KGameProperty
#include <KGame/KGameProperty>
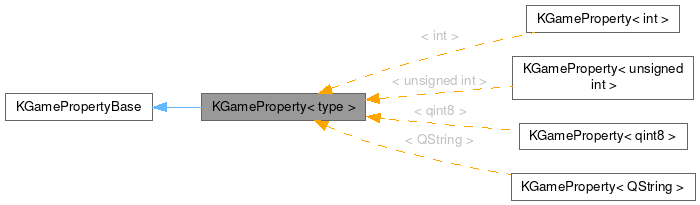
Public Member Functions | |
KGameProperty () | |
KGameProperty (int id, KGamePropertyHandler *owner) | |
void | changeValue (type v) |
void | load (QDataStream &s) override |
operator type () const | |
const type & | operator= (const KGameProperty &property) |
const type & | operator= (const type &t) |
void | save (QDataStream &stream) override |
bool | send (type v) |
bool | setLocal (type v) |
void | setValue (type v) |
const type_info * | typeinfo () override |
const type & | value () const |
![]() | |
KGamePropertyBase () | |
KGamePropertyBase (int id, KGame *parent) | |
KGamePropertyBase (int id, KGamePropertyHandler *owner) | |
KGamePropertyBase (int id, KPlayer *parent) | |
virtual void | command (QDataStream &stream, int msgid, bool isSender=false) |
int | id () const |
bool | isDirty () const |
bool | isEmittingSignal () const |
bool | isLocked () const |
bool | isOptimized () const |
bool | lock () |
PropertyPolicy | policy () const |
int | registerData (int id, KGame *owner, const QString &name=QString()) |
int | registerData (int id, KGamePropertyHandler *owner, const QString &name=QString()) |
int | registerData (int id, KGamePropertyHandler *owner, PropertyPolicy p, const QString &name=QString()) |
int | registerData (int id, KPlayer *owner, const QString &name=QString()) |
int | registerData (KGamePropertyHandler *owner, PropertyPolicy p=PolicyUndefined, const QString &name=QString()) |
void | setEmittingSignal (bool p) |
void | setOptimized (bool p) |
void | setPolicy (PropertyPolicy p) |
bool | unlock (bool force=false) |
void | unregisterData () |
Additional Inherited Members | |
![]() | |
enum | PropertyCommandIds { CmdLock = 1 , CmdAt = 51 , CmdResize = 52 , CmdFill = 53 , CmdSort = 54 , CmdInsert = 61 , CmdAppend = 62 , CmdRemove = 63 , CmdClear = 64 } |
enum | PropertyDataIds { IdGroup = 1 , IdUserId = 2 , IdAsyncInput = 3 , IdTurn = 4 , IdName = 5 , IdGameStatus = 6 , IdMaxPlayer = 7 , IdMinPlayer = 8 , IdGrabInput = 16 , IdReleaseInput = 17 , IdCommand , IdUser = 256 , IdAutomatic = 0x7000 } |
enum | PropertyPolicy { PolicyUndefined = 0 , PolicyClean = 1 , PolicyDirty = 2 , PolicyLocal = 3 } |
![]() | |
void | emitSignal () |
bool | sendProperty () |
bool | sendProperty (const QByteArray &b) |
void | setDirty (bool d) |
void | setLock (bool l) |
![]() | |
union KGamePropertyBase::Flags | mFlags |
KGamePropertyHandler * | mOwner |
Detailed Description
class KGameProperty< type >
A class for network transparent games.
Note: The entire API documentation is obsolete!
The class KGameProperty can store any form of data and will transmit it via network whenever you call send. This makes network transparent games very easy. You first have to register the data to a KGamePropertyHandler using KGamePropertyBase::registerData (which is called by the constructor). For the KGamePropertyHandler you can use KGame::dataHandler or KPlayer::dataHandler but you can also create your own data handler.
There are several concepts you can follow when writing network games. These concepts differ completely from the way how data is transferred so you should decide which one to use. You can also mix these concepts for a single property but we do not recommend this. The concepts:
- Always Consistent (clean)
- Not Always Consistent (dirty)
- A Mixture (very dirty)
I repeat: we do not recommend the third option ("a mixture"). Unless you have a good reason for this you will probably introduce some hard to find (and to fix) bugs.
consistent (clean):
This "policy" is default. Whenever you create a KGameProperty it is always consistent. This means that consistency is the most important thing for the property. This is achieved by using send to change the value of the property. send needs a running KMessageServer and therefore MUST be plugged into a KGamePropertyHandler using either registerData or the constructor. The parent of the dataHandler must be able to send messages (see above: the message server must be running). If you use send to change the value of a property you won't see the effect immediately: The new value is first transferred to the message server which queues the message. As soon as all messages in the message server which are before the changed property have been transferred the message server delivers the new value of the KGameProperty to all clients. A QTimer::singleShot is used to queue the messages inside the KMessageServer.
This means that if you do the following:
then "value" will be "0". initData is used to initialize the property (e.g. when the KMessageServer is not yet running or can not yet be reached). This is because "myProperty = 10" or "myProperty.send(10)" send a message to the KMessageServer which uses QTimer::singleShot to queue the message. The game first has to go back into the event loop where the message is received. The KGamePropertyHandler receives the new value sets the property. So if you need the new value you need to store it in a different variable (see setLocal which creates one for you until the message is received). The KGamePropertyHandler emits a signal (unless you called setEmitSignal with false) when the new value is received: KGamePropertyHandler::signalPropertyChanged. You can use this to react to a changed property.
This may look quite confusing but it has a big advantage: all KGameProperty objects are ensured to have the same value on all clients in the game at every time. This way you will save you a lot of trouble as debugging can be very difficult if the value of a property changes immediately on client A but only after one or two additional messages (function calls, status changes, ...) on client B.
The only disadvantage of this (clean) concept is that you cannot use a changed variable immediately but have to wait for the KMessageServer to change it. You probably want to use KGamePropertyHandler::signalPropertyChanged for this.
Always Consistent (dirty):
There are a lot of people who don't want to use the (sometimes quite complex) "clean" way. You can use setAlwaysConsistent to change the default behaviour of the KGameProperty. If a property is not always consistent it will use changeValue to send the property. changeValue also uses send to send the new value over network but it also uses setLocal to create a local copy of the property. This copy is created dynamically and is deleted again as soon as the next message from the network is received. To use the example above again:
Now this example will "work" so value now is 10. Additionally the KMessageServer receives a message from the local client (just as explained above in "Always Consistent"). As soon as the message returns to the local client again the local value is deleted, as the "network value" has the same value as the local one. So you won't lose the ability to use the always consistent "clean" value of the property if you use the "dirty" way. Just use networkValue to access the value which is consistent among all clients.
The advantage of this concept is clear: you can use a KGameProperty as every other variable as the changes value takes immediate effect. Additionally you can be sure that the value is transferred to all clients. You will usually not experience serious bugs just because you use the "dirty" way. Several events have to happen at once to get these "strange errors" which result in inconsistent properties (like "game running" on client A but "game ended/paused" on client B). But note that there is a very good reason for the existence of these different concepts of KGameProperty. I have myself experienced such a "strange error" and it took me several days to find the reason until I could fix it. So I personally recommend the "clean" way. On the other hand if you want to port a non-network game to a network game you will probably start with "dirty" properties as it is you will not have to change that much code...
Mixture (very dirty):
You can also mix the concepts above. Note that we really don't recommend this. With a mixture I mean something like this:
(totally senseless example, btw) I.e. I am speaking of mixing both concepts for a single property. Things like
are ok. But mixing the concepts for a single property will make it nearly impossible to you to debug your game.
So the right thing to do(tm) is to decide in the constructor whether you want a "clean" or "dirty" property.
Even if you have decided for one of the concepts you still can manually follow another concept than the "policy" of your property. So if you use an always consistent KGameProperty you still can manually call changeValue as if it was not always consistent. Note that although this is also kind of a "mixture" as described above this is very useful sometimes. In contrast to the "mixture" above you don't have the problem that you don't exactly know which concept you are currently following because you used the function of the other concept only once.
classes:
If you want to use a custom class with KGameProperty you have to implement the operators << and >> for QDataStream:
Note: unlike most QT classes KGameProperty objects are not deleted automatically! So if you create an object using e.g. KGameProperty<int>* data = new KGameProperty(id, dataHandler()) you have to put a delete data into your destructor!
Definition at line 605 of file kgameproperty.h.
Constructor & Destructor Documentation
◆ KGameProperty() [1/2]
|
inline |
Constructs a KGameProperty object.
A KGameProperty object will transmit any changes to the KMessageServer and then to all clients in the game (including the one that has sent the new value)
- Parameters
-
id The id of this property. MUST be UNIQUE! Used to send and receive changes in the property of the playere automatically via network. owner The parent of the object. Must be a KGame which manages the changes made to this object, i.e. which will send the new data. Note that in contrast to most KDE/QT classes KGameProperty objects are not deleted automatically!
Definition at line 621 of file kgameproperty.h.
◆ KGameProperty() [2/2]
|
inline |
This constructor does nothing.
You have to call KGamePropertyBase::registerData yourself before using the KGameProperty object.
Definition at line 632 of file kgameproperty.h.
◆ ~KGameProperty()
|
inlineoverride |
Definition at line 638 of file kgameproperty.h.
Member Function Documentation
◆ changeValue()
|
inline |
This function does both, change the local value and change the network value.
The value is sent over network first, then changed locally.
This function is a convenience function and just calls send followed by setLocal
Note that emitSignal is also called twice: once after setLocal and once when the value from send is received
Definition at line 777 of file kgameproperty.h.
◆ load()
|
inlineoverridevirtual |
Reads from a stream and assigns the read value to this object.
This function is called automatically when a new value is received over network (i.e. it has been sent using send on this or any other client) or when a game is loaded (and maybe on some other events).
Also calls emitSignal if isEmittingSignal is TRUE.
- Parameters
-
s The stream to read from
Implements KGamePropertyBase.
Definition at line 813 of file kgameproperty.h.
◆ operator type()
|
inline |
Yeah, you can do it!
If you don't see it: you don't have to use integerData.value()
Definition at line 864 of file kgameproperty.h.
◆ operator=() [1/2]
|
inline |
This copies the data of property to the KGameProperty object.
Equivalent to setValue(property.value());
Definition at line 851 of file kgameproperty.h.
◆ operator=() [2/2]
|
inline |
This calls setValue to change the value of the property.
Note that depending on the policy (see setAlwaysConsistent) the returned value might be different from the assigned value!!
So if you use setPolicy(PolicyClean):
Here a and b would differ! The value is actually set as soon as it is received from the KMessageServer which forwards it to ALL clients in the network.
If you use a clean policy (see setPolicy) then the returned value is the assigned value
Definition at line 840 of file kgameproperty.h.
◆ save()
|
inlineoverridevirtual |
Saves the object to a stream.
- Parameters
-
stream The stream to save to
Implements KGamePropertyBase.
Definition at line 787 of file kgameproperty.h.
◆ send()
|
inline |
This function sends a new value over network.
Note that the value DOES NOT change when you call this function. This function saves the value into a QDataStream and calls sendProperty where it gets forwarded to the owner and finally the value is sent over network. The KMessageServer now sends the value to ALL clients - even the one who called this function. As soon as the value from the message server is received load is called and then the value of the KGameProperty has been set.
This ensures that a KGameProperty has always the same value on every client in the network. Note that this means you can NOT do something like
as myProperty has not yet been set when doSomething is being called.
You are informed about a value change by a signal from the parent of the property which can be deactivated by setEmittingSignal because of performance (you probably don't have to deactivate it - except you want to write a real-time game like Command&Conquer with a lot of activity). See emitSignal
Note that if there is no KMessageServer accessible - before the property has been registered to the KGamePropertyHandler (as it is the case e.g. before a KPlayer has been plugged into the KGame object) the property is not sent but set locally (see setLocal)!
- Parameters
-
v The new value of the property
- Returns
- whether the property could be sent successfully
- See also
- setValue setLocal changeValue value
Definition at line 703 of file kgameproperty.h.
◆ setLocal()
|
inline |
This function sets the value of the property directly, i.e.
it doesn't send it to the network.
Int contrast to
- See also
- you change only the local value when using this function. You do not change the value of any other client. You probably don't want to use this if you are using a dedicated server (which is the only "client" which is allowed to change a value) but rather want to use send().
But if you use your clients as servers (i.e. all clients receive a players turn and then calculate the reaction of the game theirselves) then you probably want to use setLocal as you can do things like
on every client.
If you want to set the value locally AND send it over network you want to call changeValue!
You can also use setPolicy to set the default policy to PolicyLocal.
- See also
- setValue send changeValue value
Definition at line 748 of file kgameproperty.h.
◆ setValue()
|
inline |
Set the value depending on the current policy (see setConsistent).
By default KGameProperty just uses send to set the value of a property. This behaviour can be changed by using setConsistent.
- Parameters
-
v The new value of the property
Definition at line 649 of file kgameproperty.h.
◆ typeinfo()
|
inlineoverridevirtual |
- Returns
- a type_info of the data this property contains.
Reimplemented from KGamePropertyBase.
Definition at line 869 of file kgameproperty.h.
◆ value()
|
inline |
- Returns
- The local value (see setLocal) if it is existing, otherwise the network value which is always consistent on every client.
Definition at line 797 of file kgameproperty.h.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:57:56 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.