bool &result) |
void | signalLoadPrePlayers (QDataStream &stream) |
void | signalMessageUpdate (int msgid, quint32 receiver, quint32 sender) |
void | signalNetworkData (int msgid, const QByteArray &buffer, quint32 receiver, quint32 sender) |
void | signalPlayerJoinedGame (KPlayer *player) |
void | signalPlayerLeftGame (KPlayer *player) |
void | signalPropertyChanged (KGamePropertyBase *property, KGame *me) |
void | signalReplacePlayerIO (KPlayer *player, bool *remove) |
void | signalSave (QDataStream &stream) |
void | signalSavePrePlayers (QDataStream &stream) |
![]() | |
void | signalAdminStatusChanged (bool isAdmin) |
void | signalClientConnected (quint32 clientID) |
void | signalClientDisconnected (quint32 clientID, bool broken) |
void | signalConnectionBroken () |
void | signalNetworkErrorMessage (int error, const QString &text) |
Public Member Functions | |
KGame (int cookie=42, QObject *parent=nullptr) | |
~KGame () override | |
bool | activatePlayer (KPlayer *player) |
bool | addPlayer (KPlayer *newplayer) |
bool | addProperty (KGamePropertyBase *data) |
virtual KPlayer * | createPlayer (int rtti, int io, bool isvirtual) |
KGamePropertyHandler * | dataHandler () const |
void | Debug () override |
KPlayer * | findPlayer (quint32 id) const |
KGamePropertyBase * | findProperty (int id) const |
KGameSequence * | gameSequence () const |
int | gameStatus () const |
bool | inactivatePlayer (KPlayer *player) |
KGamePlayerList * | inactivePlayerList () |
const KGamePlayerList * | inactivePlayerList () const |
bool | isRunning () const |
virtual bool | load (const QString &filename, bool reset=true) |
virtual bool | load (QDataStream &stream, bool reset=true) |
int | maxPlayers () const |
uint | minPlayers () const |
void | networkTransmission (QDataStream &stream, int msgid, quint32 receiver, quint32 sender, quint32 clientID) override |
uint | playerCount () const |
void | playerDeleted (KPlayer *player) |
KGamePlayerList * | playerList () |
const KGamePlayerList * | playerList () const |
GamePolicy | policy () const |
bool | removePlayer (KPlayer *player) |
virtual bool | reset () |
virtual bool | save (const QString &filename, bool saveplayers=true) |
virtual bool | save (QDataStream &stream, bool saveplayers=true) |
bool | sendGroupMessage (const QByteArray &msg, int msgid, quint32 sender, const QString &group) |
bool | sendGroupMessage (const QDataStream &msg, int msgid, quint32 sender, const QString &group) |
bool | sendGroupMessage (const QString &msg, int msgid, quint32 sender, const QString &group) |
bool | sendGroupMessage (int msg, int msgid, quint32 sender, const QString &group) |
virtual bool | sendPlayerInput (QDataStream &msg, KPlayer *player, quint32 sender=0) |
bool | sendPlayerProperty (int msgid, QDataStream &s, quint32 playerId) |
void | setGameSequence (KGameSequence *sequence) |
void | setGameStatus (int status) |
void | setMaxPlayers (uint maxnumber) |
void | setMinPlayers (uint minnumber) |
void | setPolicy (GamePolicy p, bool recursive=true) |
virtual bool | systemPlayerInput (QDataStream &msg, KPlayer *player, quint32 sender=0) |
![]() | |
KGameNetwork (int cookie=42, QObject *parent=nullptr) | |
bool | connectToServer (const QString &host, quint16 port) |
bool | connectToServer (KMessageIO *connection) |
int | cookie () const |
void | disconnect () |
void | electAdmin (quint32 clientID) |
quint32 | gameId () const |
QString | hostName () const |
bool | isAdmin () const |
bool | isMaster () const |
bool | isNetwork () const |
bool | isOfferingConnections () const |
virtual void | lock () |
KMessageClient * | messageClient () const |
KMessageServer * | messageServer () const |
bool | offerConnections (quint16 port) |
quint16 | port () const |
void | sendError (int error, const QByteArray &message, quint32 receiver=0, quint32 sender=0) |
bool | sendMessage (const QByteArray &buffer, int msgid, quint32 receiver=0, quint32 sender=0) |
bool | sendMessage (const QDataStream &msg, int msgid, quint32 receiver=0, quint32 sender=0) |
bool | sendMessage (const QString &msg, int msgid, quint32 receiver=0, quint32 sender=0) |
bool | sendMessage (int data, int msgid, quint32 receiver=0, quint32 sender=0) |
bool | sendSystemMessage (const QByteArray &buffer, int msgid, quint32 receiver=0, quint32 sender=0) |
bool | sendSystemMessage (const QDataStream &msg, int msgid, quint32 receiver=0, quint32 sender=0) |
bool | sendSystemMessage (const QString &msg, int msgid, quint32 receiver=0, quint32 sender=0) |
bool | sendSystemMessage (int data, int msgid, quint32 receiver=0, quint32 sender=0) |
void | setDiscoveryInfo (const QString &type, const QString &name=QString()) |
void | setMaxClients (int max) |
bool | stopServerConnection () |
virtual void | unlock () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Slots | |
void | emitSignal (KGamePropertyBase *me) |
void | sendProperty (int msgid, QDataStream &stream, bool *sent) |
void | slotClientConnected (quint32 clientId) |
void | slotClientDisconnected (quint32 clientId, bool broken) |
void | slotServerDisconnected () |
![]() | |
void | aboutToLoseConnection (quint32 id) |
void | receiveNetworkTransmission (const QByteArray &a, quint32 clientID) |
void | slotAdminStatusChanged (bool isAdmin) |
void | slotResetConnection () |
Protected Member Functions | |
void | deleteInactivePlayers () |
void | deletePlayers () |
virtual bool | loadgame (QDataStream &stream, bool network, bool reset) |
KPlayer * | loadPlayer (QDataStream &stream, bool isvirtual=false) |
virtual void | negotiateNetworkGame (quint32 clientID) |
virtual void | newPlayersJoin (KGamePlayerList *oldplayer, KGamePlayerList *newplayer, QList< int > &inactivate) |
virtual bool | playerInput (QDataStream &msg, KPlayer *player)=0 |
KPlayer * | playerInputFinished (KPlayer *player) |
virtual bool | savegame (QDataStream &stream, bool network, bool saveplayers) |
void | savePlayer (QDataStream &stream, KPlayer *player) |
void | savePlayers (QDataStream &stream, KGamePlayerList *list=nullptr) |
bool | systemActivatePlayer (KPlayer *player) |
bool | systemAddPlayer (KPlayer *newplayer) |
bool | systemInactivatePlayer (KPlayer *player) |
void | systemRemovePlayer (KPlayer *player, bool deleteit) |
![]() | |
void | setMaster () |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Detailed Description
The main KDE game object.
The KGame class is the central game object. A game basically consists of following features:
- Player handling (add, remove,...)
- Game status (end,start,pause,...)
- load/save
- Move (and message) handling
- nextPlayer and gameOver()
- Network connection (for KGameNetwork)
Example:
Member Typedef Documentation
◆ KGamePlayerList
Member Enumeration Documentation
◆ GamePolicy
enum KGame::GamePolicy |
The policy of the property.
This can be PolicyClean (setVale uses send), PolicyDirty (setValue uses changeValue) or PolicyLocal (setValue uses setLocal).
A "clean" policy means that the property is always the same on every client. This is achieved by calling send which actually changes the value only when the message from the MessageServer is received.
A "dirty" policy means that as soon as setValue is called the property is changed immediately. And additionally sent over network. This can sometimes lead to bugs as the other clients do not immediately have the same value. For more information see changeValue.
PolicyLocal means that a KGameProperty behaves like ever "normal" variable. Whenever setValue is called (e.g. using "=") the value of the property is changes immediately without sending it over network. You might want to use this if you are sure that all clients set the property at the same time.
◆ GameStatus
enum KGame::GameStatus |
Constructor & Destructor Documentation
◆ KGame()
|
explicit |
◆ ~KGame()
Member Function Documentation
◆ activatePlayer()
bool KGame::activatePlayer | ( | KPlayer * | player | ) |
◆ addPlayer()
bool KGame::addPlayer | ( | KPlayer * | newplayer | ) |
Note that KPlayer::save must be implemented properly, as well as KPlayer::rtti This will only send a message to all clients.
The player is not added directly! See also playerInput which will be called as soon as the player really has been added.
Note that an added player will first get into a "queue" and won't be in the game. It will be added to the game as soon as systemAddPlayer is called what will happen as soon as IdAddPlayer is received.
Note: you probably want to connect to signalPlayerJoinedGame for further initialization!
- Parameters
-
newplayer The player you want to add. KGame will send a message to all clients and add the player using systemAddPlayer
◆ addProperty()
bool KGame::addProperty | ( | KGamePropertyBase * | data | ) |
◆ createPlayer()
|
virtual |
This virtual function is called if the KGame needs to create a new player.
This happens only over a network and with load/save. Doing nothing will create a default KPlayer. If you want to have your own player you have to create one with the given rtti here. Note: If your game uses a player class derived from KPlayer you MUST override this function and create your player here. Otherwise the game will crash. Example:
◆ dataHandler()
KGamePropertyHandler * KGame::dataHandler | ( | ) | const |
◆ Debug()
|
overridevirtual |
Gives debug output of the game status.
Reimplemented from KGameNetwork.
◆ deleteInactivePlayers()
◆ deletePlayers()
◆ emitSignal
|
protectedslot |
Called by KGamePropertyHandler only! Internal function!
◆ findPlayer()
KPlayer * KGame::findPlayer | ( | quint32 | id | ) | const |
◆ findProperty()
KGamePropertyBase * KGame::findProperty | ( | int | id | ) | const |
◆ gameSequence()
KGameSequence * KGame::gameSequence | ( | ) | const |
- Returns
- The KGameSequence object that is currently in use.
- See also
- setGameSequence
◆ gameStatus()
int KGame::gameStatus | ( | ) | const |
◆ inactivatePlayer()
bool KGame::inactivatePlayer | ( | KPlayer * | player | ) |
◆ inactivePlayerList() [1/2]
KGame::KGamePlayerList * KGame::inactivePlayerList | ( | ) |
◆ inactivePlayerList() [2/2]
const KGame::KGamePlayerList * KGame::inactivePlayerList | ( | ) | const |
The same as inactivePlayerList but returns a const pointer.
◆ isRunning()
bool KGame::isRunning | ( | ) | const |
◆ load() [1/2]
|
virtual |
◆ load() [2/2]
|
virtual |
Load a saved game, from file OR network.
This function has to be overwritten or you need to connect to the load signal if you have game data other than KGameProperty. For file load you should reset() the game before any load attempt to make sure you load into an clear state.
- Parameters
-
stream a data stream where you can stream the game from reset - shall the game be reset before loading
- Returns
- true?
◆ loadgame()
|
protectedvirtual |
Load a saved game, from file OR network.
Internal. Warning: loadgame must not rely that all players all already activated. Actually the network will activate a player AFTER the loadgame only. This is not true anymore. But be careful anyway.
- Parameters
-
stream a data stream where you can stream the game from network is it a network call -> make players virtual reset shall the game be reset before loading
- Returns
- true?
◆ loadPlayer()
|
protected |
◆ maxPlayers()
int KGame::maxPlayers | ( | ) | const |
◆ minPlayers()
uint KGame::minPlayers | ( | ) | const |
◆ negotiateNetworkGame()
|
protectedvirtual |
This member function will transmit e.g.
all players to that client, as well as all properties of these players (at least if they have been added by KPlayer::addProperty) so that the client will finally have the same status as the master. You want to overwrite this function if you expand KGame by any properties which have to be known by all clients.
Only the ADMIN is allowed to call this.
- Parameters
-
clientID The ID of the message client which has connected
◆ networkTransmission()
|
overridevirtual |
This will either forward an incoming message to a specified player (see KPlayer::networkTransmission) or handle the message directly (e.g.
if msgif==IdRemovePlayer it will remove the (in the stream) specified player). If both is not possible (i.e. the message is user specified data) the signal signalNetworkData is emitted.
This emits signalMessageUpdate before doing anything with the message. You can use this signal when you want to be notified about an update/change.
- Parameters
-
msgid Specifies the kind of the message. See messages.txt for further information stream The message that is being sent receiver The is of the player this message is for. 0 For broadcast. sender clientID the client from which we received the transmission - hardly used
Implements KGameNetwork.
◆ newPlayersJoin()
|
inlineprotectedvirtual |
This virtual function can be overwritten for your own player management.
It is called when a new game connects to an existing network game or to the network master. In case you do not want all players of both games to be present in the new network game, you can deactivate players here. This is of particular importance if you have a game with fixed number of player like e.g. chess. A network connect needs to disable one player of each game to make sense.
Not overwriting this function will activate a default behaviour which will deactivate players until the maxPlayers() number is reached according to the KPlayer::networkPriority() value. Players with a low value will be kicked out first. With equal priority players of the new client will leave first. This means, not setting this value and not overwriting this function will never allow a chess game to add client players!!! On the other hand setting one player of each game to a networkPriorty of say 10, already does most of the work for you.
The parameters of this function are the playerlist of the network game, which is playerList(). The second argument is the player list of the new client who wants to join and the third argument serves as return parameter. All player ID's which are written into this list will be removed from the created game. You do this by an
- Parameters
-
oldplayer - the list of the network players newplayer - the list of the client players inactivate - the value list of ids to be deactivated
◆ playerCount()
uint KGame::playerCount | ( | ) | const |
◆ playerDeleted()
void KGame::playerDeleted | ( | KPlayer * | player | ) |
◆ playerInput()
|
protectedpure virtual |
A player input occurred.
This is the most important function as the given message will contain the current move made by the given player. Note that you HAVE to overwrite this function. Otherwise your game makes no sense at all. Generally you have to return TRUE in this function. Only then the game sequence is proceeded by calling playerInputFinished which in turn will check for game over or the next player However, if you have a delayed move, because you e.g. move a card or a piece you want to return FALSE to pause the game sequence and then manually call playerInputFinished to resume it. Example:
- Parameters
-
msg the move message player the player who did the move
- Returns
- true - input ready, false: input manual
◆ playerInputFinished()
Called after the player input is processed by the game.
Here the checks for game over and nextPlayer (in the case of turn base games) are processed. Call this manually if you have a delayed move, i.e. your playerInput function returns FALSE. If it returns true you need not do anything here.
- Returns
- the current player
◆ playerList() [1/2]
KGame::KGamePlayerList * KGame::playerList | ( | ) |
◆ playerList() [2/2]
const KGame::KGamePlayerList * KGame::playerList | ( | ) | const |
The same as playerList but returns a const pointer.
◆ policy()
KGame::GamePolicy KGame::policy | ( | ) | const |
◆ removePlayer()
|
inline |
◆ reset()
|
virtual |
◆ save() [1/2]
|
virtual |
◆ save() [2/2]
|
virtual |
◆ savegame()
|
protectedvirtual |
◆ savePlayer()
|
protected |
Prepare a player for being added.
Put all data about a player into the stream so that it can be sent to the KGameCommunicationServer using addPlayer (e.g.)
This function ensures that the code for adding a player is the same in addPlayer as well as in negotiateNetworkGame
- Parameters
-
stream is the stream to add the player player The player to add
◆ savePlayers()
|
protected |
Save the player list to a stream.
Used for network game and load/save. Can be overwritten if you know what you are doing
- Parameters
-
stream is the stream to save the player ot list the optional list is the player list to be saved, default is playerList()
◆ sendGroupMessage() [1/3]
bool KGame::sendGroupMessage | ( | const QByteArray & | msg, |
int | msgid, | ||
quint32 | sender, | ||
const QString & | group ) |
See KGameNetwork::sendMessage.
Send a network message msg with a given message ID msgid to all players of a given group (see KPlayer::group)
- Parameters
-
msg the message which will be send. See messages.txt for contents msgid an id for this message sender the id of the sender group the group of the receivers
- Returns
- true if worked
◆ sendGroupMessage() [2/3]
bool KGame::sendGroupMessage | ( | const QDataStream & | msg, |
int | msgid, | ||
quint32 | sender, | ||
const QString & | group ) |
◆ sendGroupMessage() [3/3]
◆ sendPlayerInput()
|
virtual |
Called by KPlayer to send a player input to the KMessageServer.
◆ sendPlayerProperty()
bool KGame::sendPlayerProperty | ( | int | msgid, |
QDataStream & | s, | ||
quint32 | playerId ) |
This is called by KPlayer::sendProperty only! Internal function!
◆ sendProperty
|
protectedslot |
Called by KGamePropertyHandler only! Internal function!
◆ setGameSequence()
void KGame::setGameSequence | ( | KGameSequence * | sequence | ) |
Set a new KGameSequence to control player management.
By default KGame uses a normal KGameSequence object. You might want to subclass that and provide your own object.
The previous sequence will get deleted.
- Parameters
-
sequence The new game sequence object. KGame takes ownership and will delete it on destruction!
◆ setGameStatus()
void KGame::setGameStatus | ( | int | status | ) |
◆ setMaxPlayers()
void KGame::setMaxPlayers | ( | uint | maxnumber | ) |
◆ setMinPlayers()
void KGame::setMinPlayers | ( | uint | minnumber | ) |
◆ setPolicy()
void KGame::setPolicy | ( | GamePolicy | p, |
bool | recursive = true ) |
◆ signalClientJoinedGame
|
signal |
Is emitted after a client is successfully connected to the game.
The client id is the id of the new game client. An easy way to check whether that's us is
- Parameters
-
clientid - The id of the new client me - our game pointer
◆ signalClientLeftGame
|
signal |
This signal is emitted after a network partner left the game (either by a broken connection or voluntarily).
All changes to the network players have already be done. If there are not enough players left, the game might have been paused. To check this you get the old gamestatus before the disconnection as argument here. The id of the client who left the game allows to distinguish who left the game. If it is 0, the server disconnected and you were a client which has been switched back to local play. You can use this signal to, e.g. set some menus back to local player when they were network before.
- Parameters
-
clientID - 0:server left, otherwise the client who left oldgamestatus - the gamestatus before the loss me - our game pointer
◆ signalGameOver
Is emitted after a call to gameOver() returns a non zero return code.
This code is forwarded to this signal as 'status'.
- Parameters
-
status the return code of gameOver() current the player who did the last move me a pointer to the KGame object
◆ signalLoad
|
signal |
The game will be loaded from the given stream.
Load from here the data which is NOT a game or player property. It is not necessary to use this signal for a full property game.
- Parameters
-
stream the load stream
◆ signalLoadError
|
signal |
Is emitted if a game with a different version cookie is loaded.
Normally this should result in an error. But maybe you do support loading of older game versions. Here would be a good place to do a conversion.
- Parameters
-
stream - the load stream network - true if this is a network connect. False for load game cookie - the saved cookie. It differs from KGame::cookie() result - set this to true if you managed to load the game
◆ signalLoadPrePlayers
|
signal |
The game will be loaded from the given stream.
Load from here the data which is NOT a game or player property. It is not necessary to use this signal for a full property game.
This signal is emitted before the players are loaded by KGame. See also signalLoad
You must load exactly the same data from the stream that you have saved in signalSavePrePlayers. Otherwise player loading will not work anymore.
- Parameters
-
stream the load stream
◆ signalMessageUpdate
|
signal |
We got an network message.
this can be used to notify us that something changed. What changed can be seen in the message id. Whether this is the best possible method to do this is unclear...
◆ signalNetworkData
|
signal |
◆ signalPlayerJoinedGame
|
signal |
a player joined the game
- Parameters
-
player the player who joined the game
◆ signalPlayerLeftGame
|
signal |
a player left the game because of a broken connection or so!
Note that when this signal is emitted the player is not part of playerList anymore but the pointer is still valid. You should do some final cleanups here since the player is usually deleted after the signal is emitted.
- Parameters
-
player the player who left the game
◆ signalPropertyChanged
|
signal |
This signal is emitted if a player property changes its value and the property is set to notify this change.
◆ signalReplacePlayerIO
|
signal |
When a client disconnects from the game usually all players from that client are removed.
But if you use completely the KGame structure you probably don't want this. You just want to replace the KGameIO of the (human) player by a computer KGameIO. So this player continues game but is from this point on controlled by the computer.
You achieve this by connecting to this signal. It is emitted as soon as a client disconnects on all other clients. Make sure to add a new KGameIO only once! you might want to use isAdmin for this. If you added a new KGameIO set *remove=false otherwise the player is completely removed.
- Parameters
-
player The player that is about to be removed. Add your new KGameIO here - but only on one client! remove Set this to FALSE if you don't want this player to be removed completely.
◆ signalSave
|
signal |
The game will be saved to the given stream.
Fill this with data which is NOT a game or player property. It is not necessary to use this signal for a full property game.
- Parameters
-
stream the save stream
◆ signalSavePrePlayers
|
signal |
The game will be saved to the given stream.
Fill this with data which is NOT a game or player property. It is not necessary to use this signal for a full property game.
This signal is emitted before the players are saved by KGame. See also signalSave
If you can choose between signalSavePrePlayers and signalSave then better use signalSave
- Parameters
-
stream the save stream
◆ slotClientConnected
|
protectedslot |
◆ slotClientDisconnected
|
protectedslot |
This slot is called whenever the connection to a client is lost (ie the signal KGameNetwork::signalClientDisconnected is emitted) and will remove the players from that client.
- Parameters
-
clientId The client the connection has been lost to broken (ignore this - not used)
◆ slotServerDisconnected
|
protectedslot |
This slot is called whenever the connection to the server is lost (ie the signal KGameNetwork::signalConnectionBroken is emitted) and will switch to local game mode.
◆ systemActivatePlayer()
|
protected |
◆ systemAddPlayer()
|
protected |
Adds a player to the game.
Use addPlayer to send KGameMessage::IdAddPlayer. As soon as this Id is received this function is called, where the player (see KPlayer::rtti) is added as well as its properties (see KPlayer::save and KPlayer::load)
This method calls the overloaded systemAddPlayer with the created player as argument. That method will really add the player. If you need to do some changes to your newly added player just connect to signalPlayerJoinedGame Finally adds a player to the game and therefore to the list.
◆ systemInactivatePlayer()
|
protected |
◆ systemPlayerInput()
|
virtual |
Called when a player input arrives from KMessageServer.
Calls prepareNext (using QTimer::singleShot) if gameOver() returns 0. This function should normally not be used outside KGame. It could be made non-virtual,protected in a later version. At the moment it is a virtual function to give you more control over KGame.
For documentation see playerInput.
◆ systemRemovePlayer()
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:59:04 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.