Marble::GeoGraphicsScene
#include <GeoGraphicsScene.h>
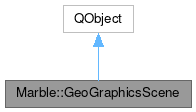
Signals | |
void | repaintNeeded () |
Public Slots | |
void | applyHighlight (const QList< GeoDataPlacemark * > &) |
Public Member Functions | |
GeoGraphicsScene (QObject *parent=nullptr) | |
void | addItem (GeoGraphicsItem *item) |
void | clear () |
QList< GeoGraphicsItem * > | items (const GeoDataLatLonBox &box, int maxZoomLevel) const |
void | removeItem (const GeoDataFeature *feature) |
void | resetStyle () |
QList< GeoGraphicsItem * > | selectedItems () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This is the home of all GeoGraphicsItems to be shown on the map.
Definition at line 28 of file GeoGraphicsScene.h.
Constructor & Destructor Documentation
◆ GeoGraphicsScene()
|
explicit |
Creates a new instance of GeoGraphicsScene.
- Parameters
-
parent the QObject parent of the Scene
Definition at line 76 of file GeoGraphicsScene.cpp.
◆ ~GeoGraphicsScene()
|
override |
Definition at line 82 of file GeoGraphicsScene.cpp.
Member Function Documentation
◆ addItem()
void Marble::GeoGraphicsScene::addItem | ( | GeoGraphicsItem * | item | ) |
Add an item to the GeoGraphicsScene Adds the item item
to the GeoGraphicsScene.
Definition at line 254 of file GeoGraphicsScene.cpp.
◆ applyHighlight
|
slot |
First set the items, which were selected previously, to use normal style
Process the placemark. which were under mouse while clicking, and update corresponding graphics items to use highlight style
If a placemark is using an inline style instead of a shared style ( e.g in case when theme file specifies the colorMap attribute ) then highlight it if any of the style maps have a highlight styleId
Definition at line 162 of file GeoGraphicsScene.cpp.
◆ clear()
void Marble::GeoGraphicsScene::clear | ( | ) |
Remove all items from the GeoGraphicsScene.
Definition at line 244 of file GeoGraphicsScene.cpp.
◆ items()
QList< GeoGraphicsItem * > Marble::GeoGraphicsScene::items | ( | const GeoDataLatLonBox & | box, |
int | maxZoomLevel ) const |
Get the list of items in the specified Box.
- Parameters
-
box The box around the items. maxZoomLevel The max zoom level of tiling
- Returns
- The list of items in the specified box in no specific order.
Definition at line 87 of file GeoGraphicsScene.cpp.
◆ removeItem()
void Marble::GeoGraphicsScene::removeItem | ( | const GeoDataFeature * | feature | ) |
Remove all concerned items from the GeoGraphicsScene Removes all items which are associated with object
from the GeoGraphicsScene.
Definition at line 228 of file GeoGraphicsScene.cpp.
◆ resetStyle()
void Marble::GeoGraphicsScene::resetStyle | ( | ) |
Definition at line 152 of file GeoGraphicsScene.cpp.
◆ selectedItems()
QList< GeoGraphicsItem * > Marble::GeoGraphicsScene::selectedItems | ( | ) | const |
Get the list of items which belong to a placemark that has been clicked.
- Returns
- Returns a list of selected Items
Definition at line 147 of file GeoGraphicsScene.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.