KGamePropertyBase
#include <KGame/KGameProperty>
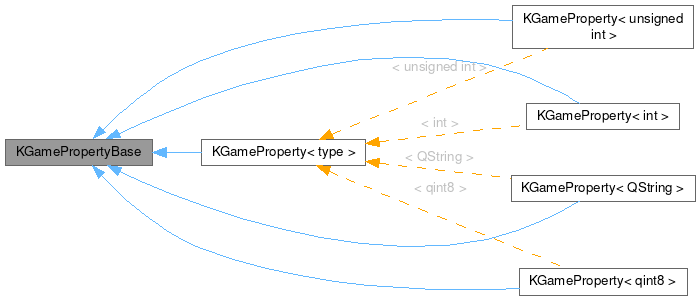
Public Types | |
enum | PropertyCommandIds { CmdLock = 1 , CmdAt = 51 , CmdResize = 52 , CmdFill = 53 , CmdSort = 54 , CmdInsert = 61 , CmdAppend = 62 , CmdRemove = 63 , CmdClear = 64 } |
enum | PropertyDataIds { IdGroup = 1 , IdUserId = 2 , IdAsyncInput = 3 , IdTurn = 4 , IdName = 5 , IdGameStatus = 6 , IdMaxPlayer = 7 , IdMinPlayer = 8 , IdGrabInput = 16 , IdReleaseInput = 17 , IdCommand , IdUser = 256 , IdAutomatic = 0x7000 } |
enum | PropertyPolicy { PolicyUndefined = 0 , PolicyClean = 1 , PolicyDirty = 2 , PolicyLocal = 3 } |
Public Member Functions | |
KGamePropertyBase () | |
KGamePropertyBase (int id, KGame *parent) | |
KGamePropertyBase (int id, KGamePropertyHandler *owner) | |
KGamePropertyBase (int id, KPlayer *parent) | |
virtual void | command (QDataStream &stream, int msgid, bool isSender=false) |
int | id () const |
bool | isDirty () const |
bool | isEmittingSignal () const |
bool | isLocked () const |
bool | isOptimized () const |
virtual void | load (QDataStream &s)=0 |
bool | lock () |
PropertyPolicy | policy () const |
int | registerData (int id, KGame *owner, const QString &name=QString()) |
int | registerData (int id, KGamePropertyHandler *owner, const QString &name=QString()) |
int | registerData (int id, KGamePropertyHandler *owner, PropertyPolicy p, const QString &name=QString()) |
int | registerData (int id, KPlayer *owner, const QString &name=QString()) |
int | registerData (KGamePropertyHandler *owner, PropertyPolicy p=PolicyUndefined, const QString &name=QString()) |
virtual void | save (QDataStream &s)=0 |
void | setEmittingSignal (bool p) |
void | setOptimized (bool p) |
void | setPolicy (PropertyPolicy p) |
virtual const type_info * | typeinfo () |
bool | unlock (bool force=false) |
void | unregisterData () |
Protected Member Functions | |
void | emitSignal () |
bool | sendProperty () |
bool | sendProperty (const QByteArray &b) |
void | setDirty (bool d) |
void | setLock (bool l) |
Protected Attributes | |
union KGamePropertyBase::Flags | mFlags |
KGamePropertyHandler * | mOwner |
Detailed Description
Base class of KGameProperty.
The KGamePropertyBase class is the base class of KGameProperty. See KGameProperty for further information.
Definition at line 36 of file kgameproperty.h.
Member Enumeration Documentation
◆ PropertyCommandIds
Commands for advanced properties (qint8)
Definition at line 65 of file kgameproperty.h.
◆ PropertyDataIds
enum KGamePropertyBase::PropertyDataIds |
Definition at line 39 of file kgameproperty.h.
◆ PropertyPolicy
The policy of the property.
This can be PolicyClean (setValue uses send), PolicyDirty (setValue uses changeValue) or PolicyLocal (setValue uses setLocal).
A "clean" policy means that the property is always the same on every client. This is achieved by calling send which actually changes the value only when the message from the MessageServer is received.
A "dirty" policy means that as soon as setValue is called the property is changed immediately. And additionally sent over network. This can sometimes lead to bugs as the other clients do not immediately have the same value. For more information see changeValue.
PolicyLocal means that a KGameProperty behaves like ever "normal" variable. Whenever setValue is called (e.g. using "=") the value of the property is changes immediately without sending it over network. You might want to use this if you are sure that all clients set the property at the same time.
Definition at line 103 of file kgameproperty.h.
Constructor & Destructor Documentation
◆ KGamePropertyBase() [1/4]
KGamePropertyBase::KGamePropertyBase | ( | int | id, |
KGamePropertyHandler * | owner ) |
Constructs a KGamePropertyBase object and calls registerData.
- Parameters
-
id The id of this property. MUST be UNIQUE! Used to send and receive changes in the property of the player automatically via network. owner The owner of the object. Must be a KGamePropertyHandler which manages the changes made to this object, i.e. which will send the new data
Definition at line 32 of file kgameproperty.cpp.
◆ KGamePropertyBase() [2/4]
KGamePropertyBase::KGamePropertyBase | ( | int | id, |
KGame * | parent ) |
Definition at line 20 of file kgameproperty.cpp.
◆ KGamePropertyBase() [3/4]
KGamePropertyBase::KGamePropertyBase | ( | int | id, |
KPlayer * | parent ) |
Definition at line 26 of file kgameproperty.cpp.
◆ KGamePropertyBase() [4/4]
KGamePropertyBase::KGamePropertyBase | ( | ) |
Creates a KGamePropertyBase object without an owner.
Remember to call registerData!
Definition at line 38 of file kgameproperty.cpp.
◆ ~KGamePropertyBase()
|
virtual |
Definition at line 43 of file kgameproperty.cpp.
Member Function Documentation
◆ command()
|
virtual |
send a command to advanced properties like arrays
- Parameters
-
stream The stream containing the data of the command msgid The ID of the command - see PropertyCommandIds isSender whether this client is also the sender of the command
Definition at line 191 of file kgameproperty.cpp.
◆ emitSignal()
|
protected |
Causes the parent object to emit a signal on value change.
Definition at line 181 of file kgameproperty.cpp.
◆ id()
|
inline |
- Returns
- The id of this property
Definition at line 254 of file kgameproperty.h.
◆ isDirty()
|
inline |
- Returns
- Whether this property is "dirty". See also setDirty
Definition at line 190 of file kgameproperty.h.
◆ isEmittingSignal()
|
inline |
See also setEmittingSignal.
- Returns
- Whether this property emits a signal on value change
Definition at line 164 of file kgameproperty.h.
◆ isLocked()
|
inline |
A locked property can only be changed by the player who has set the lock.
See also setLocked
- Returns
- Whether this property is currently locked.
Definition at line 200 of file kgameproperty.h.
◆ isOptimized()
|
inline |
See also setOptimize.
- Returns
- Whether the property optimizes access (signals,network traffic)
Definition at line 182 of file kgameproperty.h.
◆ load()
|
pure virtual |
This will read the value of this property from the stream.
You MUST overwrite this method in order to use this class
- Parameters
-
s The stream to read from
Implemented in KGameProperty< type >, KGameProperty< int >, KGameProperty< int >, KGameProperty< qint8 >, KGameProperty< qint8 >, KGameProperty< QString >, KGameProperty< QString >, KGameProperty< unsigned int >, and KGameProperty< unsigned int >.
◆ lock()
bool KGamePropertyBase::lock | ( | ) |
A locked property can only be changed by the player who has set the lock.
You can only call this if isLocked is false. A message is sent over network so that the property is locked for all players except you.
- Returns
- returns false if the property can not be locked, i.e. it is already locked
Definition at line 148 of file kgameproperty.cpp.
◆ policy()
|
inline |
- Returns
- The default policy of the property
Definition at line 145 of file kgameproperty.h.
◆ registerData() [1/5]
This is an overloaded member function, provided for convenience.
It differs from the above function only in what argument(s) it accepts.
Definition at line 67 of file kgameproperty.cpp.
◆ registerData() [2/5]
int KGamePropertyBase::registerData | ( | int | id, |
KGamePropertyHandler * | owner, | ||
const QString & | name = QString() ) |
This is an overloaded member function, provided for convenience.
It differs from the above function only in what argument(s) it accepts.
Definition at line 82 of file kgameproperty.cpp.
◆ registerData() [3/5]
int KGamePropertyBase::registerData | ( | int | id, |
KGamePropertyHandler * | owner, | ||
PropertyPolicy | p, | ||
const QString & | name = QString() ) |
You have to register a KGamePropertyBase before you can use it.
You MUST call this before you can use KGamePropertyBase!
- Parameters
-
id the id of this KGamePropertyBase object. The id MUST be unique, i.e. you cannot have two properties with the same id for one player, although (currently) nothing prevents you from doing so. But you will get strange results! owner The owner of this data. This will send the data using KPropertyHandler::sendProperty whenever you call send p If not 0 you can set the policy of the property here name if not 0 you can assign a name to this property
Definition at line 87 of file kgameproperty.cpp.
◆ registerData() [4/5]
This is an overloaded member function, provided for convenience.
It differs from the above function only in what argument(s) it accepts.
Definition at line 72 of file kgameproperty.cpp.
◆ registerData() [5/5]
int KGamePropertyBase::registerData | ( | KGamePropertyHandler * | owner, |
PropertyPolicy | p = PolicyUndefined, | ||
const QString & | name = QString() ) |
This is an overloaded member function, provided for convenience.
It differs from the above function only in what argument(s) it accepts. In particular you can use this function to create properties which will have an automatic id assigned. The new id is returned.
Definition at line 77 of file kgameproperty.cpp.
◆ save()
|
pure virtual |
Write the value into a stream.
MUST be overwritten
Implemented in KGameProperty< type >, KGameProperty< int >, KGameProperty< int >, KGameProperty< qint8 >, KGameProperty< qint8 >, KGameProperty< QString >, KGameProperty< QString >, KGameProperty< unsigned int >, and KGameProperty< unsigned int >.
◆ sendProperty() [1/2]
|
protected |
Forward the data to the owner of this property which then sends it over network.
save is used to store the data into a stream so you have to make sure that function is working properly if you implement your own property!
Note: this sends the current property!
Might be obsolete - KGamePropertyArray still uses it. Is this a bug or correct?
Definition at line 120 of file kgameproperty.cpp.
◆ sendProperty() [2/2]
|
protected |
Forward the data to the owner of this property which then sends it over network.
save is used to store the data into a stream so you have to make sure that function is working properly if you implement your own property!
This function is used by send to send the data over network. This does not send the current value but the explicitly given value.
- Returns
- TRUE if the message could be sent successfully, otherwise FALSE
Definition at line 134 of file kgameproperty.cpp.
◆ setDirty()
|
inlineprotected |
Sets the "dirty" flag of the property.
If a property is "dirty" i.e. KGameProperty::setLocal has been called there is no guarantee that all clients share the same value. You have to ensure this yourself e.g. by calling KGameProperty::setLocal on every client. You can also ignore the dirty flag and continue working withe the property depending on your situation.
Definition at line 336 of file kgameproperty.h.
◆ setEmittingSignal()
|
inline |
Sets this property to emit a signal on value changed.
As the properties do not inherit QObject for optimization this signal is emitted via the KPlayer or KGame object
Definition at line 155 of file kgameproperty.h.
◆ setLock()
|
protected |
A locked property can only be changed by the player who has set the lock.
You can only call this if isLocked is false. A message is sent over network so that the property is locked for all players except you. Usually you use lock and unlock to access this property
Definition at line 166 of file kgameproperty.cpp.
◆ setOptimized()
|
inline |
Sets this property to try to optimize signal and network handling by not sending it out when the property value is not changed.
Definition at line 173 of file kgameproperty.h.
◆ setPolicy()
|
inline |
Changes the consistency policy of a property.
The PropertyPolicy is one of PolicyClean (defaulz), PolicyDirty or PolicyLocal.
It is up to you to decide how you want to work.
Definition at line 137 of file kgameproperty.h.
◆ typeinfo()
|
inlinevirtual |
- Returns
- a type_info of the data this property contains.
Reimplemented in KGameProperty< type >, KGameProperty< int >, KGameProperty< int >, KGameProperty< qint8 >, KGameProperty< qint8 >, KGameProperty< QString >, KGameProperty< QString >, KGameProperty< unsigned int >, and KGameProperty< unsigned int >.
Definition at line 262 of file kgameproperty.h.
◆ unlock()
bool KGamePropertyBase::unlock | ( | bool | force = false | ) |
A locked property can only be changed by the player who has set the lock.
You can only call this if isLocked is false. A message is sent over network so that the property is locked for all players except you.
- Returns
- returns false if the property can not be locked, i.e. it is already locked
Definition at line 157 of file kgameproperty.cpp.
◆ unregisterData()
void KGamePropertyBase::unregisterData | ( | ) |
Definition at line 111 of file kgameproperty.cpp.
Member Data Documentation
◆ mOwner
|
protected |
Definition at line 375 of file kgameproperty.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:57:56 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.