KPlayer
#include <KPlayer>
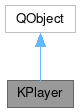
Public Types | |
typedef QList< KGameIO * > | KGameIOList |
Signals | |
void | signalNetworkData (int msgid, const QByteArray &buffer, quint32 sender, KPlayer *me) |
void | signalPropertyChanged (KGamePropertyBase *property, KPlayer *me) |
Public Member Functions | |
KPlayer () | |
bool | addGameIO (KGameIO *input) |
bool | addProperty (KGamePropertyBase *data) |
bool | asyncInput () const |
int | calcIOValue () |
KGamePropertyHandler * | dataHandler () |
void | Debug () |
KGamePropertyBase * | findProperty (int id) const |
KGameIO * | findRttiIO (int rtti) const |
virtual bool | forwardInput (QDataStream &msg, bool transmit=true, quint32 sender=0) |
virtual bool | forwardMessage (QDataStream &msg, int msgid, quint32 receiver=0, quint32 sender=0) |
KGame * | game () const |
virtual const QString & | group () const |
bool | hasRtti (int rtti) const |
quint32 | id () const |
KGameIOList * | ioList () |
bool | isActive () const |
bool | isVirtual () const |
virtual bool | load (QDataStream &stream) |
bool | myTurn () const |
virtual const QString & | name () const |
KPlayer * | networkPlayer () const |
int | networkPriority () const |
void | networkTransmission (QDataStream &stream, int msgid, quint32 sender) |
bool | removeGameIO (KGameIO *input=nullptr, bool deleteit=true) |
virtual int | rtti () const |
virtual bool | save (QDataStream &stream) |
void | setActive (bool v) |
void | setAsyncInput (bool a) |
void | setGame (KGame *game) |
void | setGroup (const QString &group) |
void | setId (quint32 i) |
void | setName (const QString &name) |
void | setNetworkPlayer (KPlayer *p) |
void | setNetworkPriority (int b) |
bool | setTurn (bool b, bool exclusive=true) |
void | setUserId (int i) |
void | setVirtual (bool v) |
int | userId () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Slots | |
void | emitSignal (KGamePropertyBase *me) |
void | sendProperty (int msgid, QDataStream &stream, bool *sent) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Base class for a game player.
The KPlayer class is the central player object. It holds information about the player and is responsible for any input the player does. For this arbitrary many KGameIO modules can be plugged into it. Main features are:
- Handling of IO devices
- load/save (mostly handled by KGamePropertyHandler)
- Turn handling (turn based, asynchronous)
A KPlayer depends on a KGame object. Call KGame::addPlayer() to plug a KPlayer into a KGame object. Note that you cannot do much with a KPlayer object before it has been plugged into a KGame. This is because most properties of KPlayer are KGameProperty which need to send messages through a KGame object to be changed.
A KGameIO represents the input methods of a player and you should make all player inputs through it. So call something like playerInput->move(4); instead which should call KGameIO::sendInput() to actually move. This way you gain a very big advantage: you can exchange a KGameIO whenever you want! You can e.g. remove the KGameIO of a local (human) player and just replace it by a computerIO on the fly! So from that point on all playerInputs are done by the computerIO instead of the human player. You also can replace all network players by computer players when the network connection is broken or a player wants to quit. So remember: use KGameIO whenever possible! A KPlayer should just contain all data of the player (KGameIO must not!) and several common functions which are shared by all of your KGameIOs.
Member Typedef Documentation
◆ KGameIOList
typedef QList<KGameIO *> KPlayer::KGameIOList |
Constructor & Destructor Documentation
◆ KPlayer()
|
explicit |
Create a new player object.
It will be automatically deleted if the game it belongs to is deleted.
Definition at line 56 of file kplayer.cpp.
◆ ~KPlayer()
|
override |
Definition at line 88 of file kplayer.cpp.
Member Function Documentation
◆ addGameIO()
bool KPlayer::addGameIO | ( | KGameIO * | input | ) |
Adds an IO device for the player.
Possible KGameIO devices can either be taken from the existing ones or be self written. Existing are e.g. Keyboard, Mouse, Computerplayer
- Parameters
-
input the inut device
- Returns
- true if ok
Definition at line 267 of file kplayer.cpp.
◆ addProperty()
bool KPlayer::addProperty | ( | KGamePropertyBase * | data | ) |
Adds a property to a player.
You would add all your player specific game data as KGameProperty and they are automatically saved and exchanged over network.
- Parameters
-
data The property to be added. Must have an unique id!
- Returns
- false if the given id is not valid (ie another property owns the id) or true if the property could be added successfully
Definition at line 419 of file kplayer.cpp.
◆ asyncInput()
bool KPlayer::asyncInput | ( | ) | const |
Query whether this player does asynchronous input.
- Returns
- true/false
Definition at line 130 of file kplayer.cpp.
◆ calcIOValue()
int KPlayer::calcIOValue | ( | ) |
Calculates a checksum over the IO devices.
Can be used to restore the IO handlers. The value returned is the 'or'ed value of the KGameIO rtti's. this is internally used for saving and restoring a player.
Definition at line 319 of file kplayer.cpp.
◆ dataHandler()
KGamePropertyHandler * KPlayer::dataHandler | ( | ) |
- Returns
- the property handler
Definition at line 232 of file kplayer.cpp.
◆ Debug()
void KPlayer::Debug | ( | ) |
Gives debug output of the game status.
Definition at line 448 of file kplayer.cpp.
◆ emitSignal
|
protectedslot |
◆ findProperty()
KGamePropertyBase * KPlayer::findProperty | ( | int | id | ) | const |
Searches for a property of the player given its id.
- Parameters
-
id The id of the property
- Returns
- The property with the specified id
Definition at line 414 of file kplayer.cpp.
◆ findRttiIO()
KGameIO * KPlayer::findRttiIO | ( | int | rtti | ) | const |
Finds the KGameIO devies with the given rtti code.
E.g. find the mouse or network device
- Parameters
-
rtti the rtti code to be searched for
- Returns
- the KGameIO device
Definition at line 307 of file kplayer.cpp.
◆ forwardInput()
|
virtual |
Forwards input to the game object..internal use only.
This method is used by KGameIO::sendInput(). Use that function instead to send player inputs!
This function forwards a player input (see KGameIO classes) to the game object, see KGame, either to KGame::sendPlayerInput() (if transmit=true, ie the message has just been created) or to KGame::playerInput() (if player=false, ie the message was sent through KGame::sendPlayerInput).
Definition at line 173 of file kplayer.cpp.
◆ forwardMessage()
|
virtual |
Forwards Message to the game object..internal use only.
Definition at line 160 of file kplayer.cpp.
◆ game()
KGame * KPlayer::game | ( | ) | const |
Query to which game the player belongs to.
- Returns
- the game
Definition at line 120 of file kplayer.cpp.
◆ group()
|
virtual |
Query the group the player belongs to.
Definition at line 212 of file kplayer.cpp.
◆ hasRtti()
bool KPlayer::hasRtti | ( | int | rtti | ) | const |
Checks whether this player has a IO device of the given rtti type.
- Parameters
-
rtti the rtti typed to be checked for
- Returns
- true if it exists
Definition at line 302 of file kplayer.cpp.
◆ id()
quint32 KPlayer::id | ( | ) | const |
◆ ioList()
KPlayer::KGameIOList * KPlayer::ioList | ( | ) |
◆ isActive()
bool KPlayer::isActive | ( | ) | const |
Is this player an active player.
An player is usually inactivated if it is replaced by a network connection. But this could also be called manually
- Returns
- true/false
Definition at line 135 of file kplayer.cpp.
◆ isVirtual()
bool KPlayer::isVirtual | ( | ) | const |
Is this player a virtual player, i.e.
is it created by mirroring a real player from another network game. This mirroring is done automatically as soon as a network connection is build and it affects all players regardless what type
- Returns
- true/false
Definition at line 242 of file kplayer.cpp.
◆ load()
|
virtual |
Load a saved player, from file OR network.
By default all KGameProperty objects in the dataHandler of this player are loaded and saved when using load or save. If you need to save/load more you have to replace this function (and save). You will probably still want to call the default implementation additionally!
- Parameters
-
stream a data stream where you can stream the player from
- Returns
- true?
Definition at line 352 of file kplayer.cpp.
◆ myTurn()
bool KPlayer::myTurn | ( | ) | const |
◆ name()
|
virtual |
- Returns
- The name of the player.
Definition at line 222 of file kplayer.cpp.
◆ networkPlayer()
KPlayer * KPlayer::networkPlayer | ( | ) | const |
Returns the player which got inactivated to allow this player to be set up via network.
Mostly internal function
Definition at line 252 of file kplayer.cpp.
◆ networkPriority()
int KPlayer::networkPriority | ( | ) | const |
Returns whether this player can be replaced by a network connection player.
The name of this function can be improved ;-) If you do not overwrite the function to select what players shall play in a network the KGame does an automatic selection based on the networkPriority This is not a terrible important function at the moment.
- Returns
- true/false
Definition at line 257 of file kplayer.cpp.
◆ networkTransmission()
void KPlayer::networkTransmission | ( | QDataStream & | stream, |
int | msgid, | ||
quint32 | sender ) |
Receives a message.
- Parameters
-
msgid The kind of the message. See messages.txt for further information stream The message itself sender
Definition at line 387 of file kplayer.cpp.
◆ removeGameIO()
bool KPlayer::removeGameIO | ( | KGameIO * | input = nullptr, |
bool | deleteit = true ) |
remove (and delete) a game IO device
The remove IO(s) is/are deleted by default. If you do not want this set the parameter deleteit to false
- Parameters
-
input the device to be removed or 0 for all devices deleteit true (default) to delete the device otherwise just remove it
- Returns
- true on ok
Definition at line 278 of file kplayer.cpp.
◆ rtti()
|
virtual |
The identification of the player.
Overwrite this in classes inheriting KPlayer to run time identify them.
- Returns
- 0 for default KPlayer.
Definition at line 105 of file kplayer.cpp.
◆ save()
|
virtual |
Save a player to a file OR to network.
See also load
- Parameters
-
stream a data stream to load the player from
- Returns
- true?
Definition at line 375 of file kplayer.cpp.
◆ sendProperty
|
protectedslot |
◆ setActive()
void KPlayer::setActive | ( | bool | v | ) |
Set an player as active (true) or inactive (false)
- Parameters
-
v true=active, false=inactive
Definition at line 140 of file kplayer.cpp.
◆ setAsyncInput()
void KPlayer::setAsyncInput | ( | bool | a | ) |
Set whether this player can make turns/input all the time (true) or only when it is its turn (false) as it is used in turn based games.
- Parameters
-
a async=true turn based=false
Definition at line 125 of file kplayer.cpp.
◆ setGame()
void KPlayer::setGame | ( | KGame * | game | ) |
sets the game the player belongs to.
This is usually automatically done when adding a player
- Parameters
-
game the game
Definition at line 115 of file kplayer.cpp.
◆ setGroup()
void KPlayer::setGroup | ( | const QString & | group | ) |
A group the player belongs to.
This Can be set arbitrary by you.
Definition at line 207 of file kplayer.cpp.
◆ setId()
void KPlayer::setId | ( | quint32 | i | ) |
Definition at line 201 of file kplayer.cpp.
◆ setName()
void KPlayer::setName | ( | const QString & | name | ) |
Sets the name of the player.
This can be chosen arbitrary.
- Parameters
-
name The player's name
Definition at line 217 of file kplayer.cpp.
◆ setNetworkPlayer()
void KPlayer::setNetworkPlayer | ( | KPlayer * | p | ) |
◆ setNetworkPriority()
void KPlayer::setNetworkPriority | ( | int | b | ) |
Set whether this player can be replaced by a network player.
There are to possible games. The first type of game has arbitrary many players. As soon as a network players connects the game runs with more players (not tagged situation). The other type is e.g. games like chess which require a constant player number. In a network game situation you would tag one or both players of all participants. As soon as the connect the tagged player will then be replaced by the network partner and it is then controlled over the network. On connection loss the old situation is automatically restored.
The name of this function can be improved;-)
- Parameters
-
b should this player be tagged
Definition at line 262 of file kplayer.cpp.
◆ setTurn()
bool KPlayer::setTurn | ( | bool | b, |
bool | exclusive = true ) |
Sets whether this player is the next to turn.
If exclusive is given all other players are set to setTurn(false) and only this player can move
- Parameters
-
b true/false exclusive true (default)/ false
- Returns
- should be void
Definition at line 329 of file kplayer.cpp.
◆ setUserId()
void KPlayer::setUserId | ( | int | i | ) |
Definition at line 150 of file kplayer.cpp.
◆ setVirtual()
void KPlayer::setVirtual | ( | bool | v | ) |
Sets whether this player is virtual. This is internally called
- Parameters
-
v virtual true/false
Definition at line 237 of file kplayer.cpp.
◆ signalNetworkData
|
signal |
The player object got a message which was targeted at it but has no default method to process it.
This means probably a user message. Connecting to this signal allowed to process it.
◆ signalPropertyChanged
|
signal |
This signal is emitted if a player property changes its value and the property is set to notify this change.
This is an important signal as you should base the actions on a reaction to this property changes.
◆ userId()
int KPlayer::userId | ( | ) | const |
Returns the user defined id of the player This value can be used arbitrary by you to have some user identification for your player, e.g.
0 for a white chess player, 1 for a black one. This value is more reliable than the player id which can even change when you make a network connection.
- Returns
- the user defined player id
Definition at line 145 of file kplayer.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:56:01 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.