KGamePropertyHandler
#include <KGame/KGamePropertyHandler>
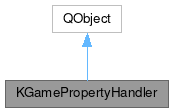
Signals | |
void | signalPropertyChanged (KGamePropertyBase *) |
void | signalRequestValue (KGamePropertyBase *property, QString &value) |
void | signalSendMessage (int msgid, QDataStream &, bool *sent) |
Public Member Functions | |
KGamePropertyHandler (int id, const QObject *receiver, const char *sendf, const char *emitf, QObject *parent=nullptr) | |
KGamePropertyHandler (QObject *parent=nullptr) | |
bool | addProperty (KGamePropertyBase *data, const QString &name=QString()) |
void | clear () |
void | Debug () |
QMultiHash< int, KGamePropertyBase * > & | dict () const |
void | emitSignal (KGamePropertyBase *data) |
KGamePropertyBase * | find (int id) |
void | flush () |
int | id () const |
virtual bool | load (QDataStream &stream) |
void | lockDirectEmit () |
void | lockProperties () |
KGamePropertyBase::PropertyPolicy | policy () |
bool | processMessage (QDataStream &stream, int id, bool isSender) |
QString | propertyName (int id) const |
QString | propertyValue (KGamePropertyBase *property) |
void | registerHandler (int id, const QObject *receiver, const char *send, const char *emit) |
bool | removeProperty (KGamePropertyBase *data) |
virtual bool | save (QDataStream &stream) |
void | sendLocked (bool l) |
bool | sendProperty (QDataStream &s) |
void | setId (int id) |
void | setPolicy (KGamePropertyBase::PropertyPolicy p, bool userspace=true) |
int | uniquePropertyId () |
void | unlockDirectEmit () |
void | unlockProperties () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
A collection class for KGameProperty objects.
The KGamePropertyHandler class is some kind of a collection class for KGameProperty. You usually don't have to create one yourself, as both KPlayer and KGame provide a handler. In most cases you do not even have to care about the KGamePropertHandler. KGame and KPlayer implement all features of KGamePropertyHandler so you will rather use it there.
You have to use the KGamePropertyHandler as parent for all KGameProperty objects but you can also use KPlayer or KGame as parent - then KPlayer::dataHandler or KGame::dataHandler will be used.
Every KGamePropertyHandler must have - just like every KGameProperty - a unique ID. This ID is provided either in the constructor or in registerHandler. The ID is used to assign an incoming message (e.g. a changed property) to the correct handler. Inside the handler the property ID is used to change the correct property.
The constructor or registerHandler takes 3 additional arguments: a receiver and two slots. The first slot is connected to signalSendMessage, the second to signalPropertyChanged. You must provide these in order to use the KGamePropertyHandler.
The most important function of KGamePropertyHandler is processMessage which assigns an incoming value to the correct property.
A KGamePropertyHandler is also used - indirectly using emitSignal - to emit a signal when the value of a property changes. This is done this way because a KGameProperty does not inherit QObject because of memory advantages. Many games can have dozens or even hundreds of KGameProperty objects so every additional variable in KGameProperty would be multiplied.
Definition at line 63 of file kgamepropertyhandler.h.
Constructor & Destructor Documentation
◆ KGamePropertyHandler() [1/2]
|
explicit |
Construct an unregistered KGamePropertyHandler.
You have to call registerHandler before you can use this handler!
Definition at line 52 of file kgamepropertyhandler.cpp.
◆ KGamePropertyHandler() [2/2]
KGamePropertyHandler::KGamePropertyHandler | ( | int | id, |
const QObject * | receiver, | ||
const char * | sendf, | ||
const char * | emitf, | ||
QObject * | parent = nullptr ) |
Construct a registered handler.
- See also
- registerHandler
Definition at line 45 of file kgamepropertyhandler.cpp.
◆ ~KGamePropertyHandler()
|
override |
Definition at line 58 of file kgamepropertyhandler.cpp.
Member Function Documentation
◆ addProperty()
bool KGamePropertyHandler::addProperty | ( | KGamePropertyBase * | data, |
const QString & | name = QString() ) |
Adds a KGameProperty property to the handler.
- Parameters
-
data the property name A description of the property, which will be returned by propertyName. This is used for debugging
- Returns
- true on success
Definition at line 134 of file kgamepropertyhandler.cpp.
◆ clear()
void KGamePropertyHandler::clear | ( | ) |
Clear the KGamePropertyHandler.
Note that the properties are not deleted so if you created your KGameProperty objects dynamically like
you also have to delete it:
Definition at line 314 of file kgamepropertyhandler.cpp.
◆ Debug()
void KGamePropertyHandler::Debug | ( | ) |
Writes some debug output to the console.
Definition at line 366 of file kgamepropertyhandler.cpp.
◆ dict()
QMultiHash< int, KGamePropertyBase * > & KGamePropertyHandler::dict | ( | ) | const |
Reference to the internal dictionary.
Definition at line 330 of file kgamepropertyhandler.cpp.
◆ emitSignal()
void KGamePropertyHandler::emitSignal | ( | KGamePropertyBase * | data | ) |
called by a property to emit a signal This call is simply forwarded to the parent object
Definition at line 284 of file kgamepropertyhandler.cpp.
◆ find()
KGamePropertyBase * KGamePropertyHandler::find | ( | int | id | ) |
- Parameters
-
id The ID of the property. See KGamePropertyBase::id
- Returns
- The KGameProperty this ID is assigned to
Definition at line 307 of file kgamepropertyhandler.cpp.
◆ flush()
void KGamePropertyHandler::flush | ( | ) |
Sends all properties which are marked dirty over the network.
This will make a forced synchronization of the properties and mark them all not dirty.
Definition at line 252 of file kgamepropertyhandler.cpp.
◆ id()
int KGamePropertyHandler::id | ( | ) | const |
- Returns
- the id of the handler
Definition at line 65 of file kgamepropertyhandler.cpp.
◆ load()
|
virtual |
Loads properties from the datastream.
- Parameters
-
stream the datastream to load from
- Returns
- true on success otherwise false
Definition at line 171 of file kgamepropertyhandler.cpp.
◆ lockDirectEmit()
void KGamePropertyHandler::lockDirectEmit | ( | ) |
Called by the KGame or KPlayer object or the handler itself to delay emitting of signals.
Locking keeps a counter and unlock is only achieved when every lock is canceled by an unlock. While this is set signals are queued and only emitted after this is reset. Its deeper meaning is to prevent inconsistencies in a game load or network transfer where a emit could access a property not yet loaded or transmitted. Calling this by yourself you better know what your are doing.
Definition at line 266 of file kgamepropertyhandler.cpp.
◆ lockProperties()
void KGamePropertyHandler::lockProperties | ( | ) |
Calls KGamePropertyBase::setReadOnly(true) for all properties of this handler.
Use with care! This will even lock the core properties, like name, group and myTurn!!
- See also
- unlockProperties
Definition at line 238 of file kgamepropertyhandler.cpp.
◆ policy()
KGamePropertyBase::PropertyPolicy KGamePropertyHandler::policy | ( | ) |
Returns the default policy for this property handler.
All properties registered newly, will have this property.
Definition at line 210 of file kgamepropertyhandler.cpp.
◆ processMessage()
bool KGamePropertyHandler::processMessage | ( | QDataStream & | stream, |
int | id, | ||
bool | isSender ) |
Main message process function.
This has to be called by the parent's message event handler. If the id of the message agrees with the id of the handler, the message is extracted and processed. Otherwise false is returned. Example:
- Parameters
-
stream The data stream containing the message id the message id of the message isSender Whether the receiver is also the sender
- Returns
- true on message processed otherwise false
Definition at line 86 of file kgamepropertyhandler.cpp.
◆ propertyName()
QString KGamePropertyHandler::propertyName | ( | int | id | ) | const |
- Parameters
-
id The ID of the property
- Returns
- A name of the property which can be used for debugging. Don't depend on this function! It it possible not to provide a name or to provide the same name for multiple properties!
Definition at line 155 of file kgamepropertyhandler.cpp.
◆ propertyValue()
QString KGamePropertyHandler::propertyValue | ( | KGamePropertyBase * | property | ) |
In several situations you just want to have a QString of a KGameProperty object.
This function will provide you with such a QString for all the types used inside of all KGame classes. If you have a non-standard property (probably a self defined class or something like this) you also need to connect to signalRequestValue to make this function useful.
- Parameters
-
property Return the value of this KGameProperty
- Returns
- The value of a KGameProperty
Definition at line 335 of file kgamepropertyhandler.cpp.
◆ registerHandler()
void KGamePropertyHandler::registerHandler | ( | int | id, |
const QObject * | receiver, | ||
const char * | send, | ||
const char * | emit ) |
Register the handler with a parent.
This is to use if the constructor without arguments has been chosen. Otherwise you need not call this.
- Parameters
-
id The id of the message to listen for receiver The object that will receive the signals of KGamePropertyHandler send A slot that is being connected to signalSendMessage emit A slot that is being connected to signalPropertyChanged
Definition at line 75 of file kgamepropertyhandler.cpp.
◆ removeProperty()
bool KGamePropertyHandler::removeProperty | ( | KGamePropertyBase * | data | ) |
Removes a property from the handler.
- Parameters
-
data the property
- Returns
- true on success
Definition at line 124 of file kgamepropertyhandler.cpp.
◆ save()
|
virtual |
Saves properties into the datastream.
- Parameters
-
stream the datastream to save to
- Returns
- true on success otherwise false
Definition at line 193 of file kgamepropertyhandler.cpp.
◆ sendProperty()
bool KGamePropertyHandler::sendProperty | ( | QDataStream & | s | ) |
called by a property to send itself into the datastream.
This call is simply forwarded to the parent object
Definition at line 300 of file kgamepropertyhandler.cpp.
◆ setId()
void KGamePropertyHandler::setId | ( | int | id | ) |
Use id as new ID for this KGamePropertyHandler.
This is used internally only.
Definition at line 70 of file kgamepropertyhandler.cpp.
◆ setPolicy()
void KGamePropertyHandler::setPolicy | ( | KGamePropertyBase::PropertyPolicy | p, |
bool | userspace = true ) |
Set the policy for all kgame variables which are currently registered in the KGame property handler.
See KGamePropertyBase::setPolicy
- Parameters
-
p is the new policy for all properties of this handler userspace if userspace is true (default) only user properties are changed. Otherwise the system properties are also changed.
Definition at line 215 of file kgamepropertyhandler.cpp.
◆ signalPropertyChanged
|
signal |
This is emitted by a property.
KGamePropertyBase::emitSignal calls emitSignal which emits this signal.
This signal is emitted whenever the property is changed. Note that you can switch off this behaviour using KGamePropertyBase::setEmittingSignal in favor of performance. Note that you won't experience any performance loss using signals unless you use dozens or hundreds of properties which change very often.
◆ signalRequestValue
|
signal |
If you call propertyValue with a non-standard KGameProperty it is possible that the value cannot automatically be converted into a QString.
Then this signal is emitted and asks you to provide the correct value. You probably want to use something like this to achieve this:
- Parameters
-
property The KGamePropertyBase the value is requested for value The value of this property. You have to set this.
◆ signalSendMessage
|
signal |
This signal is emitted when a property needs to be sent.
Only the parent has to react to this.
- Parameters
-
msgid The id of the handler sent set this to true if the property was sent successfully - otherwise don't touch
◆ uniquePropertyId()
int KGamePropertyHandler::uniquePropertyId | ( | ) |
returns a unique property ID starting called usually with a base of KGamePropertyBase::IdAutomatic.
This is used internally by the property base to assign automatic id's. Not much need to call this yourself.
Definition at line 247 of file kgamepropertyhandler.cpp.
◆ unlockDirectEmit()
void KGamePropertyHandler::unlockDirectEmit | ( | ) |
Removes the lock from the emitting of property signals.
Corresponds to the lockIndirectEmits
Definition at line 271 of file kgamepropertyhandler.cpp.
◆ unlockProperties()
void KGamePropertyHandler::unlockProperties | ( | ) |
Calls KGamePropertyBase::setReadOnly(false) for all properties of this player.
See also lockProperties
Definition at line 229 of file kgamepropertyhandler.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:57:56 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.