Marble::StackedTileLoader
#include <StackedTileLoader.h>
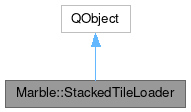
Signals | |
void | cleared () |
void | tileLoaded (TileId const &tileId) |
Public Member Functions | |
StackedTileLoader (MergedLayerDecorator *mergedLayerDecorator, QObject *parent=nullptr) | |
void | cleanupTilehash () |
void | clear () |
const StackedTile * | loadTile (TileId const &stackedTileId) |
RenderState | renderState () const |
void | resetTilehash () |
void | setVolatileCacheLimit (quint64 kiloBytes) |
int | tileColumnCount (int level) const |
int | tileCount () const |
const GeoSceneAbstractTileProjection * | tileProjection () const |
int | tileRowCount (int level) const |
QSize | tileSize () const |
void | updateTile (TileId const &tileId, QImage const &tileImage) |
QList< TileId > | visibleTiles () const |
quint64 | volatileCacheLimit () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Tile loading from a quad tree.
This class loads tiles into memory. For faster access we keep the tileIDs and their respective pointers to the tiles in a hashtable. The class also contains convenience methods to remove entries from the hashtable and to return more detailed properties about each tile level and their tiles.
Definition at line 44 of file StackedTileLoader.h.
Constructor & Destructor Documentation
◆ StackedTileLoader()
|
explicit |
Creates a new tile loader.
- Parameters
-
mergedLayerDecorator The decorator that shall be used to decorate the layer. parent The parent widget.
Definition at line 42 of file StackedTileLoader.cpp.
◆ ~StackedTileLoader()
|
override |
Definition at line 48 of file StackedTileLoader.cpp.
Member Function Documentation
◆ cleanupTilehash()
void Marble::StackedTileLoader::cleanupTilehash | ( | ) |
Cleans up the internal tile hash.
Removes all superfluous tiles from the hash.
Definition at line 84 of file StackedTileLoader.cpp.
◆ clear()
void Marble::StackedTileLoader::clear | ( | ) |
Effectively triggers a reload of all tiles that are currently in use and clears the tile cache in physical memory.
Definition at line 204 of file StackedTileLoader.cpp.
◆ loadTile()
const StackedTile * Marble::StackedTileLoader::loadTile | ( | TileId const & | stackedTileId | ) |
Loads a tile and returns it.
- Parameters
-
stackedTileId The Id of the requested tile, containing the x and y coordinate and the zoom level.
Definition at line 102 of file StackedTileLoader.cpp.
◆ renderState()
RenderState Marble::StackedTileLoader::renderState | ( | ) | const |
Definition at line 193 of file StackedTileLoader.cpp.
◆ resetTilehash()
void Marble::StackedTileLoader::resetTilehash | ( | ) |
Resets the internal tile hash.
Definition at line 74 of file StackedTileLoader.cpp.
◆ setVolatileCacheLimit()
void Marble::StackedTileLoader::setVolatileCacheLimit | ( | quint64 | kiloBytes | ) |
Set the limit of the volatile (in RAM) cache.
- Parameters
-
kiloBytes The limit in kilobytes.
Definition at line 166 of file StackedTileLoader.cpp.
◆ tileColumnCount()
int Marble::StackedTileLoader::tileColumnCount | ( | int | level | ) | const |
Definition at line 54 of file StackedTileLoader.cpp.
◆ tileCount()
int Marble::StackedTileLoader::tileCount | ( | ) | const |
Return the number of tiles in the cache.
- Returns
- number of tiles in cache
Definition at line 161 of file StackedTileLoader.cpp.
◆ tileProjection()
const GeoSceneAbstractTileProjection * Marble::StackedTileLoader::tileProjection | ( | ) | const |
Definition at line 64 of file StackedTileLoader.cpp.
◆ tileRowCount()
int Marble::StackedTileLoader::tileRowCount | ( | int | level | ) | const |
Definition at line 59 of file StackedTileLoader.cpp.
◆ tileSize()
QSize Marble::StackedTileLoader::tileSize | ( | ) | const |
Definition at line 69 of file StackedTileLoader.cpp.
◆ updateTile()
void Marble::StackedTileLoader::updateTile | ( | TileId const & | tileId, |
QImage const & | tileImage ) |
Definition at line 172 of file StackedTileLoader.cpp.
◆ visibleTiles()
QList< TileId > Marble::StackedTileLoader::visibleTiles | ( | ) | const |
Reloads the tiles that are currently displayed.
Definition at line 156 of file StackedTileLoader.cpp.
◆ volatileCacheLimit()
quint64 Marble::StackedTileLoader::volatileCacheLimit | ( | ) | const |
Returns the limit of the volatile (in RAM) cache.
- Returns
- the cache limit in kilobytes
Definition at line 151 of file StackedTileLoader.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.